Using the Sage 100 API to Get Customers in Python
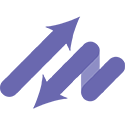
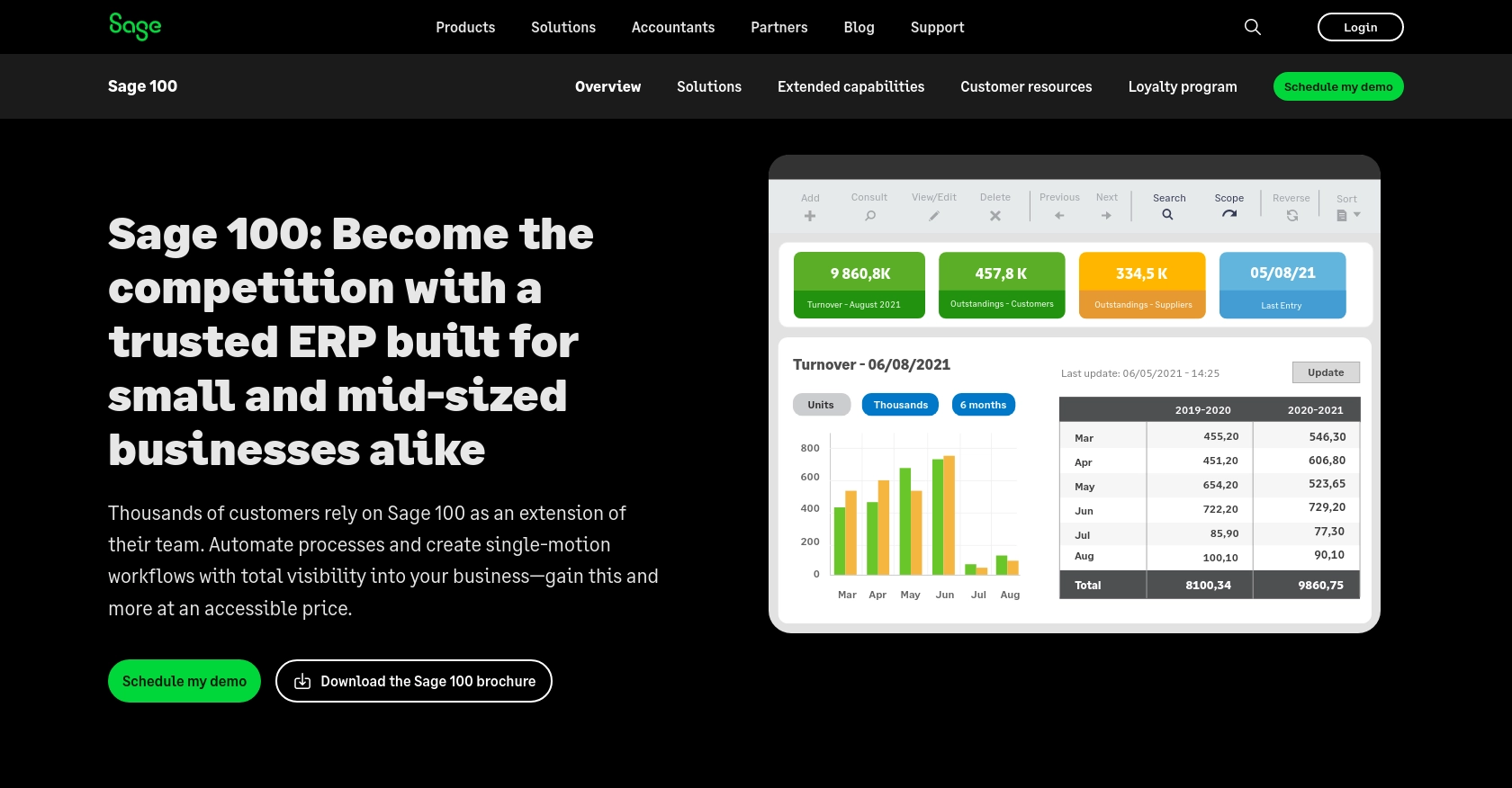
Introduction to Sage 100 API
Sage 100 is a comprehensive ERP solution designed to streamline business operations across various departments, including accounting, inventory management, and customer relationship management. It is widely used by small to medium-sized businesses to enhance efficiency and productivity.
Integrating with the Sage 100 API allows developers to access and manage critical business data programmatically. For example, using the Sage 100 API to retrieve customer information can help automate processes such as generating customer reports or syncing customer data with other applications.
Setting Up Your Sage 100 Test Environment
Before you can start interacting with the Sage 100 API, it's essential to set up a test environment. This involves configuring the Sage 100 ODBC driver to connect to the Sage 100 database, allowing you to perform queries and manipulate data programmatically.
Installing and Configuring the Sage 100 ODBC Driver
To begin, ensure that the Sage 100 ODBC driver is installed on your system. This driver is crucial for establishing a connection to the Sage 100 database. Follow these steps to configure the driver:
- Access the ODBC Data Source Administrator on your system.
- Create a Data Source Name (DSN) that connects to the Sage 100 ERP system. Ensure you use the correct server, database, and authentication settings.
- Configure the DSN to point to the Sage 100 database you wish to access.
For detailed instructions, refer to the Sage 100 ODBC Driver Configuration Guide.
Creating a Sage 100 Test Account
To interact with the Sage 100 API, you may need to create a test account within the Sage 100 environment. This allows you to safely test API calls without affecting live data. Follow these steps:
- Log in to your Sage 100 system as an administrator.
- Navigate to the user management section and create a new user account designated for testing purposes.
- Assign appropriate permissions to this account, ensuring it has access to the necessary modules and data for testing.
Configuring Sage 100 for API Access
Once your test account is set up, configure Sage 100 to allow API access:
- In Sage 100, go to Library Master > Setup > System Configuration.
- On the ODBC Driver tab, select the Enable C/S ODBC Driver checkbox.
- Enter the server name or IP address where the client/server ODBC application or service is running.
- Specify the server port, or leave it blank to use the default port, 20222.
- Ensure the ODBC driver is enabled for the test user account.
For more detailed setup instructions, visit the official Sage 100 documentation.
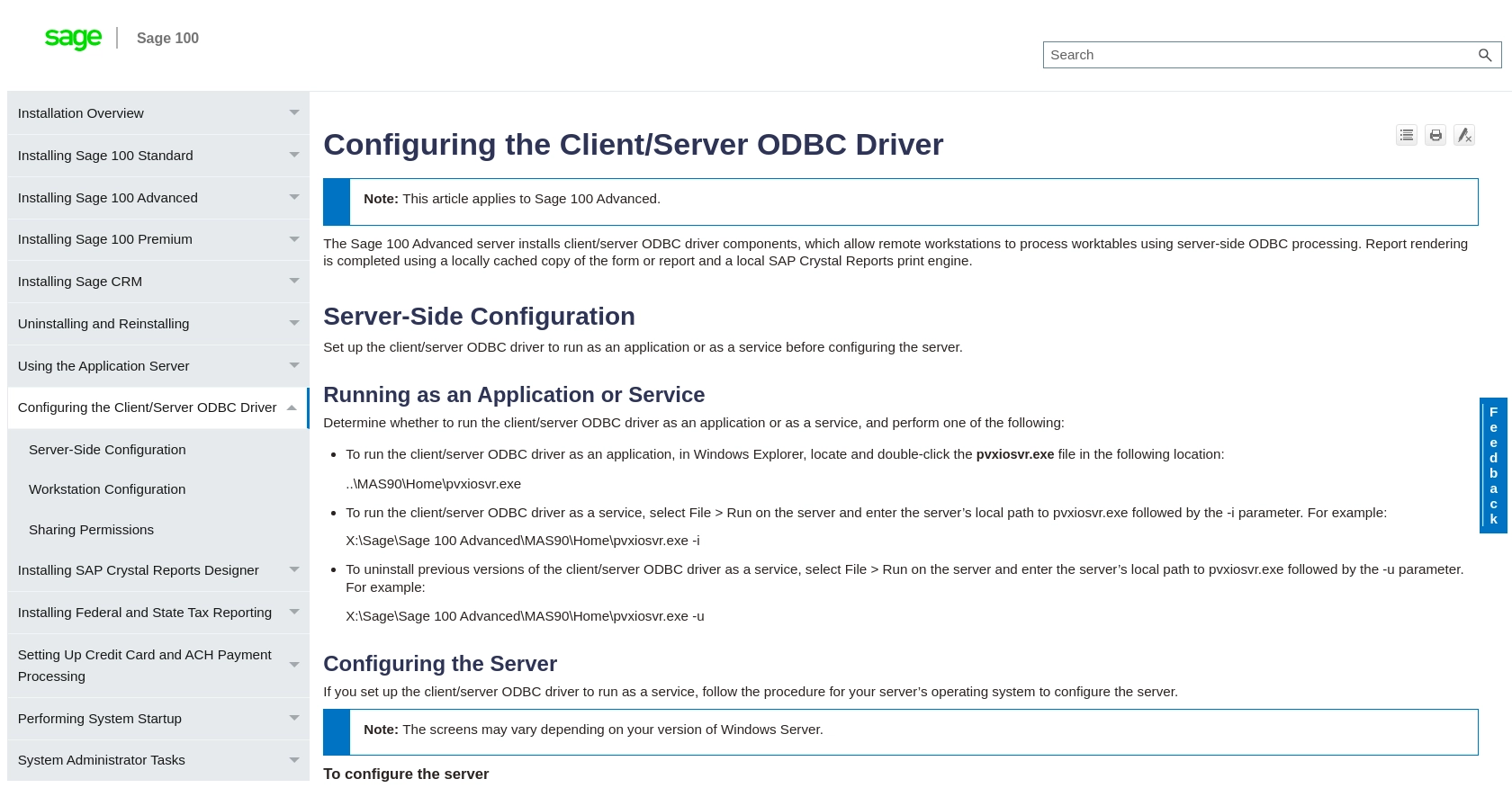
sbb-itb-96038d7
Making API Calls to Retrieve Customers from Sage 100 Using Python
To interact with the Sage 100 API and retrieve customer data, you'll need to use Python along with the ODBC driver configured earlier. This section will guide you through the process of setting up your Python environment, writing the code to make the API call, and handling the response.
Setting Up Your Python Environment for Sage 100 API Integration
Before making API calls, ensure you have Python installed on your system. This tutorial uses Python 3.11.1. Additionally, you'll need the pyodbc
library to interact with the ODBC driver.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Open your terminal or command prompt and install the
pyodbc
library using pip:
pip install pyodbc
Writing Python Code to Connect to Sage 100 and Retrieve Customer Data
With your environment set up, you can now write the Python code to connect to Sage 100 and retrieve customer information. Follow these steps:
import pyodbc # Define the DSN and connection parameters dsn = 'Your_DSN_Name' user = 'Your_Username' password = 'Your_Password' # Establish a connection to the Sage 100 database connection = pyodbc.connect(f'DSN={dsn};UID={user};PWD={password}') # Create a cursor object to execute SQL queries cursor = connection.cursor() # Define the SQL query to retrieve customer data query = "SELECT * FROM AR_Customer" # Execute the query cursor.execute(query) # Fetch and print the results for row in cursor.fetchall(): print(row) # Close the connection connection.close()
Replace Your_DSN_Name
, Your_Username
, and Your_Password
with your actual DSN, username, and password.
Verifying the API Call and Handling Errors
After running the script, you should see the customer data printed in your terminal. To verify the request succeeded, cross-check the output with the data in your Sage 100 test environment.
Handle potential errors using try-except blocks to manage connection issues or query failures:
try: # Connection and query code here except pyodbc.Error as e: print(f"Error: {e}") finally: connection.close()
For more information on error codes, refer to the Sage 100 API documentation.
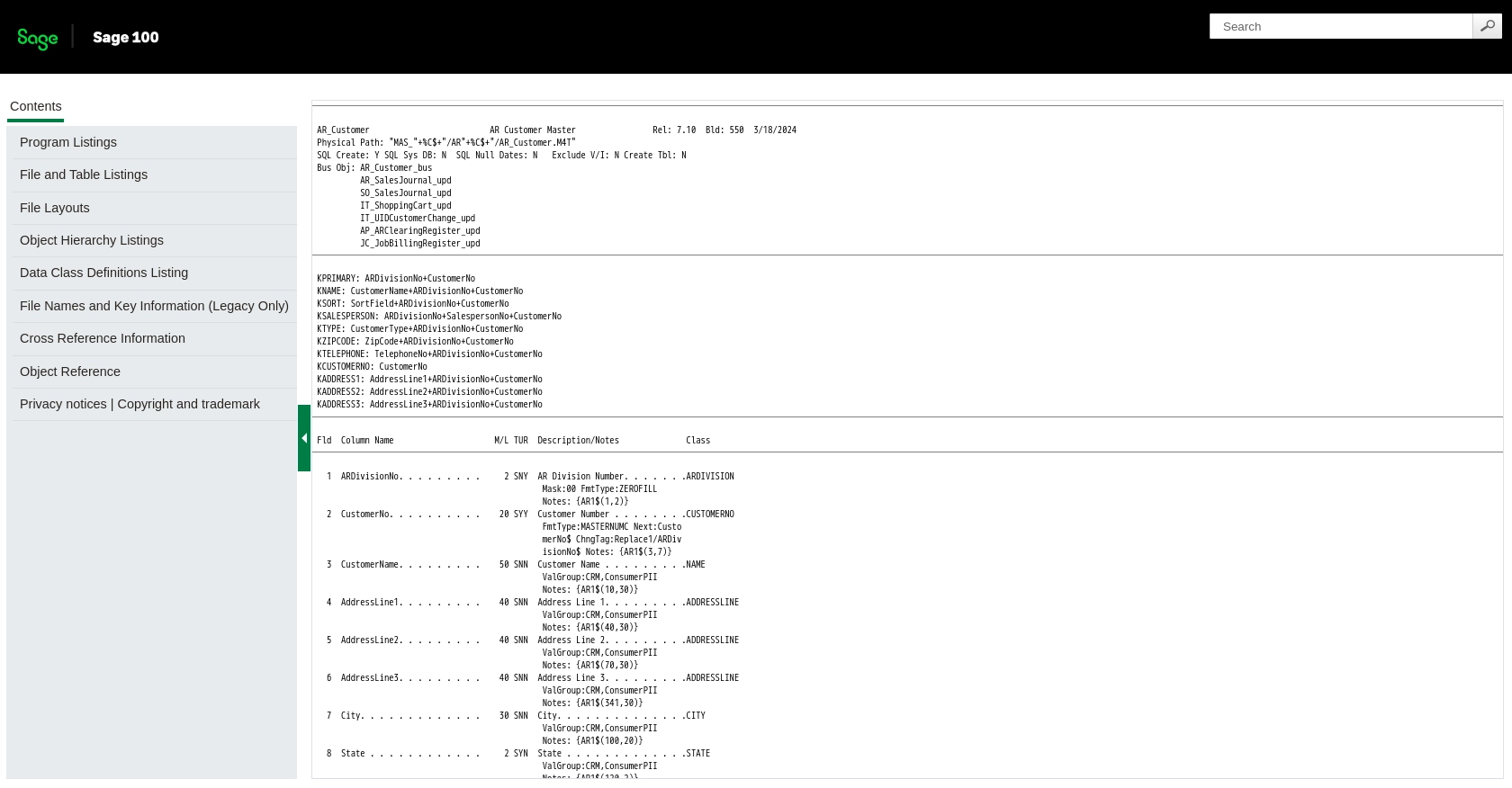
Conclusion and Best Practices for Using the Sage 100 API in Python
Integrating with the Sage 100 API using Python provides a powerful way to automate and streamline business processes by accessing critical customer data. By following the steps outlined in this guide, developers can efficiently connect to the Sage 100 database and perform necessary operations.
Best Practices for Secure and Efficient Sage 100 API Integration
- Secure Storage of Credentials: Always store your DSN, username, and password securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Sage 100 API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data retrieved from Sage 100 is standardized and transformed as needed to fit into your application's data model.
- Error Handling: Use try-except blocks to manage exceptions and log errors for troubleshooting and monitoring purposes.
Streamlining Integrations with Endgrate
While integrating with Sage 100 directly can be powerful, it can also be complex and time-consuming. Endgrate offers a simplified solution by providing a unified API endpoint that connects to multiple platforms, including Sage 100. This allows developers to focus on core product development while outsourcing integration complexities.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can save you time and resources by visiting Endgrate's website.
Read More
Ready to get started?