How to Get Contacts with the Cloze API in Python
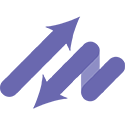
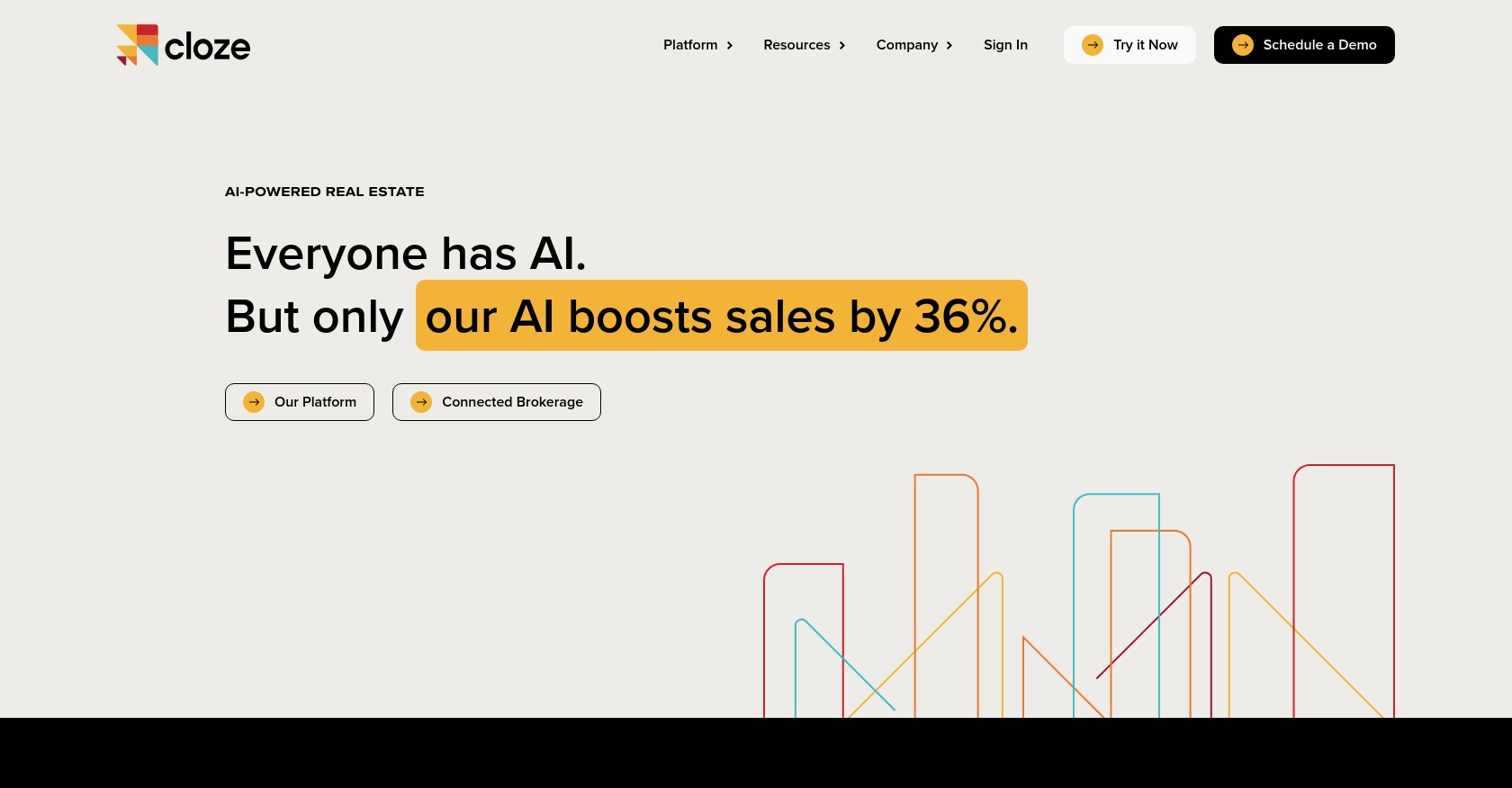
Introduction to Cloze API for Contact Management
Cloze is a powerful relationship management platform that helps businesses manage their contacts, projects, and communications effectively. By integrating with the Cloze API, developers can streamline access to contact data, enabling seamless automation and enhanced productivity.
Connecting with the Cloze API allows developers to retrieve and manage contact information programmatically. For example, a developer might use the Cloze API to fetch contact details and integrate them into a custom CRM system, ensuring that all customer interactions are up-to-date and accessible.
Setting Up Your Cloze Test or Sandbox Account for API Integration
Before you can begin interacting with the Cloze API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Step 1: Sign Up for a Cloze Account
If you don't already have a Cloze account, visit the Cloze website and sign up for a free trial or demo account. This will give you access to the features necessary for testing the API.
Step 2: Generate an API Key for Authentication
The Cloze API uses API key-based authentication. To generate your API key, follow these steps:
- Log in to your Cloze account.
- Navigate to the settings page by clicking on your profile icon and selecting "Settings."
- Under the "Integrations" section, find the "API Key" option.
- Click "Generate API Key" and copy the key provided. Keep this key secure as it will be used to authenticate your API requests.
For more detailed instructions, refer to the Cloze API Key documentation.
Step 3: Configure Your API Client
With your API key in hand, you can now configure your API client. When making requests to the Cloze API, you'll need to include your API key and Cloze account email address as query parameters:
import requests
# Define your Cloze account email and API key
user_email = "your_email@domain.com"
api_key = "your_api_key"
# Example API endpoint
endpoint = "https://api.cloze.com/v1/people/get"
# Make a GET request with authentication parameters
response = requests.get(endpoint, params={"user": user_email, "api_key": api_key})
# Check the response
if response.status_code == 200:
print("API call successful!")
else:
print("Failed to authenticate with the Cloze API.")
Replace your_email@domain.com
and your_api_key
with your actual Cloze account email and API key.
Step 4: Verify Your Setup
To ensure everything is set up correctly, make a simple API call to retrieve your profile information. This will confirm that your API key and email are correctly configured:
# Verify API setup by retrieving user profile
profile_endpoint = "https://api.cloze.com/v1/profile"
profile_response = requests.get(profile_endpoint, params={"user": user_email, "api_key": api_key})
if profile_response.status_code == 200:
print("Profile retrieved successfully:", profile_response.json())
else:
print("Error retrieving profile:", profile_response.status_code)
By following these steps, you will have successfully set up your Cloze test or sandbox account, allowing you to begin integrating with the Cloze API.
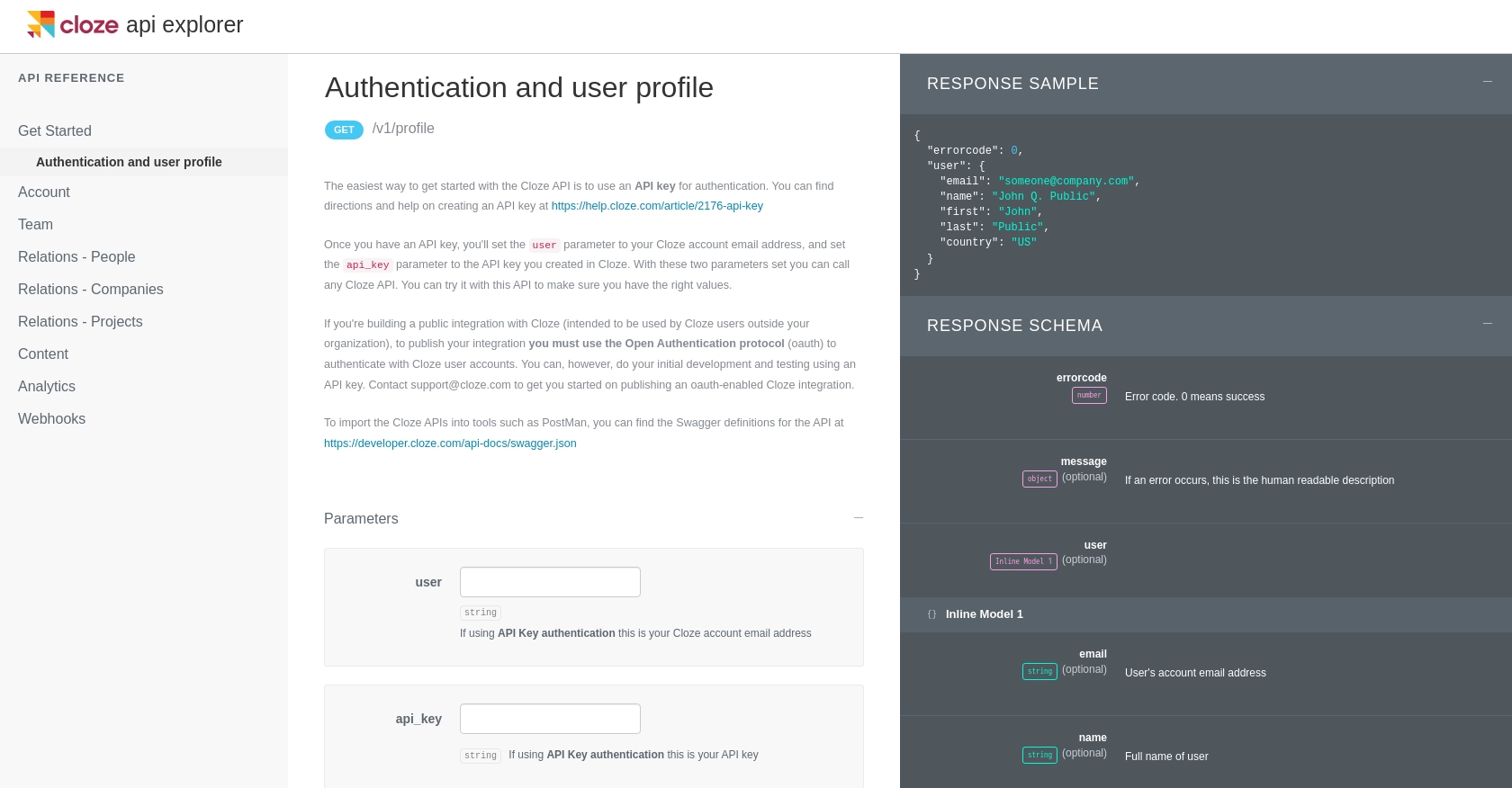
sbb-itb-96038d7
Making API Calls to Retrieve Contacts Using the Cloze API in Python
Now that you have set up your Cloze account and configured your API client, it's time to make API calls to retrieve contact information. This section will guide you through the process of using Python to interact with the Cloze API and fetch contact details.
Prerequisites for Using Python with Cloze API
Before proceeding, ensure you have the following installed on your machine:
- Python 3.11.1 or later
- The Python package installer
pip
Additionally, you'll need the requests
library to make HTTP requests. Install it using the following command:
pip install requests
Example Code to Retrieve Contacts from Cloze API
Create a new Python file named get_cloze_contacts.py
and add the following code:
import requests
# Define your Cloze account email and API key
user_email = "your_email@domain.com"
api_key = "your_api_key"
# Set the API endpoint for retrieving contacts
endpoint = "https://api.cloze.com/v1/people/find"
# Make a GET request to the API
response = requests.get(endpoint, params={"user": user_email, "api_key": api_key})
# Check if the request was successful
if response.status_code == 200:
contacts = response.json().get("people", [])
for contact in contacts:
print(f"Name: {contact.get('name')}, Email: {contact.get('emails', [{}])[0].get('value')}")
else:
print("Failed to retrieve contacts:", response.status_code)
Replace your_email@domain.com
and your_api_key
with your actual Cloze account email and API key.
Running the Code and Verifying the Output
Run the script from your terminal or command line using the following command:
python get_cloze_contacts.py
If successful, the script will output the names and email addresses of the contacts retrieved from your Cloze account.
Handling Errors and Verifying API Requests
It's crucial to handle potential errors when making API calls. If the request fails, the script will print the status code, which can help diagnose issues. Ensure that your API key and email are correct and that your network connection is stable.
For more detailed error information, refer to the Cloze API documentation.
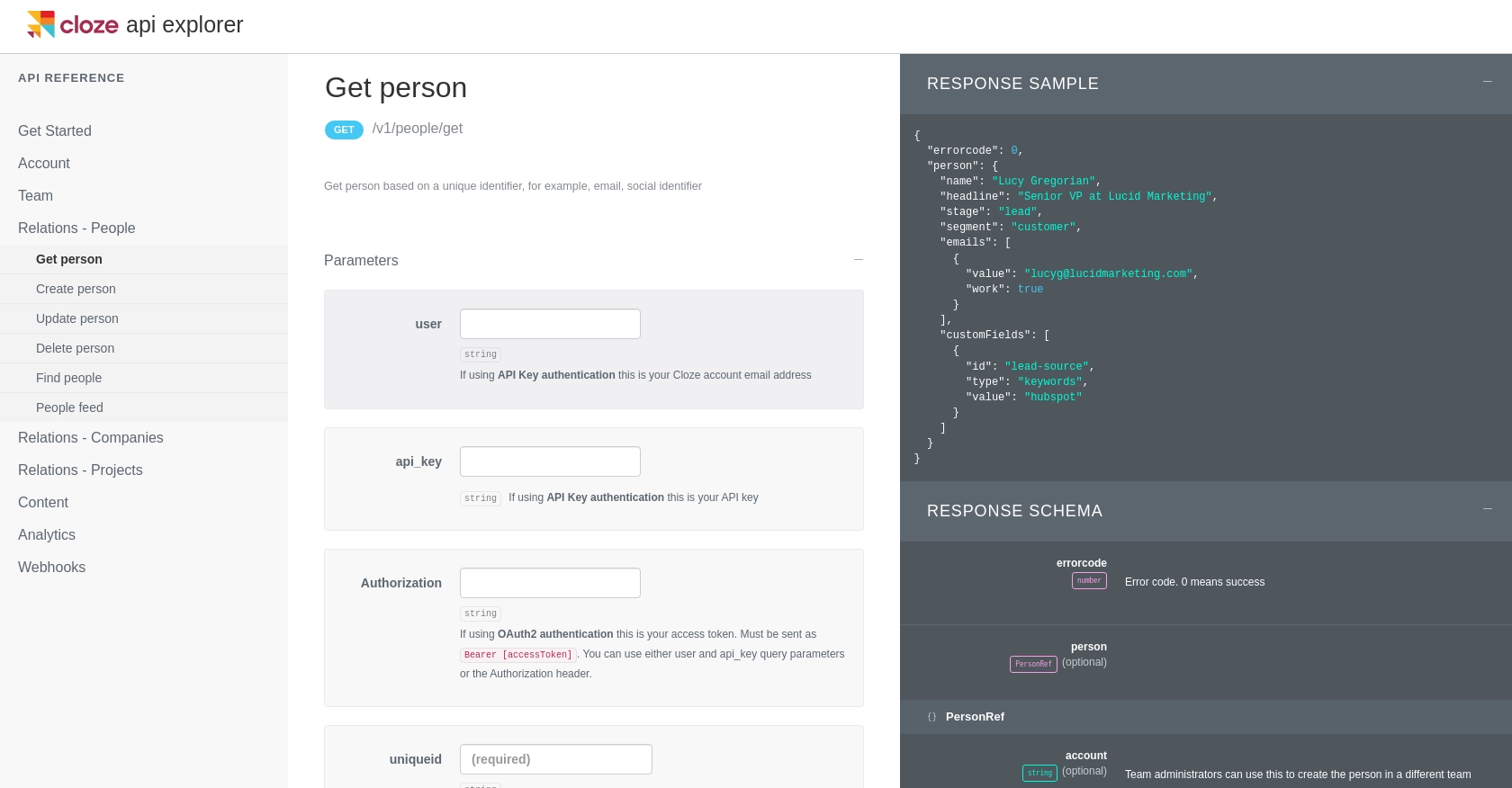
Conclusion and Best Practices for Using the Cloze API with Python
Integrating with the Cloze API using Python provides a powerful way to manage and automate contact data within your applications. By following the steps outlined in this guide, you can efficiently retrieve and handle contact information, enhancing your CRM capabilities.
Best Practices for Secure and Efficient API Integration with Cloze
- Securely Store API Credentials: Always store your API key and user credentials securely, using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Standardize Data Fields: Ensure that contact data retrieved from the Cloze API is standardized and transformed as needed to fit your application's data model.
- Error Handling: Implement robust error handling to manage API call failures and log errors for troubleshooting. Refer to the Cloze API documentation for detailed error codes and responses.
Streamlining API Integrations with Endgrate
For developers looking to simplify and accelerate their integration processes, consider using Endgrate. Endgrate offers a unified API endpoint that connects to multiple platforms, including Cloze, allowing you to manage integrations more efficiently. By leveraging Endgrate, you can focus on your core product development while outsourcing complex integration tasks.
Visit Endgrate to learn more about how you can enhance your integration experience and save valuable development time.
Read More
Ready to get started?