Using the Sugar Market API to Get Opportunities (with PHP examples)
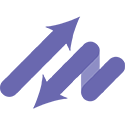
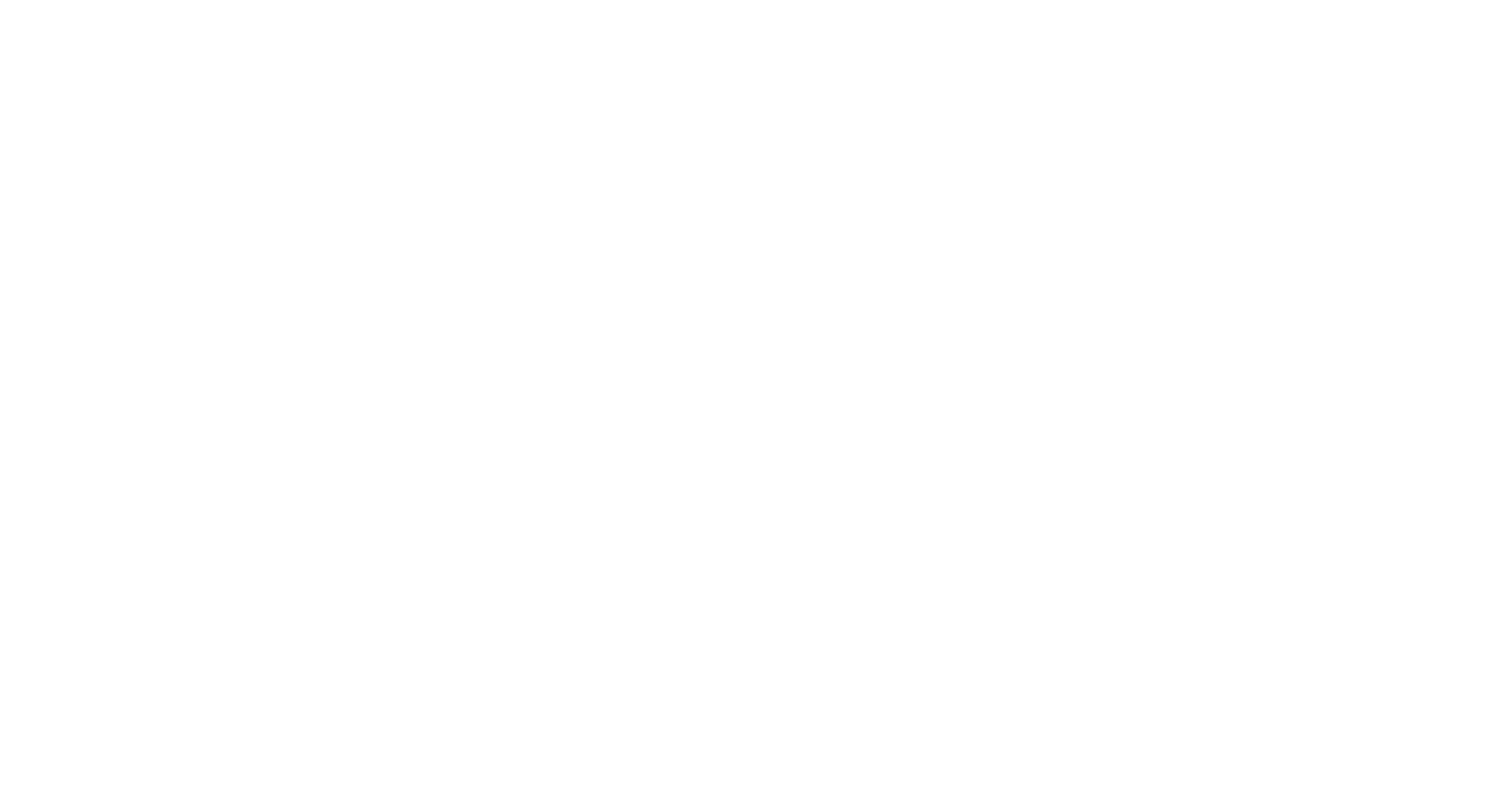
Introduction to Sugar Market
Sugar Market is a comprehensive marketing automation platform designed to enhance the marketing efforts of B2B businesses. It offers a suite of tools that streamline campaign management, lead nurturing, and analytics, allowing marketers to effectively engage with their audience and drive conversions.
Integrating with the Sugar Market API enables developers to access and manage marketing data programmatically. For example, you might want to retrieve opportunities data to analyze sales prospects and tailor marketing strategies accordingly. This integration can help automate data retrieval processes, ensuring that your marketing team has up-to-date information to make informed decisions.
Setting Up Your Sugar Market Test/Sandbox Account
Before you can start interacting with the Sugar Market API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a Sugar Market Account
If you don't already have a Sugar Market account, you can sign up for a free trial or request access to a sandbox environment through the Sugar Market website. This will provide you with the necessary credentials to access the API.
Generating API Credentials for Sugar Market
Once you have access to your Sugar Market account, follow these steps to generate the API credentials:
- Log in to your Sugar Market account.
- Navigate to the API settings section, which is typically found under the integrations or developer tools menu.
- Create a new API application. You will need to provide a name and description for your application.
- After creating the application, you will receive a client ID and client secret. Make sure to store these securely, as they will be used for authentication.
Authenticating with Sugar Market API
Sugar Market uses a custom authentication method. To authenticate your API requests, you'll need to include the client ID and client secret in your request headers. Refer to the Sugar Market API documentation for detailed instructions on how to authenticate your requests.
With your test account and API credentials set up, you're ready to start making API calls to retrieve opportunities data from Sugar Market.
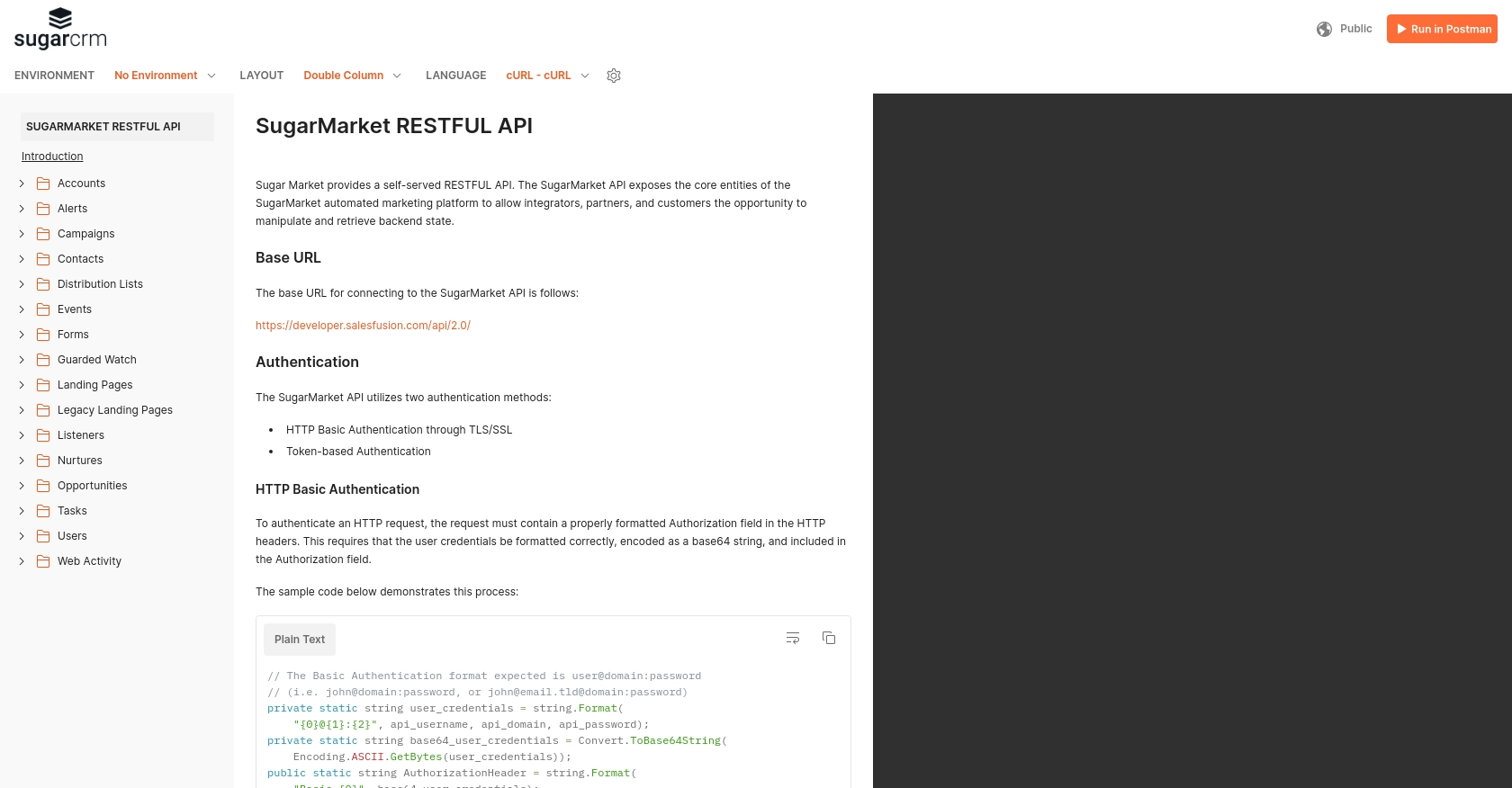
sbb-itb-96038d7
Making API Calls to Retrieve Opportunities from Sugar Market Using PHP
To interact with the Sugar Market API and retrieve opportunities data, you will need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, writing the code to make the API call, and handling the response.
Setting Up Your PHP Environment
Before you begin, ensure that you have PHP installed on your machine. You can download it from the official PHP website. Additionally, you'll need the cURL
extension enabled in your PHP configuration to make HTTP requests.
To verify that cURL
is enabled, create a PHP file with the following content and run it:
<?php
phpinfo();
?>
Look for the cURL
section in the output. If it's not enabled, you may need to uncomment the extension=curl
line in your php.ini
file and restart your web server.
Writing the PHP Code to Retrieve Opportunities
Once your environment is set up, you can write the PHP script to make the API call to Sugar Market. Create a new PHP file named get_opportunities.php
and add the following code:
<?php
// Set the API endpoint and credentials
$endpoint = "https://api.sugarmarket.com/opportunities";
$clientId = "Your_Client_ID";
$clientSecret = "Your_Client_Secret";
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Basic " . base64_encode("$clientId:$clientSecret"),
"Content-Type: application/json"
]);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo "cURL error: " . curl_error($ch);
} else {
// Parse and display the response
$data = json_decode($response, true);
foreach ($data['opportunities'] as $opportunity) {
echo "Opportunity Name: " . $opportunity['name'] . "\n";
echo "Status: " . $opportunity['status'] . "\n\n";
}
}
// Close the cURL session
curl_close($ch);
?>
Replace Your_Client_ID
and Your_Client_Secret
with the credentials you obtained from your Sugar Market account.
Running the PHP Script and Handling the Response
To execute the script, run the following command in your terminal:
php get_opportunities.php
If the request is successful, you should see a list of opportunities with their names and statuses. If there are any errors, the script will output the cURL error message.
Verifying the API Call and Handling Errors
After running the script, verify that the data matches the opportunities in your Sugar Market sandbox account. If you encounter any issues, check the API documentation for error codes and troubleshooting tips. Refer to the Sugar Market API documentation for more details.
Conclusion and Best Practices for Using Sugar Market API with PHP
Integrating with the Sugar Market API using PHP allows developers to efficiently access and manage marketing data, such as opportunities, to enhance marketing strategies and drive business growth. By following the steps outlined in this article, you can set up your environment, authenticate your requests, and retrieve valuable data from Sugar Market.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your client ID and client secret securely. Consider using environment variables or a secure vault to keep these credentials safe.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sugar Market API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your existing systems and processes.
- Error Handling: Implement robust error handling to manage API errors and exceptions effectively. Refer to the Sugar Market API documentation for detailed error code information.
Streamlining Integrations with Endgrate
While integrating with Sugar Market API directly is a powerful option, consider leveraging Endgrate for a more streamlined integration experience. Endgrate allows you to connect with multiple platforms through a single API endpoint, saving time and resources. Focus on your core product while Endgrate handles the complexities of integration.
Explore how Endgrate can simplify your integration processes and enhance your product offerings by visiting Endgrate today.
Read More
Ready to get started?