How to Create or Update Organizations with the Affinity API in PHP
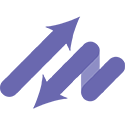
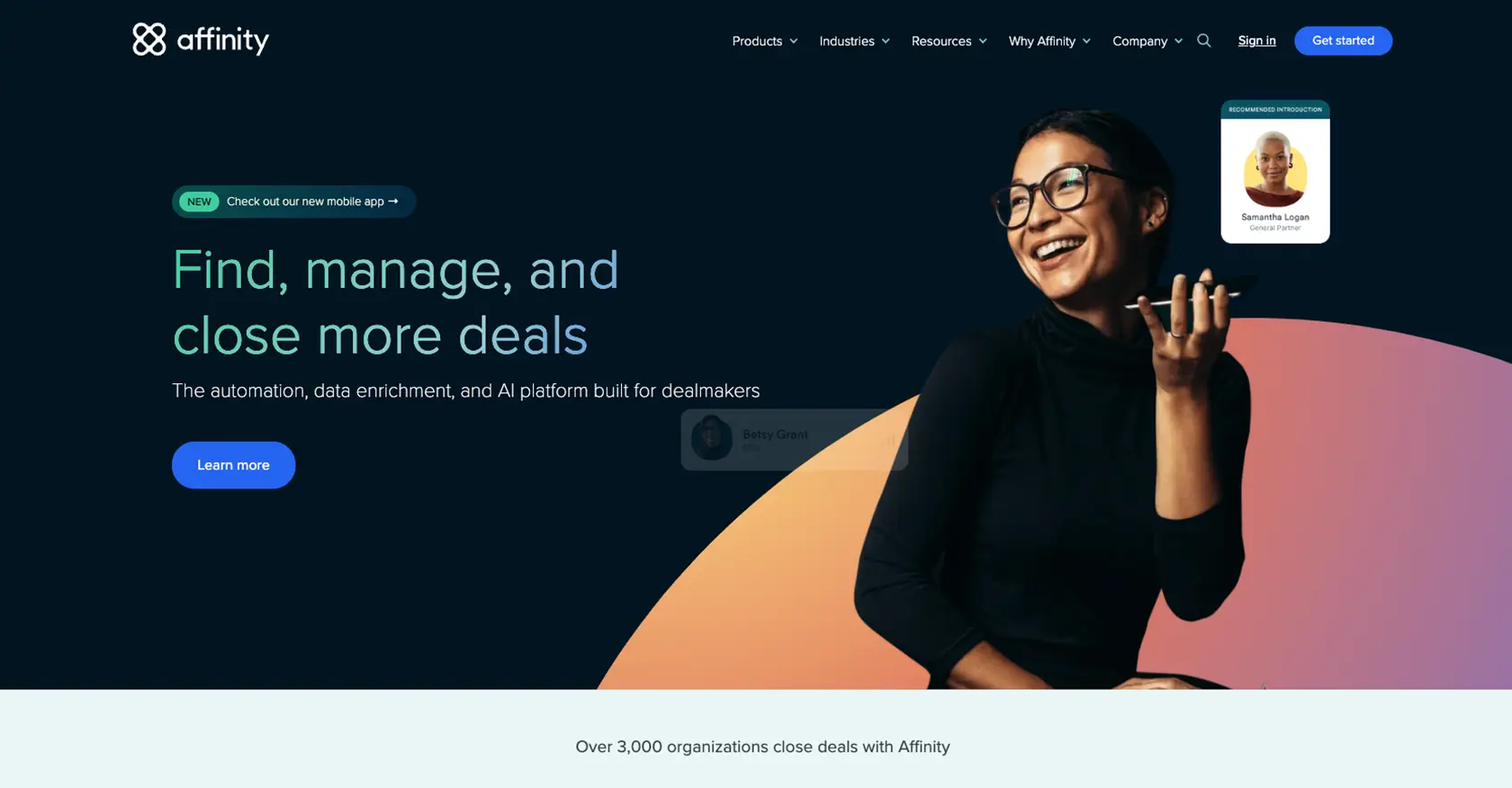
Introduction to Affinity API for Organization Management
Affinity is a powerful relationship intelligence platform designed to help businesses manage and leverage their professional networks. It offers a comprehensive suite of tools that enable users to track interactions, manage contacts, and analyze relationship data to drive business growth.
Integrating with the Affinity API allows developers to automate and streamline the management of organizational data. By using the API, developers can create or update organization records, ensuring that their CRM systems are always up-to-date with the latest information. This integration can be particularly useful for businesses looking to maintain accurate records of their interactions with various organizations, facilitating better relationship management and strategic decision-making.
For example, a developer might use the Affinity API to automatically update an organization's details whenever there is a change in its contact information or to add new organizations as they are identified through business development activities.
Setting Up Your Affinity API Test Account
Before you can start integrating with the Affinity API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to create your test environment:
Create an Affinity Account
- Visit the Affinity website and sign up for a free trial or demo account.
- Follow the on-screen instructions to complete the registration process.
- Once your account is created, log in to access the Affinity dashboard.
Generate an API Key for Affinity Integration
Affinity uses API key-based authentication to secure API requests. Here's how to generate your API key:
- Navigate to the Settings Panel, accessible from the left sidebar of the Affinity web app.
- Locate the API section and click on it to open the API settings.
- Click on "Generate API Key" to create a new key.
- Copy the generated API key and store it securely, as you will need it for authentication in your API requests.
For more detailed instructions, refer to the Affinity API documentation.
Configure Your PHP Environment
To interact with the Affinity API using PHP, ensure your development environment is set up correctly:
- Install PHP 7.4 or later on your machine.
- Ensure you have access to a package manager like Composer to manage dependencies.
- Install the necessary PHP extensions for making HTTP requests, such as cURL.
With your test account and API key ready, you can now proceed to make API calls to create or update organizations in Affinity.
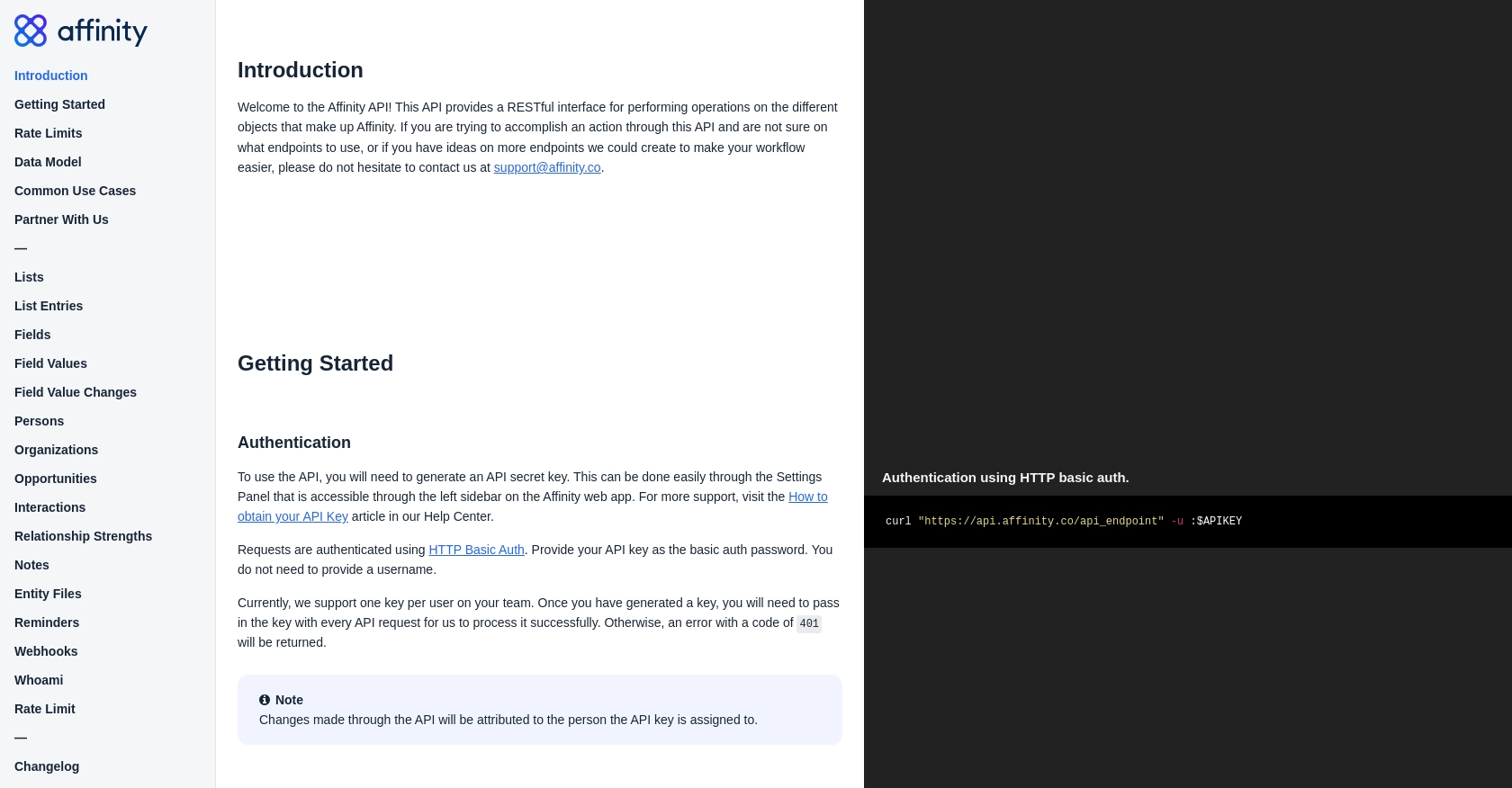
sbb-itb-96038d7
Making API Calls to Create or Update Organizations with Affinity API in PHP
To interact with the Affinity API for creating or updating organizations, you'll need to make HTTP requests using PHP. This section will guide you through the process of setting up your PHP environment, writing the necessary code, and handling responses effectively.
Setting Up Your PHP Environment for Affinity API Integration
Ensure your PHP environment is ready to make HTTP requests:
- Install PHP 7.4 or later.
- Use Composer to manage dependencies.
- Ensure the cURL extension is enabled for making HTTP requests.
Writing PHP Code to Interact with Affinity API
Below is a sample PHP script to create or update an organization using the Affinity API:
<?php
// Affinity API endpoint for creating or updating organizations
$endpoint = "https://api.affinity.co/organizations";
// Your API key
$apiKey = "Your_API_Key";
// Organization data
$data = [
"name" => "New Organization",
"domain" => "neworg.com",
"person_ids" => [12345, 67890]
];
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Content-Type: application/json",
"Authorization: Basic " . base64_encode(":$apiKey")
]);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Decode the response
$responseData = json_decode($response, true);
echo "Organization ID: " . $responseData['id'];
}
// Close the cURL session
curl_close($ch);
?>
Handling API Responses and Errors
After executing the API call, it's crucial to handle the response correctly:
- Check for HTTP errors using
curl_errno()
andcurl_error()
. - Decode the JSON response to access the data returned by the API.
- Handle specific error codes as outlined in the Affinity API documentation, such as 401 for unauthorized access or 429 for rate limits.
Verifying API Call Success in Affinity Dashboard
To ensure your API call was successful, verify the changes in your Affinity dashboard:
- Log in to your Affinity account.
- Navigate to the Organizations section to see the newly created or updated organization.
By following these steps, you can efficiently manage organizations in Affinity using PHP, ensuring your CRM data remains accurate and up-to-date.
Conclusion and Best Practices for Using Affinity API with PHP
Integrating with the Affinity API using PHP provides a robust solution for managing organizational data efficiently. By automating the creation and updating of organization records, businesses can ensure their CRM systems are always current, facilitating better relationship management and strategic decision-making.
Best Practices for Secure and Efficient Affinity API Integration
- Secure API Key Storage: Always store your API keys securely and avoid hardcoding them in your scripts. Consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of the Affinity API's rate limits. Implement retry logic with exponential backoff to handle 429 errors gracefully. According to the Affinity API documentation, the per-minute limit is 900 requests per user.
- Data Validation: Validate and sanitize all inputs before making API calls to prevent errors and ensure data integrity.
- Error Handling: Implement comprehensive error handling to manage different HTTP status codes effectively, especially 401 (Unauthorized) and 500 (Internal Server Error).
- Data Transformation: Standardize and transform data fields as necessary to maintain consistency across different systems.
Leverage Endgrate for Streamlined Integration Solutions
While integrating with APIs like Affinity can be powerful, it can also be time-consuming and complex. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to multiple platforms, including Affinity. This allows developers to focus on core product development while outsourcing integration tasks.
By using Endgrate, you can save time and resources, build once for each use case, and provide an intuitive integration experience for your customers. Explore more about how Endgrate can enhance your integration processes by visiting Endgrate.
Read More
Ready to get started?