Using the Quickbooks API to Create Or Update Products (with Python examples)
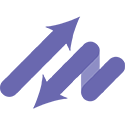
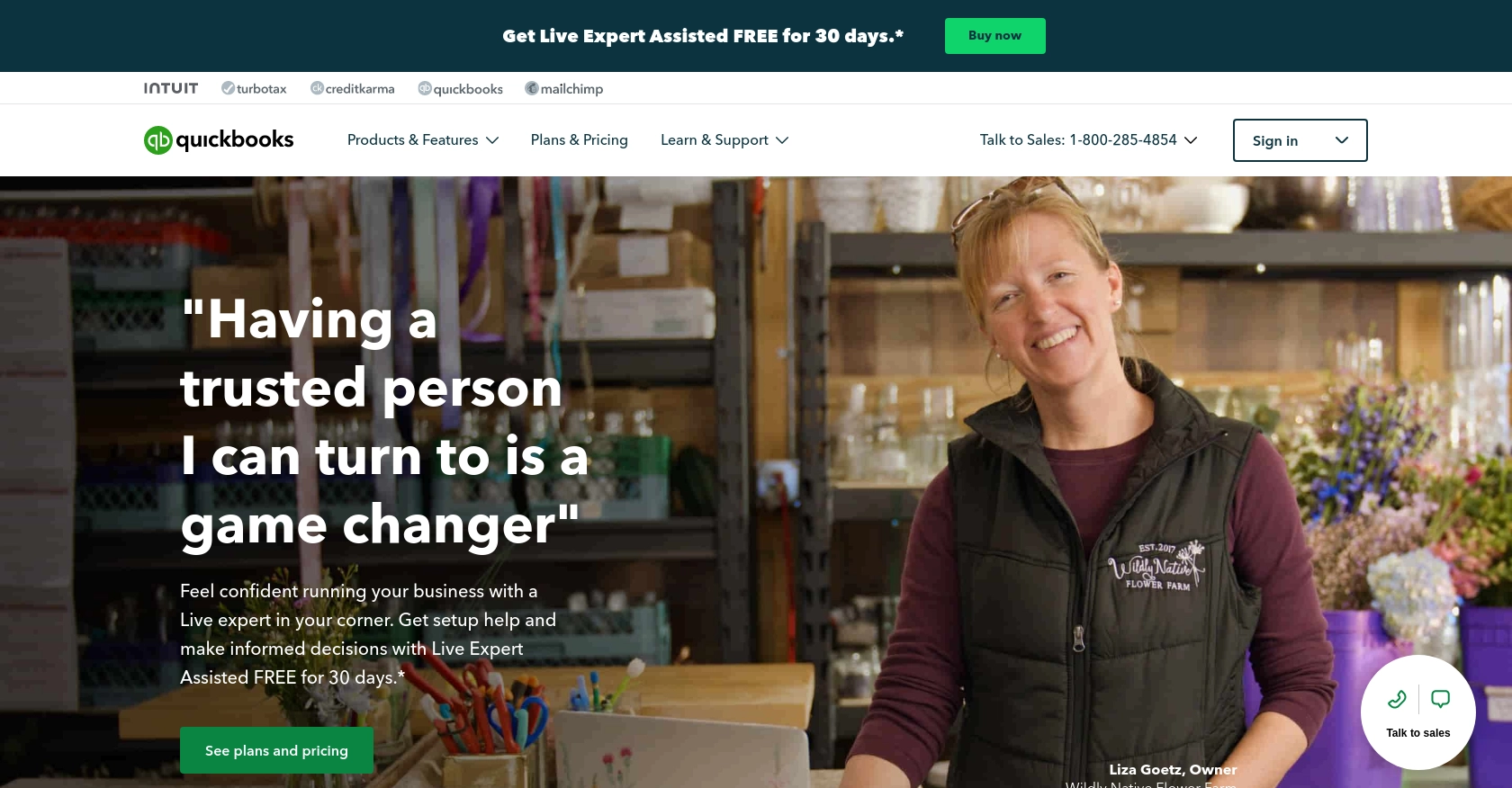
Introduction to QuickBooks API Integration
QuickBooks is a widely-used accounting software that helps businesses manage their financial operations efficiently. It offers a range of features including invoicing, expense tracking, payroll, and financial reporting, making it an essential tool for small to medium-sized enterprises.
Integrating with the QuickBooks API allows developers to automate and streamline accounting tasks, such as creating or updating product information. For example, a developer might use the QuickBooks API to automatically update inventory levels or add new products to the system, ensuring that the accounting records are always up-to-date and accurate.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start integrating with the QuickBooks API, you'll need to set up a sandbox account. This allows you to test your application in a safe environment without affecting real data. Follow these steps to create your QuickBooks sandbox account and obtain the necessary credentials for OAuth authentication.
Creating a QuickBooks Developer Account
If you don't already have a QuickBooks Developer account, you'll need to create one. Visit the Intuit Developer Portal and sign up for a free account. Once registered, log in to access the developer dashboard.
Setting Up a QuickBooks Sandbox Company
After logging in, navigate to the "Sandbox" section in the dashboard. Here, you can create a new sandbox company, which will serve as your testing environment. Follow the on-screen instructions to set up your sandbox company, which will mimic a real QuickBooks account.
Creating a QuickBooks App for OAuth Authentication
To interact with the QuickBooks API, you'll need to create an app within your developer account. This app will provide you with the client ID and client secret required for OAuth authentication.
- Go to the "My Apps" section in the developer dashboard.
- Click on "Create an App" and select "QuickBooks Online and Payments" as the platform.
- Fill in the necessary details such as app name and description.
- Once the app is created, navigate to the "Keys & OAuth" section to find your client ID and client secret.
Make sure to securely store these credentials as they are essential for authenticating API requests.
Configuring OAuth Settings
In the app settings, you'll need to configure the OAuth redirect URI. This is the URL where users will be redirected after they authorize your app. Ensure that this URI matches the one used in your application code.
For more detailed instructions, refer to the official Intuit Developer Documentation.
Generating Access Tokens
With your client ID and client secret, you can now generate access tokens to authenticate API requests. Use the OAuth 2.0 flow to obtain these tokens, which will allow your application to access QuickBooks data.
For further guidance on OAuth implementation, consult the Intuit Developer Documentation.
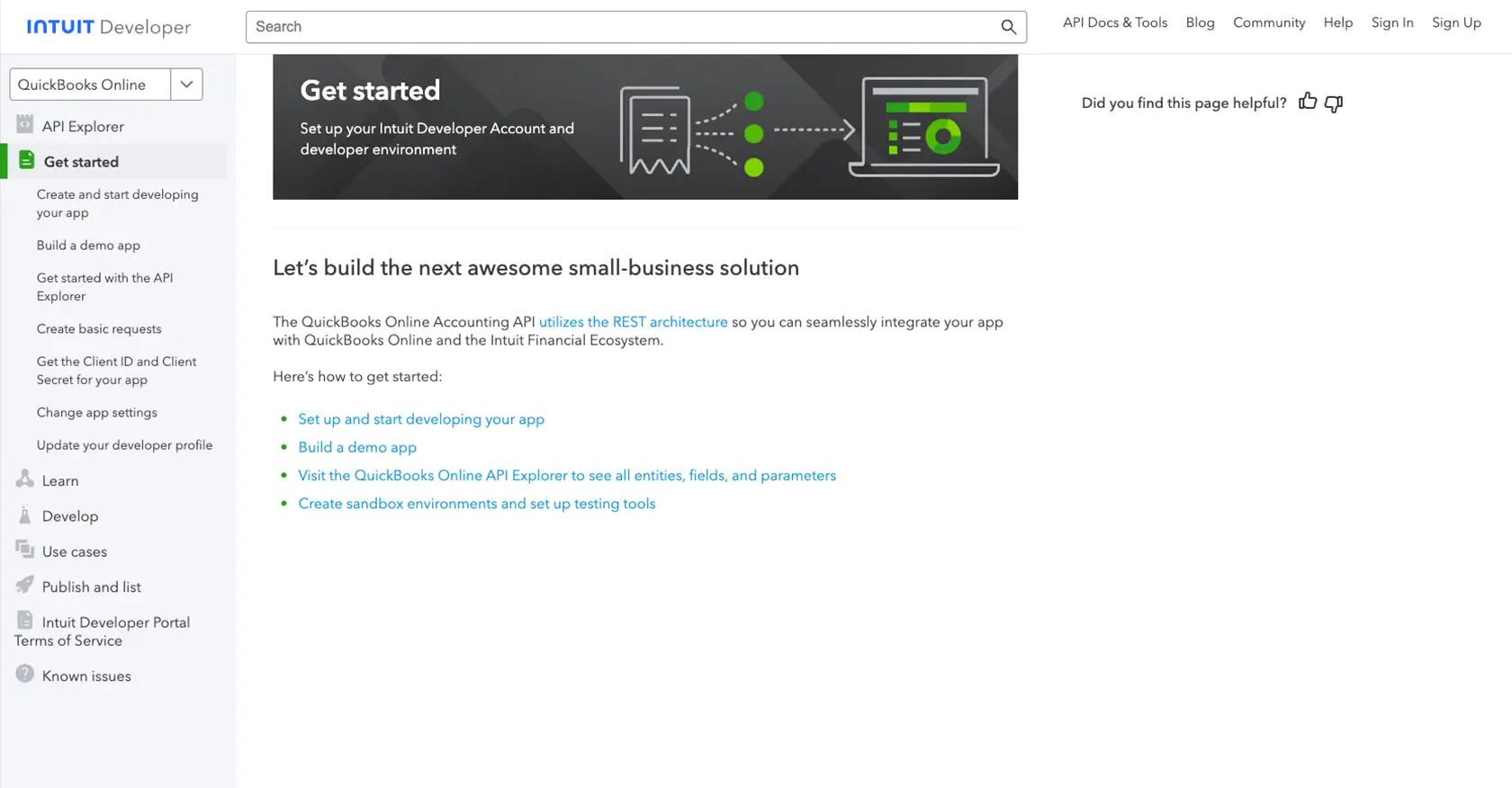
sbb-itb-96038d7
Making API Calls to QuickBooks for Creating or Updating Products Using Python
To interact with the QuickBooks API for creating or updating products, you'll need to use Python. This section will guide you through the necessary steps, including setting up your Python environment, writing the code to make API calls, and handling responses and errors.
Setting Up Your Python Environment for QuickBooks API Integration
Before you begin, ensure you have Python 3.11.1 installed on your machine. You'll also need to install the requests
library, which is used to make HTTP requests. You can install it using pip:
pip install requests
Creating or Updating Products in QuickBooks Using Python
To create or update products in QuickBooks, you'll need to make POST requests to the QuickBooks API. Below is a sample Python script that demonstrates how to do this:
import requests
import json
# Set the API endpoint for creating or updating products
url = "https://quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/item"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Accept": "application/json"
}
# Define the product data
product_data = {
"Name": "New Product",
"Type": "Inventory",
"IncomeAccountRef": {
"value": "79",
"name": "Sales of Product Income"
},
"ExpenseAccountRef": {
"value": "80",
"name": "Cost of Goods Sold"
},
"AssetAccountRef": {
"value": "81",
"name": "Inventory Asset"
}
}
# Make the POST request to create or update the product
response = requests.post(url, headers=headers, data=json.dumps(product_data))
# Check if the request was successful
if response.status_code == 200:
print("Product created or updated successfully.")
print(response.json())
else:
print("Failed to create or update product.")
print(response.status_code, response.text)
Replace YOUR_COMPANY_ID
and YOUR_ACCESS_TOKEN
with your actual QuickBooks company ID and access token.
Verifying API Call Success in QuickBooks Sandbox
After running the script, you can verify the success of your API call by checking the QuickBooks sandbox environment. Navigate to the products section to see if the new product has been created or updated.
Handling Errors and QuickBooks API Error Codes
It's crucial to handle potential errors when making API calls. The QuickBooks API may return various error codes, such as:
- 400 - Bad Request: The request was invalid or cannot be otherwise served.
- 401 - Unauthorized: Authentication failed or user does not have permissions for the desired action.
- 404 - Not Found: The requested resource could not be found.
- 500 - Internal Server Error: An error occurred on the server.
Always check the response status code and handle errors appropriately to ensure robust integration.
For more details on error handling, refer to the Intuit Developer Documentation.
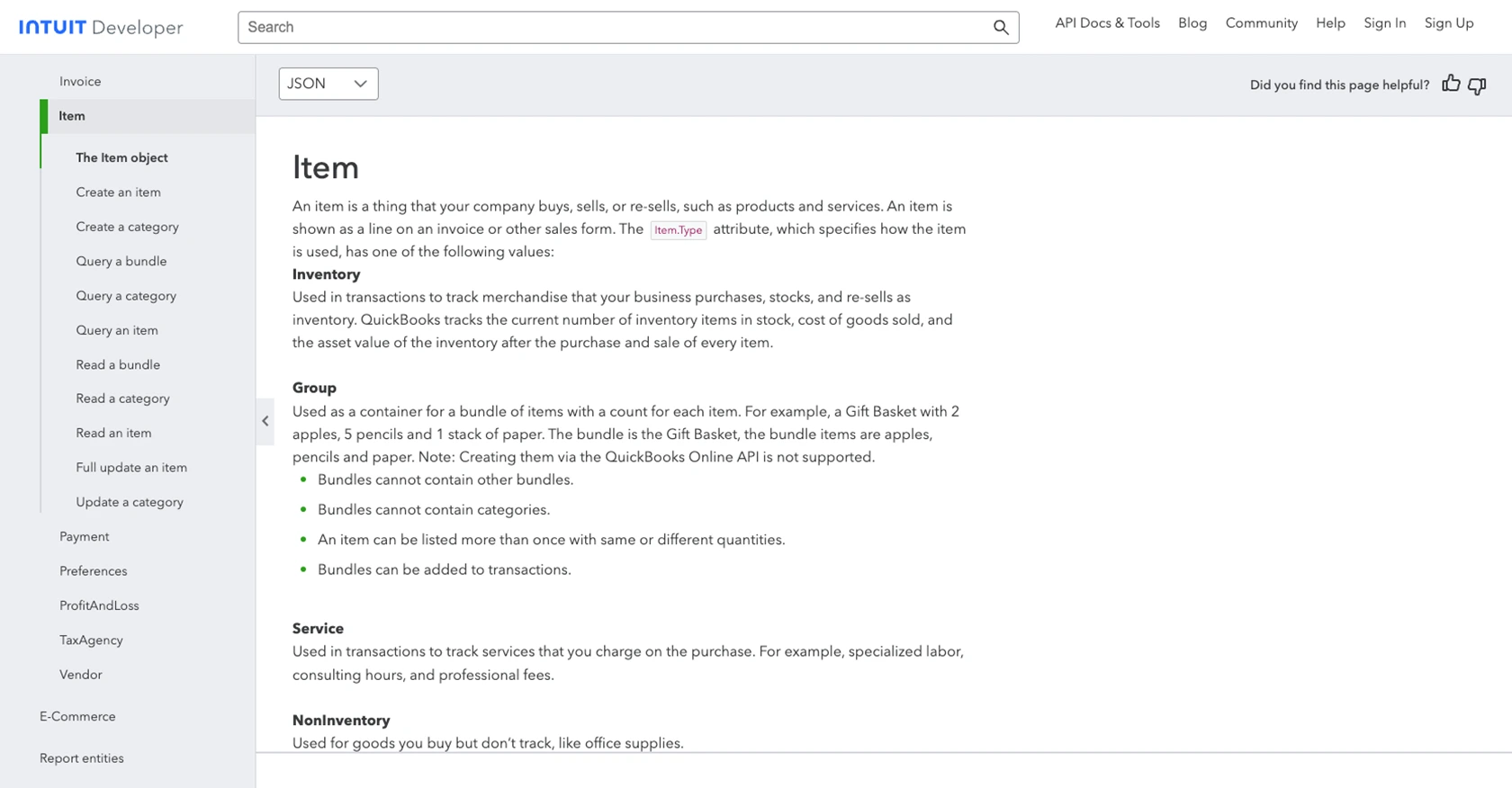
Conclusion and Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API can significantly enhance your business operations by automating accounting tasks and ensuring data accuracy. By following the steps outlined in this guide, you can efficiently create or update products using Python, streamlining your inventory management processes.
Best Practices for Secure and Efficient QuickBooks API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: QuickBooks API has rate limits to prevent abuse. Ensure your application handles these limits gracefully by implementing retry logic and monitoring API usage. For specific rate limit details, refer to the Intuit Developer Documentation.
- Standardize Data Fields: Consistently format and validate data before sending it to QuickBooks to avoid errors and ensure data integrity.
- Error Handling: Implement robust error handling to manage API response codes effectively. This will help maintain a seamless integration experience.
Streamline Your Integration Process with Endgrate
While integrating with QuickBooks API can be highly beneficial, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development. With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can save you time and resources by visiting Endgrate today.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/most-commonly-used/item
Ready to get started?