How to Get Tax with the Avalara API in Python
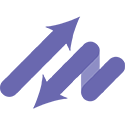
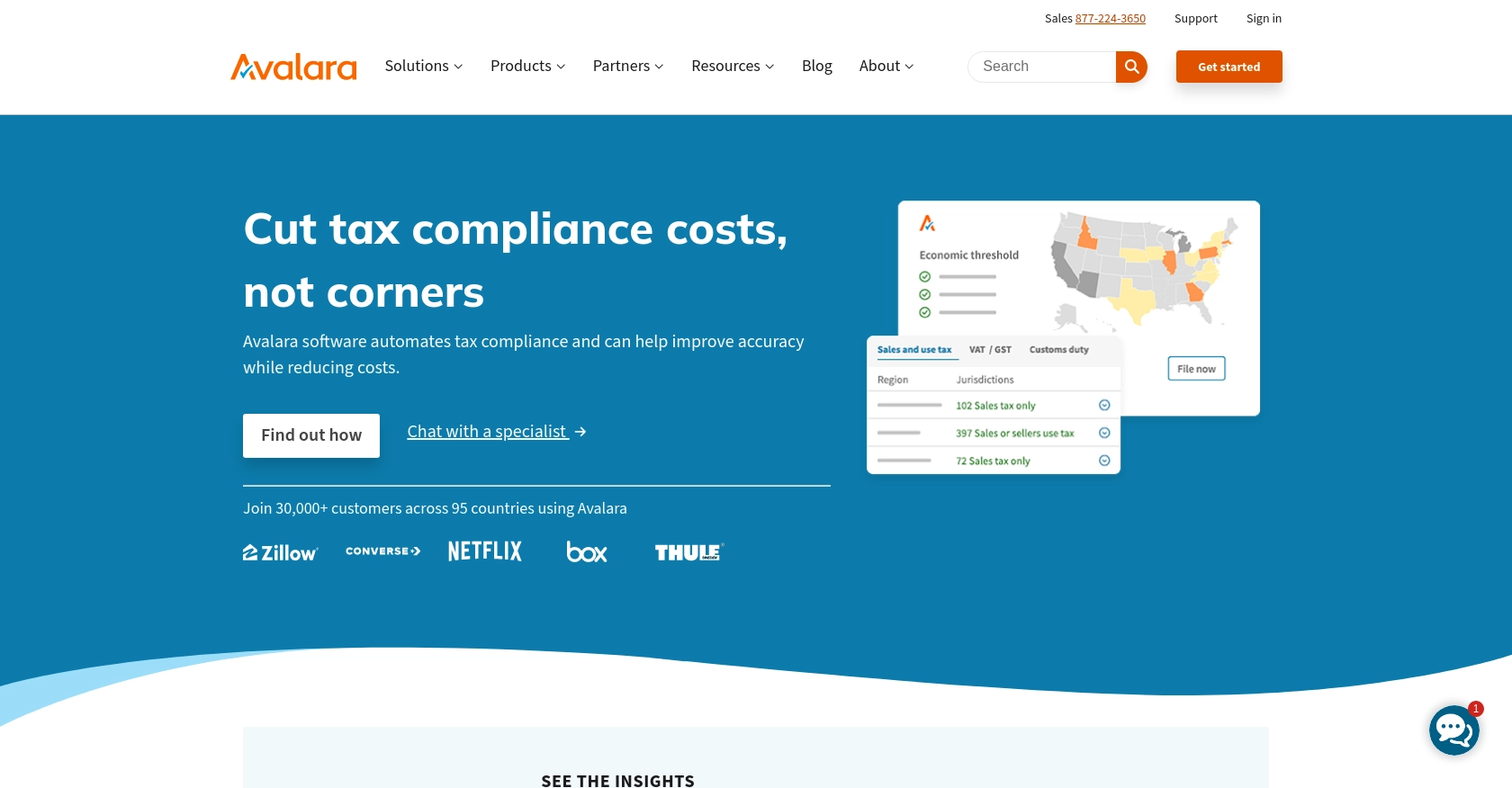
Introduction to Avalara and Tax Automation
Avalara is a leading provider of cloud-based tax compliance solutions, offering a comprehensive suite of tools to automate and streamline tax calculations for businesses of all sizes. With its robust API, Avalara enables developers to integrate tax calculation capabilities directly into their applications, ensuring accurate and efficient tax management.
Integrating with Avalara's API can significantly enhance a developer's ability to manage tax-related tasks. For example, a developer might use the Avalara API to automatically calculate sales tax for transactions in an e-commerce platform, ensuring compliance with various tax jurisdictions and reducing the risk of errors.
This article will guide you through the process of using Python to interact with the Avalara API, specifically focusing on retrieving tax information. By the end of this tutorial, you'll be equipped with the knowledge to seamlessly integrate Avalara's tax calculation features into your Python applications.
Setting Up Your Avalara Test Account for API Integration
Before you can start using the Avalara API to retrieve tax information, you'll need to set up a test account. This will allow you to experiment with the API without affecting any live data. Avalara provides a sandbox environment specifically for development and testing purposes.
Creating an Avalara Sandbox Account
To begin, you'll need to create a sandbox account on the Avalara website. Follow these steps to get started:
- Visit the Avalara Developer Portal and sign up for a sandbox account.
- Fill out the registration form with your details and submit it.
- Once registered, log in to your Avalara sandbox account.
Generating API Credentials for Avalara
After setting up your sandbox account, you'll need to generate API credentials to authenticate your requests. Avalara uses Basic HTTP Authentication, which requires a username and password. Here’s how to obtain these credentials:
- Navigate to the "Settings" section in your Avalara account dashboard.
- Select "Generate License Key" to create a new license key for your account.
- Note down your account ID and the newly generated license key. These will be used to authenticate your API requests.
Understanding Avalara API Authentication
To authenticate with the Avalara API, you'll need to construct an authorization header using your account ID and license key. Follow these steps:
- Combine your account ID and license key in the format:
accountid:licensekey
. - Encode this string using Base64 encoding.
- Include the encoded string in your API request headers as follows:
headers = {
"Authorization": "Basic "
}
For more details on authentication, refer to the Avalara Authentication Documentation.
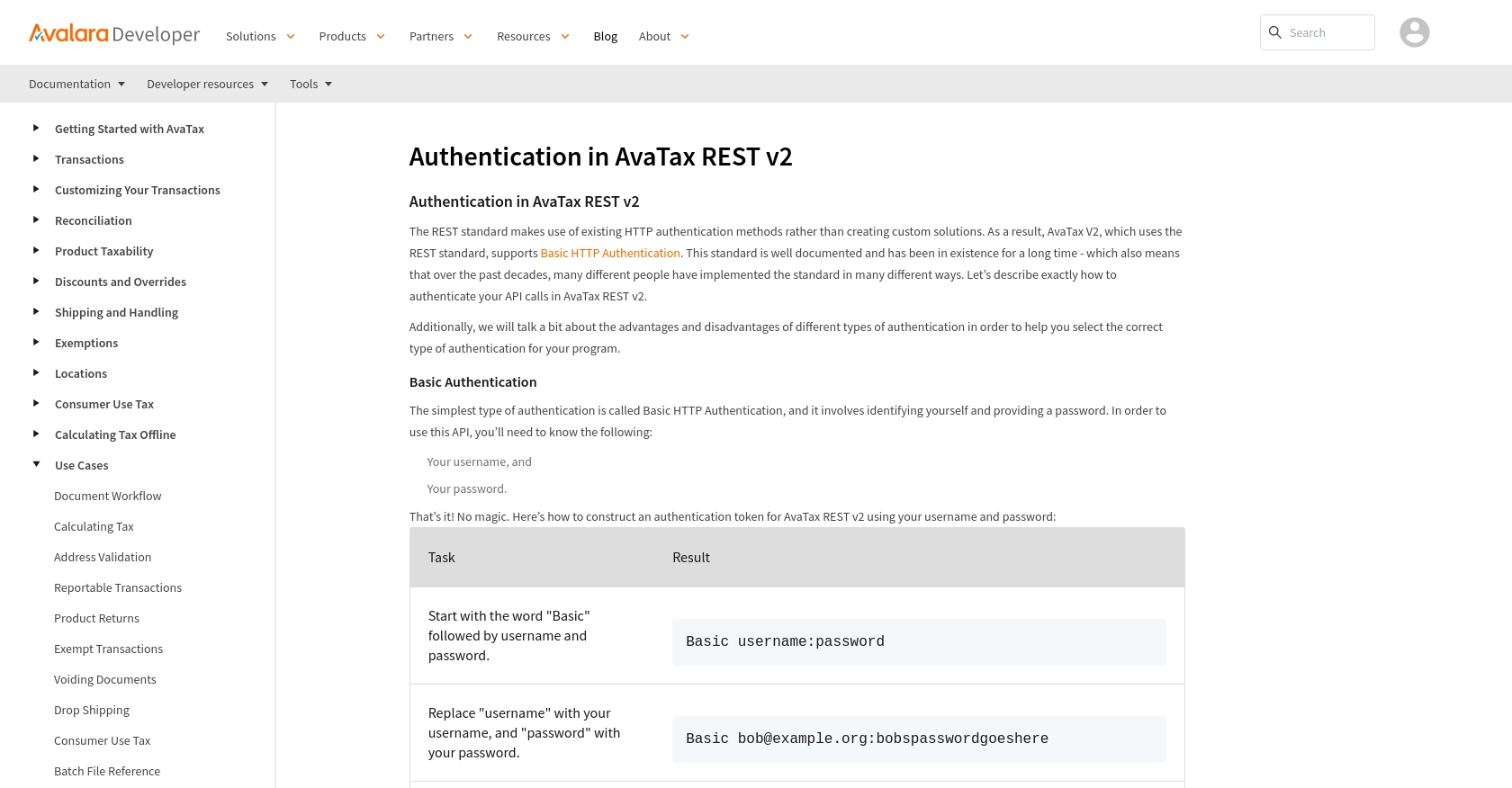
sbb-itb-96038d7
Making API Calls to Retrieve Tax Information with Avalara in Python
To effectively interact with the Avalara API and retrieve tax information, you'll need to use Python to make HTTP requests. This section will guide you through the necessary steps, including setting up your Python environment, writing the code to make API calls, and handling potential errors.
Setting Up Your Python Environment for Avalara API Integration
Before making API calls, ensure your Python environment is properly configured. You'll need Python 3.11.1 and the requests
library to handle HTTP requests. Install the library using the following command:
pip install requests
Writing Python Code to Call the Avalara API
Once your environment is set up, you can write a Python script to interact with the Avalara API. Create a file named get_tax_info.py
and add the following code:
import requests
import base64
# Set your Avalara account ID and license key
account_id = "Your_Account_ID"
license_key = "Your_License_Key"
# Encode the credentials
credentials = f"{account_id}:{license_key}"
encoded_credentials = base64.b64encode(credentials.encode()).decode()
# Set the API endpoint and headers
url = "https://sandbox-rest.avatax.com/api/v2/tax/get"
headers = {
"Authorization": f"Basic {encoded_credentials}",
"Content-Type": "application/json"
}
# Define the request payload
payload = {
"lines": [
{
"number": "1",
"quantity": 1,
"amount": 100,
"taxCode": "P0000000"
}
],
"type": "SalesInvoice",
"companyCode": "DEFAULT",
"date": "2023-10-01",
"customerCode": "ABC123",
"addresses": {
"shipFrom": {
"line1": "123 Main Street",
"city": "Seattle",
"region": "WA",
"country": "US",
"postalCode": "98101"
},
"shipTo": {
"line1": "456 Elm Street",
"city": "Bellevue",
"region": "WA",
"country": "US",
"postalCode": "98004"
}
}
}
# Make the API request
response = requests.post(url, json=payload, headers=headers)
# Check if the request was successful
if response.status_code == 200:
tax_data = response.json()
print("Tax Information Retrieved Successfully:")
print(tax_data)
else:
print(f"Failed to retrieve tax information. Status Code: {response.status_code}")
print(response.json())
Replace Your_Account_ID
and Your_License_Key
with your actual Avalara account ID and license key.
Verifying API Call Success and Handling Errors
After running the script, you should see the tax information printed in the console if the request is successful. If there's an error, the script will print the status code and error message. Common error codes include:
- 401 Unauthorized: Check your credentials and ensure they are correctly encoded.
- 400 Bad Request: Verify the request payload for any missing or incorrect fields.
For more detailed error information, refer to the Avalara Authentication Documentation.
Conclusion and Best Practices for Using Avalara API in Python
Integrating Avalara's API into your Python applications can greatly enhance your ability to manage tax calculations efficiently and accurately. By following the steps outlined in this guide, you can seamlessly retrieve tax information and ensure compliance with various tax jurisdictions.
Best Practices for Secure and Efficient Avalara API Integration
- Secure Storage of Credentials: Always store your Avalara account ID and license key securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handling Rate Limits: Be mindful of Avalara's API rate limits to avoid service disruptions. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data you send to Avalara is standardized and validated to prevent errors and ensure accurate tax calculations.
Leverage Endgrate for Streamlined Integration Management
While integrating with Avalara's API can be straightforward, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Avalara. By using Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate's website and discover the benefits of a streamlined integration experience.
Read More
Ready to get started?