How to Create Deals with the Hubspot API in Javascript
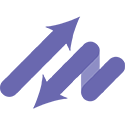
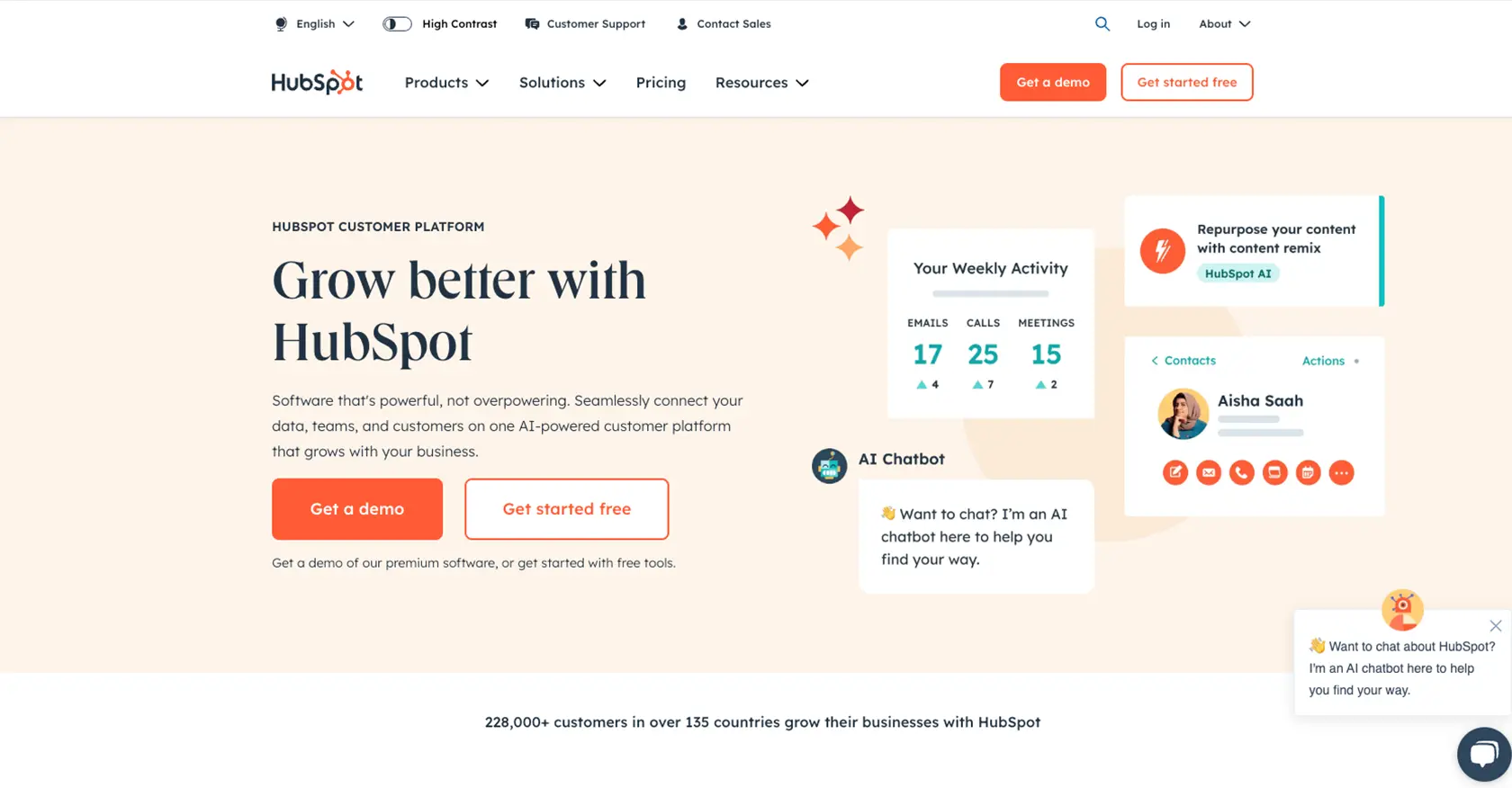
Introduction to HubSpot API Integration
HubSpot is a powerful CRM platform that offers a suite of tools to help businesses manage their marketing, sales, and customer service efforts. Its robust API allows developers to integrate HubSpot's capabilities into their own applications, enabling seamless data exchange and automation.
Developers might want to connect with HubSpot to streamline their sales processes by creating deals programmatically. For example, using the HubSpot API, a developer can automate the creation of deals in response to specific triggers, such as a new lead entering the sales funnel, ensuring that sales teams can focus on closing deals rather than manual data entry.
Setting Up Your HubSpot Test/Sandbox Account for API Integration
Before diving into creating deals with the HubSpot API using JavaScript, you need to set up a HubSpot test or sandbox account. This environment allows you to safely test your API calls without affecting live data.
Creating a HubSpot Developer Account
If you don't already have a HubSpot developer account, follow these steps to create one:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill in the required information.
- Once your account is created, log in to access the developer dashboard.
Setting Up a HubSpot Sandbox Account
HubSpot provides sandbox accounts for testing purposes. Here's how to set one up:
- In your HubSpot developer account, navigate to the "Test Accounts" section.
- Click on "Create a test account" to generate a new sandbox environment.
- Use this sandbox account to test your API integrations without impacting real data.
Configuring OAuth for HubSpot API Access
HubSpot uses OAuth for secure API access. Follow these steps to configure OAuth:
- In your developer account, go to "Apps" and click "Create a private app."
- Fill in the app details and navigate to the "Scopes" section.
- Select the necessary scopes for deals, such as
crm.objects.deals.write
andcrm.objects.deals.read
. - Save your app and note down the client ID and client secret for OAuth authentication.
For more detailed information on OAuth setup, refer to the HubSpot Authentication Documentation.
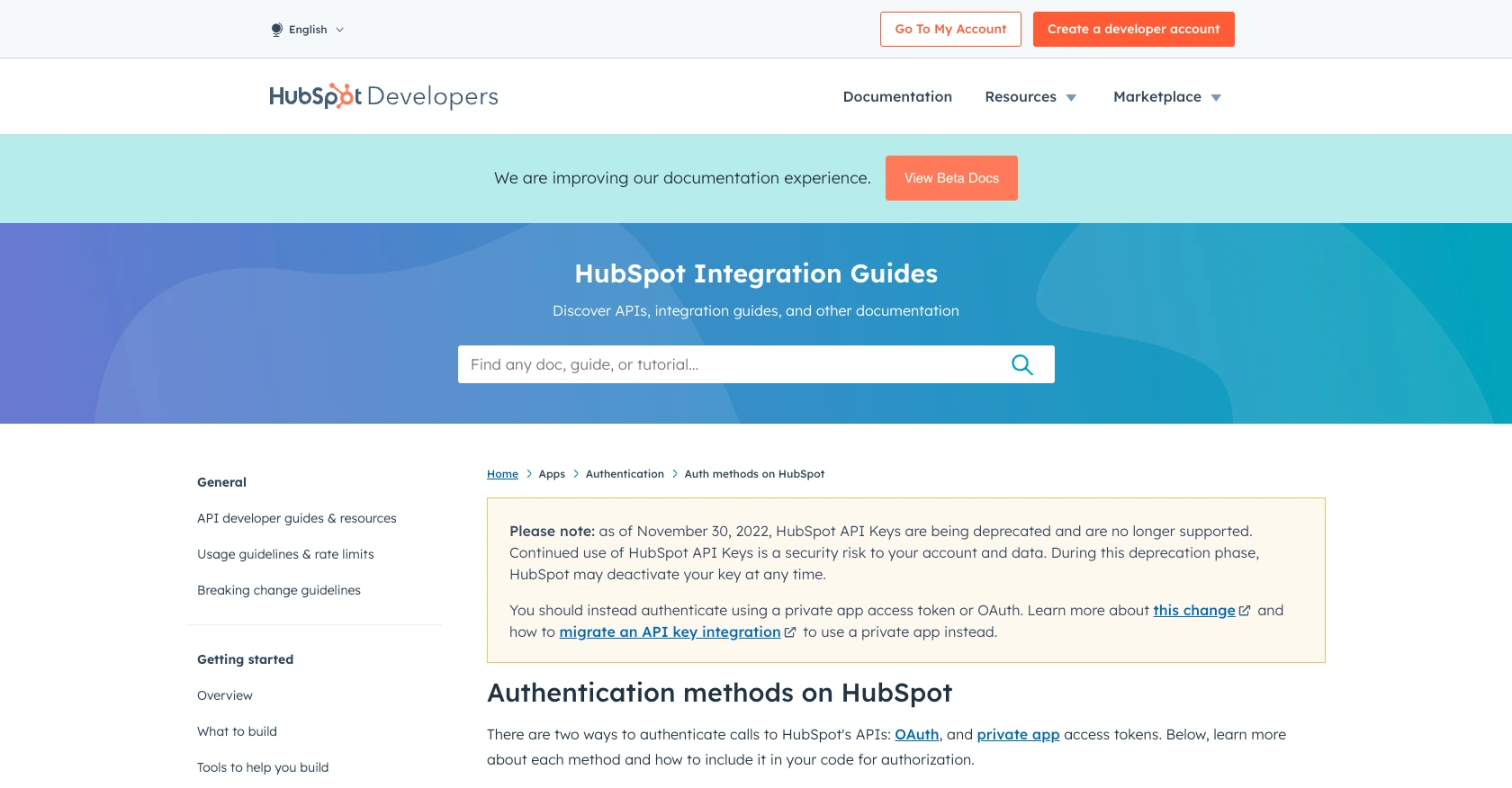
sbb-itb-96038d7
Making API Calls to Create Deals with HubSpot API in JavaScript
To create deals using the HubSpot API in JavaScript, you'll need to set up your development environment and write the necessary code to interact with the API. This section will guide you through the process, including setting up Node.js, installing dependencies, and writing the code to create deals in HubSpot.
Setting Up Your JavaScript Development Environment
Before you start coding, ensure you have the following installed on your machine:
- Node.js (version 14.x or higher)
- npm (Node Package Manager)
Once installed, create a new directory for your project and navigate into it:
mkdir hubspot-deals
cd hubspot-deals
Installing Required Node.js Packages
You'll need the axios
package to make HTTP requests to the HubSpot API. Install it using npm:
npm init -y
npm install axios
Writing JavaScript Code to Create Deals in HubSpot
Create a new file named createDeal.js
and add the following code:
const axios = require('axios');
// Set your HubSpot API endpoint and access token
const endpoint = 'https://api.hubapi.com/crm/v3/objects/deals';
const accessToken = 'Your_Access_Token';
// Define the deal data
const dealData = {
properties: {
dealname: 'New Deal',
amount: '1000',
dealstage: 'contractsent',
pipeline: 'default',
closedate: '2023-12-31T23:59:59Z'
}
};
// Function to create a deal
async function createDeal() {
try {
const response = await axios.post(endpoint, dealData, {
headers: {
Authorization: `Bearer ${accessToken}`,
'Content-Type': 'application/json'
}
});
console.log('Deal created successfully:', response.data);
} catch (error) {
console.error('Error creating deal:', error.response ? error.response.data : error.message);
}
}
// Execute the function
createDeal();
Replace Your_Access_Token
with the access token obtained from your HubSpot app setup.
Running Your JavaScript Code
To execute your code and create a deal in HubSpot, run the following command in your terminal:
node createDeal.js
Upon successful execution, you should see a confirmation message with the details of the created deal. If there are any errors, the error message will help you troubleshoot the issue.
Verifying Deal Creation in HubSpot
To verify that the deal was created successfully, log in to your HubSpot sandbox account and navigate to the Deals section. You should see the newly created deal listed there.
Handling Errors and Rate Limits
When making API calls, it's important to handle potential errors and respect HubSpot's rate limits. If you receive a 429 error, it means you've hit the rate limit. HubSpot's rate limit for OAuth apps is 100 requests every 10 seconds. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
For more details on error handling and rate limits, refer to the HubSpot API Usage Guidelines.
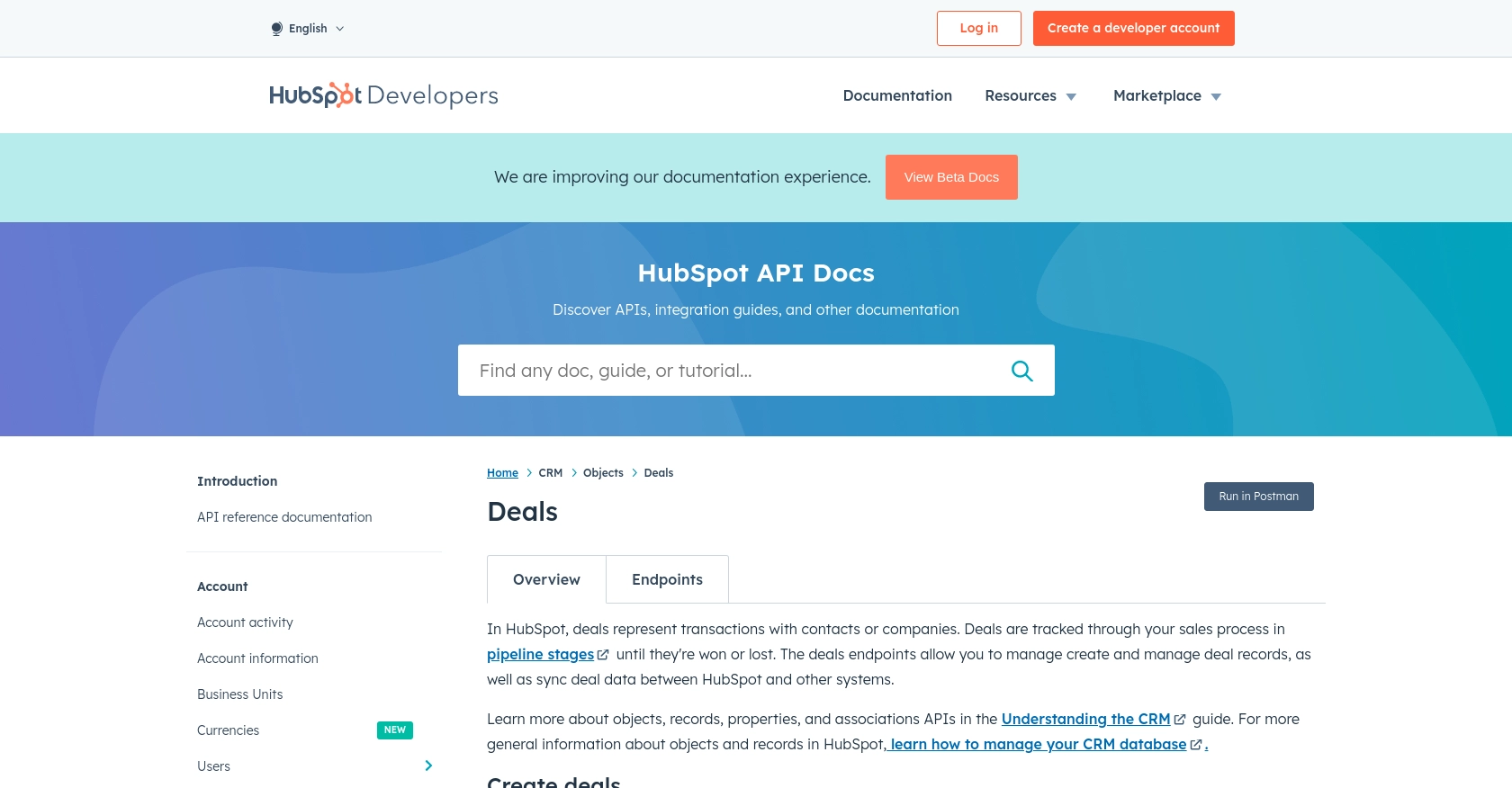
Best Practices for HubSpot API Integration and Deal Management
Successfully integrating with the HubSpot API to create deals is just the beginning. To ensure a robust and efficient integration, consider the following best practices:
Securely Storing User Credentials
Always store your OAuth tokens securely. Use environment variables or a secure vault to keep your credentials safe and avoid hardcoding them in your source code.
Handling HubSpot API Rate Limits
HubSpot enforces rate limits to ensure fair usage. For OAuth apps, the limit is 100 requests every 10 seconds. Implement retry logic with exponential backoff to handle 429 errors gracefully. This approach helps you stay within the limits while maintaining a smooth user experience.
Transforming and Standardizing Data Fields
Ensure that the data you send to HubSpot is clean and standardized. This includes formatting dates correctly and ensuring that all required fields are populated. Consistent data helps maintain the integrity of your CRM records.
Utilizing Endgrate for Streamlined Integrations
Consider using Endgrate to simplify your integration processes. With Endgrate, you can build once for each use case and leverage a unified API to connect with multiple platforms, including HubSpot. This not only saves time but also allows you to focus on your core product development.
By following these best practices, you can enhance your integration with HubSpot, ensuring a seamless and efficient workflow for managing deals and other CRM activities.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/deals
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?