Using the Zoho Books API to Create or Update Vendors (with PHP examples)
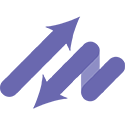
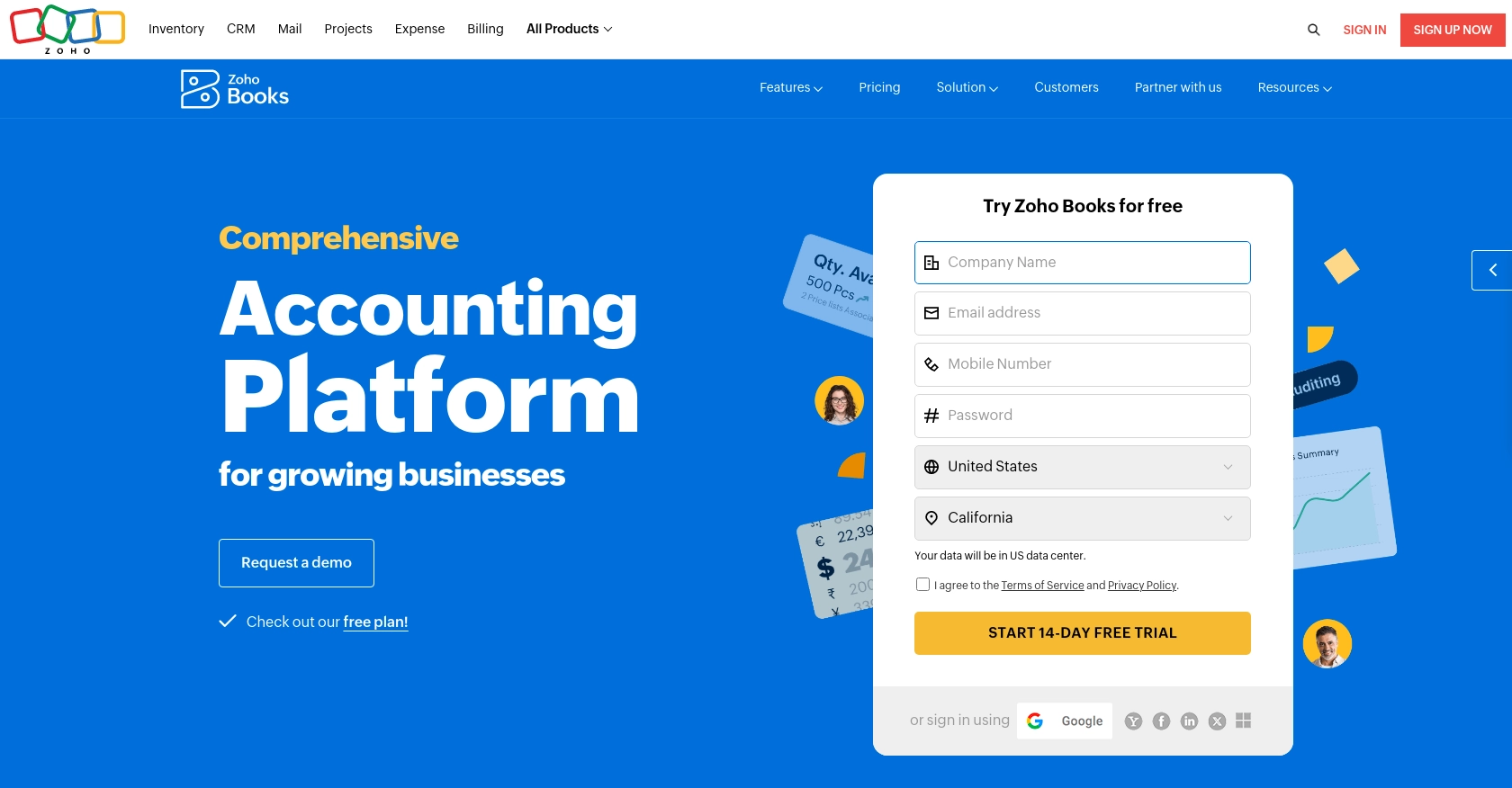
Introduction to Zoho Books API for Vendor Management
Zoho Books is a comprehensive cloud-based accounting software designed to streamline financial operations for businesses of all sizes. It offers a wide range of features, including invoicing, expense tracking, and inventory management, making it a popular choice for businesses seeking an efficient accounting solution.
For developers, integrating with the Zoho Books API can significantly enhance the automation of accounting tasks. One common use case is managing vendor information. By using the API, developers can create or update vendor records programmatically, ensuring that the vendor data is always up-to-date and accurate.
This article will guide you through using PHP to interact with the Zoho Books API, specifically focusing on creating or updating vendor information. This integration can help automate vendor management processes, saving time and reducing errors in data entry.
Setting Up Your Zoho Books Test/Sandbox Account for API Integration
Before you can start integrating with the Zoho Books API, you need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting your live data. Follow these steps to get started:
Create a Zoho Books Account
- Visit the Zoho Books sign-up page and register for a free trial account.
- Complete the registration process by providing the necessary details such as your name, email, and business information.
- Once registered, log in to your Zoho Books account to access the dashboard.
Register Your Application in Zoho Developer Console
To interact with the Zoho Books API, you need to register your application to obtain the necessary OAuth credentials:
- Go to the Zoho Developer Console.
- Click on Add Client ID to register a new application.
- Fill in the required details, such as the application name and redirect URI.
- Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure as they are essential for API authentication.
Generate OAuth Tokens for Zoho Books API Access
Zoho Books uses OAuth 2.0 for secure API access. Follow these steps to generate the necessary tokens:
- Redirect users to the following authorization URL to obtain a grant token:
- After the user authorizes the application, Zoho will redirect to your specified URI with a code parameter.
- Exchange this code for an access token by making a POST request to:
- The response will include both an access_token and a refresh_token. Use the access token for API calls, and the refresh token to obtain new access tokens when needed.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.fullaccess.all&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI&access_type=offline
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
With your Zoho Books account set up and OAuth tokens generated, you're ready to start integrating with the API to manage vendor information programmatically.
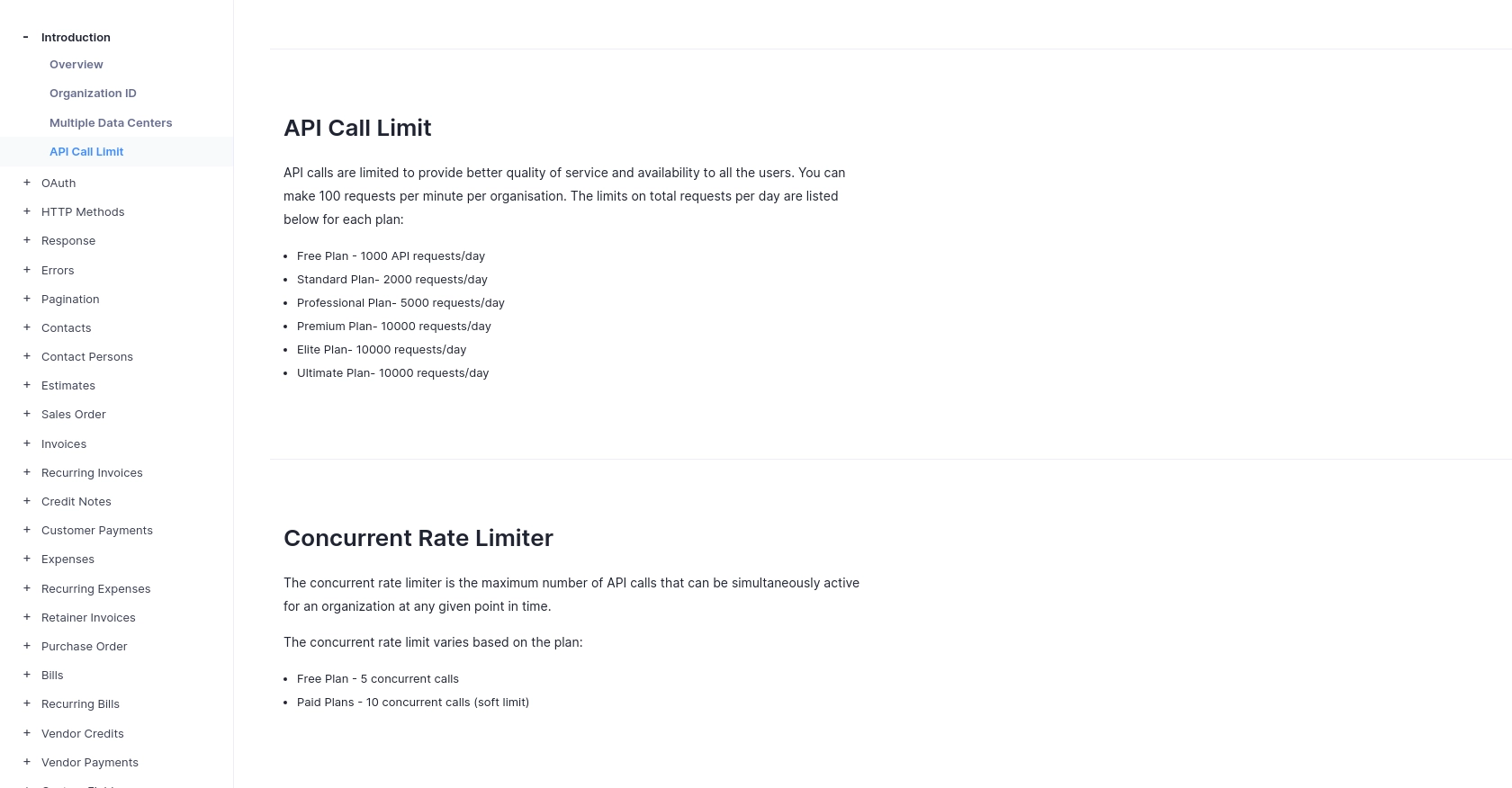
sbb-itb-96038d7
Making API Calls to Zoho Books for Vendor Management Using PHP
To interact with the Zoho Books API for creating or updating vendor information, you need to make HTTP requests using PHP. This section will guide you through the process of setting up your PHP environment and executing API calls to manage vendor data efficiently.
Setting Up Your PHP Environment
Before making API calls, ensure your PHP environment is ready:
- Install PHP 7.4 or later.
- Ensure the
cURL
extension is enabled in yourphp.ini
file. - Install Composer to manage dependencies if needed.
Creating a Vendor in Zoho Books Using PHP
To create a vendor, you need to send a POST request to the Zoho Books API endpoint. Below is a sample PHP script to create a vendor:
<?php
$accessToken = 'YOUR_ACCESS_TOKEN';
$organizationId = 'YOUR_ORGANIZATION_ID';
$url = "https://www.zohoapis.com/books/v3/contacts?organization_id=$organizationId";
$vendorData = [
'contact_name' => 'Vendor Name',
'company_name' => 'Vendor Company',
'contact_type' => 'vendor',
'billing_address' => [
'attention' => 'Billing Attention',
'address' => '123 Vendor St',
'city' => 'Vendor City',
'state' => 'Vendor State',
'zip' => '12345',
'country' => 'Vendor Country'
]
];
$options = [
CURLOPT_URL => $url,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_HTTPHEADER => [
"Authorization: Zoho-oauthtoken $accessToken",
'Content-Type: application/json'
],
CURLOPT_POST => true,
CURLOPT_POSTFIELDS => json_encode($vendorData)
];
$ch = curl_init();
curl_setopt_array($ch, $options);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
Replace YOUR_ACCESS_TOKEN
and YOUR_ORGANIZATION_ID
with your actual access token and organization ID. This script sends a POST request with the vendor details to Zoho Books, creating a new vendor record.
Updating a Vendor in Zoho Books Using PHP
To update an existing vendor, use a PUT request. Here's how you can update vendor information:
<?php
$accessToken = 'YOUR_ACCESS_TOKEN';
$organizationId = 'YOUR_ORGANIZATION_ID';
$vendorId = 'VENDOR_ID';
$url = "https://www.zohoapis.com/books/v3/contacts/$vendorId?organization_id=$organizationId";
$updatedVendorData = [
'contact_name' => 'Updated Vendor Name',
'billing_address' => [
'attention' => 'Updated Attention',
'address' => '456 Updated St',
'city' => 'Updated City',
'state' => 'Updated State',
'zip' => '67890',
'country' => 'Updated Country'
]
];
$options = [
CURLOPT_URL => $url,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_HTTPHEADER => [
"Authorization: Zoho-oauthtoken $accessToken",
'Content-Type: application/json'
],
CURLOPT_CUSTOMREQUEST => 'PUT',
CURLOPT_POSTFIELDS => json_encode($updatedVendorData)
];
$ch = curl_init();
curl_setopt_array($ch, $options);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
Replace VENDOR_ID
with the ID of the vendor you wish to update. This script modifies the vendor's details using a PUT request.
Handling API Responses and Errors
After making API calls, it's crucial to handle responses and potential errors. Zoho Books API uses HTTP status codes to indicate success or failure:
- 200 OK: The request was successful.
- 201 Created: A new resource was successfully created.
- 400 Bad Request: The request was invalid.
- 401 Unauthorized: Authentication failed.
- 404 Not Found: The requested resource was not found.
- 429 Rate Limit Exceeded: Too many requests.
- 500 Internal Server Error: A server error occurred.
Check the response code and message to ensure the API call succeeded. For more details on error codes, refer to the Zoho Books API Error Documentation.
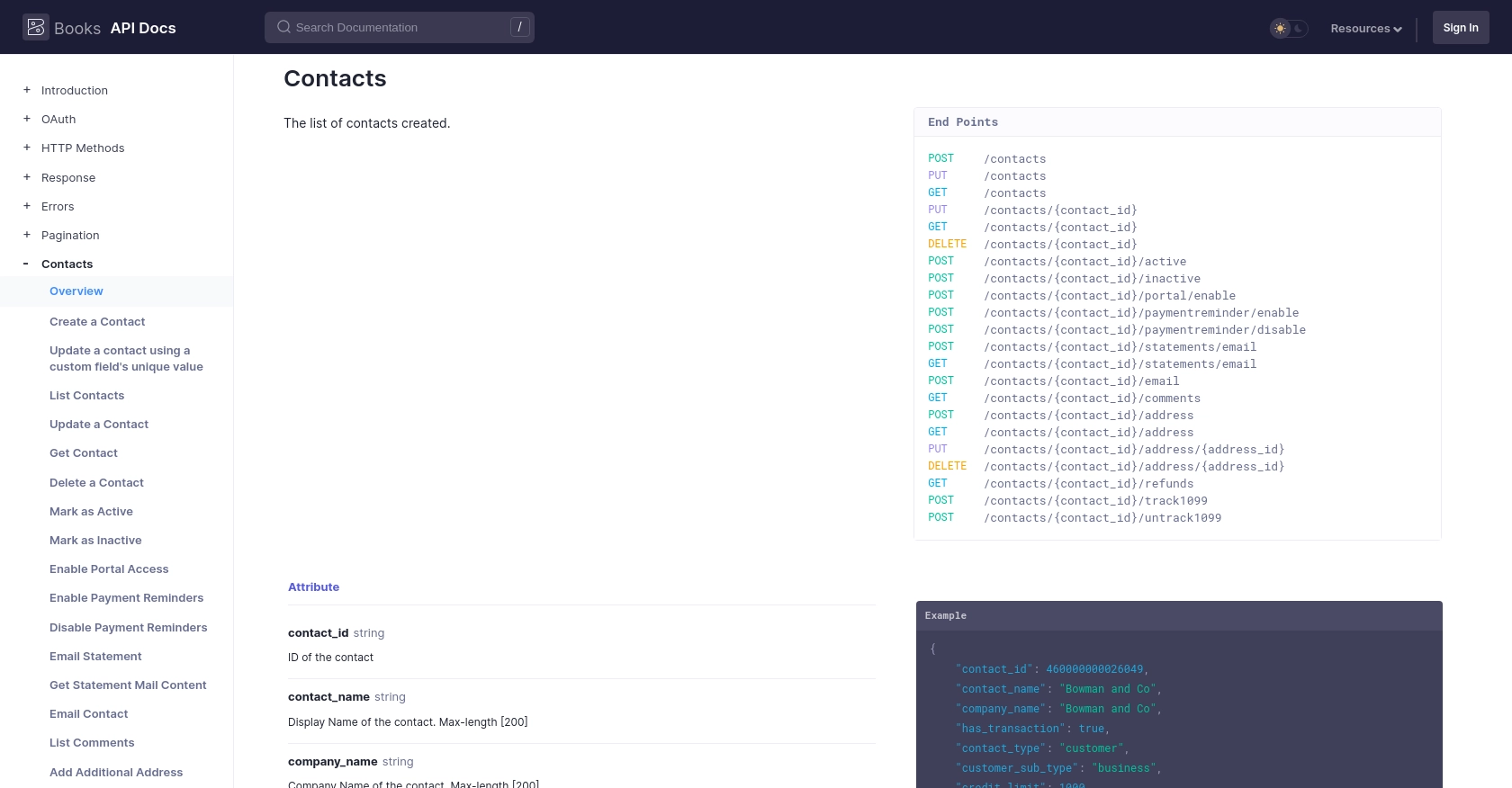
Best Practices for Zoho Books API Integration
When integrating with the Zoho Books API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth credentials, such as the client ID, client secret, and tokens, securely. Avoid hardcoding them in your codebase and consider using environment variables or secure vaults.
- Handle Rate Limiting: Zoho Books API has rate limits to ensure fair usage. The limit is 100 requests per minute per organization. Implement logic to handle rate limit errors (HTTP 429) by retrying requests after a delay. For more details, refer to the Zoho Books API Call Limit Documentation.
- Standardize Data Fields: Ensure that data fields are consistent and standardized across your application to prevent data mismatches and errors.
- Monitor API Usage: Regularly monitor your API usage to ensure you stay within the limits and optimize your integration for performance.
Streamlining Vendor Management with Endgrate
Integrating with multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoho Books. With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the integrations.
- Build Once, Use Everywhere: Develop a single integration for each use case and apply it across different platforms.
- Enhance Customer Experience: Provide an intuitive integration experience for your users.
Explore how Endgrate can streamline your integration processes by visiting Endgrate.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/contacts/#overview
Ready to get started?