Using the Zoho Books API to Get Tax Rates (with Javascript examples)
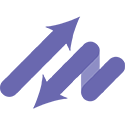
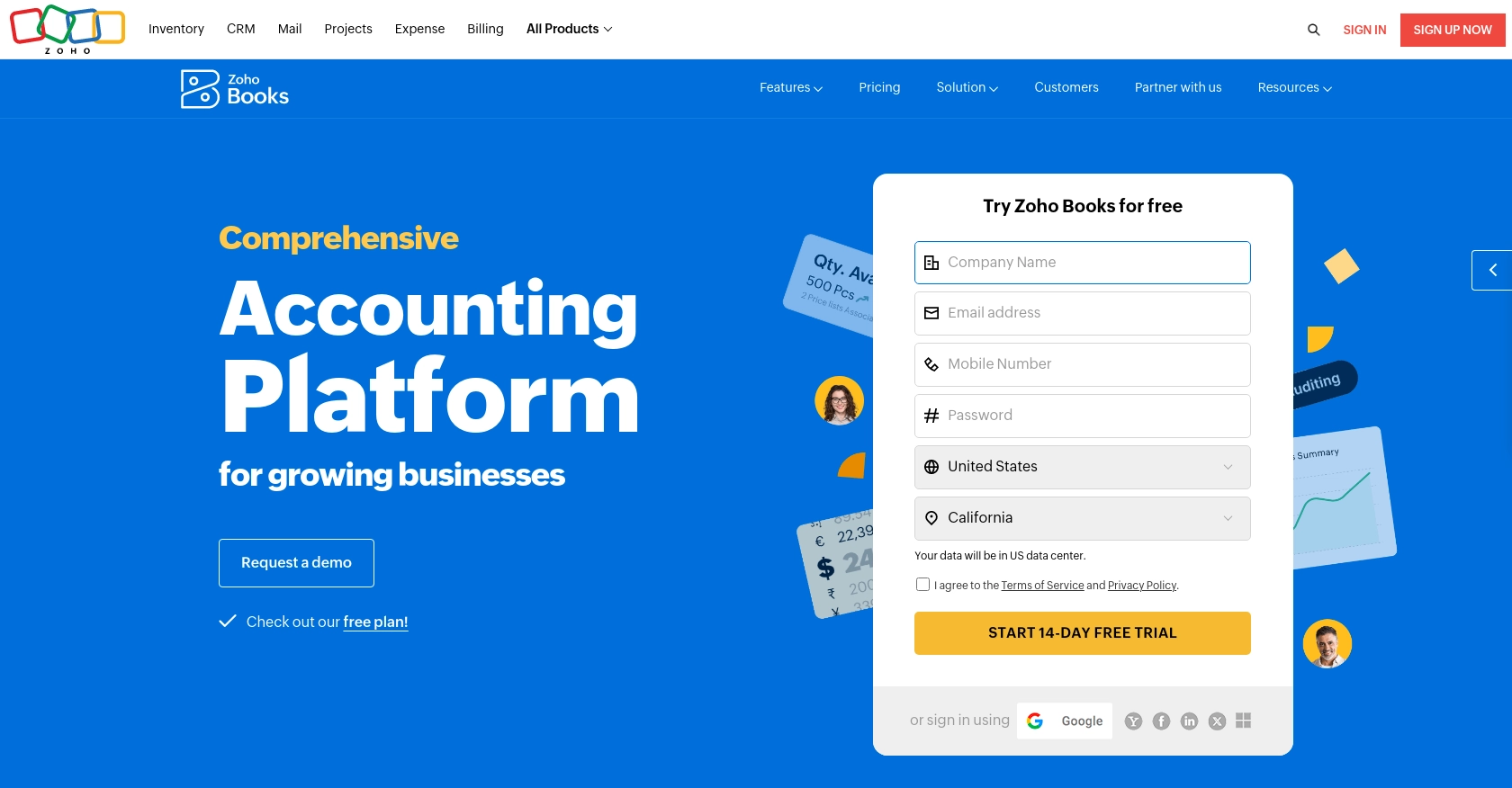
Introduction to Zoho Books API
Zoho Books is a comprehensive online accounting software designed to manage your finances, automate business workflows, and help you work collectively across departments. It offers a range of features including invoicing, expense tracking, and tax management, making it a preferred choice for businesses of all sizes.
Integrating with the Zoho Books API allows developers to streamline financial operations by automating tasks such as retrieving tax rates. For example, you can use the API to fetch tax rates directly into your application, ensuring accurate and up-to-date financial calculations without manual intervention.
This article will guide you through using JavaScript to interact with the Zoho Books API to retrieve tax rates, providing a step-by-step approach to setting up and executing API calls efficiently.
Setting Up Your Zoho Books Test Account for API Integration
Before diving into the Zoho Books API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting real data. Zoho Books offers a free trial that you can use to explore its features and test integrations.
Creating a Zoho Books Account
- Visit the Zoho Books website and sign up for a free trial account.
- Follow the on-screen instructions to complete the registration process.
- Once registered, log in to your Zoho Books account to access the dashboard.
Setting Up OAuth for Zoho Books API
Zoho Books uses OAuth 2.0 for authentication, ensuring secure access to your data. Follow these steps to set up OAuth:
- Navigate to the Zoho Developer Console.
- Click on Add Client ID to register your application.
- Fill in the required details, including the redirect URI, and submit the form.
- Upon successful registration, you'll receive a Client ID and Client Secret. Keep these credentials secure.
Generating OAuth Tokens
To interact with the Zoho Books API, you'll need to generate access and refresh tokens:
- Redirect users to the following URL to obtain an authorization code:
- After user consent, Zoho will redirect to your specified URI with a code parameter.
- Exchange this code for access and refresh tokens by making a POST request:
- Store the access and refresh tokens securely for future API calls.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.settings.READ&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI&access_type=offline
fetch('https://accounts.zoho.com/oauth/v2/token', {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
},
body: new URLSearchParams({
code: 'YOUR_AUTHORIZATION_CODE',
client_id: 'YOUR_CLIENT_ID',
client_secret: 'YOUR_CLIENT_SECRET',
redirect_uri: 'YOUR_REDIRECT_URI',
grant_type: 'authorization_code'
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
With your Zoho Books account and OAuth setup complete, you're ready to start making API calls to retrieve tax rates and more.
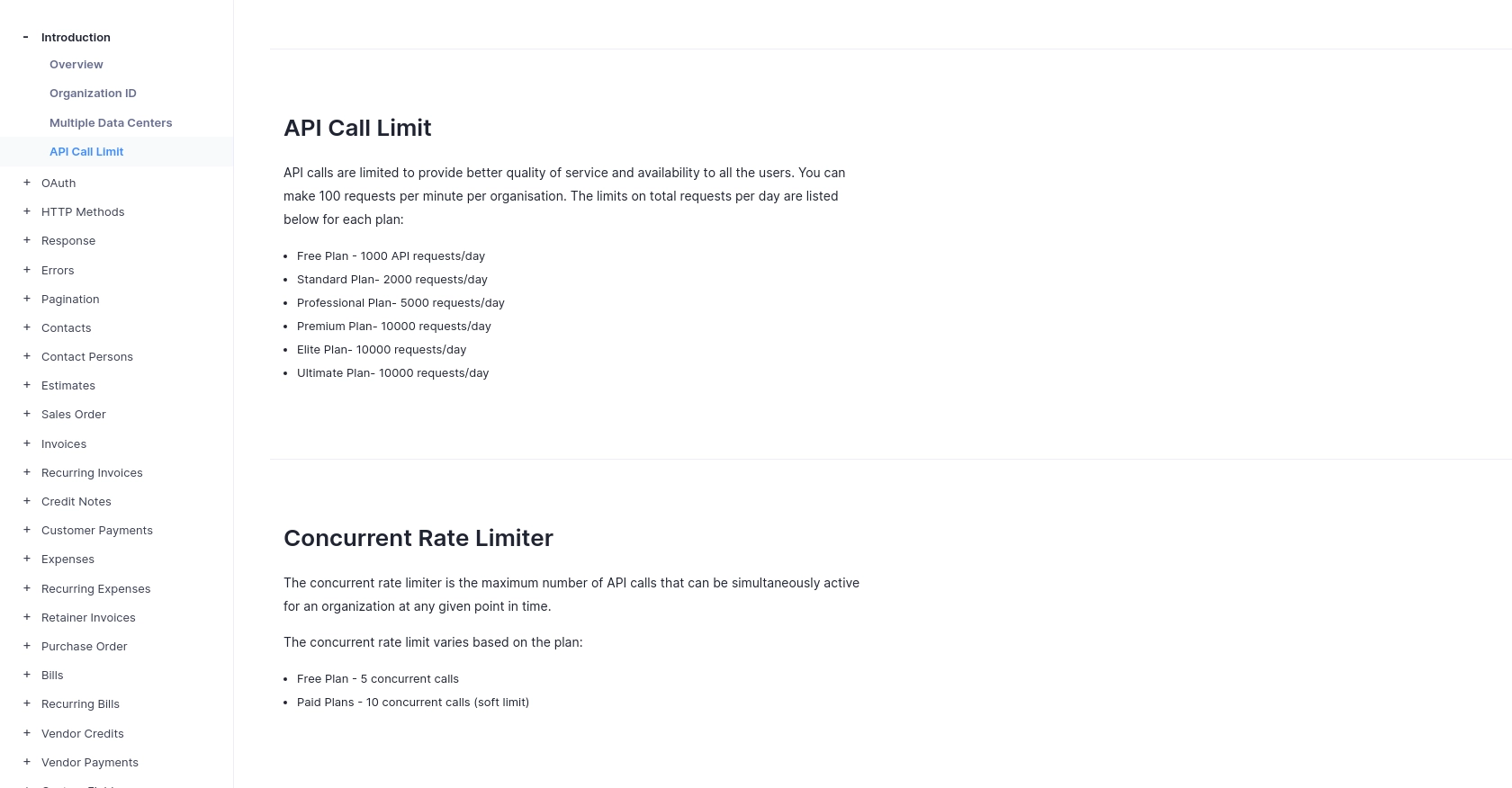
sbb-itb-96038d7
Making API Calls to Retrieve Tax Rates from Zoho Books Using JavaScript
With your Zoho Books account and OAuth setup complete, you can now proceed to make API calls to retrieve tax rates. This section will guide you through the process of using JavaScript to interact with the Zoho Books API effectively.
JavaScript Prerequisites for Zoho Books API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A text editor or IDE for writing JavaScript code.
- Access and refresh tokens obtained from the OAuth setup.
Installing Required JavaScript Libraries
To make HTTP requests, you can use the node-fetch
library. Install it using the following command:
npm install node-fetch
Fetching Tax Rates from Zoho Books API
Follow these steps to fetch tax rates using JavaScript:
- Create a new JavaScript file, for example,
getTaxRates.js
. - Add the following code to make a GET request to the Zoho Books API:
- Replace
YOUR_ORG_ID
andYOUR_ACCESS_TOKEN
with your actual organization ID and access token. - Run the script using the following command:
const fetch = require('node-fetch');
// Define the API endpoint and headers
const endpoint = 'https://www.zohoapis.com/books/v3/settings/taxes?organization_id=YOUR_ORG_ID';
const headers = {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN'
};
// Function to fetch tax rates
async function getTaxRates() {
try {
const response = await fetch(endpoint, { headers });
const data = await response.json();
if (response.ok) {
console.log('Tax Rates:', data.taxes);
} else {
console.error('Error:', data.message);
}
} catch (error) {
console.error('Fetch Error:', error);
}
}
// Call the function
getTaxRates();
node getTaxRates.js
Handling API Responses and Errors
Upon executing the script, you should see the list of tax rates printed to the console. If the request fails, the error message will be displayed. Common HTTP status codes to watch for include:
- 200 OK: The request was successful.
- 401 Unauthorized: Invalid access token.
- 429 Rate Limit Exceeded: Too many requests in a short period.
For more details on error codes, refer to the Zoho Books API documentation.
With this setup, you can efficiently retrieve tax rates from Zoho Books using JavaScript, enabling seamless integration into your applications.
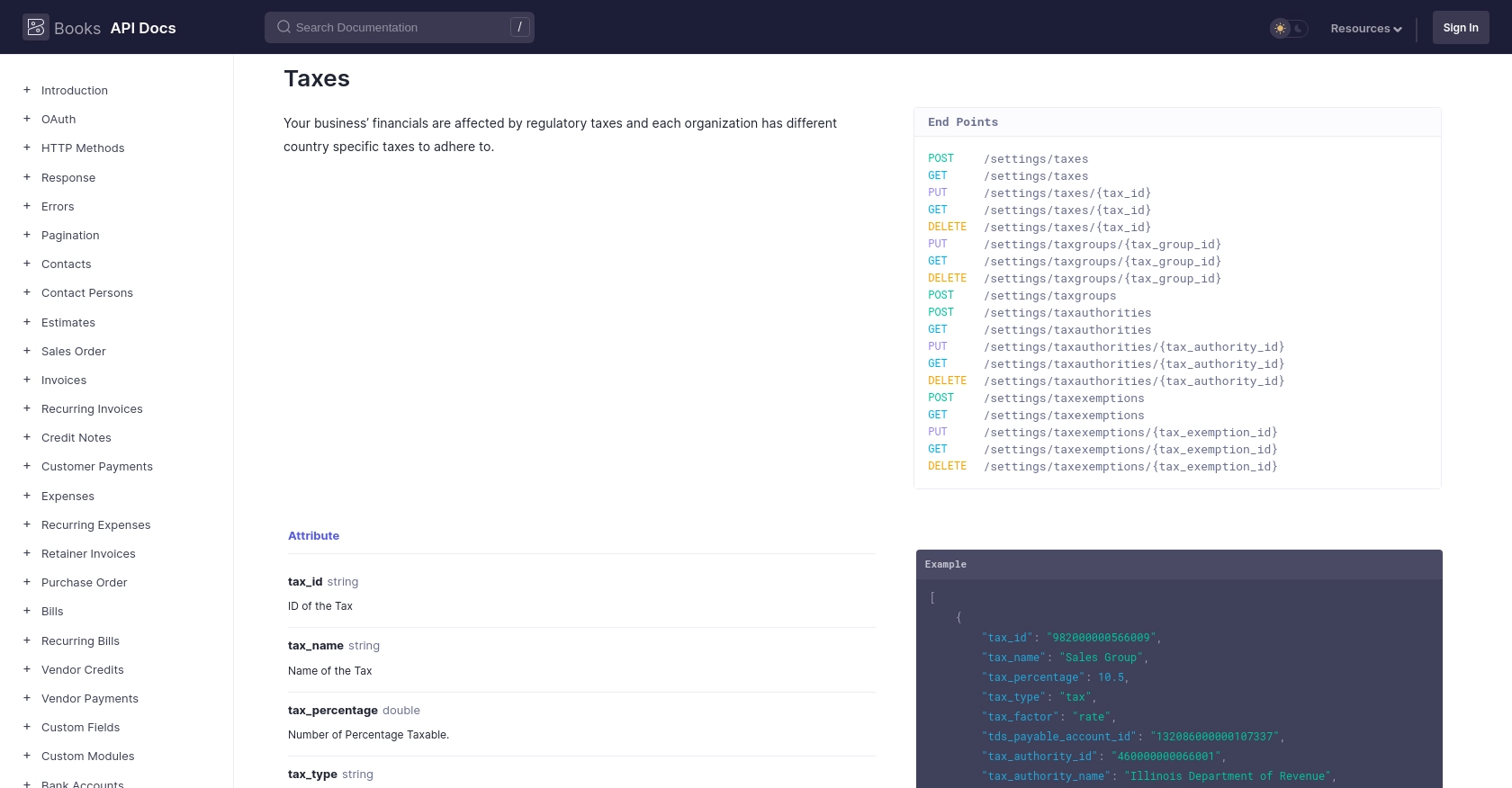
Conclusion: Best Practices for Zoho Books API Integration and Next Steps
Integrating with the Zoho Books API to retrieve tax rates using JavaScript can significantly streamline your financial operations. By automating the retrieval of tax rates, you ensure that your application remains accurate and up-to-date, reducing the risk of manual errors.
Best Practices for Secure and Efficient Zoho Books API Usage
- Secure Storage of Credentials: Always store your OAuth credentials, such as the client ID, client secret, access token, and refresh token, securely. Consider using environment variables or a secure vault.
- Handle Rate Limiting: Zoho Books imposes a rate limit of 100 requests per minute per organization. Implement logic to handle rate limit errors (HTTP 429) by retrying requests after a delay. For more details, refer to the API call limit documentation.
- Data Transformation: Standardize and transform the retrieved data as needed to fit your application's requirements, ensuring consistency across different integrations.
- Error Handling: Implement robust error handling to manage various HTTP status codes. Refer to the Zoho Books error documentation for guidance.
Enhance Your Integration Strategy with Endgrate
While integrating with Zoho Books API can be straightforward, managing multiple integrations across different platforms can be complex and time-consuming. This is where Endgrate can be a game-changer for your business.
Endgrate provides a unified API endpoint that connects to multiple platforms, including Zoho Books, allowing you to build once and deploy across various services. This not only saves time but also reduces the complexity of maintaining separate integrations.
By leveraging Endgrate, you can focus on your core product development while ensuring a seamless integration experience for your customers. Visit Endgrate to learn more about how you can optimize your integration strategy.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/taxes/#overview
Ready to get started?