How to Create Or Update Companies with the Pipeliner CRM API in Python
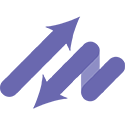
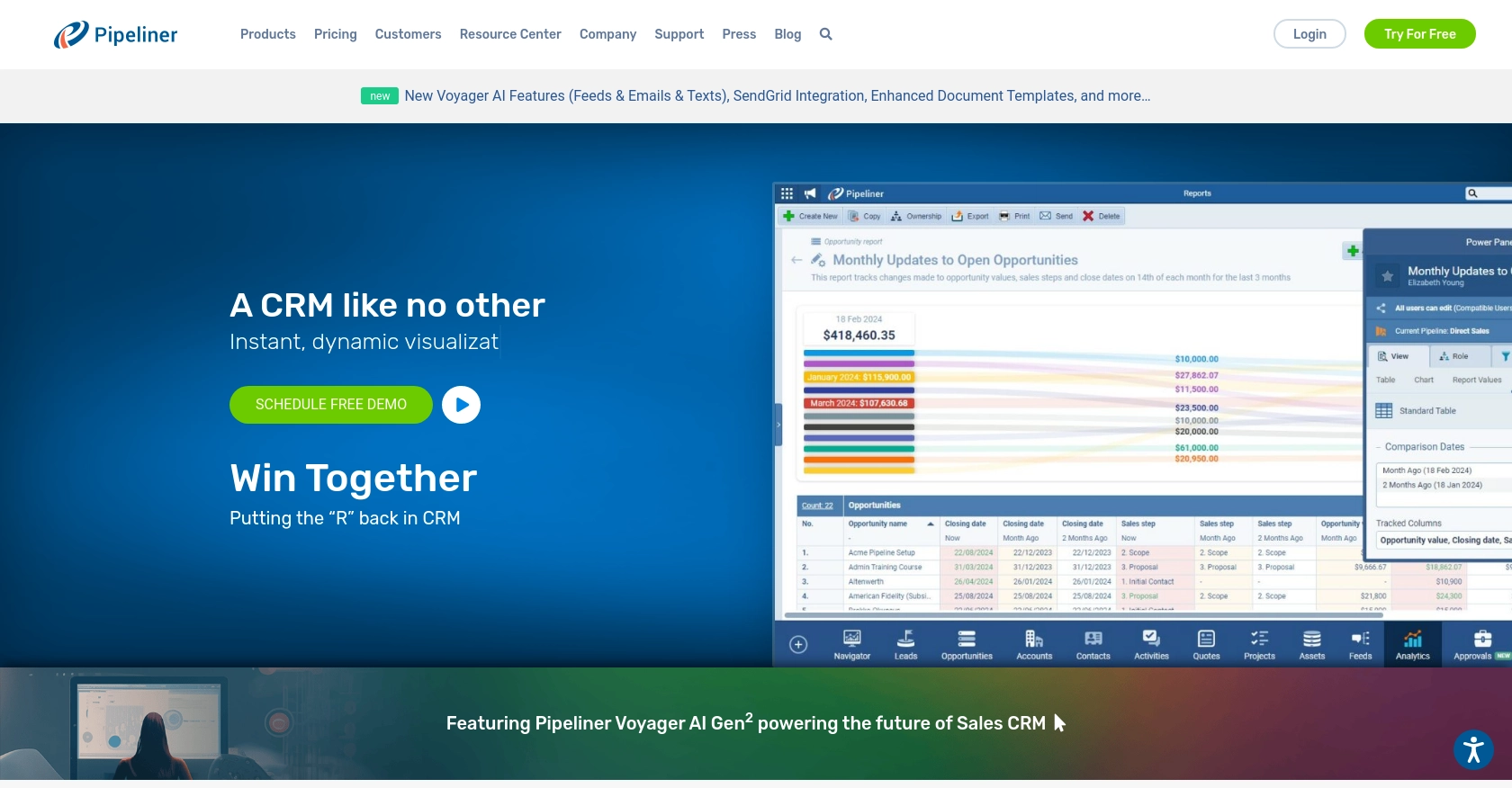
Introduction to Pipeliner CRM
Pipeliner CRM is a robust customer relationship management platform designed to enhance sales processes and improve team collaboration. It offers a comprehensive suite of tools for managing leads, opportunities, and customer interactions, making it a popular choice for businesses aiming to streamline their sales operations.
Integrating with the Pipeliner CRM API allows developers to automate and optimize various sales activities, such as creating or updating company records. For example, a developer might use the Pipeliner CRM API to automatically update company information based on new data from external sources, ensuring that sales teams always have access to the most current information.
Setting Up Your Pipeliner CRM Test Account
Before you can start integrating with the Pipeliner CRM API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to create a sandbox environment:
Step 1: Sign Up for a Pipeliner CRM Account
If you don't already have a Pipeliner CRM account, visit the Pipeliner CRM website and sign up for a free trial. This will give you access to the platform's features and allow you to create a test environment.
Step 2: Access Your Pipeliner CRM Space
Once your account is set up, log in to your Pipeliner CRM space. This is where you'll manage your CRM data and configure API access.
Step 3: Create an Application for API Access
To interact with the Pipeliner CRM API, you'll need to create an application within your CRM space:
- Navigate to the Administration section.
- Under the Unit, Users & Roles tab, select Applications.
- Create a new application by clicking on the Create button.
Step 4: Obtain API Keys for Authentication
Pipeliner CRM uses Basic Authentication, which requires a username and password. These are generated as API keys:
- After creating the application, click on Show API Access.
- Copy the Username and Password. These credentials are generated only once, so store them securely.
These credentials will be used to authenticate your API requests.
Step 5: Note Your Space ID and Service URL
Every API request requires a Space ID and Service URL. Ensure you have these details noted down as they are crucial for making API calls.
With your test account and API keys ready, you can now proceed to make API calls to create or update companies in Pipeliner CRM using Python.
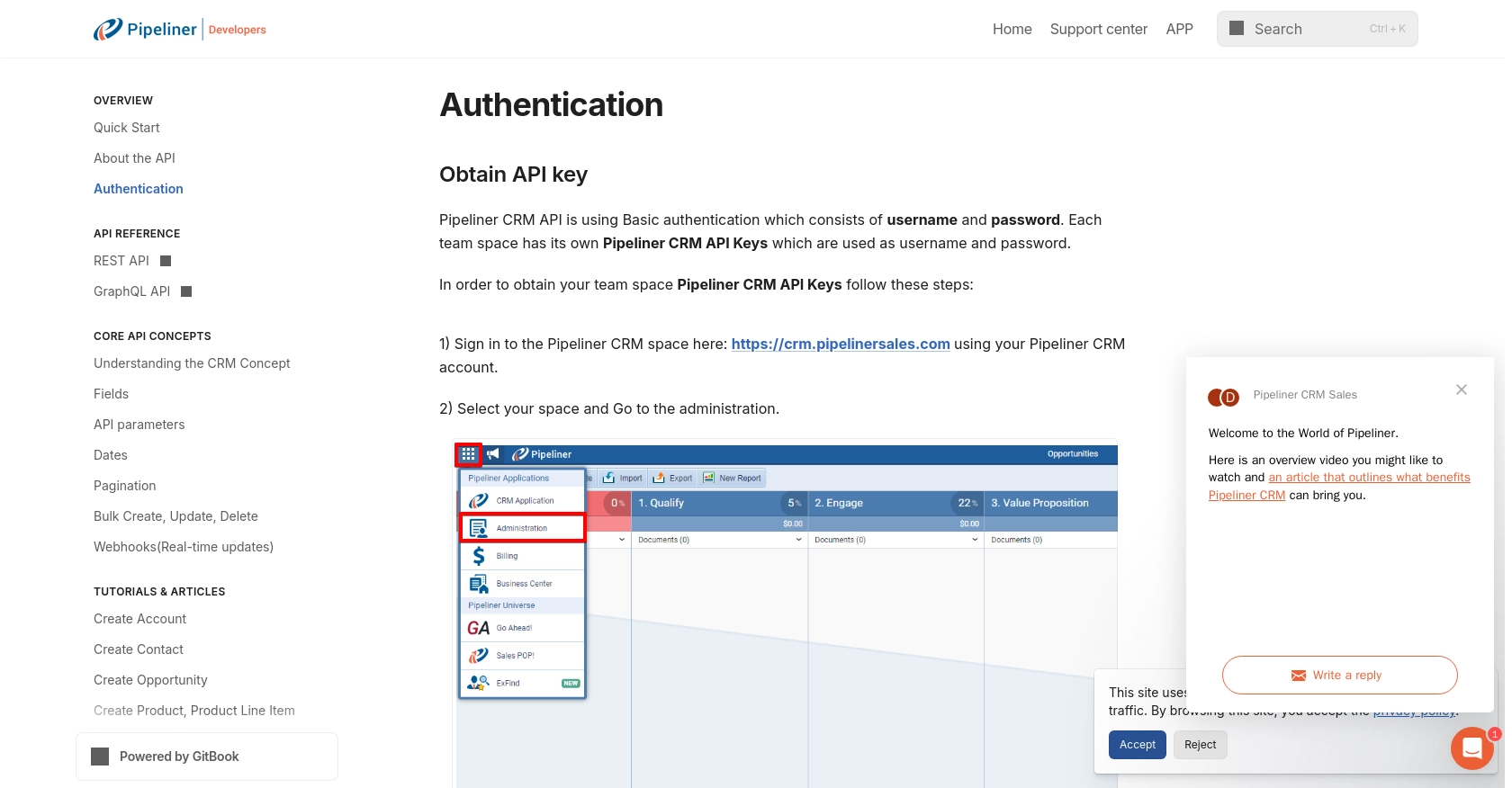
sbb-itb-96038d7
How to Make API Calls to Create or Update Companies in Pipeliner CRM Using Python
Setting Up Your Python Environment for Pipeliner CRM API Integration
To interact with the Pipeliner CRM API using Python, you'll need to ensure your development environment is properly configured. This includes having the correct version of Python and necessary libraries installed.
- Ensure you have Python 3.7 or later installed on your machine.
- Install the
requests
library, which is essential for making HTTP requests. You can do this by running the following command in your terminal:
pip install requests
Creating a Company in Pipeliner CRM Using Python
To create a company in Pipeliner CRM, you'll need to make a POST request to the appropriate endpoint. Here's a step-by-step guide:
import requests
# Define the API endpoint and your credentials
service_url = "https://your-service-url"
space_id = "your-space-id"
username = "your-username"
password = "your-password"
# Set the endpoint for creating a company
url = f"{service_url}/api/v1/{space_id}/companies"
# Define the company data
company_data = {
"name": "New Company",
"industry": "Technology",
"website": "https://newcompany.com"
}
# Make the POST request
response = requests.post(url, json=company_data, auth=(username, password))
# Check if the request was successful
if response.status_code == 201:
print("Company created successfully.")
else:
print(f"Failed to create company: {response.status_code} - {response.text}")
Replace your-service-url
, your-space-id
, your-username
, and your-password
with your actual Pipeliner CRM credentials. Upon successful execution, the script will create a new company in your Pipeliner CRM space.
Updating a Company in Pipeliner CRM Using Python
To update an existing company, you'll need to make a PUT request. Here's how you can do it:
import requests
# Define the API endpoint and your credentials
service_url = "https://your-service-url"
space_id = "your-space-id"
username = "your-username"
password = "your-password"
# Set the endpoint for updating a company
company_id = "existing-company-id"
url = f"{service_url}/api/v1/{space_id}/companies/{company_id}"
# Define the updated company data
updated_data = {
"name": "Updated Company Name",
"industry": "Updated Industry"
}
# Make the PUT request
response = requests.put(url, json=updated_data, auth=(username, password))
# Check if the request was successful
if response.status_code == 200:
print("Company updated successfully.")
else:
print(f"Failed to update company: {response.status_code} - {response.text}")
Replace existing-company-id
with the ID of the company you wish to update. This script will modify the specified company's details in your Pipeliner CRM space.
Verifying API Call Success in Pipeliner CRM
After making API calls, you can verify the success of your requests by checking the Pipeliner CRM interface. Newly created or updated companies should reflect the changes made through your API calls.
- Log in to your Pipeliner CRM account.
- Navigate to the Companies section to view the list of companies.
- Confirm that the new or updated company details are present.
Handling Errors and API Response Codes
When making API calls, it's crucial to handle potential errors effectively. The Pipeliner CRM API will return various HTTP status codes to indicate the result of your request:
- 201 Created: The company was successfully created.
- 200 OK: The company was successfully updated.
- 400 Bad Request: The request was malformed or missing required fields.
- 401 Unauthorized: Authentication failed due to invalid credentials.
- 404 Not Found: The specified company ID does not exist.
Ensure your code includes error handling to manage these responses appropriately and provide meaningful feedback to users.
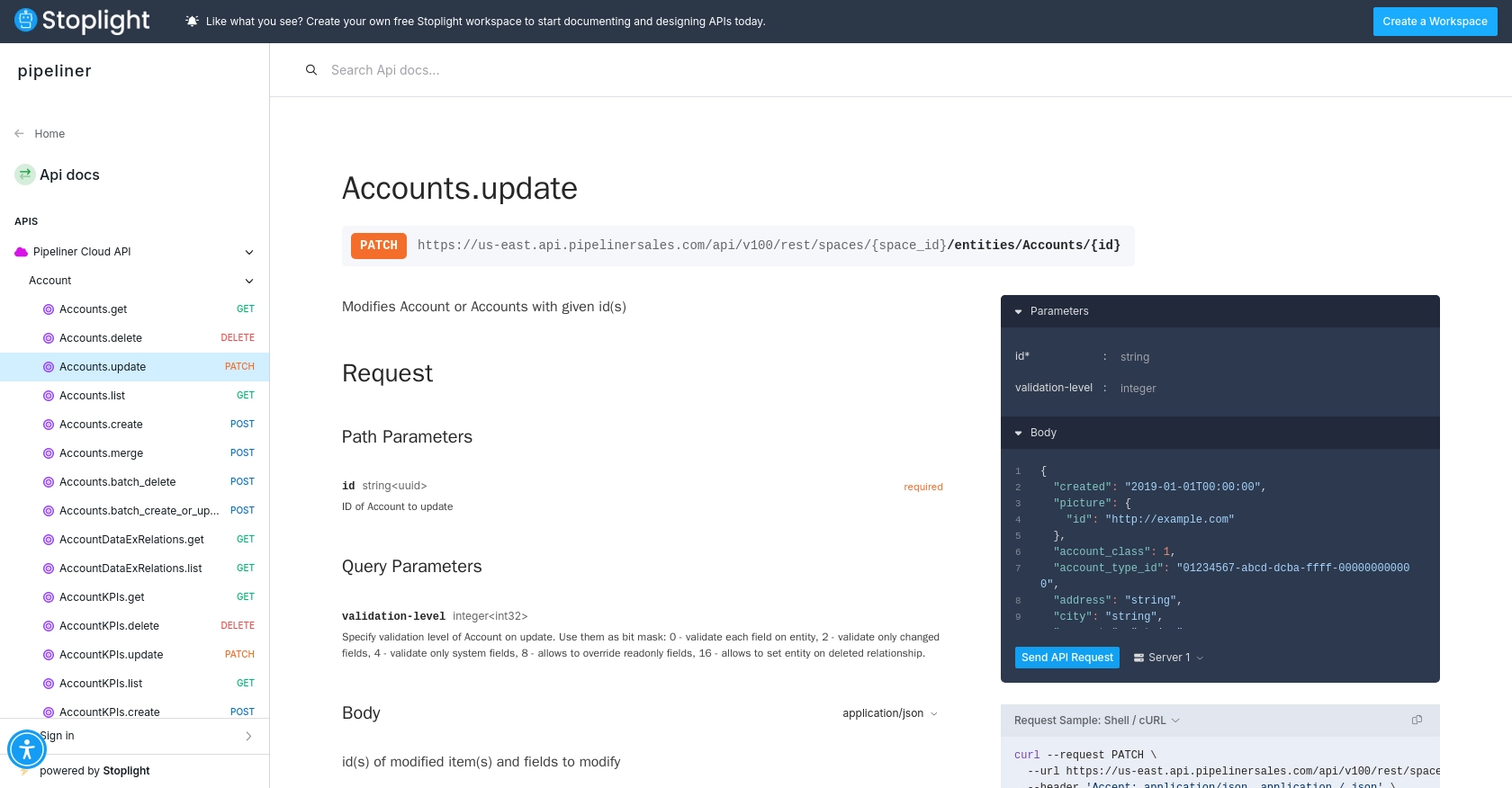
Conclusion and Best Practices for Pipeliner CRM API Integration
Integrating with the Pipeliner CRM API using Python can significantly enhance your sales processes by automating the creation and updating of company records. By following the steps outlined in this guide, you can ensure a smooth and efficient integration experience.
Best Practices for Storing Pipeliner CRM API Credentials
- Store API credentials securely, using environment variables or a secure vault, to prevent unauthorized access.
- Regularly rotate your API keys and update your application to use the new credentials.
Handling Pipeliner CRM API Rate Limits
Be mindful of the API rate limits to avoid service disruptions. Implement logic to handle rate limiting by checking the response headers for rate limit information and retrying requests after the specified wait time.
Data Transformation and Standardization
- Ensure data consistency by transforming and standardizing data fields before sending them to the Pipeliner CRM API.
- Use validation checks to ensure data integrity and prevent errors during API calls.
Streamlining Integrations with Endgrate
For developers looking to simplify their integration processes, consider using Endgrate. Endgrate provides a unified API endpoint that connects to multiple platforms, including Pipeliner CRM, allowing you to focus on your core product while outsourcing complex integrations.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can help streamline your integration efforts.
Read More
- https://endgrate.com/provider/pipelinercrm
- https://developers.pipelinersales.com/api-docs/overview/authentication
- https://developers.pipelinersales.com/api-docs/core-api-concepts/pagination
- https://pipeliner.stoplight.io/docs/api-docs/p5b3cyeorbick-accounts-update
- https://pipeliner.stoplight.io/docs/api-docs/89p8xi32mv7cj-accounts-create
Ready to get started?