Using the Affinity API to Get People in Python
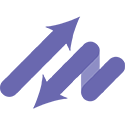
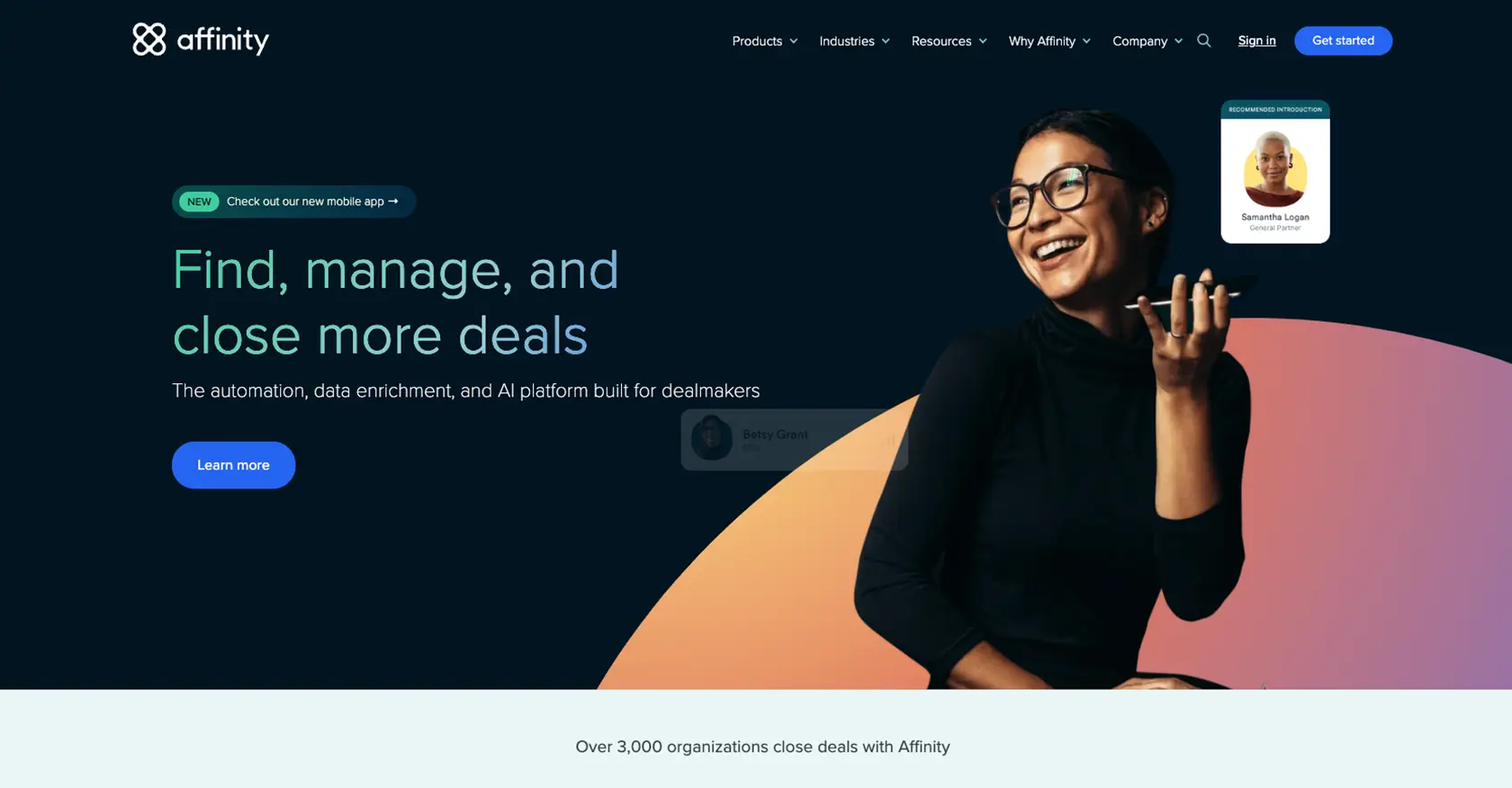
Introduction to Affinity API
Affinity is a powerful relationship intelligence platform that helps businesses manage and leverage their professional networks. By providing a comprehensive view of interactions and connections, Affinity enables organizations to build stronger relationships and drive growth.
Developers might want to integrate with the Affinity API to access and manage contact data efficiently. For example, using the Affinity API, a developer can retrieve detailed information about people within their network, enabling personalized communication strategies and enhancing relationship management.
Setting Up Your Affinity API Test Account
Before you can start using the Affinity API to retrieve people data, you need to set up a test account. This involves generating an API key that will allow you to authenticate your requests securely.
Creating an Affinity Account
If you don't already have an Affinity account, you can sign up for one on the Affinity website. Follow the instructions to create your account, and once completed, you will have access to the Affinity platform.
Generating an API Key for Affinity
To interact with the Affinity API, you need an API key. Follow these steps to generate your API key:
- Log in to your Affinity account.
- Navigate to the Settings panel, accessible from the left sidebar.
- In the Settings panel, find the section for API keys.
- Click on Generate API Key to create a new key.
- Copy the generated API key and store it securely, as you will need it for authenticating your API requests.
For more detailed instructions, you can refer to the Affinity API documentation.
Authenticating API Requests with Your API Key
Affinity uses HTTP Basic Auth for authentication. When making API requests, provide your API key as the password. You do not need to provide a username. Here's an example of how to authenticate your requests:
import requests
# Define the API endpoint
url = "https://api.affinity.co/api_endpoint"
# Make a request with the API key
response = requests.get(url, auth=('', 'Your_API_Key'))
# Check the response
if response.status_code == 200:
print("Request was successful")
else:
print(f"Failed with status code: {response.status_code}")
Replace Your_API_Key
with the API key you generated earlier. This setup will allow you to authenticate and interact with the Affinity API seamlessly.
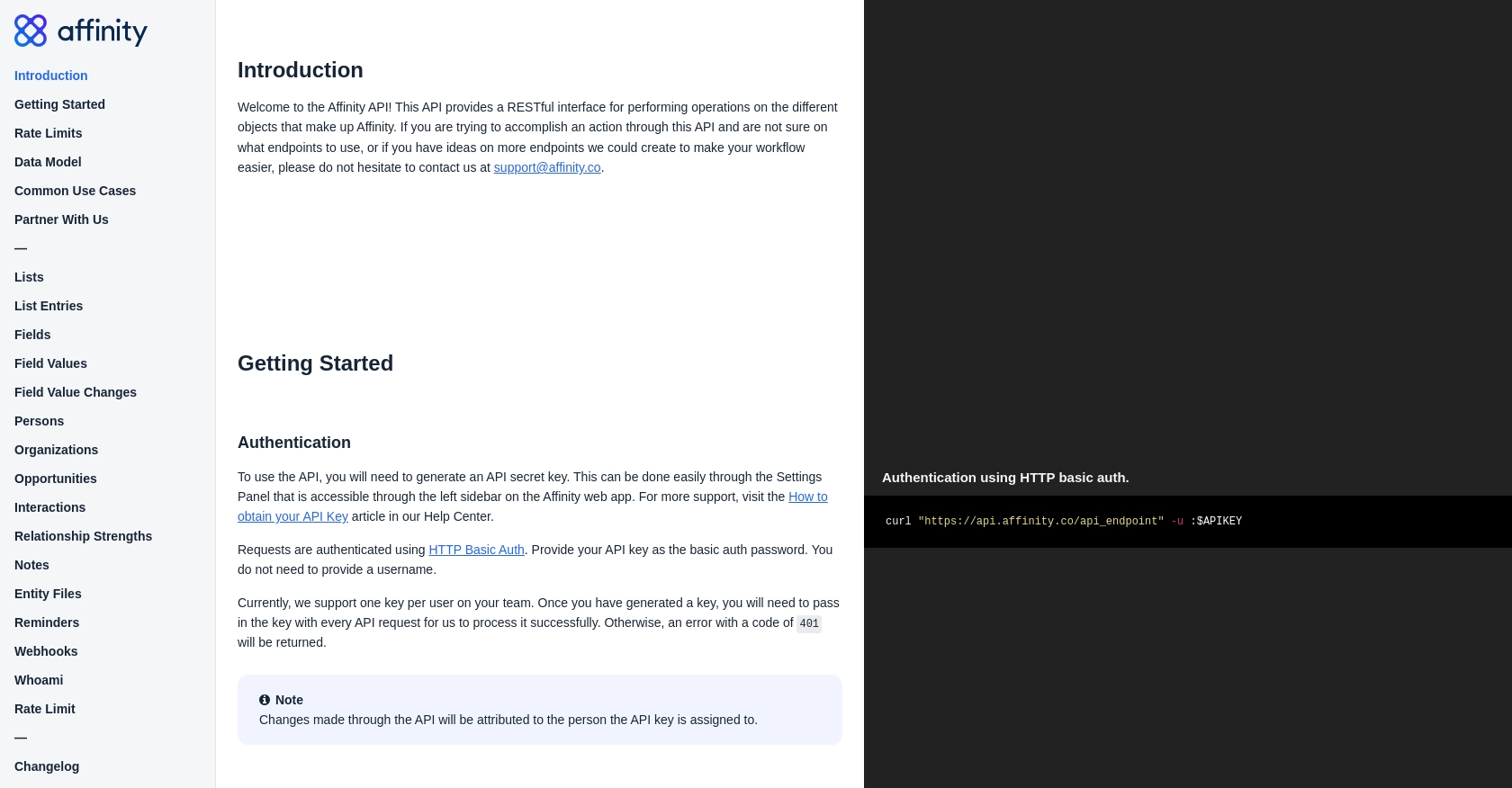
sbb-itb-96038d7
Making API Calls to Retrieve People Data from Affinity Using Python
To interact with the Affinity API and retrieve people data, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the process of making API calls to Affinity, ensuring you have the necessary setup and code to get started.
Setting Up Your Python Environment for Affinity API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Example Code to Retrieve People Data from Affinity API
Once your environment is ready, you can proceed with writing the code to fetch people data. Create a file named get_affinity_people.py
and add the following code:
import requests
# Define the API endpoint for retrieving people
url = "https://api.affinity.co/persons"
# Set up authentication with your API key
api_key = "Your_API_Key"
# Make a GET request to the API
response = requests.get(url, auth=('', api_key))
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON response
data = response.json()
# Loop through the people and print their information
for person in data.get('persons', []):
print(f"Name: {person['first_name']} {person['last_name']}, Email: {person['primary_email']}")
else:
print(f"Failed to retrieve data: {response.status_code}")
Replace Your_API_Key
with the API key you generated earlier. This script makes a GET request to the Affinity API to fetch a list of people and prints their names and primary emails.
Verifying Successful API Requests and Handling Errors
After running the script, you should see a list of people from your Affinity account printed in the console. If the request fails, ensure your API key is correct and check the status code for more information. Common error codes include:
- 401 Unauthorized: Your API key is invalid.
- 403 Forbidden: Insufficient rights to access the resource.
- 404 Not Found: The requested resource does not exist.
- 429 Too Many Requests: You have exceeded the rate limit.
For more details on error codes, refer to the Affinity API documentation.
Conclusion and Best Practices for Using Affinity API in Python
Integrating with the Affinity API using Python provides developers with a powerful tool to manage and leverage professional networks effectively. By accessing detailed contact data, organizations can enhance their relationship management strategies and drive growth.
Best Practices for Secure and Efficient API Integration
- Secure Storage of API Keys: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of the Affinity API's rate limits. The API allows up to 900 requests per user per minute. Implement retry logic to handle
429 Too Many Requests
errors gracefully. - Data Standardization: Ensure that data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage different HTTP status codes and provide meaningful feedback to users.
Streamlining API Integrations with Endgrate
For developers looking to simplify their integration processes, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that connects to multiple platforms, including Affinity, offering an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can streamline your API integrations and enhance your development workflow.
Read More
Ready to get started?