Using the Microsoft Dynamics 365 API to Create or Update Custom Objects (with Javascript examples)
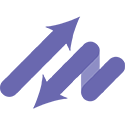
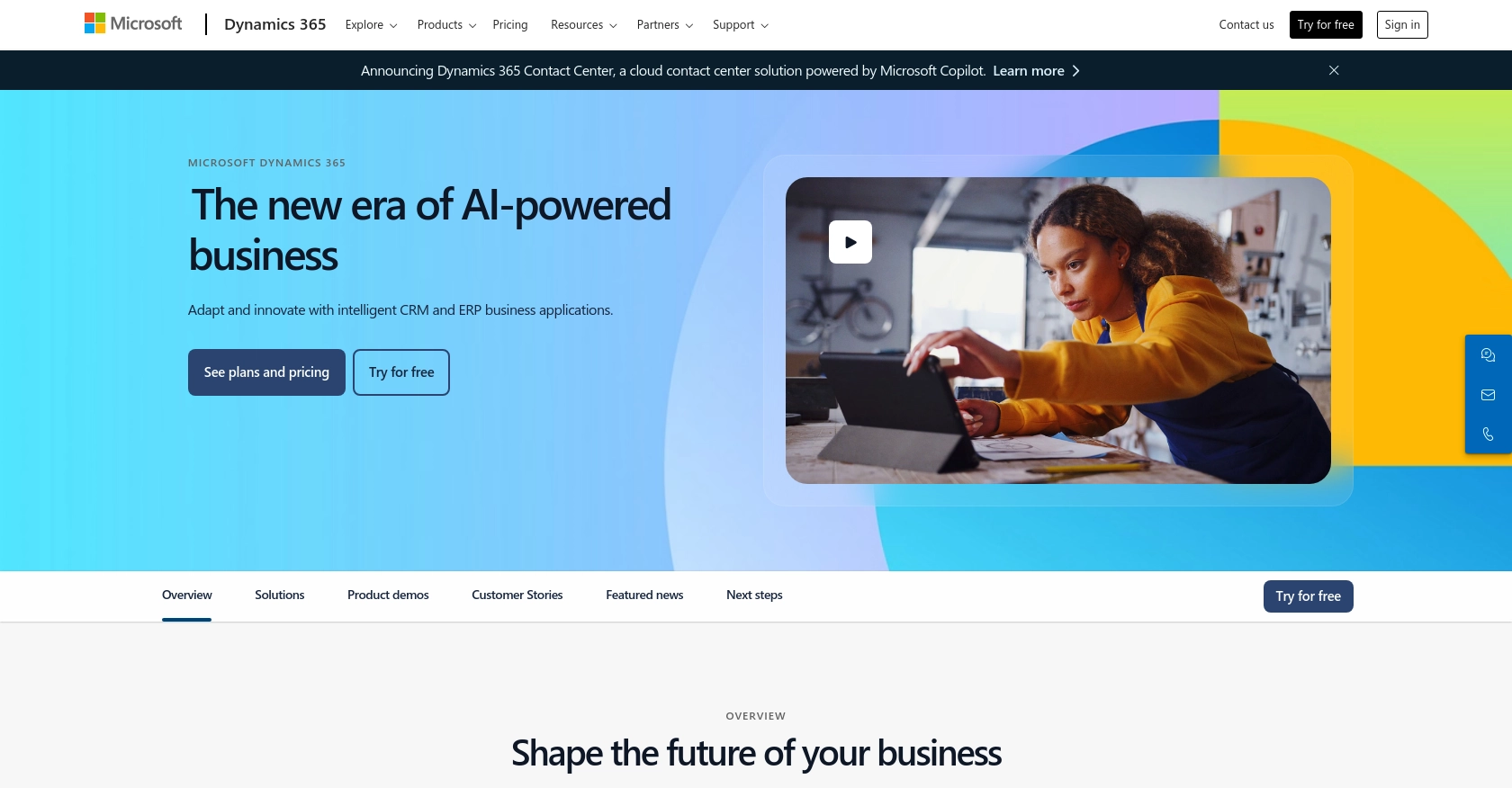
Introduction to Microsoft Dynamics 365 API
Microsoft Dynamics 365 is a comprehensive suite of business applications that combines CRM and ERP capabilities to streamline business processes and improve customer engagement. It offers a flexible and scalable platform that integrates seamlessly with other Microsoft products, making it a popular choice for businesses looking to enhance their operational efficiency.
Developers may want to integrate with the Microsoft Dynamics 365 API to create or update custom objects, enabling them to tailor the platform to specific business needs. For example, a developer might use the API to automate the creation of custom sales reports or update customer records in real-time, enhancing the decision-making process and improving customer service.
Setting Up a Microsoft Dynamics 365 Test/Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 API, you need to set up a test or sandbox account. This environment allows you to safely develop and test your applications without affecting live data. Follow these steps to create your sandbox account and configure OAuth authentication.
Step 1: Sign Up for a Microsoft Dynamics 365 Free Trial
To begin, sign up for a free trial of Microsoft Dynamics 365. Visit the Microsoft Dynamics 365 Free Trial page and follow the instructions to create your account. This trial provides access to a sandbox environment where you can test API interactions.
Step 2: Register Your Application in Microsoft Entra ID
Once your account is set up, you need to register your application in Microsoft Entra ID to enable OAuth authentication. This process involves creating an app registration that will provide you with the necessary credentials to authenticate API requests.
- Navigate to the Azure Portal and sign in with your Microsoft account.
- In the left-hand menu, select Azure Active Directory.
- Click on App registrations and then select New registration.
- Enter a name for your application and select the appropriate account type.
- For the redirect URI, use
https://localhost
for local development. - Click Register to create your application.
Step 3: Configure API Permissions
After registering your application, you need to configure the API permissions to allow access to Microsoft Dynamics 365 data.
- In the app registration page, select API permissions.
- Click on Add a permission and choose Dynamics CRM.
- Select the permissions required for your application, such as user_impersonation.
- Click Add permissions to apply the changes.
Step 4: Generate Client Secret
To authenticate API requests, you need a client secret. Follow these steps to generate one:
- In the app registration page, select Certificates & secrets.
- Click on New client secret.
- Provide a description and select an expiration period.
- Click Add and copy the client secret value. Store it securely as it will not be shown again.
Step 5: Obtain OAuth Access Token
With your application registered and client secret generated, you can now obtain an OAuth access token to authenticate your API requests. Use the Microsoft Authentication Library (MSAL) to acquire the token.
// Example using MSAL.js
const msalConfig = {
auth: {
clientId: "Your_Client_ID",
authority: "https://login.microsoftonline.com/common",
redirectUri: "https://localhost"
}
};
const msalInstance = new msal.PublicClientApplication(msalConfig);
msalInstance.loginPopup({
scopes: ["https://yourorg.crm.dynamics.com/.default"]
}).then(response => {
console.log("Access Token:", response.accessToken);
}).catch(error => {
console.error("Error acquiring token:", error);
});
Replace Your_Client_ID
with your application's client ID. This code snippet demonstrates how to acquire an access token using a popup login method.
With these steps completed, your Microsoft Dynamics 365 sandbox environment is ready for API integration. You can now proceed to create or update custom objects using the API.
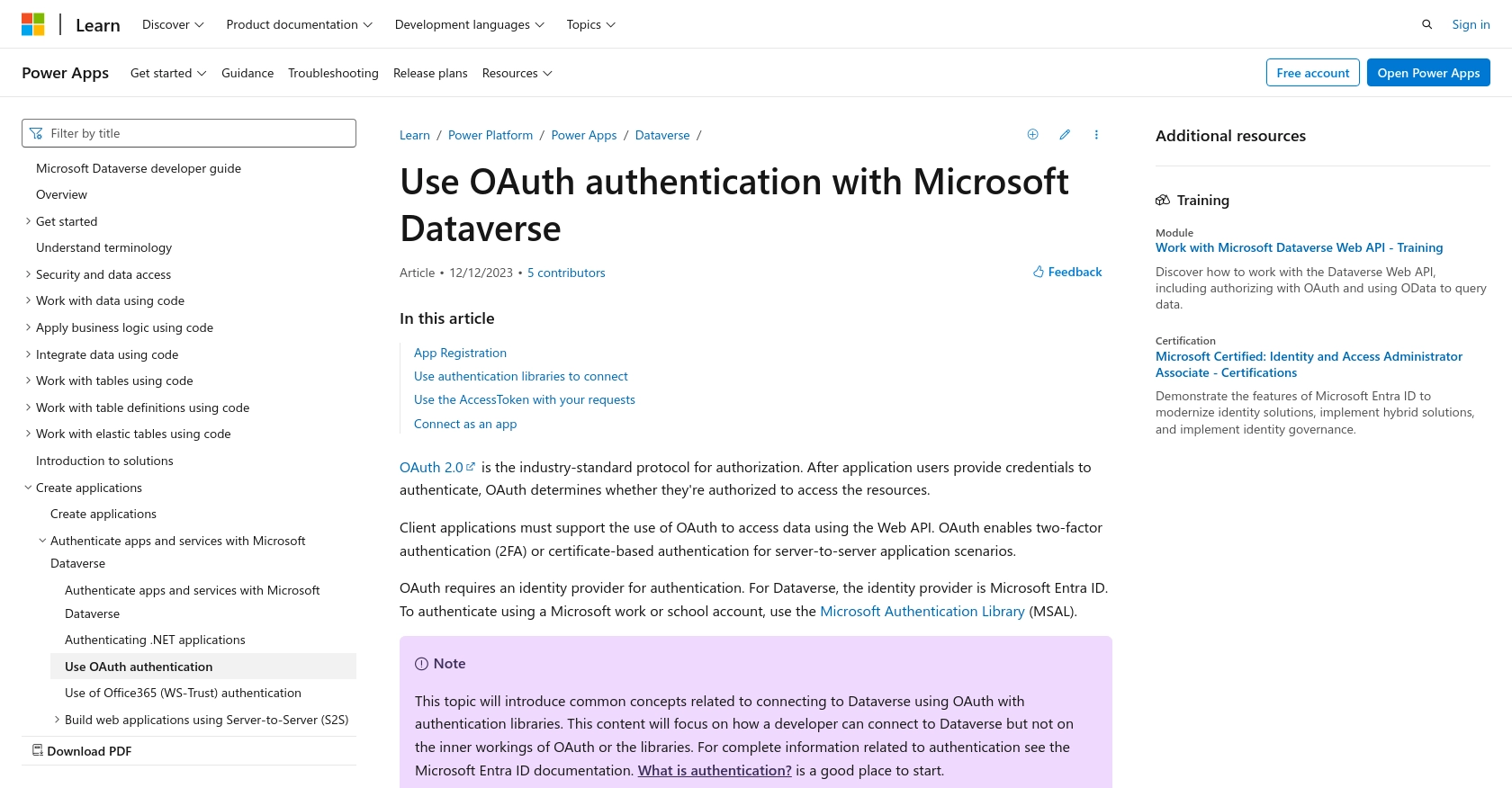
sbb-itb-96038d7
Making API Calls to Microsoft Dynamics 365 with JavaScript
To interact with Microsoft Dynamics 365 and perform operations such as creating or updating custom objects, you need to make API calls using JavaScript. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses effectively.
Prerequisites for JavaScript API Integration with Microsoft Dynamics 365
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A text editor or IDE for writing JavaScript code.
- The
axios
library for making HTTP requests. Install it using the command:
npm install axios
Creating and Updating Custom Objects in Microsoft Dynamics 365
To create or update custom objects in Microsoft Dynamics 365, you'll use the Web API endpoint. Here's a step-by-step guide:
Step 1: Set Up Your JavaScript Environment
Create a new JavaScript file, for example, dynamics365-api.js
, and import the necessary libraries:
const axios = require('axios');
Step 2: Configure API Request Headers
Set up the headers required for authentication and content type:
const headers = {
'Authorization': 'Bearer Your_Access_Token',
'Content-Type': 'application/json',
'OData-MaxVersion': '4.0',
'OData-Version': '4.0',
'Accept': 'application/json'
};
Replace Your_Access_Token
with the token obtained from the OAuth process.
Step 3: Define the API Endpoint and Data
Specify the API endpoint and the data for the custom object you wish to create or update:
const endpoint = 'https://yourorg.crm.dynamics.com/api/data/v9.2/entities';
const data = {
name: 'New Custom Object',
description: 'Description of the custom object'
};
Step 4: Make the API Call
Use the axios
library to send a POST request to create a new custom object:
axios.post(endpoint, data, { headers })
.then(response => {
console.log('Custom Object Created:', response.data);
})
.catch(error => {
console.error('Error Creating Object:', error.response.data);
});
For updating an existing object, you would use the PATCH method and include the object ID in the endpoint URL.
Step 5: Verify the API Call Success
After executing the API call, verify the success by checking the response data. You can also log into your Microsoft Dynamics 365 sandbox environment to confirm the creation or update of the custom object.
Handling Errors and Response Codes
It's crucial to handle errors effectively. The API may return various HTTP status codes indicating success or failure. Common error codes include:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server.
Refer to the Microsoft Dynamics 365 API documentation for a complete list of error codes and their meanings.
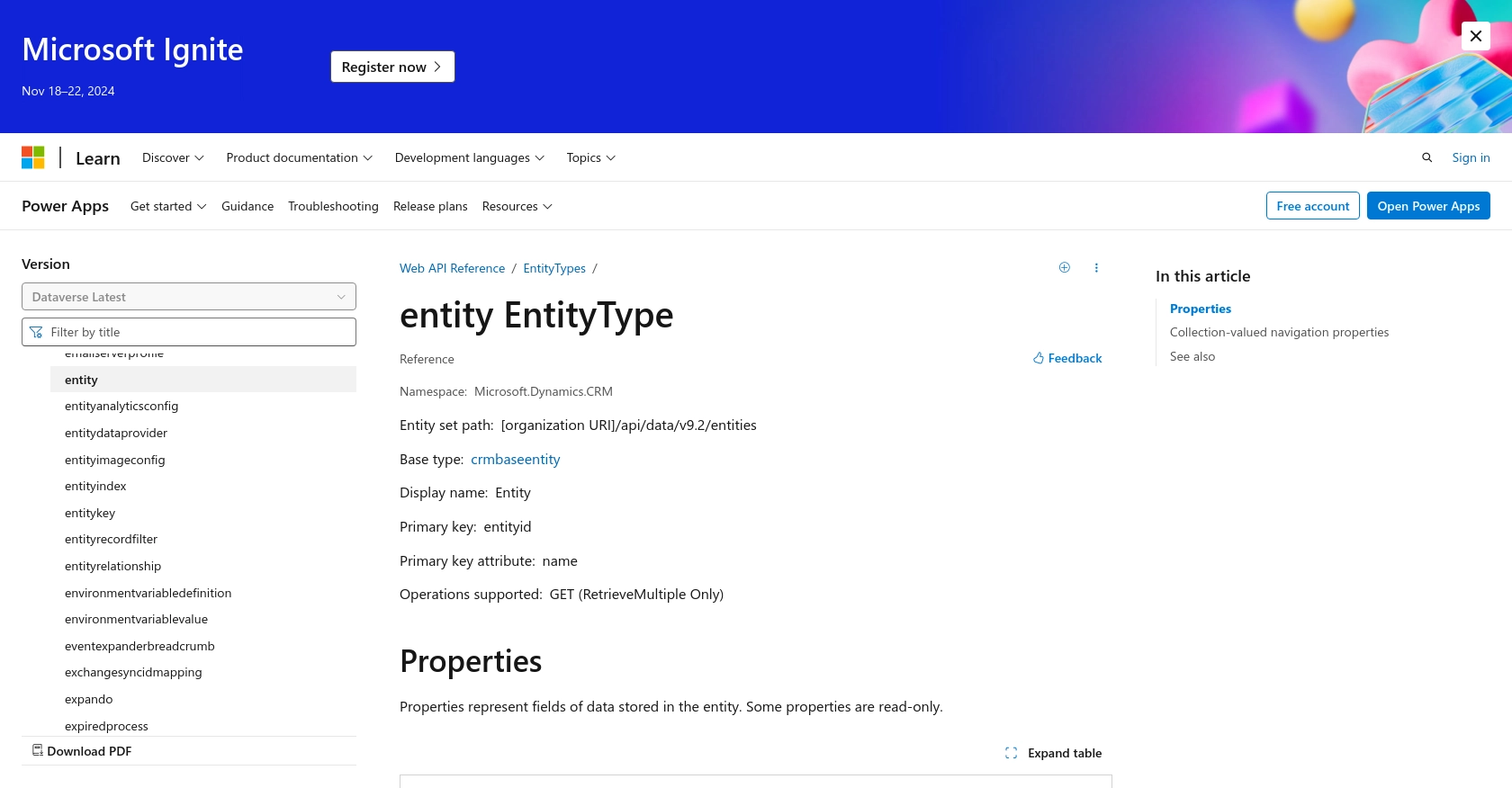
Conclusion and Best Practices for Microsoft Dynamics 365 API Integration
Integrating with the Microsoft Dynamics 365 API allows developers to create and update custom objects, enhancing the platform's functionality to meet specific business needs. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate using OAuth, and make API calls using JavaScript.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your client secrets and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be aware of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Standardize Data Fields: Ensure that data fields are standardized and consistent across different integrations to maintain data integrity and simplify data processing.
- Monitor API Usage: Regularly monitor your API usage and performance to identify any issues or areas for optimization.
Leverage Endgrate for Seamless Integration Management
For developers looking to streamline their integration processes, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can enhance your integration strategy.
Read More
- https://endgrate.com/provider/microsoftdynamics365
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/webapi/reference/entity?view=dataverse-latest
Ready to get started?