Using the Quickbooks API to Get Customers (with Javascript examples)
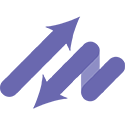
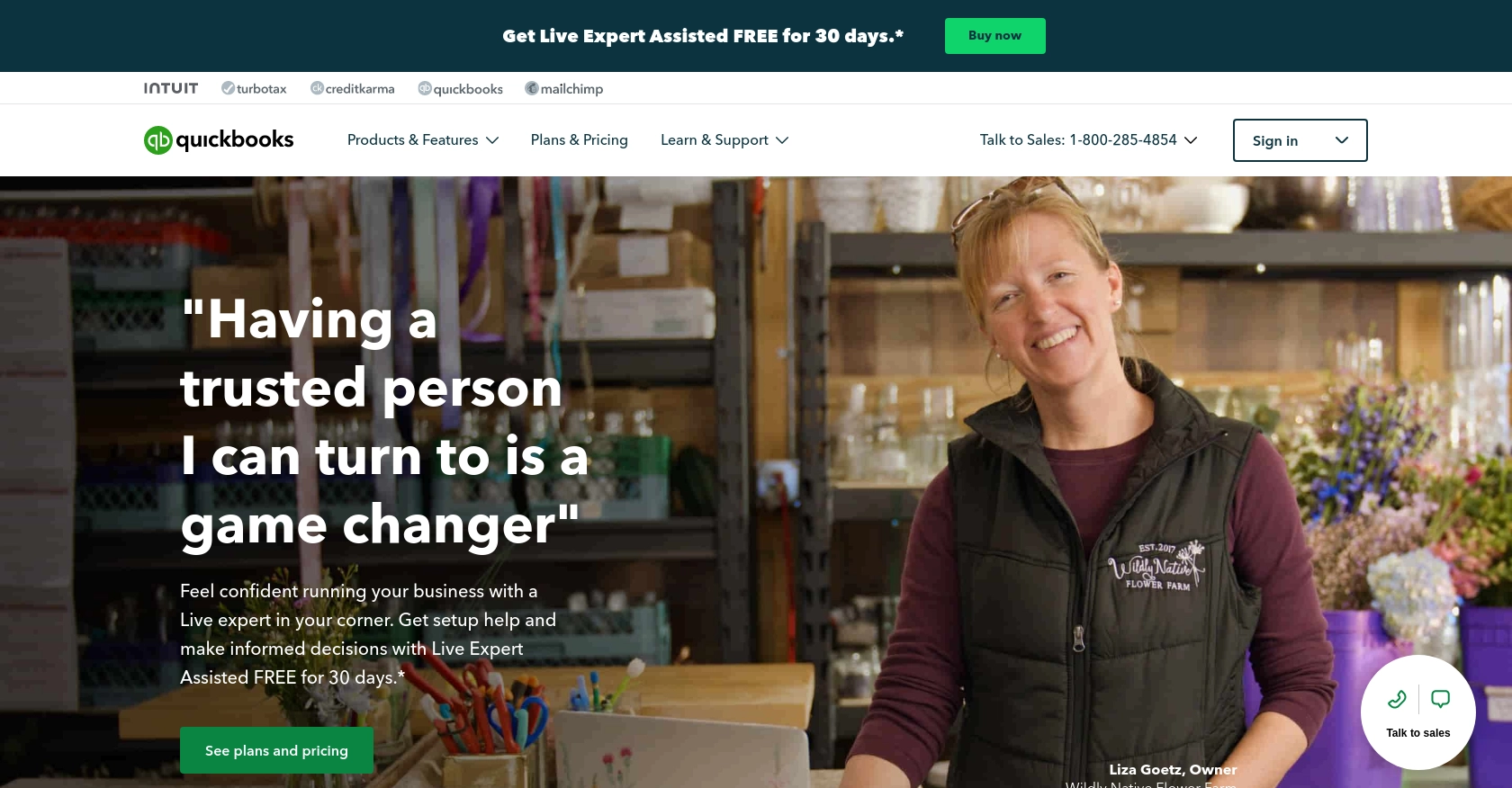
Introduction to Quickbooks API
Quickbooks is a widely-used accounting software that helps businesses manage their finances efficiently. It offers a range of features, including invoicing, expense tracking, payroll, and financial reporting, making it a popular choice for small to medium-sized enterprises.
Integrating with Quickbooks API allows developers to automate and streamline financial processes. For example, you can use the Quickbooks API to retrieve customer data, enabling seamless synchronization of customer information between your application and Quickbooks. This can be particularly useful for generating invoices, managing customer relationships, and enhancing overall business operations.
Setting Up Your Quickbooks Test/Sandbox Account
Before you can start interacting with the Quickbooks API, you need to set up a test or sandbox account. This environment allows you to safely test your integration without affecting live data.
Creating a Quickbooks Developer Account
If you don't already have a Quickbooks Developer account, you'll need to create one. Follow these steps to get started:
- Visit the Quickbooks Developer Portal.
- Click on "Sign Up" and fill in the required information to create your account.
- Once registered, log in to your Quickbooks Developer account.
Setting Up a Quickbooks Sandbox Company
After creating your developer account, you'll need to set up a sandbox company to test your API interactions:
- Navigate to the "Sandbox" section in your Quickbooks Developer dashboard.
- Click on "Add Sandbox" to create a new sandbox company.
- Follow the prompts to configure your sandbox environment.
Creating a Quickbooks App for OAuth Authentication
To interact with the Quickbooks API, you'll need to create an app to obtain the necessary OAuth credentials:
- Go to the App Management section in your Quickbooks Developer account.
- Click on "Create an App" and select "Quickbooks Online and Payments" as the platform.
- Fill in the required details for your app, such as name and description.
- Once your app is created, navigate to the "Keys & OAuth" section to find your Client ID and Client Secret.
Configuring OAuth Scopes and Redirect URIs
To ensure your app has the correct permissions, configure the OAuth scopes and redirect URIs:
- In the "Keys & OAuth" section, specify the necessary scopes for accessing customer data.
- Set up the redirect URIs that will handle the OAuth callback in your application.
With your sandbox account and app set up, you're ready to start making API calls to Quickbooks. For more detailed instructions, refer to the Quickbooks App Settings Documentation.
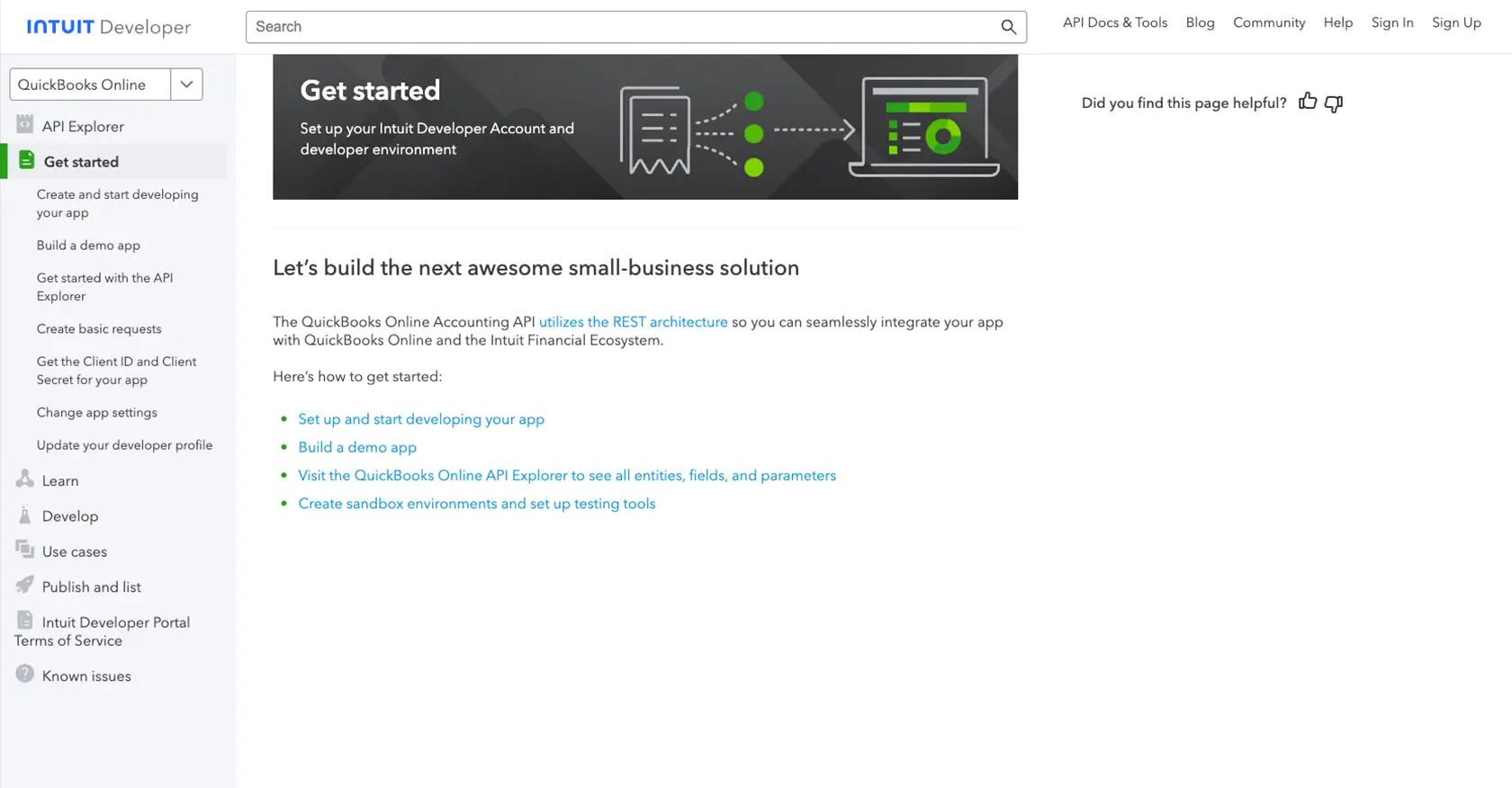
sbb-itb-96038d7
Making API Calls to Retrieve Customers from Quickbooks Using JavaScript
To interact with the Quickbooks API and retrieve customer data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for Quickbooks API
Before making API calls, ensure you have the following prerequisites installed:
- Node.js (version 14 or later)
- NPM (Node Package Manager)
Once you have Node.js and NPM installed, create a new project directory and initialize it:
mkdir quickbooks-api-example
cd quickbooks-api-example
npm init -y
Next, install the necessary dependencies:
npm install axios
Writing JavaScript Code to Retrieve Customers from Quickbooks
Create a file named getCustomers.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://sandbox-quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/query';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/text'
};
// Define the query to retrieve customers
const query = 'SELECT * FROM Customer';
// Function to get customers
async function getCustomers() {
try {
const response = await axios.post(endpoint, query, { headers });
const customers = response.data.QueryResponse.Customer;
console.log(customers);
} catch (error) {
console.error('Error fetching customers:', error.response ? error.response.data : error.message);
}
}
getCustomers();
Replace YOUR_COMPANY_ID
and YOUR_ACCESS_TOKEN
with your actual Quickbooks company ID and OAuth access token.
Running the JavaScript Code and Handling Responses
Run the script using the following command:
node getCustomers.js
If successful, you should see a list of customers printed in the console. This indicates that the API call was successful and the data was retrieved from Quickbooks.
Handling Errors and Verifying API Call Success
To ensure your API calls are successful, check the HTTP status codes returned by the API:
- 200: Success - The request was successful, and the response contains the requested data.
- 401: Unauthorized - Check your access token and ensure it is valid.
- 403: Forbidden - Verify that your app has the necessary permissions.
- 404: Not Found - Ensure the endpoint URL is correct.
Refer to the Quickbooks API Documentation for more detailed information on error codes and handling.
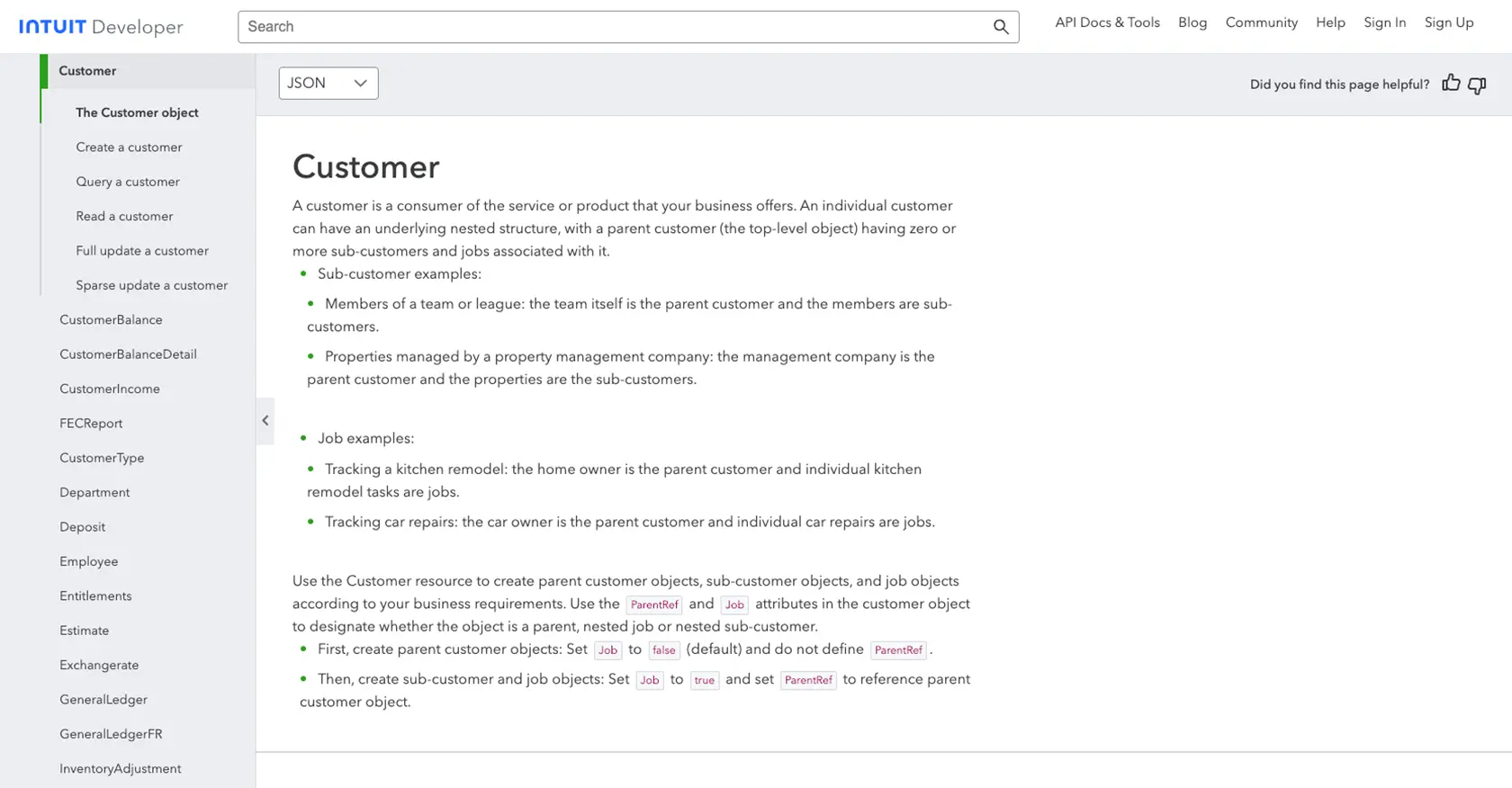
Conclusion and Best Practices for Using Quickbooks API with JavaScript
Integrating with the Quickbooks API using JavaScript can significantly enhance your application's ability to manage financial data efficiently. By automating the retrieval of customer information, you can streamline operations and improve data accuracy across platforms.
Best Practices for Secure and Efficient Quickbooks API Integration
- Securely Store Credentials: Always store your OAuth credentials, such as Client ID and Client Secret, securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Quickbooks API may impose rate limits. Ensure your application handles these limits gracefully by implementing retry logic and monitoring API usage. Refer to the Quickbooks API Documentation for specific rate limit details.
- Data Standardization: When retrieving customer data, consider transforming and standardizing fields to match your application's data model. This ensures consistency and simplifies data processing.
Enhance Your Integration Strategy with Endgrate
While integrating with Quickbooks API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that allows you to connect with various platforms, including Quickbooks, through a single endpoint. This approach saves time and resources, enabling you to focus on your core product development.
Explore how Endgrate can simplify your integration process by visiting Endgrate's website. With Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/customer
Ready to get started?