Using the BigCommerce API to Get Orders (with Python examples)
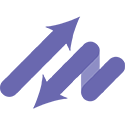
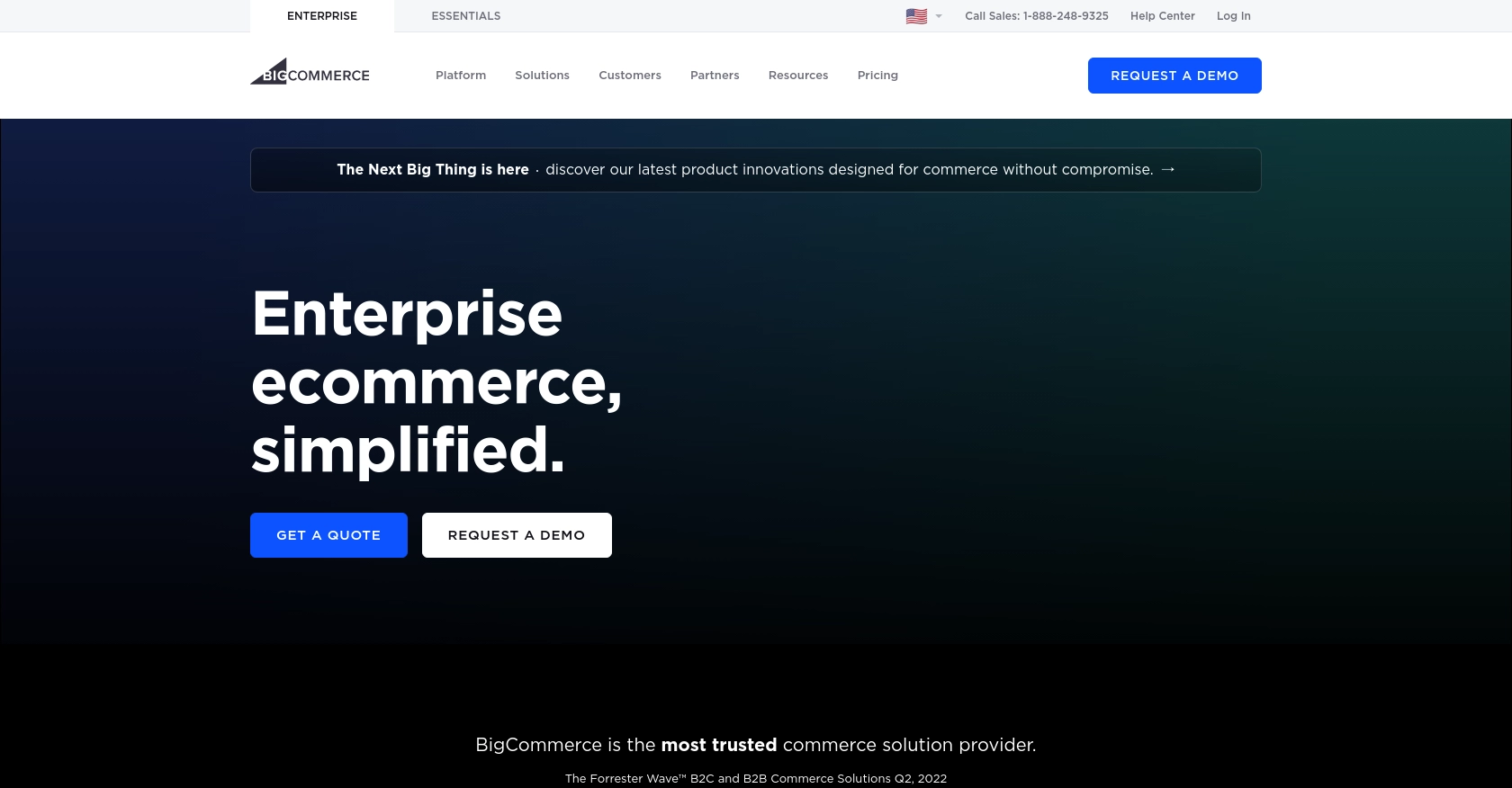
Introduction to BigCommerce API Integration
BigCommerce is a robust e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a wide range of features, including customizable templates, SEO tools, and multi-channel selling capabilities, making it a popular choice for businesses looking to expand their online presence.
Integrating with the BigCommerce API allows developers to access and manage store data programmatically, enabling automation and customization of various store operations. For example, a developer might use the BigCommerce API to retrieve order details and integrate them with an external inventory management system, streamlining the order fulfillment process.
This article will guide you through using Python to interact with the BigCommerce API, specifically focusing on retrieving order information. By following this tutorial, you'll learn how to efficiently access and manage order data, enhancing your store's operational capabilities.
Setting Up Your BigCommerce Test Account for API Integration
Before you can start interacting with the BigCommerce API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live store data.
Creating a BigCommerce Sandbox Account
To begin, you'll need to create a BigCommerce sandbox account. Follow these steps:
- Visit the BigCommerce Sandbox page and sign up for a free sandbox account.
- Fill in the required information, including your email and password, to create your account.
- Once registered, log in to your sandbox account to access the BigCommerce control panel.
Generating API Credentials for BigCommerce
To interact with the BigCommerce API, you'll need to generate API credentials. Here's how:
- In your BigCommerce control panel, navigate to Advanced Settings > API Accounts.
- Click on Create API Account and select Create V2/V3 API Token.
- Enter a name for your API account and select the necessary API scopes. For retrieving orders, ensure you have read access to orders.
- Click Save to generate your API credentials, including the Client ID, Client Secret, and Access Token.
- Store these credentials securely, as you'll need them to authenticate your API requests.
Configuring OAuth Authentication for BigCommerce API
BigCommerce uses OAuth for API authentication. Follow these steps to configure it:
- Use the Access Token generated in the previous step to authenticate your API requests.
- Include the token in the
X-Auth-Token
header of your HTTP requests.
For more details on authentication, refer to the BigCommerce Authentication Documentation.
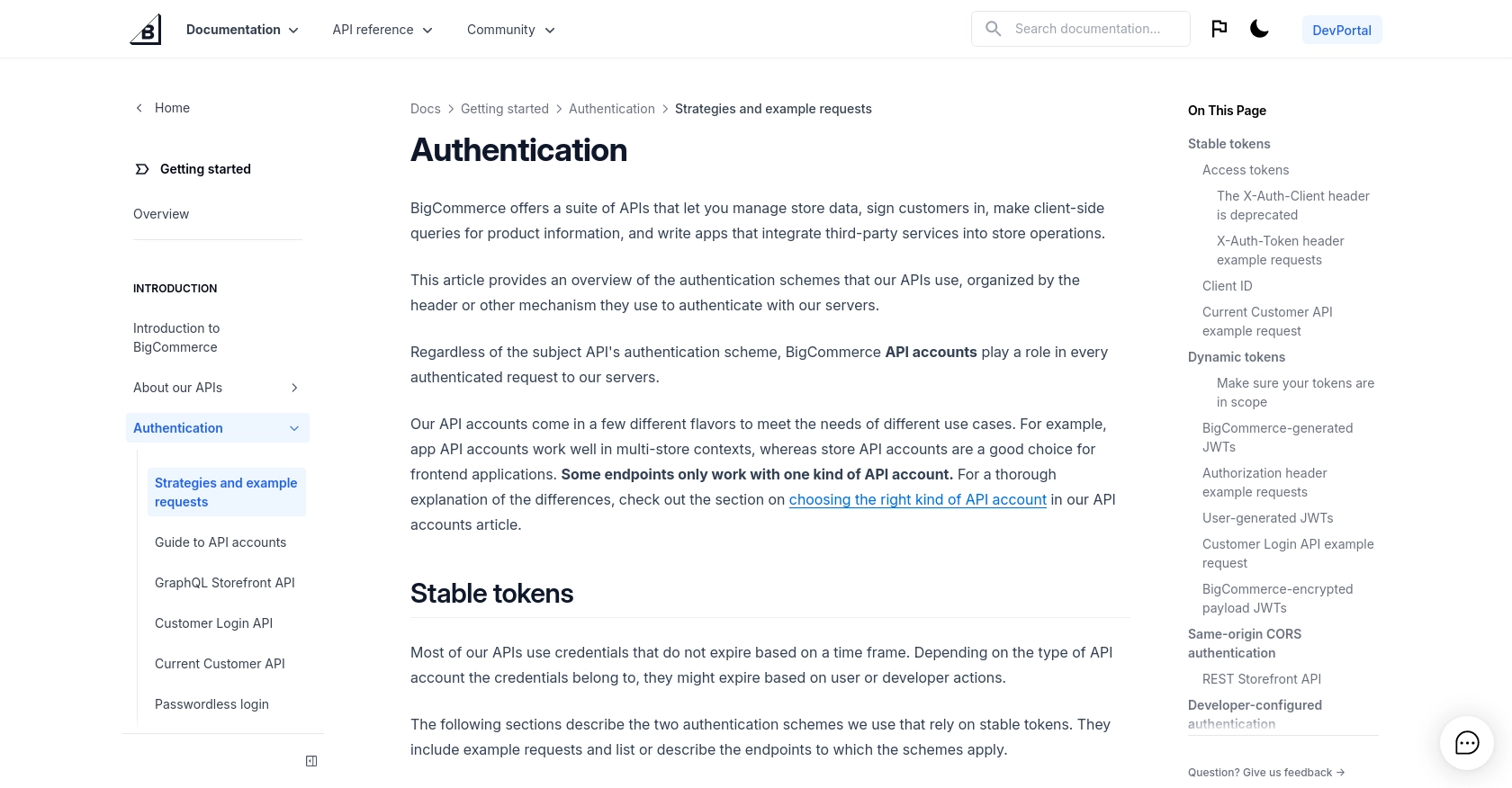
sbb-itb-96038d7
How to Make an API Call to Retrieve Orders from BigCommerce Using Python
To interact with the BigCommerce API and retrieve order data, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for BigCommerce API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Next, install the requests
library, which will be used to make HTTP requests to the BigCommerce API:
pip install requests
Writing Python Code to Retrieve Orders from BigCommerce
Now, let's write the Python code to fetch orders from your BigCommerce store. Create a file named get_bigcommerce_orders.py
and add the following code:
import requests
# Set the API endpoint and headers
store_hash = "your_store_hash"
endpoint = f"https://api.bigcommerce.com/stores/{store_hash}/v2/orders"
headers = {
"X-Auth-Token": "your_access_token",
"Accept": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
orders = response.json()
for order in orders:
print(f"Order ID: {order['id']}, Status: {order['status']}")
else:
print(f"Failed to retrieve orders. Status code: {response.status_code}, Error: {response.text}")
Replace your_store_hash
and your_access_token
with your actual store hash and access token obtained from the BigCommerce control panel.
Running the Python Script to Fetch BigCommerce Orders
Execute the script from your terminal or command line:
python get_bigcommerce_orders.py
If successful, you should see a list of orders with their IDs and statuses printed to the console.
Verifying the API Call and Handling Errors
To ensure the request succeeded, check the response status code. A status code of 200
indicates success. If you encounter errors, refer to the error message returned in the response for troubleshooting.
Common error codes include:
- 401 Unauthorized: Check your access token and ensure it has the correct permissions.
- 404 Not Found: Verify the endpoint URL and store hash.
For more details on error codes, refer to the BigCommerce Orders API Documentation.
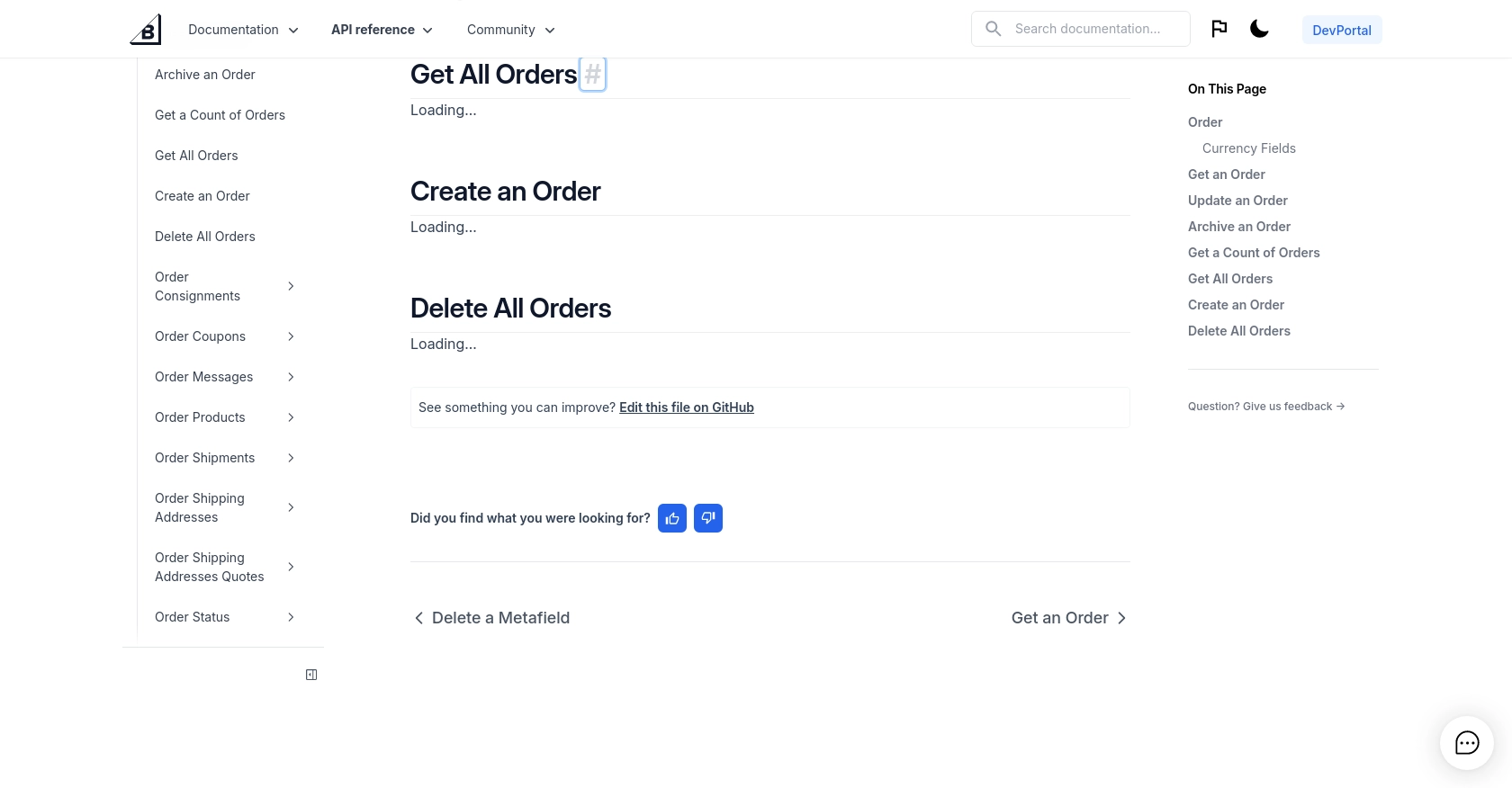
Conclusion and Best Practices for BigCommerce API Integration
Integrating with the BigCommerce API using Python offers a powerful way to automate and enhance your e-commerce operations. By retrieving order data programmatically, you can streamline processes such as inventory management, order fulfillment, and customer service.
Best Practices for Secure and Efficient BigCommerce API Usage
- Secure Storage of Credentials: Always store your API credentials securely. Consider using environment variables or a secure vault to protect sensitive information like your access token.
- Handle Rate Limiting: Be mindful of BigCommerce's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from the API is transformed and standardized according to your application's requirements. This will help maintain consistency across different systems.
- Error Handling: Implement robust error handling to manage API call failures. Log errors for further analysis and provide meaningful feedback to users when issues arise.
Enhance Your Integration Strategy with Endgrate
While integrating with the BigCommerce API can significantly boost your store's capabilities, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including BigCommerce.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
Ready to get started?