How to Get Accounts with the Xero API in Python
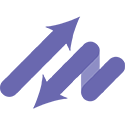
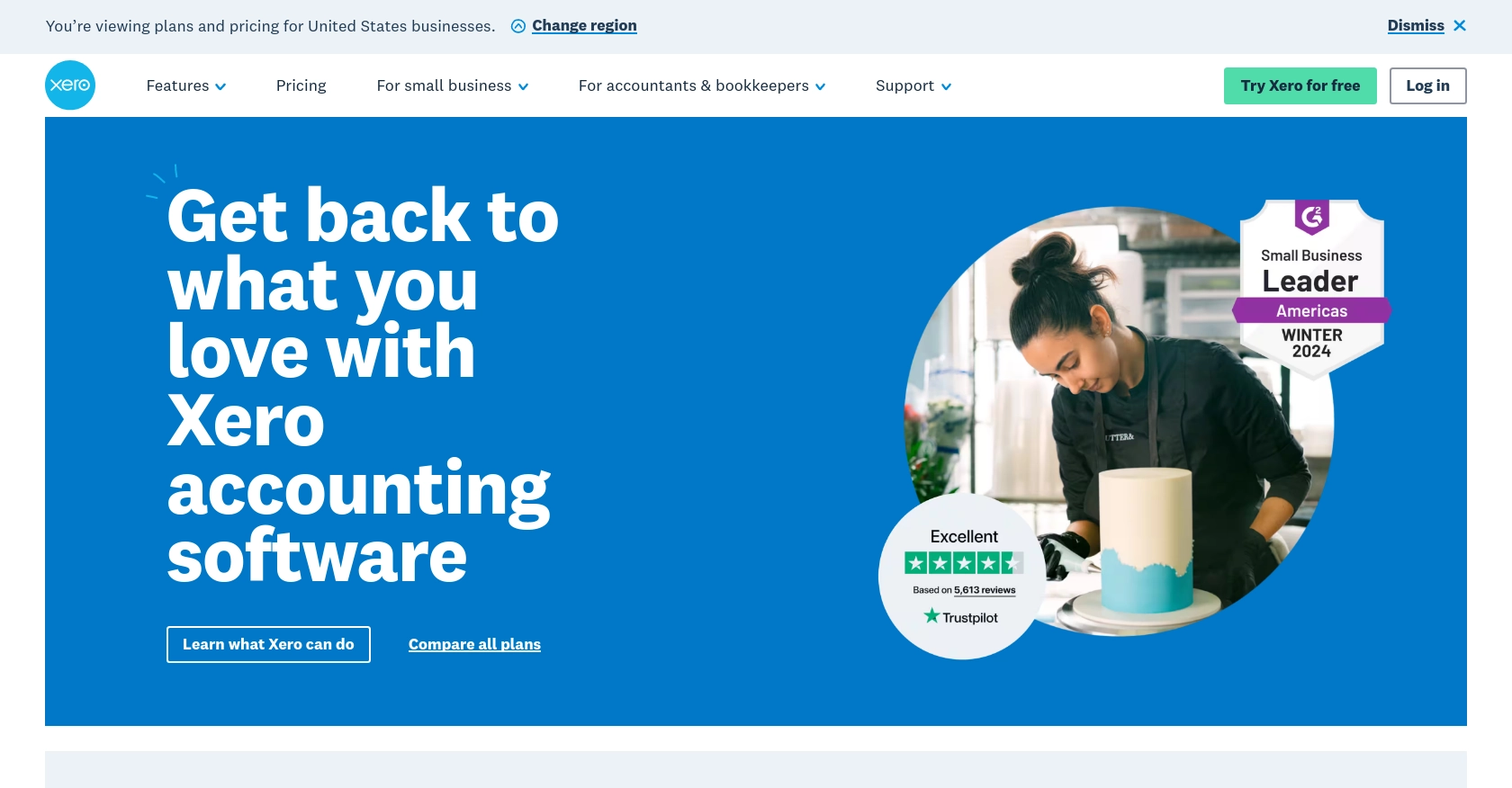
Introduction to Xero Accounting Software
Xero is a powerful cloud-based accounting software designed to help businesses manage their finances efficiently. With features such as invoicing, payroll, and expense tracking, Xero provides a comprehensive solution for small to medium-sized enterprises looking to streamline their financial operations.
Integrating with Xero's API allows developers to access and manipulate accounting data programmatically, enabling automation and enhanced functionality. For example, a developer might use the Xero API to retrieve account information, which can then be used to generate financial reports or synchronize data with other business applications.
Setting Up Your Xero Test/Sandbox Account for API Integration
Before you can start interacting with the Xero API, you'll need to set up a test or sandbox account. This allows you to experiment with the API without affecting live data. Xero provides a demo company that you can use for testing purposes.
Creating a Xero Account and Accessing the Demo Company
If you don't already have a Xero account, you can sign up for a free trial on the Xero website. Once your account is created, log in to the Xero dashboard.
To access the demo company, navigate to the organization menu in the top left corner and select "My Xero." From there, you can add the demo company to your account, which will provide you with a sandbox environment to test API calls.
Setting Up OAuth 2.0 Authentication for Xero API
Xero uses OAuth 2.0 for authentication, which requires you to create an app to obtain the necessary credentials. Follow these steps to set up OAuth 2.0:
- Go to the Xero Developer Portal and log in with your Xero account.
- Click on "New App" to create a new application.
- Fill in the required details such as the app name, company or application URL, and redirect URI. The redirect URI is where users will be redirected after they authorize your app.
- Once your app is created, you'll receive a client ID and client secret. Keep these credentials secure as they are essential for authenticating API requests.
Configuring Scopes and Permissions for API Access
To interact with the Xero API, you'll need to configure the appropriate scopes and permissions for your app:
- In the Xero Developer Portal, navigate to your app's settings and select "Scopes."
- Choose the scopes that correspond to the data you wish to access. For retrieving account information, ensure you have the "accounting.transactions" scope enabled.
- Save your changes to update the app's permissions.
With your test account and OAuth 2.0 authentication set up, you're ready to start making API calls to Xero. For more details on OAuth 2.0, refer to the Xero OAuth 2.0 documentation.
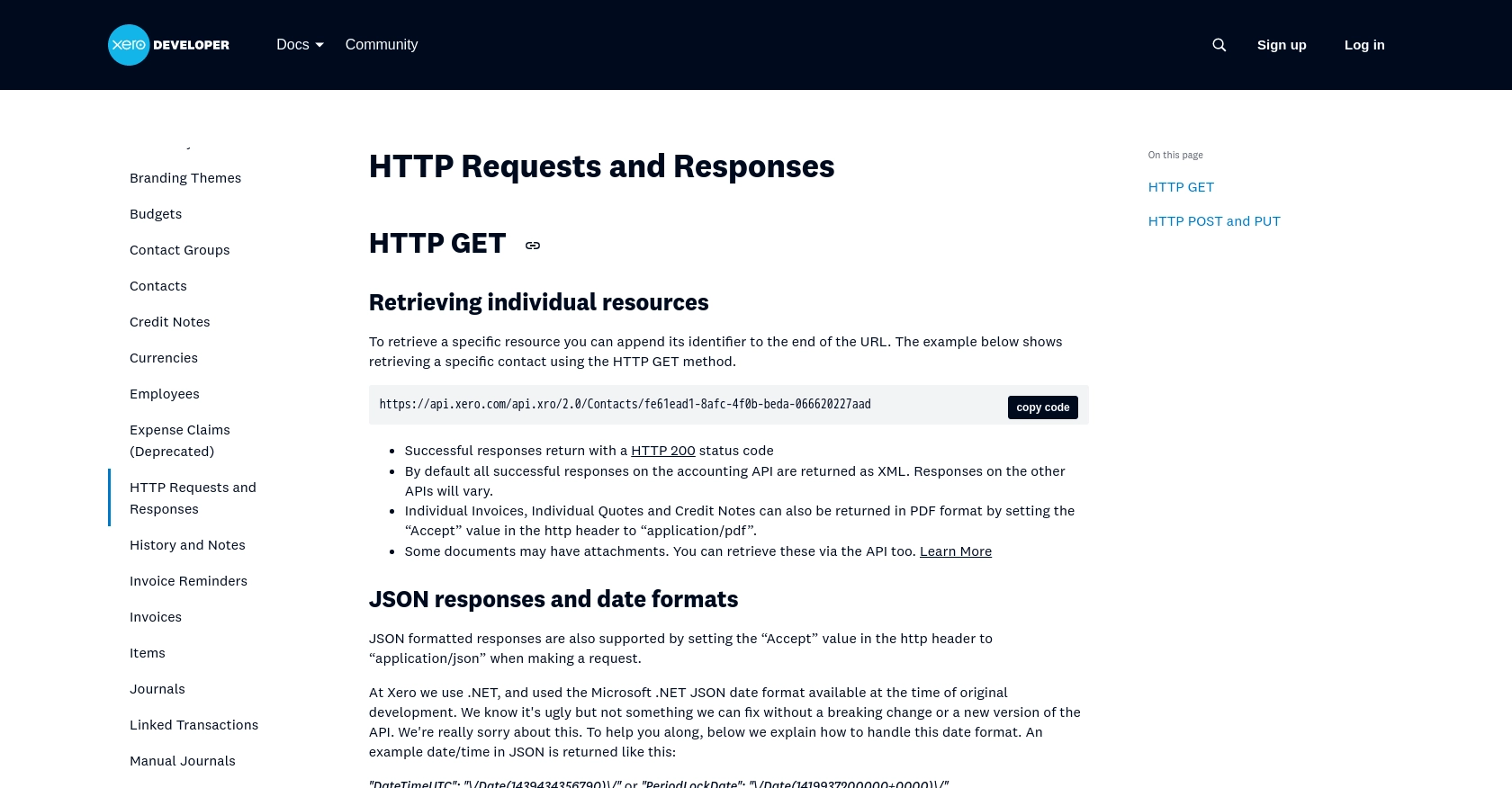
sbb-itb-96038d7
Making API Calls to Retrieve Accounts from Xero Using Python
With your Xero account and OAuth 2.0 authentication set up, you can now proceed to make API calls to retrieve account information. This section will guide you through the process of using Python to interact with the Xero API.
Setting Up Your Python Environment for Xero API Integration
Before making API calls, ensure you have the necessary tools and libraries installed. You'll need Python 3.11.1 and the requests
library to handle HTTP requests.
pip3 install requests
Writing Python Code to Fetch Accounts from Xero API
Create a new Python file named get_xero_accounts.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.xero.com/api.xro/2.0/Accounts"
headers = {
"Authorization": "Bearer Your_Token",
"Accept": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
accounts = response.json()
for account in accounts['Accounts']:
print(f"Account Name: {account['Name']}, Account Type: {account['Type']}")
else:
print(f"Failed to retrieve accounts: {response.status_code} - {response.text}")
Replace Your_Token
with the access token obtained during the OAuth 2.0 setup.
Running the Python Script to Retrieve Xero Accounts
Execute the script using the following command in your terminal:
python3 get_xero_accounts.py
If successful, the script will output the list of accounts from your Xero demo company. You can verify the retrieved data by comparing it with the accounts listed in your Xero sandbox environment.
Handling Errors and Understanding Xero API Response Codes
When making API calls, it's crucial to handle potential errors. The Xero API may return various HTTP status codes indicating the success or failure of a request. Common status codes include:
- 200 OK: The request was successful, and the response contains the requested data.
- 400 Bad Request: The request was malformed or contains invalid parameters.
- 401 Unauthorized: Authentication failed due to invalid credentials.
- 403 Forbidden: The request is not allowed, possibly due to insufficient permissions.
- 429 Too Many Requests: The request rate limit has been exceeded.
For more details on handling errors, refer to the Xero API documentation.
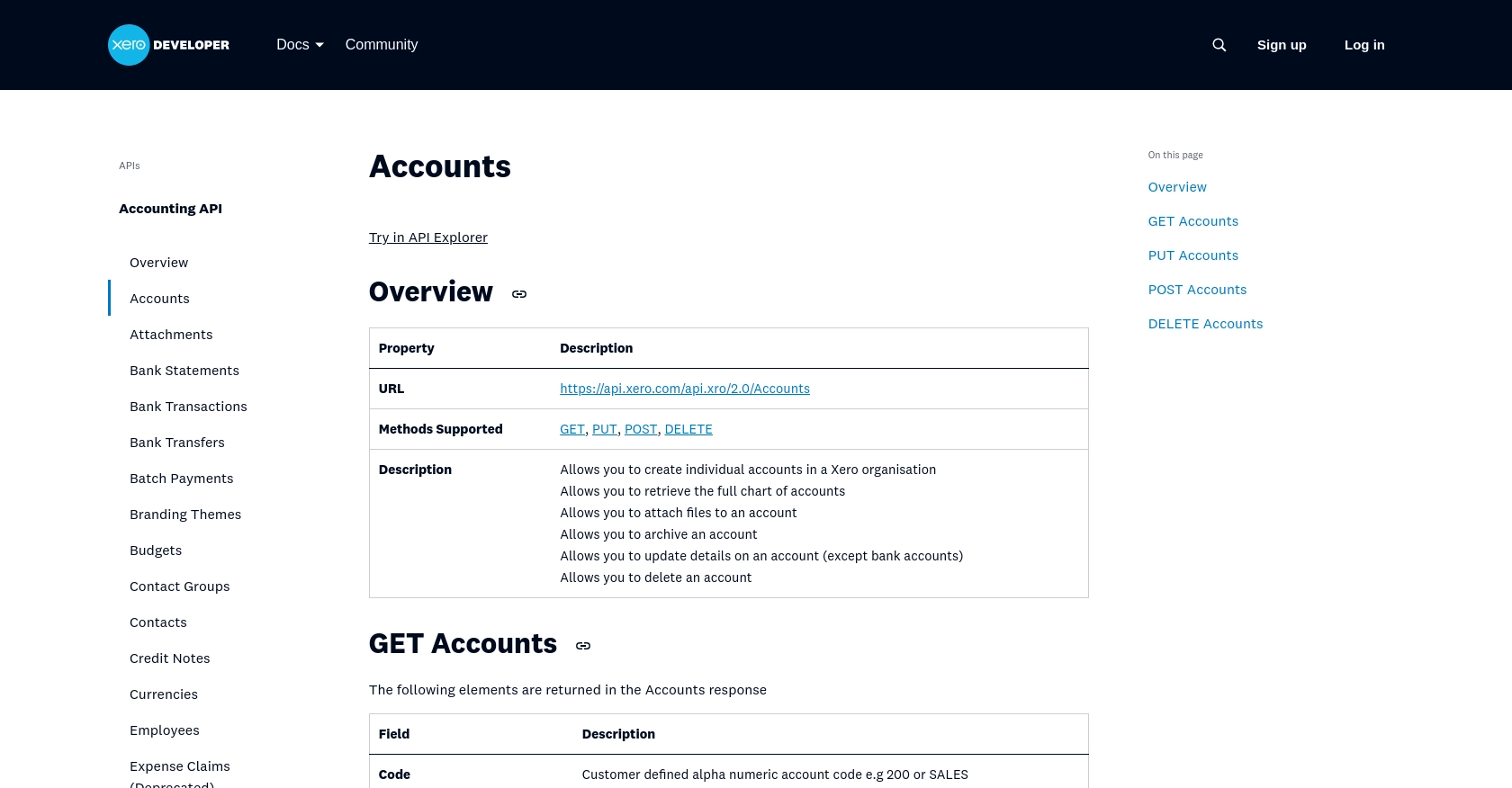
Conclusion and Best Practices for Xero API Integration
Integrating with the Xero API using Python offers developers a powerful way to automate and enhance financial data management. By following the steps outlined in this guide, you can efficiently retrieve account information and leverage it for various business applications.
Best Practices for Secure and Efficient Xero API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Xero's API has rate limits in place to ensure fair usage. Be mindful of these limits and implement retry logic to handle
429 Too Many Requests
errors gracefully. For more details, refer to the Xero API rate limits documentation. - Data Transformation and Standardization: When retrieving data from Xero, ensure that it is transformed and standardized to fit your application's requirements. This will help maintain consistency across different systems.
Streamline Your Integration Process with Endgrate
While building integrations with Xero can be rewarding, it can also be time-consuming and complex, especially when managing multiple integrations. Endgrate simplifies this process by providing a single API endpoint that connects to various platforms, including Xero.
By using Endgrate, you can save time and resources, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/accounts
Ready to get started?