How to Create or Update Companies with the Teamwork CRM API in Javascript
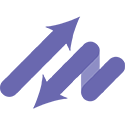
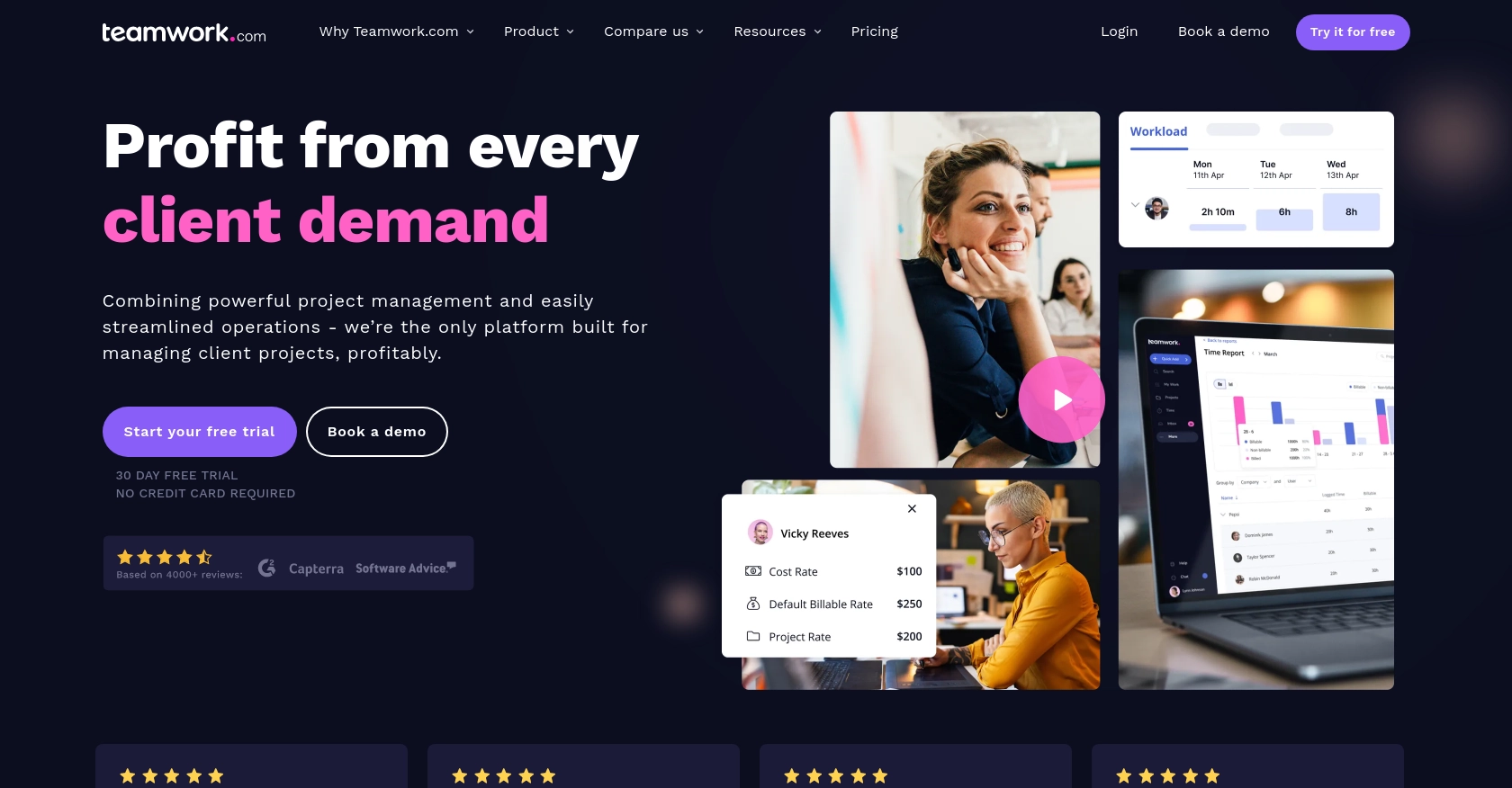
Introduction to Teamwork CRM API
Teamwork CRM is a robust customer relationship management platform designed to help businesses manage their sales pipelines and customer interactions effectively. With features like deal tracking, contact management, and reporting, Teamwork CRM provides a comprehensive solution for sales teams looking to enhance their productivity and close deals faster.
Integrating with the Teamwork CRM API allows developers to automate and streamline CRM processes, such as creating or updating company records. For example, a developer might want to automatically update company details in Teamwork CRM whenever there is a change in their internal database, ensuring that sales teams always have access to the most current information.
Setting Up a Teamwork CRM Test or Sandbox Account
Before you can start interacting with the Teamwork CRM API, it's essential to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Register for a Teamwork CRM Account
- Visit the Teamwork CRM website and sign up for a free trial or demo account.
- Follow the on-screen instructions to complete the registration process. Once registered, log in to your account.
Creating an App for API Access
To interact with the Teamwork CRM API, you'll need to create an app to obtain the necessary credentials for authentication.
- Navigate to the Developer Portal within your Teamwork CRM account.
- Select the option to create a new app and fill in the required details, such as app name and description.
- Once your app is created, note down the client ID and client secret, as these will be used for OAuth 2.0 authentication.
Authenticating with OAuth 2.0
Teamwork CRM supports OAuth 2.0 for secure API access. Follow these steps to authenticate:
- Use the client ID and client secret obtained from your app to request an access token.
- Include the access token in the Authorization header of your API requests as follows:
Authorization: Bearer {access_token}
For more details on authentication, refer to the Teamwork CRM Authentication Documentation.
Testing Your Setup
With your test account and app set up, you can now begin making API calls to create or update company records. Ensure that you verify the results within your sandbox environment to confirm successful interactions.
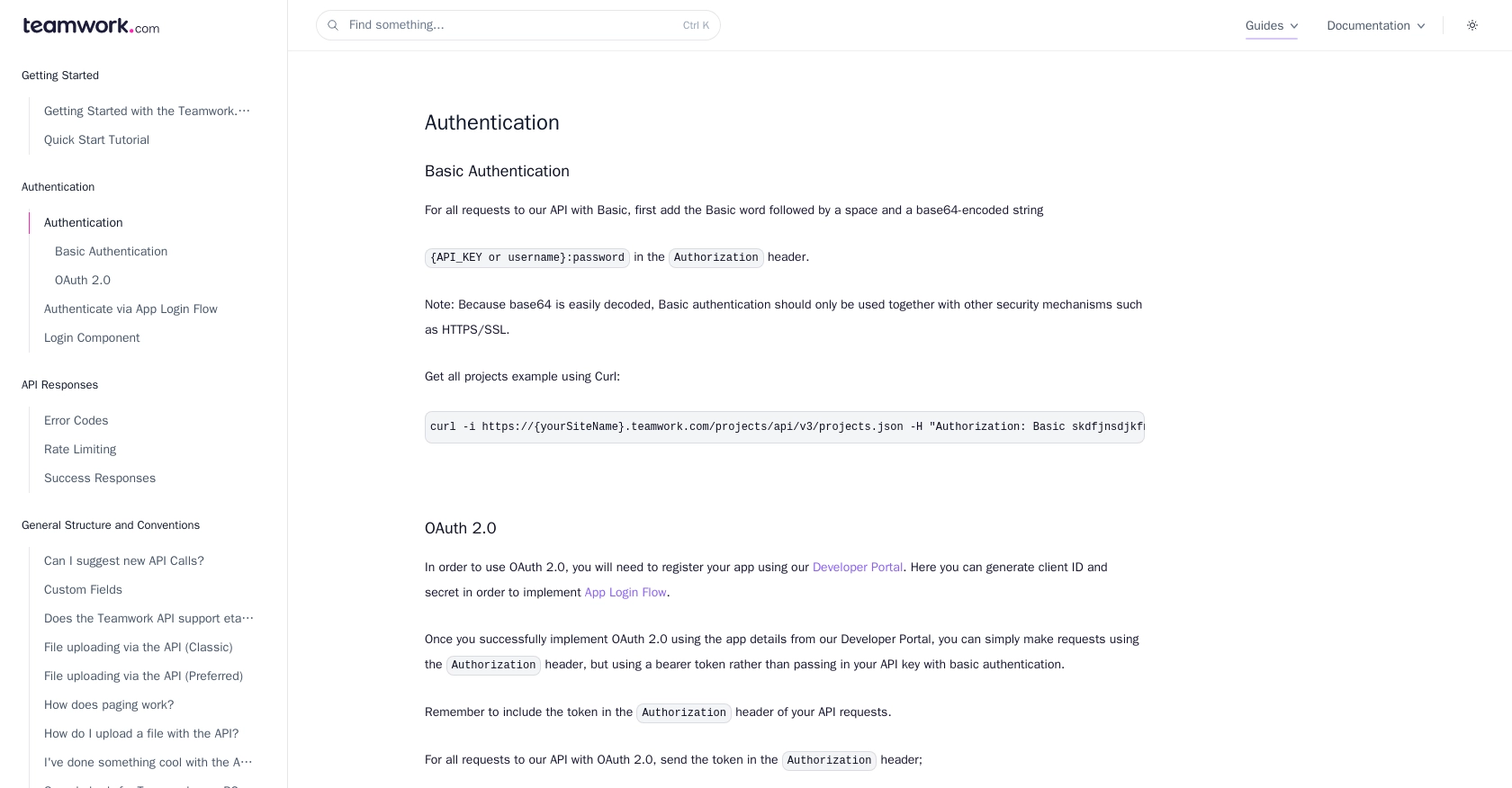
sbb-itb-96038d7
Making API Calls to Create or Update Companies with Teamwork CRM in JavaScript
To interact with the Teamwork CRM API using JavaScript, you'll need to set up your environment and write code to make HTTP requests. This section will guide you through the process of creating or updating company records in Teamwork CRM.
Setting Up Your JavaScript Environment for Teamwork CRM API
Before making API calls, ensure you have Node.js installed on your machine. You can download it from the official Node.js website. Additionally, you'll need the axios
library to handle HTTP requests. Install it using npm:
npm install axios
Creating a JavaScript Function to Interact with Teamwork CRM API
Now, let's write a JavaScript function to create or update a company record in Teamwork CRM. This function will use the axios
library to send a POST or PUT request to the API.
const axios = require('axios');
async function createOrUpdateCompany(companyData, accessToken) {
const url = 'https://{yourSiteName}.teamwork.com/crm/v2/companies';
const headers = {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
};
try {
const response = await axios.post(url, companyData, { headers });
console.log('Company created/updated successfully:', response.data);
} catch (error) {
console.error('Error creating/updating company:', error.response ? error.response.data : error.message);
}
}
// Example usage
const companyData = {
name: 'Example Company',
address: '123 Example Street',
phone: '123-456-7890'
};
const accessToken = 'your_access_token_here';
createOrUpdateCompany(companyData, accessToken);
Replace {yourSiteName}
with your actual Teamwork site name and your_access_token_here
with your OAuth 2.0 access token.
Verifying API Call Success in Teamwork CRM
After running the script, check your Teamwork CRM sandbox account to verify that the company record has been created or updated. If successful, you should see the new or modified company details in your CRM dashboard.
Handling Errors and Understanding Error Codes
When making API calls, it's crucial to handle potential errors. The Teamwork CRM API may return various error codes, such as:
- 401 Unauthorized: Check your API key or access token.
- 422 Unprocessable Entity: Ensure the JSON data is correctly formatted.
- 429 Too Many Requests: You have exceeded the rate limit. Wait before retrying.
For more detailed error information, refer to the Teamwork CRM Error Codes Documentation.
Conclusion and Best Practices for Using Teamwork CRM API in JavaScript
Integrating with the Teamwork CRM API using JavaScript can significantly enhance your ability to manage company records efficiently. By automating the creation and updating of company data, you ensure that your sales teams have access to the most current information, improving productivity and decision-making.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth 2.0 access tokens securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the rate limits imposed by Teamwork CRM. The API allows up to 150 requests per minute for most plans. Implement retry logic with exponential backoff to handle HTTP 429 errors gracefully. For more details, refer to the Teamwork CRM Rate Limiting Documentation.
- Validate and Standardize Data: Ensure that the data you send to the API is validated and standardized to prevent errors and maintain data integrity.
- Error Handling: Implement robust error handling to manage different HTTP status codes and provide meaningful feedback to users or logs for debugging.
Leverage Endgrate for Streamlined Integration Management
While integrating with Teamwork CRM API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies integration management, allowing you to focus on your core product development. By using Endgrate, you can build once for each use case and easily connect to various platforms, providing an intuitive integration experience for your customers.
Explore how Endgrate can help you save time and resources by visiting Endgrate today.
Read More
Ready to get started?