How to Create or Update Customers with the Xero API in Python
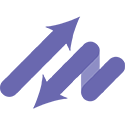
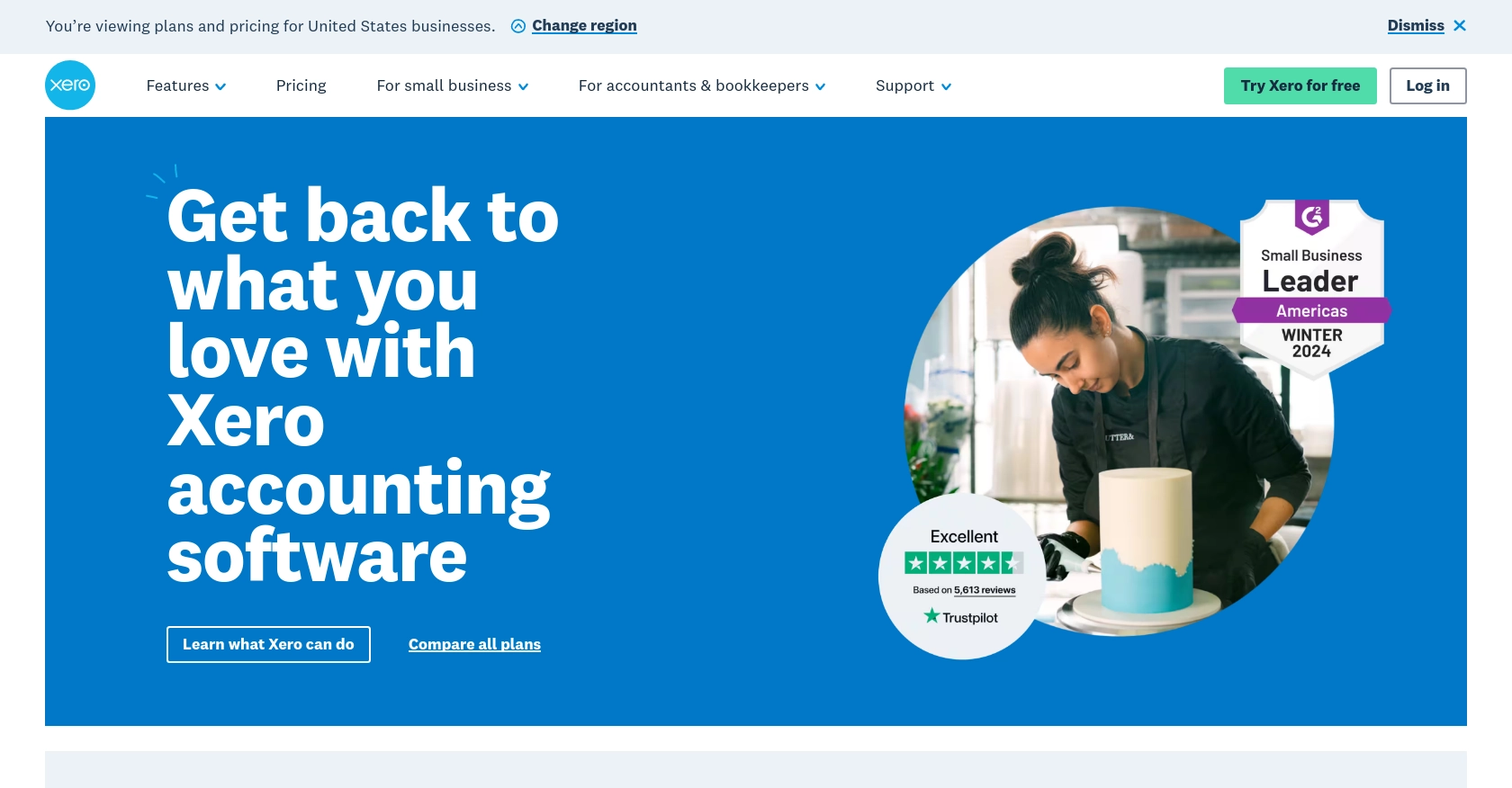
Introduction to Xero API Integration
Xero is a powerful cloud-based accounting software platform designed to help small and medium-sized businesses manage their finances efficiently. With features like invoicing, bank reconciliation, and expense tracking, Xero provides a comprehensive solution for financial management.
Developers may want to integrate with Xero's API to automate accounting tasks and streamline customer management. For example, using the Xero API, a developer can create or update customer records directly from their application, ensuring that customer data is always up-to-date and accurate.
This article will guide you through the process of using Python to interact with the Xero API, focusing on creating and updating customer records. By the end of this tutorial, you'll be able to efficiently manage customer data within Xero using Python.
Setting Up Your Xero Test/Sandbox Account for API Integration
Before you can start integrating with the Xero API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting real data. Xero provides a free trial and a dedicated sandbox environment for developers to test their applications.
Creating a Xero Developer Account
To begin, you'll need to create a Xero developer account. Follow these steps:
- Visit the Xero Developer Portal and sign up for a free developer account.
- Once registered, log in to your developer account to access the dashboard.
Setting Up a Xero Sandbox Organization
After creating your developer account, you can set up a sandbox organization:
- Navigate to the "My Apps" section in your Xero developer dashboard.
- Click on "Try the API" to create a new sandbox organization.
- Follow the prompts to set up your sandbox environment, which will mimic a real Xero organization.
Creating a Xero App for OAuth 2.0 Authentication
Xero uses OAuth 2.0 for authentication, which requires you to create an app to obtain the necessary credentials:
- In the "My Apps" section, click on "New App" to create a new application.
- Fill in the required details, such as the app name and company URL.
- Set the redirect URI to a valid endpoint where Xero will send the authorization code.
- Once your app is created, you'll receive a client ID and client secret. Keep these credentials secure as they are essential for authenticating API requests.
Obtaining OAuth 2.0 Access Tokens
With your app created, you can now obtain access tokens to authenticate API requests:
- Direct users to the Xero authorization URL, including your client ID and requested scopes.
- Upon user consent, Xero will redirect to your specified URI with an authorization code.
- Exchange this code for an access token by making a POST request to the Xero token endpoint, including your client ID, client secret, and authorization code.
For more details on the OAuth 2.0 flow, refer to the Xero OAuth 2.0 documentation.
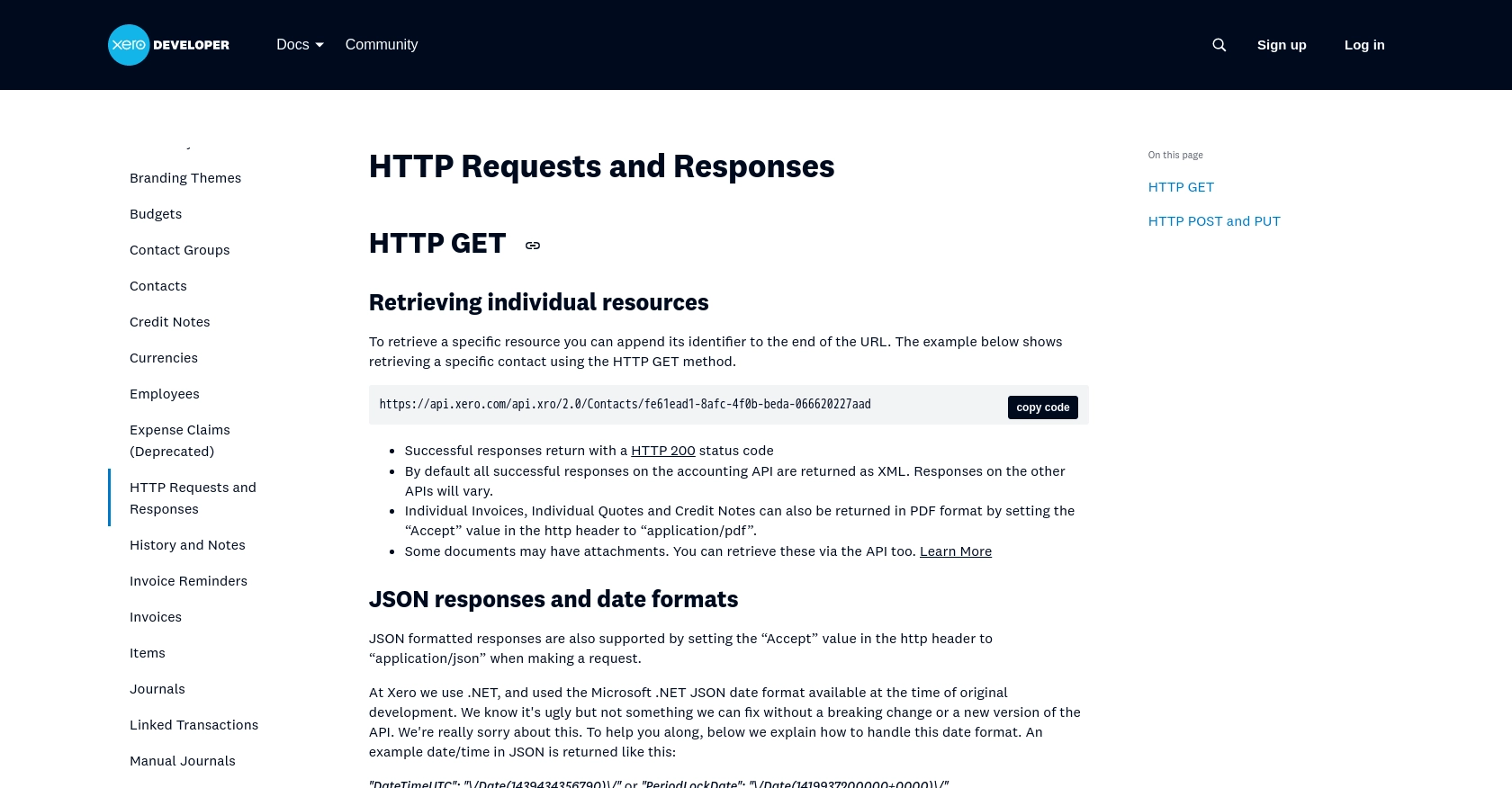
sbb-itb-96038d7
Making API Calls to Create or Update Customers with Xero API in Python
To interact with the Xero API using Python, you'll need to set up your environment and write code to make API requests. This section will guide you through the necessary steps, including setting up Python, installing dependencies, and writing the code to create or update customer records in Xero.
Setting Up Your Python Environment for Xero API Integration
Before making API calls, ensure you have the following installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once you have these installed, open your terminal or command prompt and install the required libraries using the following command:
pip install requests
The requests
library will help you make HTTP requests to the Xero API.
Writing Python Code to Create or Update Customers in Xero
Now that your environment is set up, you can write the code to interact with the Xero API. Below is an example of how to create or update a customer record:
import requests
import json
# Set the API endpoint
url = "https://api.xero.com/api.xro/2.0/Contacts"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the customer data
customer_data = {
"Contacts": [
{
"Name": "John Doe",
"EmailAddress": "johndoe@example.com",
"FirstName": "John",
"LastName": "Doe"
}
]
}
# Make the POST request to create or update the customer
response = requests.post(url, headers=headers, data=json.dumps(customer_data))
# Check if the request was successful
if response.status_code == 200:
print("Customer created or updated successfully.")
else:
print(f"Failed to create or update customer. Status code: {response.status_code}")
print(response.json())
Replace Your_Access_Token
with the access token obtained from the OAuth 2.0 authentication process.
Verifying Successful API Requests in Xero Sandbox
After running the code, you can verify the success of the API request by checking the Xero sandbox account. If the customer was created or updated successfully, the changes should reflect in the sandbox environment.
Handling Errors and Understanding Xero API Error Codes
When making API requests, it's crucial to handle potential errors. The Xero API may return various error codes, such as:
- 400 Bad Request: The request was invalid or cannot be otherwise served.
- 401 Unauthorized: Authentication credentials were missing or incorrect.
- 403 Forbidden: The request is understood, but it has been refused or access is not allowed.
- 404 Not Found: The requested resource could not be found.
For more detailed information on error codes, refer to the Xero API documentation.
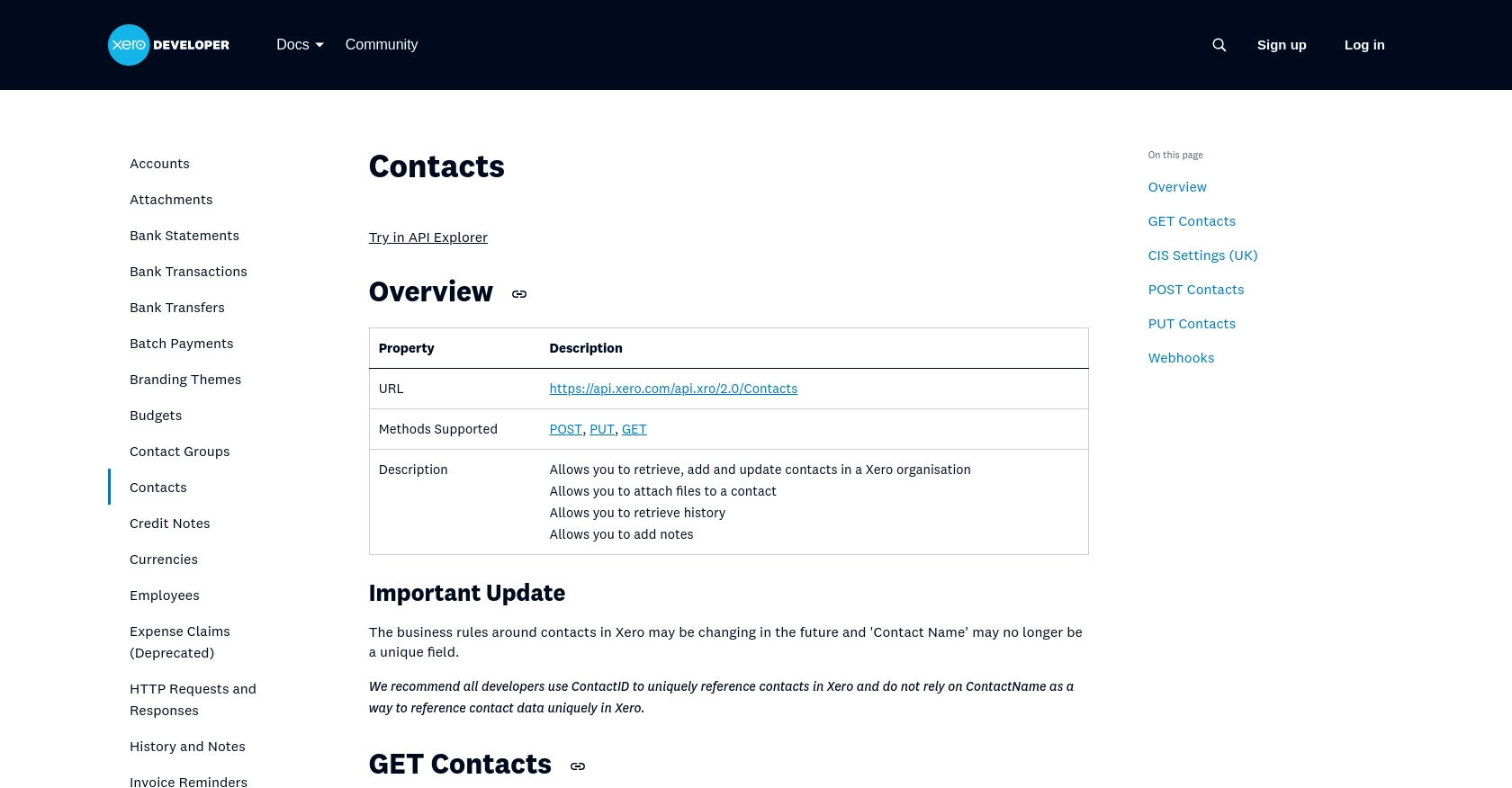
Conclusion and Best Practices for Xero API Integration
Integrating with the Xero API using Python allows developers to automate and streamline customer management processes, ensuring that customer data remains accurate and up-to-date. By following the steps outlined in this guide, you can efficiently create or update customer records within Xero, leveraging the power of Python and the Xero API.
Best Practices for Secure and Efficient Xero API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be aware of Xero's API rate limits to avoid exceeding them. Implement retry logic with exponential backoff to handle rate limit responses gracefully. For more details, refer to the Xero API rate limits documentation.
- Transform and Standardize Data: Ensure that customer data is consistently formatted before sending it to Xero. This helps maintain data integrity and prevents errors during API calls.
- Monitor and Log API Requests: Implement logging to track API requests and responses. This will help in debugging issues and understanding the interaction patterns with the Xero API.
Streamline Your Integration Process with Endgrate
While integrating with Xero's API can be a powerful way to enhance your application's capabilities, it can also be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Xero. By using Endgrate, you can save time and resources, allowing you to focus on your core product and deliver an intuitive integration experience for your customers.
Explore how Endgrate can help you streamline your integration efforts by visiting Endgrate today.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/contacts
Ready to get started?