Using the Sugar Market API to Get Opportunities (with Javascript examples)
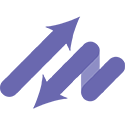
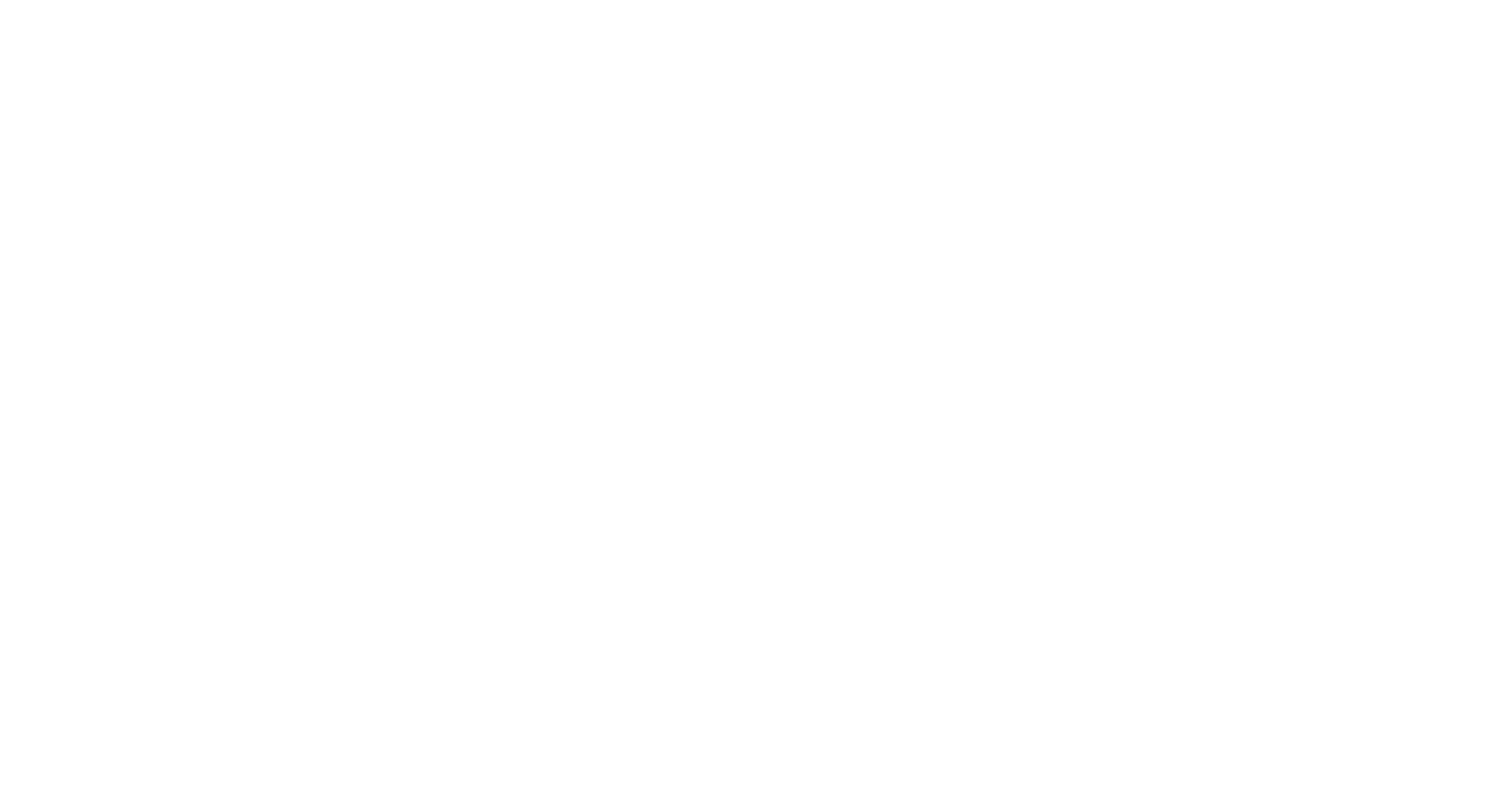
Introduction to Sugar Market API
Sugar Market is a robust marketing automation platform designed to enhance the marketing efforts of businesses by providing tools for lead generation, email marketing, and analytics. It offers a comprehensive suite of features that help businesses streamline their marketing processes and improve customer engagement.
Developers may want to integrate with the Sugar Market API to access and manage marketing data, such as opportunities, to optimize sales strategies and enhance customer relationship management. For example, a developer could use the Sugar Market API to retrieve opportunity data and analyze it to identify potential sales leads, thereby improving the sales pipeline.
Setting Up Your Sugar Market Test/Sandbox Account
Before you can start interacting with the Sugar Market API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with the API without affecting live data.
Creating a Sugar Market Account
If you don't already have a Sugar Market account, you can sign up for a free trial or demo account on the Sugar Market website. This will give you access to the necessary tools and features to begin your integration.
- Visit the Sugar Market website and navigate to the sign-up page.
- Follow the instructions to create your account, providing the required information.
- Once your account is created, log in to access the dashboard.
Generating API Credentials for Sugar Market
To interact with the Sugar Market API, you'll need to generate API credentials. These credentials will allow you to authenticate your requests.
- Log in to your Sugar Market account and navigate to the API settings section.
- Follow the prompts to create a new API application.
- Once created, you'll receive a client ID and client secret. Keep these credentials secure as they are essential for API authentication.
Understanding Sugar Market's Custom Authentication
Sugar Market uses a custom authentication method for API access. Ensure you understand how to implement this authentication in your code. Refer to the Sugar Market API documentation for detailed instructions on setting up authentication.
With your test account and API credentials ready, you can now proceed to make API calls and explore the capabilities of the Sugar Market API.
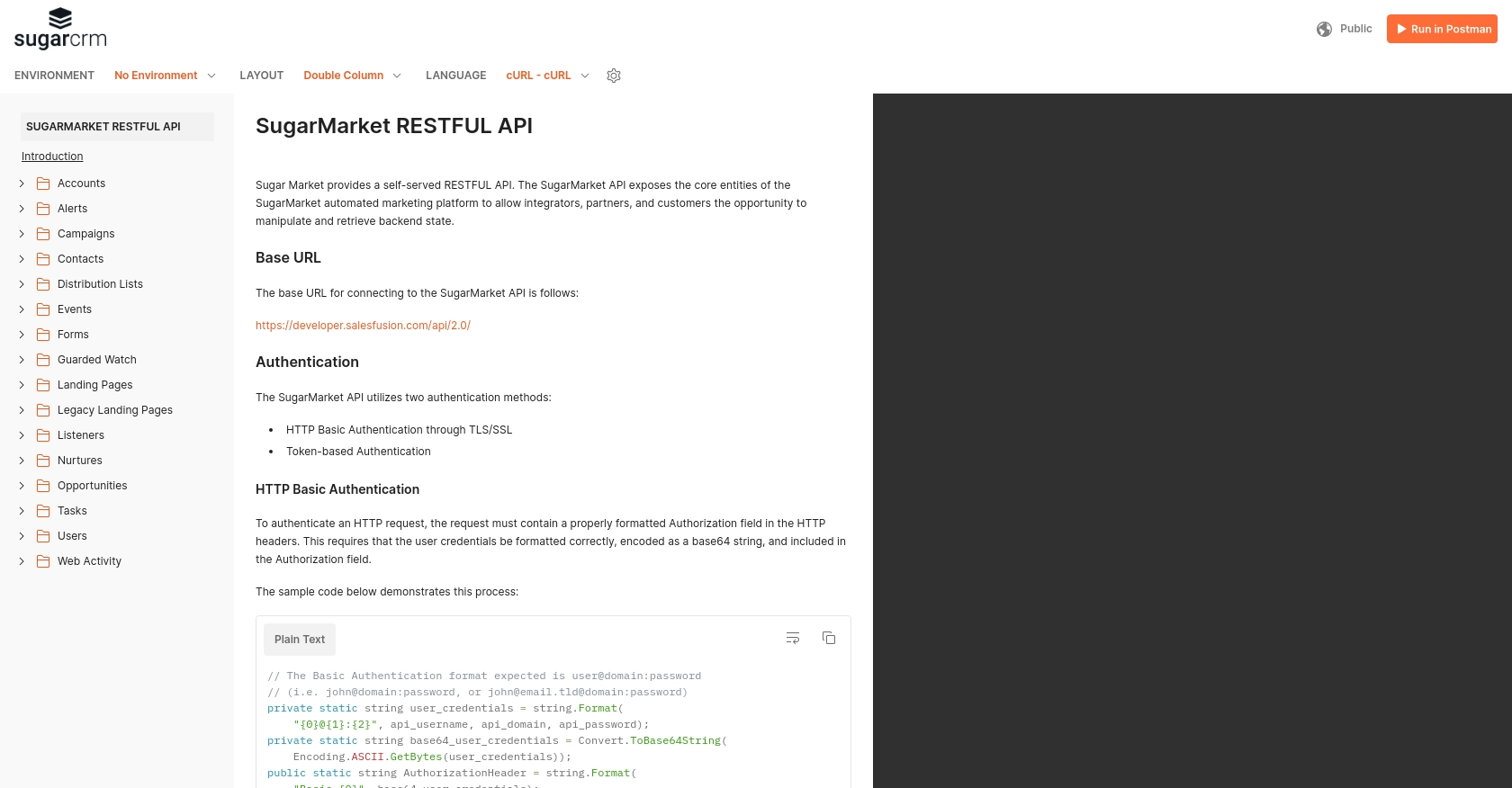
sbb-itb-96038d7
Making API Calls to Retrieve Opportunities with Sugar Market API Using JavaScript
To effectively interact with the Sugar Market API and retrieve opportunity data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses.
Setting Up Your JavaScript Environment for Sugar Market API
Before making API calls, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, which is essential for server-side scripting.
- Download and install Node.js from the official Node.js website.
- Verify the installation by running
node -v
andnpm -v
in your terminal to check the versions. - Initialize a new Node.js project by running
npm init -y
in your project directory. - Install the Axios library for making HTTP requests with
npm install axios
.
Writing JavaScript Code to Fetch Opportunities from Sugar Market API
With your environment set up, you can now write the JavaScript code to interact with the Sugar Market API. The following example demonstrates how to retrieve opportunities using Axios.
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://api.sugarmarket.com/opportunities';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_Access_Token'
};
// Function to fetch opportunities
async function getOpportunities() {
try {
const response = await axios.get(endpoint, { headers });
const opportunities = response.data;
console.log('Opportunities:', opportunities);
} catch (error) {
console.error('Error fetching opportunities:', error.response ? error.response.data : error.message);
}
}
// Call the function
getOpportunities();
Replace Your_Access_Token
with the token obtained from your Sugar Market API credentials. This code sets up the API endpoint and headers, makes a GET request to retrieve opportunities, and logs the results to the console.
Verifying API Call Success and Handling Errors
After running the script, you should see the opportunity data printed in your console. To verify the success of your API call, check the response status code and data structure.
- If the request is successful, the status code should be
200
, and the response should contain the opportunity data. - If an error occurs, the catch block will log the error message. Common issues include authentication errors or incorrect endpoint URLs.
For more detailed error handling, refer to the Sugar Market API documentation to understand the specific error codes and their meanings.
Conclusion and Best Practices for Using Sugar Market API with JavaScript
Integrating with the Sugar Market API using JavaScript can significantly enhance your ability to manage and analyze marketing opportunities. By following the steps outlined in this article, you can efficiently retrieve and utilize opportunity data to optimize your sales strategies.
Best Practices for Secure and Efficient API Integration with Sugar Market
- Securely Store API Credentials: Always keep your API credentials, such as client ID and client secret, secure. Consider using environment variables or secure vaults to store sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to manage requests efficiently.
- Standardize Data Fields: Ensure that data retrieved from the API is transformed and standardized to fit your application's requirements, facilitating seamless integration.
- Error Handling: Implement robust error handling to manage potential issues such as network errors or invalid responses. Refer to the Sugar Market API documentation for detailed error code explanations.
Enhance Your Integration Experience with Endgrate
While integrating with the Sugar Market API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Sugar Market.
With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, streamlining your development process.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?