Using the NetHunt API to Create Records in PHP
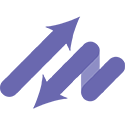
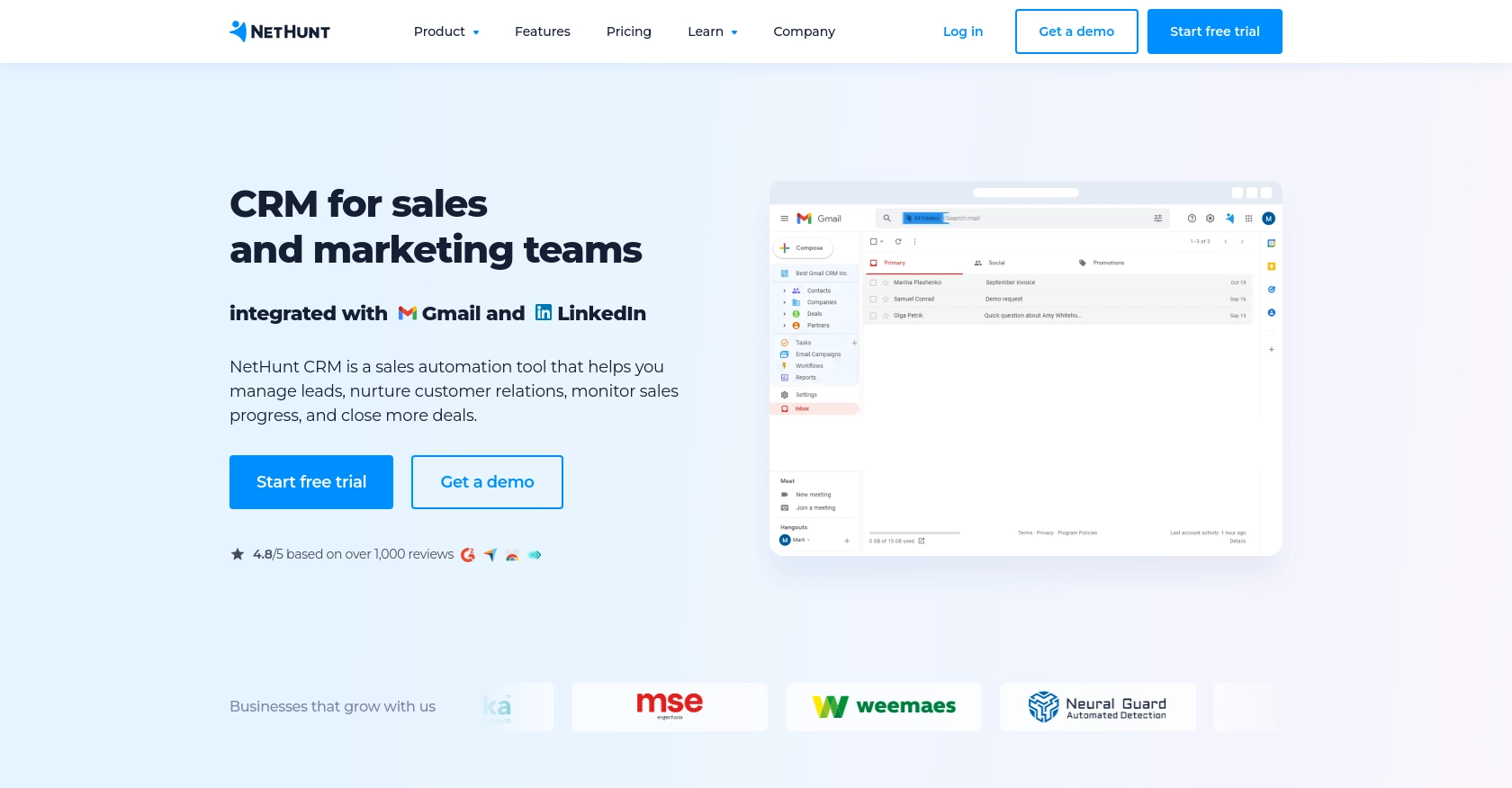
Introduction to NetHunt CRM
NetHunt CRM is a powerful customer relationship management tool that seamlessly integrates with Gmail, providing businesses with a comprehensive solution for managing customer interactions and data. With features like email campaigns, lead management, and sales automation, NetHunt CRM is designed to enhance productivity and streamline business processes.
Developers may want to integrate with NetHunt's API to automate tasks such as creating and managing records within the CRM. For example, you could use the API to automatically create new customer records from an external data source, ensuring that your CRM is always up-to-date with the latest information.
Setting Up Your NetHunt CRM Account for API Access
Before you can start integrating with the NetHunt API, you need to set up your NetHunt CRM account and obtain the necessary API key. This key will allow you to authenticate your requests and interact with the CRM system programmatically.
Creating a NetHunt CRM Account
If you don't already have a NetHunt CRM account, you can sign up for a free trial on the NetHunt website. Follow the instructions to create your account and log in to the NetHunt dashboard.
Generating Your NetHunt API Key
To interact with the NetHunt API, you'll need to generate an API key. This key acts as a password for your API requests, ensuring secure communication between your application and NetHunt CRM.
- Log in to your NetHunt CRM account.
- Navigate to the Settings menu.
- Select the Integrations option.
- Find the section for API access and click on Generate API Key.
- Copy the generated API key and store it securely, as you'll need it for authentication in your API requests.
For more detailed instructions, you can refer to the official documentation on where to get the NetHunt API key.
Understanding NetHunt API Authentication
NetHunt uses a custom authentication method that requires a combination of your email and API key. This combination is encoded in base64 and included in the request headers. Here’s how you can set it up:
// Example of setting up authentication headers in PHP
$email = 'your-email@example.com';
$apiKey = 'your-api-key';
$credentials = base64_encode("$email:$apiKey");
$headers = [
'Authorization: Basic ' . $credentials,
'Content-Type: application/json'
];
Ensure you replace your-email@example.com
and your-api-key
with your actual email and API key.
For more information on authentication, visit the NetHunt API documentation.
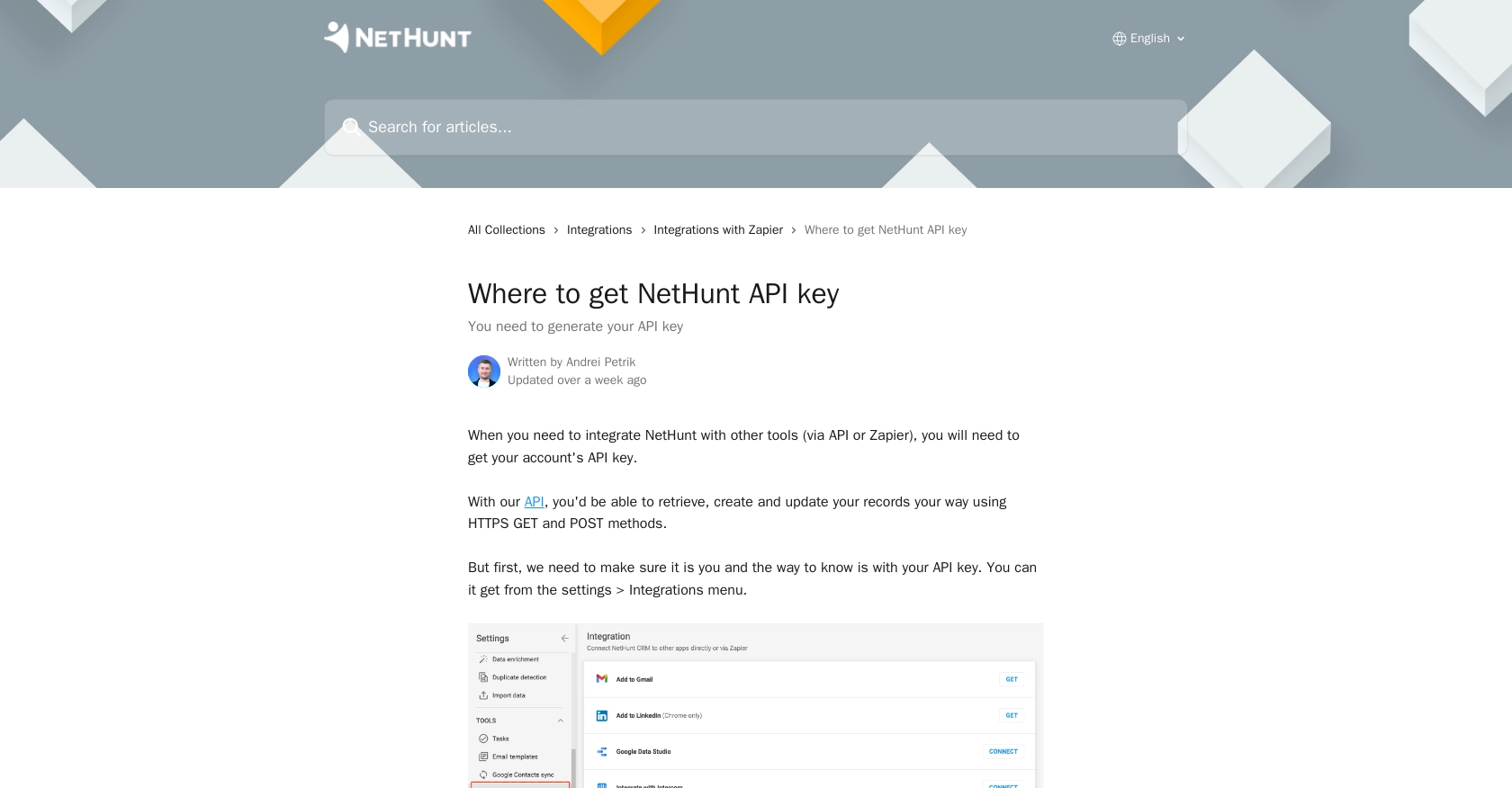
sbb-itb-96038d7
Making API Calls to Create Records in NetHunt Using PHP
To create records in NetHunt CRM using PHP, you'll need to make HTTP POST requests to the appropriate API endpoint. This section will guide you through setting up your PHP environment, writing the code to make the API call, and handling the response.
Setting Up Your PHP Environment
Before you begin, ensure you have PHP installed on your machine. You can download it from the official PHP website. Additionally, you'll need the cURL
extension enabled, which is commonly included in most PHP installations.
Creating a New Record in NetHunt CRM
To create a new record, you'll need to specify the folder ID where the record will be stored and the data fields for the record. Here's a step-by-step guide:
// Define your API endpoint and folder ID
$folderId = 'your-folder-id';
$url = "https://nethunt.com/api/v1/zapier/actions/create-record/$folderId";
// Set up the data for the new record
$data = [
'timeZone' => 'Europe/London',
'fields' => [
'Name' => 'John Doe',
'Birthday' => '1980-01-01',
'Employed' => true,
'Salary' => 100000,
'Primary Email Address' => 'john.doe@example.com'
]
];
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Parse and display the response
$result = json_decode($response, true);
echo 'Record Created: ' . $result['recordId'];
}
// Close cURL session
curl_close($ch);
Replace your-folder-id
with the actual folder ID where you want to create the record. The $headers
variable should contain your authentication headers as described in the previous section.
Verifying the API Request Success
After executing the API call, you can verify the success of the request by checking the response. A successful creation will return a recordId
in the response. You can also log in to your NetHunt CRM account to confirm the new record appears in the specified folder.
Handling Errors and Common Error Codes
It's essential to handle potential errors when making API calls. Common error codes include:
- 400 Bad Request: The request was invalid or cannot be served. Check the request data.
- 401 Unauthorized: Authentication failed. Verify your API key and credentials.
- 404 Not Found: The specified folder ID does not exist.
Ensure you have proper error handling in place to manage these scenarios effectively.
Best Practices for Using NetHunt API in PHP
When integrating with the NetHunt API, it's crucial to follow best practices to ensure secure and efficient interactions. Here are some recommendations:
- Securely Store API Credentials: Always store your API key and credentials securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Implement Rate Limiting: Be mindful of the API rate limits to prevent your application from being throttled. Implement logic to handle rate limit responses and retry requests as needed.
- Standardize Data Fields: Ensure that the data fields you send to NetHunt are standardized and validated to prevent errors and maintain data integrity.
Leveraging Endgrate for Seamless NetHunt Integrations
Building and maintaining integrations can be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including NetHunt. This allows you to focus on your core product while outsourcing integration complexities.
With Endgrate, you can build once for each use case and apply it across different integrations, saving time and resources. Take advantage of an intuitive integration experience that enhances your product's capabilities.
Visit Endgrate to learn more about how you can streamline your integration processes and deliver a seamless experience to your customers.
Read More
Ready to get started?