How to Get Contacts with the FreshDesk API in Javascript
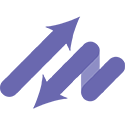
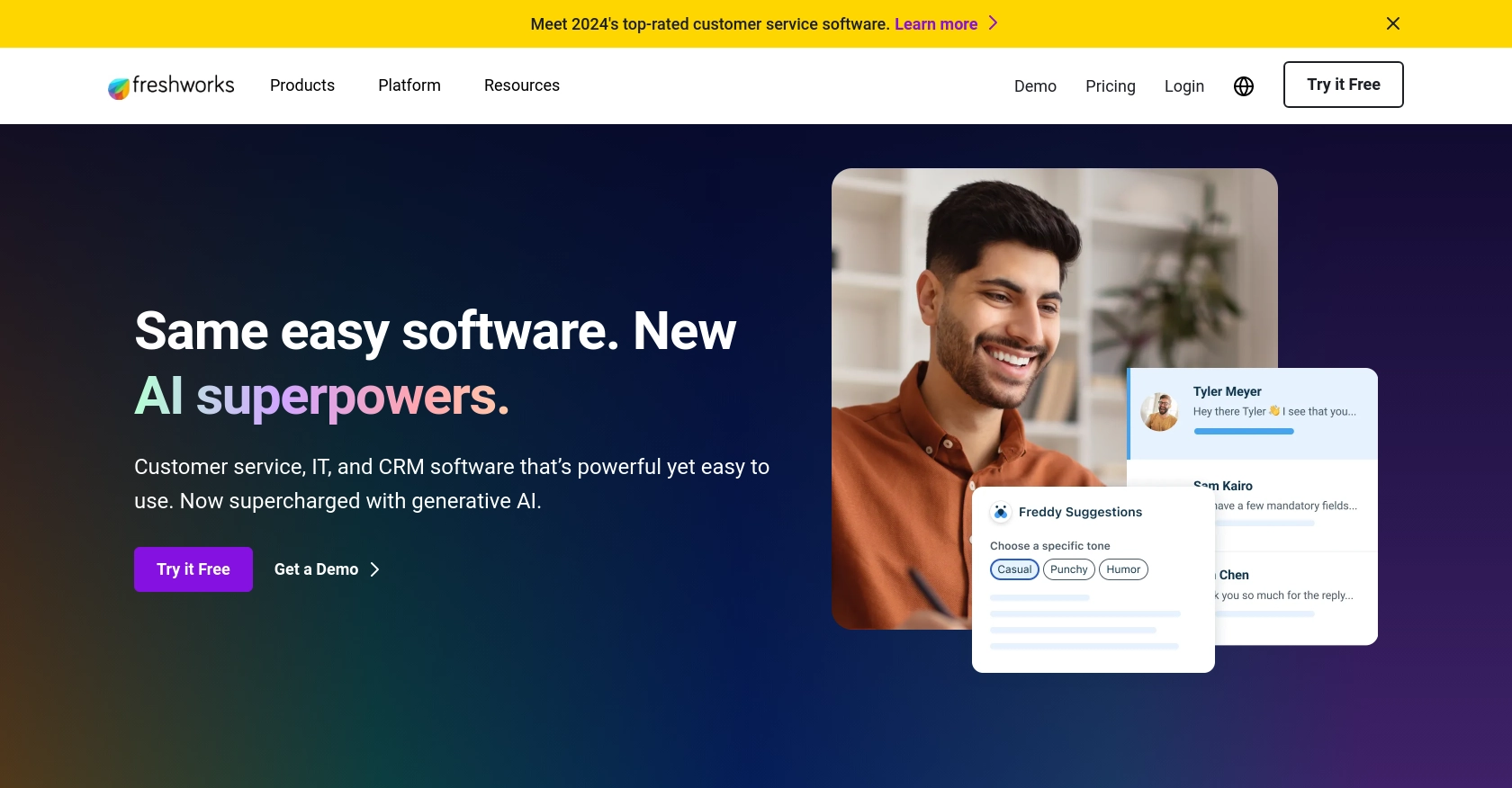
Introduction to FreshDesk API Integration
FreshDesk is a powerful customer support software that enables businesses to manage customer queries efficiently. It offers a suite of tools designed to streamline communication, automate repetitive tasks, and enhance customer satisfaction. With its robust API, FreshDesk allows developers to integrate its functionalities into their own applications, providing a seamless customer support experience.
Connecting with the FreshDesk API can be particularly beneficial for developers looking to manage customer data programmatically. For example, you might want to retrieve contact information from FreshDesk to synchronize it with your CRM system, ensuring that your sales and support teams have access to the most up-to-date customer information.
Setting Up Your FreshDesk Test or Sandbox Account
Before you can start interacting with the FreshDesk API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting any live data.
Creating a FreshDesk Account
If you don't already have a FreshDesk account, you can sign up for a free trial on the FreshDesk website. This trial will give you access to the full suite of FreshDesk features, including API access.
- Visit the FreshDesk signup page.
- Fill in the required details such as your name, email, and company information.
- Follow the instructions to complete the signup process.
Generating an API Key for FreshDesk
FreshDesk uses API key-based authentication to authorize API requests. Here's how you can generate your API key:
- Log in to your FreshDesk account.
- Navigate to your profile settings by clicking on your profile picture in the top right corner.
- Select "Profile Settings" from the dropdown menu.
- In the "Your API Key" section, you'll find your API key. Copy this key and store it securely, as you'll need it to authenticate your API requests.
Setting Up a FreshDesk Sandbox Environment
While FreshDesk does not provide a dedicated sandbox environment, you can use your trial account to test API interactions. Ensure that you use test data to avoid any disruptions to real customer data.
With your FreshDesk account set up and your API key in hand, you're ready to start making API calls to retrieve contact information and integrate FreshDesk functionalities into your applications.
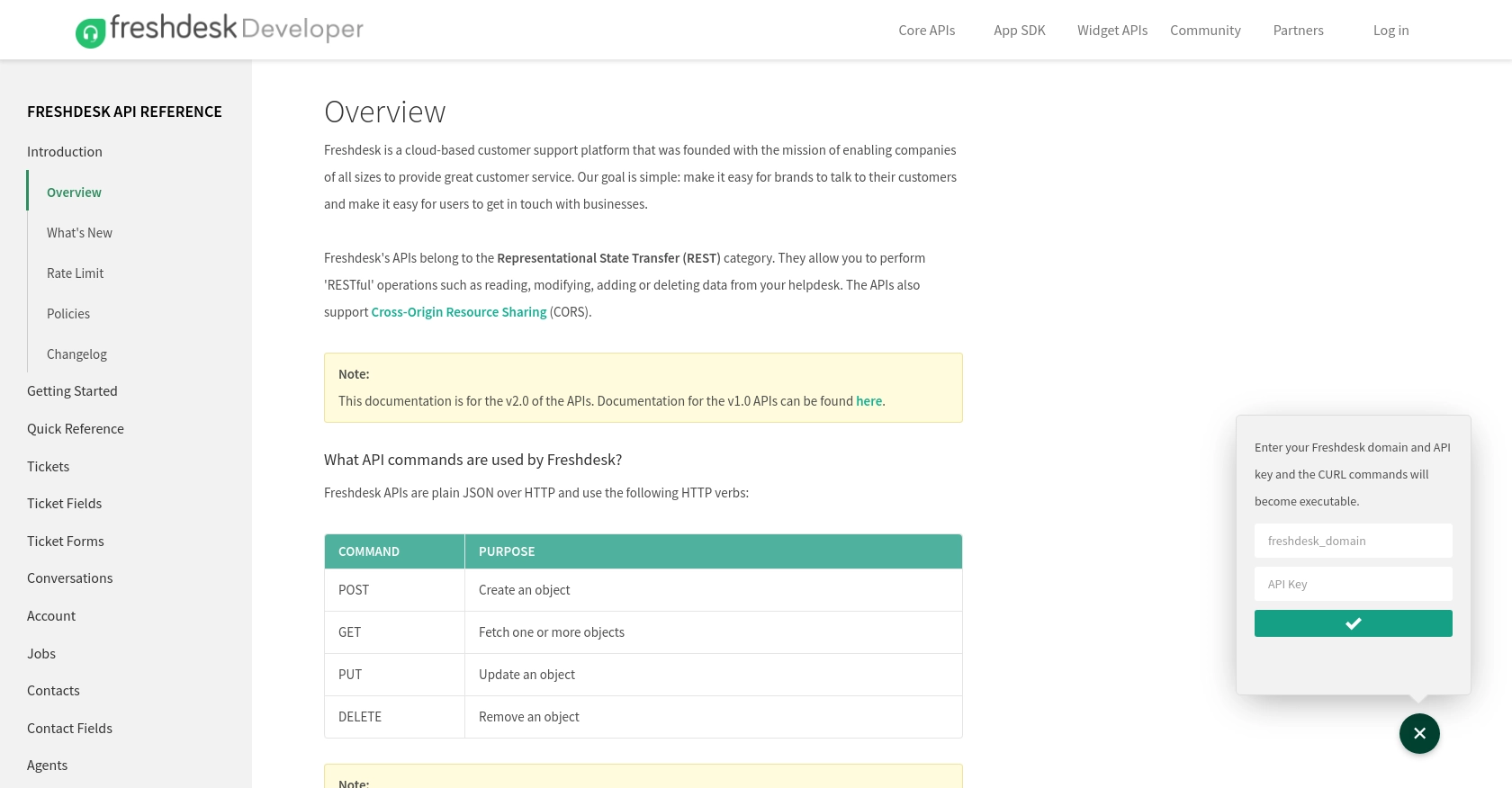
sbb-itb-96038d7
Making API Calls to Retrieve Contacts with FreshDesk API in JavaScript
To interact with the FreshDesk API using JavaScript, you'll need to ensure your development environment is properly set up. This section will guide you through the necessary steps to make successful API calls to retrieve contact information.
Setting Up Your JavaScript Environment for FreshDesk API
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides a runtime environment for executing JavaScript outside the browser, which is essential for server-side operations.
- Download and install Node.js from the official website.
- Verify the installation by running
node -v
andnpm -v
in your terminal to check the versions.
Next, you'll need to install the axios
library, a popular HTTP client for making requests in JavaScript:
npm install axios
Writing JavaScript Code to Fetch Contacts from FreshDesk
With your environment set up, you can now write the JavaScript code to interact with the FreshDesk API. Create a new file named getContacts.js
and add the following code:
const axios = require('axios');
// Set the FreshDesk API endpoint and your API key
const endpoint = 'https://yourdomain.freshdesk.com/api/v2/contacts';
const apiKey = 'Your_API_Key';
// Make a GET request to the FreshDesk API
axios.get(endpoint, {
auth: {
username: apiKey,
password: 'X' // Password can be any character
}
})
.then(response => {
// Handle successful response
console.log('Contacts retrieved successfully:', response.data);
})
.catch(error => {
// Handle errors
console.error('Error fetching contacts:', error.response ? error.response.data : error.message);
});
Replace Your_API_Key
with the API key you generated from your FreshDesk account. The password field can be any character, as FreshDesk uses basic authentication with the API key as the username.
Running the JavaScript Code and Verifying Results
To execute the code, run the following command in your terminal:
node getContacts.js
If successful, the console will display the list of contacts retrieved from your FreshDesk account. You can verify the results by checking the contact data in your FreshDesk dashboard.
Handling Errors and Troubleshooting FreshDesk API Calls
When making API calls, it's crucial to handle potential errors gracefully. The code above includes a catch
block to log any errors that occur during the request. Common issues might include incorrect API keys, network problems, or rate limiting.
For more detailed error information, refer to the FreshDesk API documentation.
Conclusion and Best Practices for FreshDesk API Integration
Integrating with the FreshDesk API using JavaScript allows developers to efficiently manage customer data and enhance their customer support systems. By following the steps outlined in this guide, you can retrieve contact information and seamlessly integrate FreshDesk functionalities into your applications.
Best Practices for Storing FreshDesk API Credentials
- Store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Avoid hardcoding API keys directly in your source code to enhance security.
Handling FreshDesk API Rate Limiting
FreshDesk imposes rate limits on API requests to ensure fair usage. Monitor the response headers for rate limit information and implement retry logic with exponential backoff to handle rate limit errors gracefully.
Data Transformation and Standardization
When integrating FreshDesk data with other systems, ensure that data fields are transformed and standardized to match the target system's requirements. This helps maintain data consistency across platforms.
Enhance Your Integration Strategy with Endgrate
For developers looking to streamline multiple integrations, Endgrate offers a unified API solution that simplifies the process. By using Endgrate, you can save time and resources, focusing on your core product while ensuring a seamless integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover how you can build once for each use case instead of multiple times for different integrations.
Read More
Ready to get started?