How to Create or Update Accounts with the Xero API in PHP
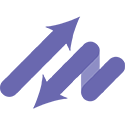
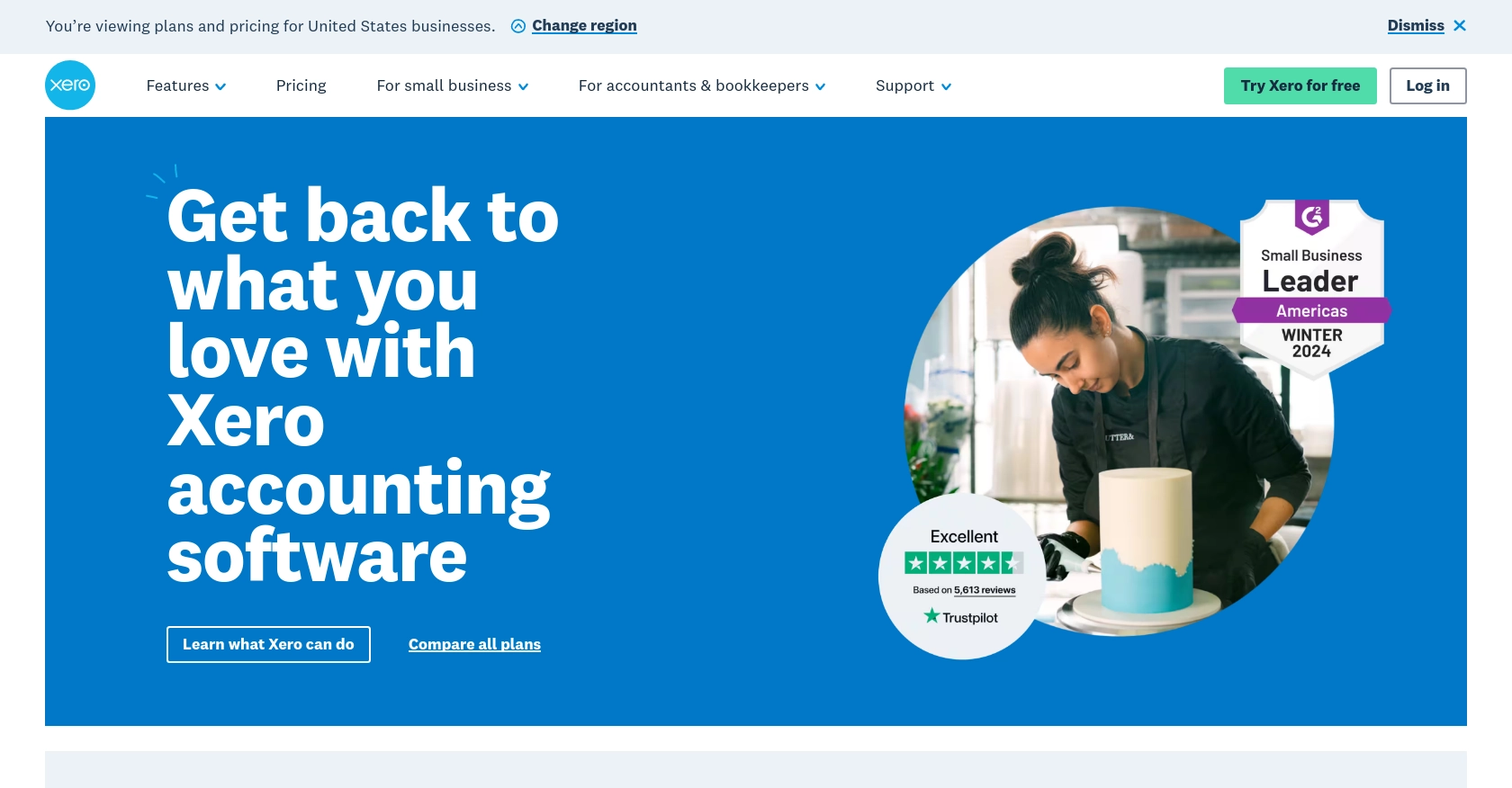
Introduction to Xero Accounting Software
Xero is a powerful cloud-based accounting software designed to simplify financial management for small to medium-sized businesses. With features like invoicing, bank reconciliation, and financial reporting, Xero provides a comprehensive solution for managing business finances efficiently.
Developers may want to integrate with Xero's API to automate accounting tasks, such as creating or updating accounts. For example, a developer could use the Xero API to automatically update account details when a transaction occurs, ensuring that financial records are always up-to-date and accurate.
This article will guide you through the process of using PHP to interact with the Xero API, specifically focusing on creating or updating accounts. By following this tutorial, you'll learn how to streamline your accounting processes and enhance your application's functionality.
Setting Up Your Xero Sandbox Account for API Integration
Before you can start interacting with the Xero API using PHP, you'll need to set up a sandbox account. This will allow you to test your integration without affecting live data. Xero provides a demo company that you can use for this purpose, which is perfect for developers looking to experiment with API calls.
Creating a Xero Developer Account
To begin, you'll need a Xero developer account. Follow these steps to create one:
- Visit the Xero Developer Portal and sign up for a free account.
- Once registered, log in to your developer account.
Accessing the Xero Demo Company
After setting up your developer account, you can access the Xero demo company:
- Navigate to the "My Apps" section in your Xero developer dashboard.
- Select "Try the Demo Company" to access a sandbox environment with sample data.
Creating a Xero App for OAuth Authentication
To interact with the Xero API, you'll need to create an app to obtain the necessary OAuth credentials:
- In the "My Apps" section, click on "New App" to create a new application.
- Fill in the required details, such as the app name and company URL.
- Set the redirect URI to a valid endpoint where you can handle OAuth responses.
- Once your app is created, you'll receive a client ID and client secret. Keep these credentials secure as they are essential for authentication.
Configuring OAuth 2.0 for Xero API Access
Xero uses OAuth 2.0 for authentication. Follow these steps to configure it:
- Use the client ID and client secret obtained from your app to initiate the OAuth flow.
- Direct users to Xero's authorization URL to obtain an authorization code.
- Exchange the authorization code for an access token using Xero's token endpoint.
- Store the access token securely, as it will be used to authenticate API requests.
For more detailed information on OAuth 2.0 with Xero, refer to the Xero OAuth 2.0 Guide.
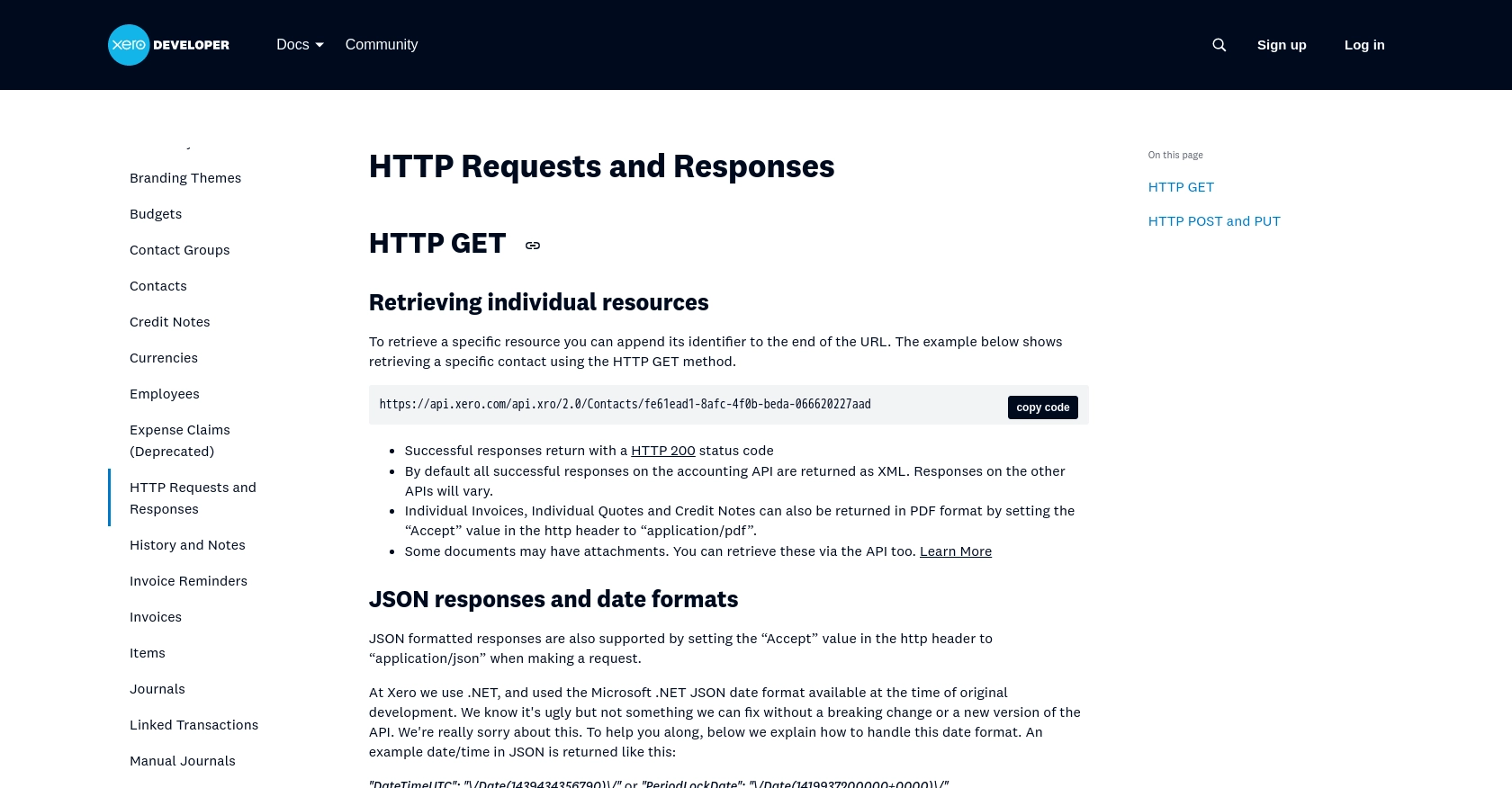
sbb-itb-96038d7
Making API Calls to Xero for Creating or Updating Accounts Using PHP
To interact with the Xero API for creating or updating accounts, you'll need to use PHP to send HTTP requests. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code, and handling responses.
Setting Up Your PHP Environment for Xero API Integration
Before making API calls, ensure your PHP environment is properly configured:
- Install PHP 7.4 or later on your machine.
- Ensure you have Composer installed for managing dependencies.
- Use Composer to install the
guzzlehttp/guzzle
library, which simplifies HTTP requests:
composer require guzzlehttp/guzzle
Writing PHP Code to Create or Update Accounts with Xero API
Now, let's write the PHP code to interact with the Xero API:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token';
$tenantId = 'Your_Tenant_Id';
$url = 'https://api.xero.com/api.xro/2.0/Accounts';
$headers = [
'Authorization' => 'Bearer ' . $accessToken,
'Xero-tenant-id' => $tenantId,
'Content-Type' => 'application/json'
];
$accountData = [
'Code' => '200',
'Name' => 'New Account Name',
'Type' => 'EXPENSE'
];
$response = $client->post($url, [
'headers' => $headers,
'json' => $accountData
]);
if ($response->getStatusCode() == 200) {
echo "Account created or updated successfully.";
} else {
echo "Failed to create or update account.";
}
Replace Your_Access_Token
and Your_Tenant_Id
with your actual access token and tenant ID. The code above uses Guzzle to send a POST request to the Xero API, creating or updating an account with the specified data.
Verifying API Request Success in Xero Sandbox
After running the PHP script, verify the success of your API request:
- Log in to your Xero demo company account.
- Navigate to the Accounts section to check if the new account appears or if existing account details have been updated.
Handling Errors and Understanding Xero API Error Codes
Handling errors is crucial for robust API integration. If the API call fails, check the response status code and message:
- 400 Bad Request: Check your request parameters and data format.
- 401 Unauthorized: Verify your access token and tenant ID.
- 429 Too Many Requests: Respect Xero's rate limits and implement retry logic.
For more details on error handling, refer to the Xero API Requests and Responses Guide.
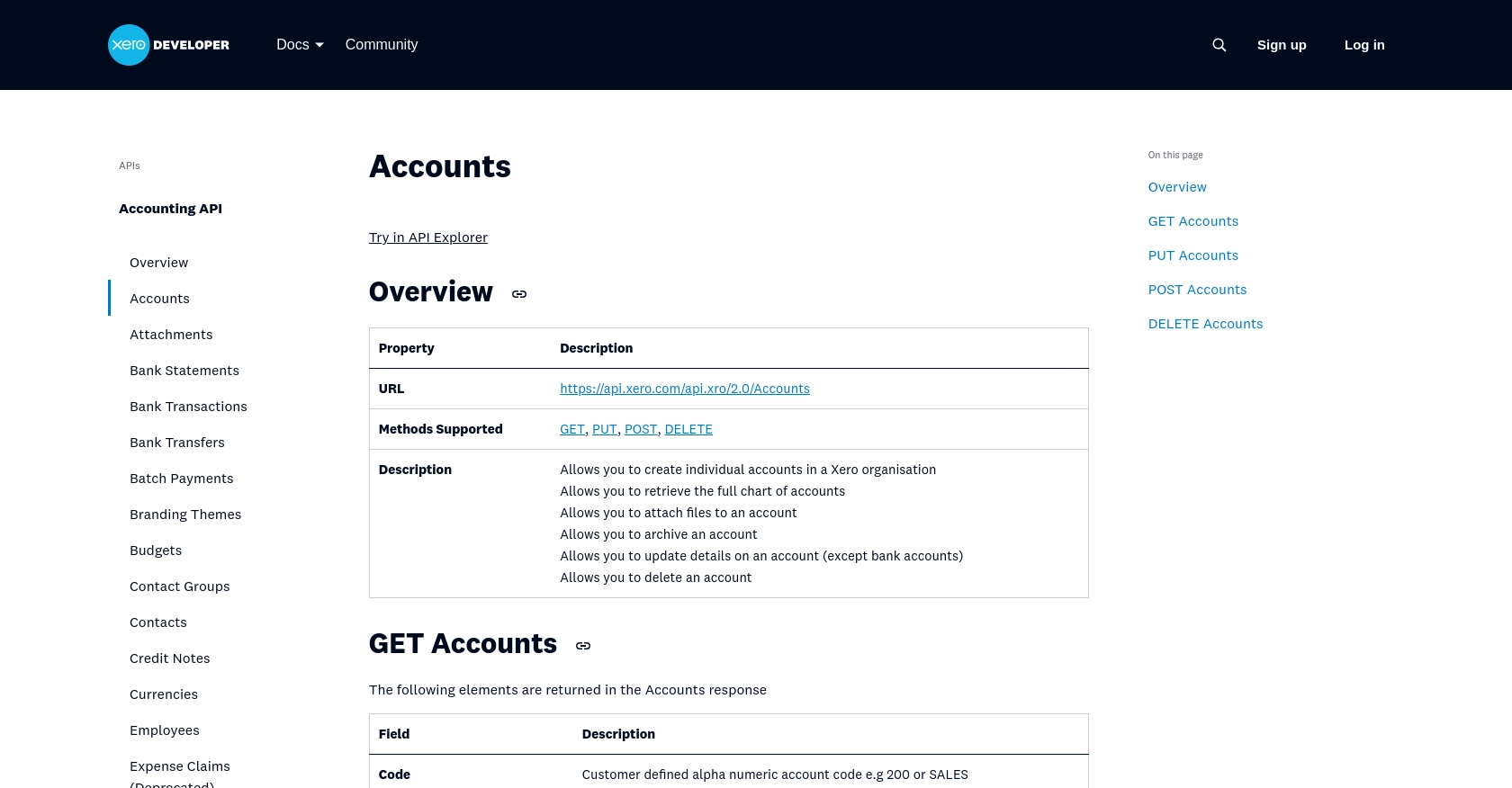
Conclusion and Best Practices for Xero API Integration with PHP
Integrating with the Xero API using PHP can significantly enhance your application's ability to manage accounting tasks efficiently. By automating the creation and updating of accounts, you ensure that your financial data remains accurate and up-to-date, reducing manual effort and the risk of errors.
Best Practices for Secure and Efficient Xero API Usage
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Xero imposes rate limits on API requests. Implement logic to handle
429 Too Many Requests
errors by retrying requests after a delay. For more details, refer to the Xero API Rate Limits Guide. - Data Transformation and Standardization: Ensure that data sent to and received from the Xero API is correctly formatted and standardized to avoid errors and maintain consistency.
Streamlining Integration with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate to simplify the process. Endgrate allows you to build once for each use case, reducing the need for multiple integrations and enabling you to focus on your core product. With Endgrate, you can provide an intuitive integration experience for your customers, saving time and resources.
For more information on how Endgrate can help streamline your integration efforts, visit Endgrate.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/accounts
Ready to get started?