Using the Insightly API to Create or Update Contacts in PHP
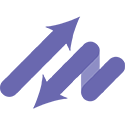
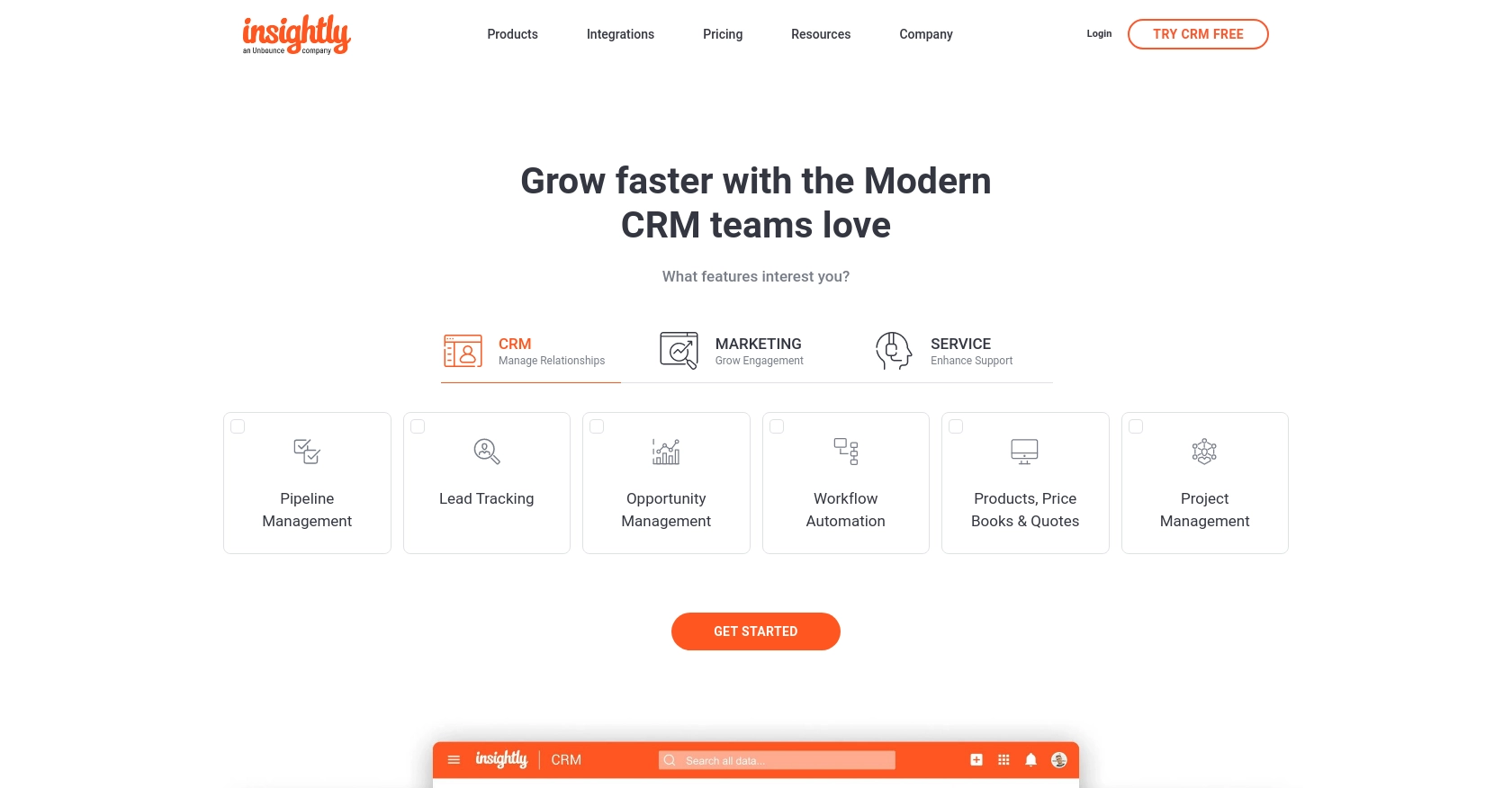
Introduction to Insightly CRM and Its API
Insightly is a powerful CRM platform that helps businesses manage their customer relationships, projects, and sales processes. Known for its user-friendly interface and robust features, Insightly is a popular choice for businesses looking to streamline their operations and improve customer engagement.
Developers may want to integrate with Insightly's API to automate and enhance their CRM functionalities. For example, using the Insightly API, a developer can create or update contact information directly from an external application, ensuring that customer data is always up-to-date and accessible across platforms.
This article will guide you through the process of using PHP to interact with the Insightly API, specifically focusing on creating or updating contacts. By following this tutorial, you can efficiently manage contact data within Insightly, leveraging its API to enhance your business processes.
Setting Up Your Insightly Test Account for API Integration
Before you can start using the Insightly API to create or update contacts, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data.
Creating an Insightly Account
If you don't already have an Insightly account, you can sign up for a free trial or use the free version. Visit the Insightly signup page and follow the instructions to create your account. Once your account is set up, log in to access the Insightly dashboard.
Generating Your Insightly API Key
Insightly uses API key-based authentication to authorize API requests. Follow these steps to obtain your API key:
- Log in to your Insightly account.
- Click on your user profile in the upper right corner and select User Settings.
- Navigate to the API Key and URL section.
- Your API key will be displayed here. Copy this key and keep it secure, as you'll need it to authenticate your API requests.
For more detailed instructions, you can refer to the Insightly API key documentation.
Understanding Insightly API Authentication
Insightly's API uses HTTP Basic authentication with the API key as the username and a blank password. When making API calls, ensure that your API key is Base64-encoded in the Authorization header. If you're testing the API via the sandbox, you can paste your API key directly without encoding.
For more information on authentication, visit the Insightly API documentation.
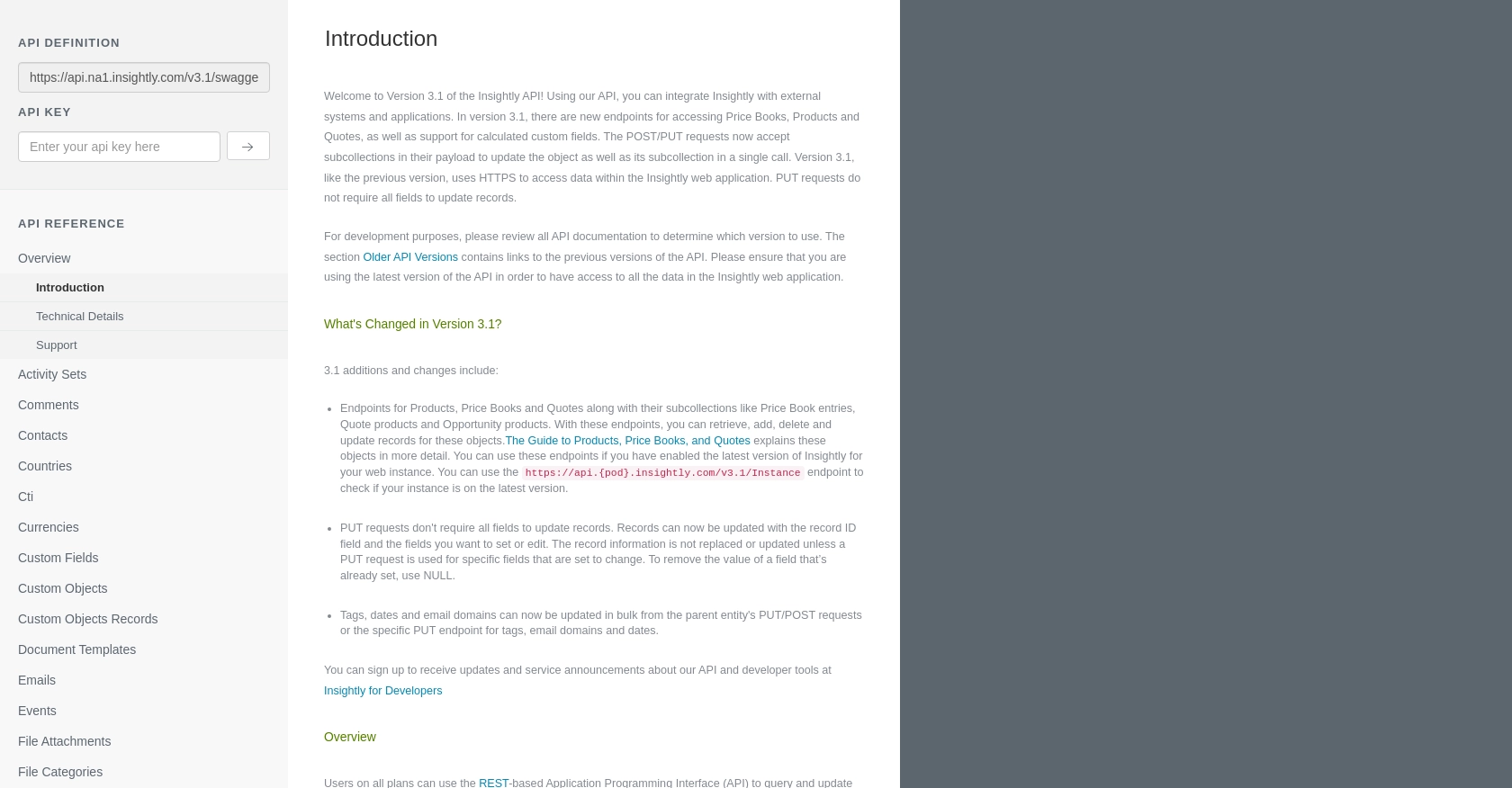
sbb-itb-96038d7
How to Make API Calls to Insightly for Creating or Updating Contacts Using PHP
To interact with the Insightly API for creating or updating contacts, you'll need to use PHP to send HTTP requests. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to make API calls, and handling responses.
Setting Up Your PHP Environment for Insightly API Integration
Before making API calls, ensure you have the following prerequisites:
- PHP version 7.4 or higher installed on your machine.
- The
cURL
extension enabled in your PHP configuration.
To verify your PHP version and extensions, you can run the following command in your terminal:
php -v
Ensure that cURL
is listed among the enabled extensions.
Writing PHP Code to Create or Update Contacts in Insightly
Now, let's write the PHP code to create or update contacts using the Insightly API. We'll use the cURL
library to handle HTTP requests.
<?php
// Set your Insightly API key
$apiKey = 'Your_API_Key';
// Base64 encode the API key for authentication
$authHeader = 'Authorization: Basic ' . base64_encode($apiKey . ':');
// Define the API endpoint for creating or updating contacts
$endpoint = 'https://api.na1.insightly.com/v3.1/Contacts';
// Create a new contact or update an existing one
$contactData = [
'FIRST_NAME' => 'John',
'LAST_NAME' => 'Doe',
'EMAIL' => 'john.doe@example.com'
];
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, [
$authHeader,
'Content-Type: application/json'
]);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($contactData));
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Parse the response
$responseData = json_decode($response, true);
echo 'Contact ID: ' . $responseData['CONTACT_ID'];
}
// Close cURL session
curl_close($ch);
?>
Replace Your_API_Key
with your actual Insightly API key. This script sends a POST request to the Insightly API to create or update a contact. The response will include the contact ID if successful.
Verifying API Call Success and Handling Errors
After executing the API call, you should verify the success of the request:
- Check the HTTP status code in the response. A status code of
200
indicates success. - Inspect the response data to ensure the contact was created or updated as expected.
If you encounter errors, the Insightly API documentation provides guidance on error codes and troubleshooting. Common issues include authentication errors (401) and rate limit exceeded errors (429).
Testing API Calls in the Insightly Sandbox Environment
To ensure your API calls work correctly, test them in the Insightly sandbox environment. This allows you to safely experiment without affecting live data. You can verify the creation or update of contacts by checking the sandbox account for changes.
Conclusion and Best Practices for Using the Insightly API with PHP
Integrating with the Insightly API using PHP offers a powerful way to automate CRM processes and ensure that your contact data is always current and accessible. By following the steps outlined in this guide, you can efficiently create or update contacts within Insightly, enhancing your business operations.
Best Practices for Secure and Efficient API Integration
- Securely Store API Keys: Always store your API keys securely and avoid hardcoding them directly in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of Insightly's rate limits, which allow up to 10 requests per second and vary daily based on your plan. Implement logic to handle HTTP 429 errors gracefully by retrying requests after a delay.
- Standardize Data Fields: Ensure consistent data formats across your applications to avoid discrepancies when creating or updating contacts.
- Monitor API Usage: Regularly review your API usage to optimize performance and identify any potential issues early.
Enhancing Your Integration Strategy with Endgrate
While integrating with Insightly's API can significantly improve your CRM capabilities, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Insightly.
By leveraging Endgrate, you can focus on your core product development while outsourcing integration tasks. This not only saves time and resources but also ensures a seamless integration experience for your customers.
Explore how Endgrate can streamline your integration strategy by visiting Endgrate and discover the benefits of a unified API solution.
Read More
Ready to get started?