How to Get Contacts with the SendGrid API in Python
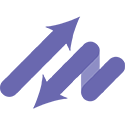
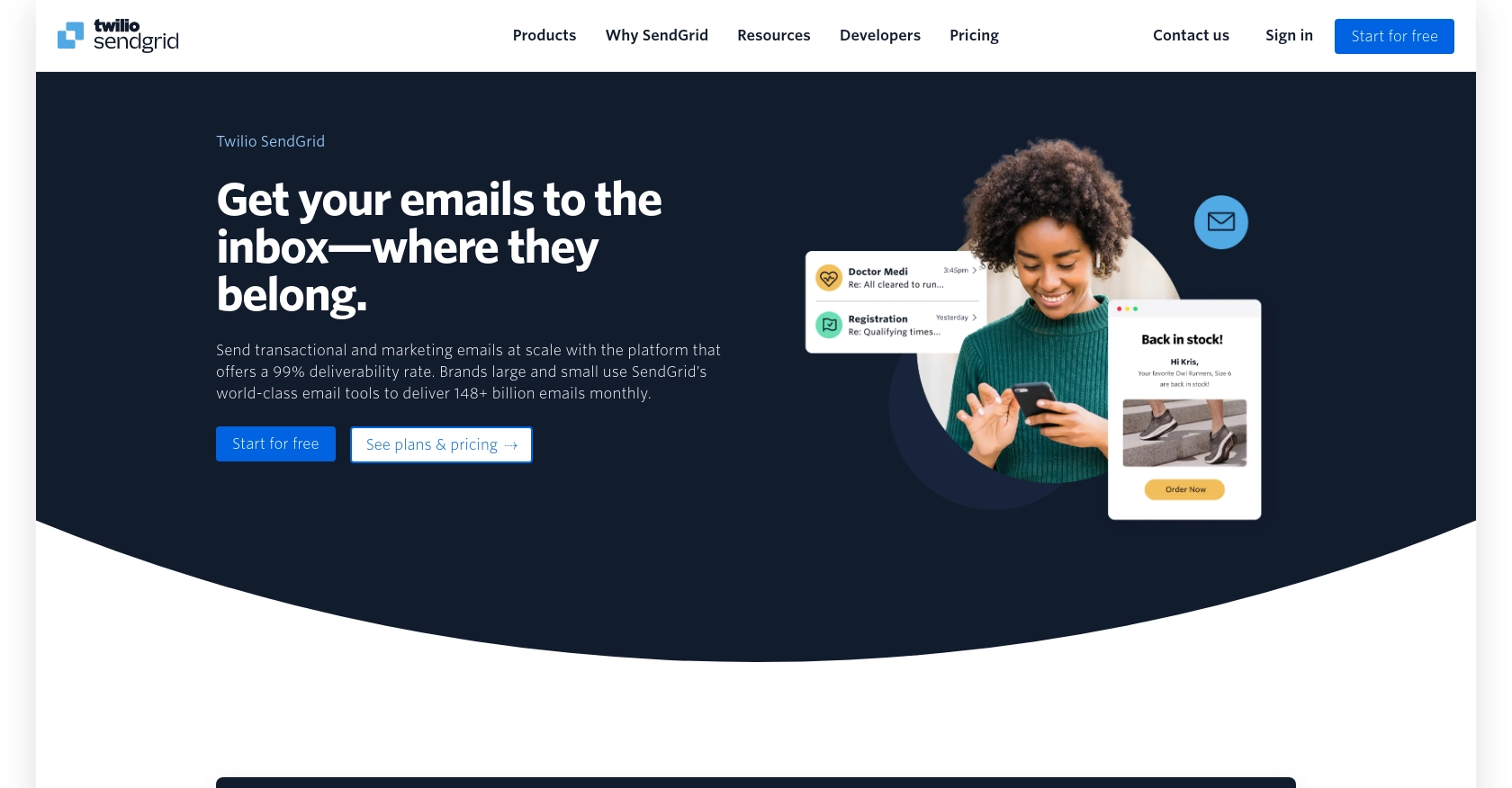
Introduction to SendGrid API for Contact Management
SendGrid, a cloud-based email delivery service, is renowned for its robust email marketing capabilities and reliable transactional email services. It offers a comprehensive API that allows developers to manage email campaigns, track email performance, and handle contact data efficiently.
Integrating with SendGrid's API can significantly enhance a developer's ability to manage contacts within their email marketing campaigns. For example, using the SendGrid API, developers can retrieve contact information based on email addresses, enabling them to personalize communications and improve engagement rates.
This article will guide you through the process of using Python to interact with the SendGrid API, specifically focusing on retrieving contacts by their email addresses. By following this tutorial, you'll learn how to streamline your email marketing efforts and automate contact management tasks.
Setting Up Your SendGrid Account for API Access
Before you can start using the SendGrid API to manage contacts, you'll need to set up your SendGrid account. This involves creating an account, generating an API key, and configuring the necessary permissions. Follow these steps to get started:
Create a SendGrid Account
If you don't already have a SendGrid account, you can sign up for a free account on the SendGrid website. The free plan provides access to essential features, including API access, which is sufficient for testing and development purposes.
Generate a SendGrid API Key
Once your account is set up, you'll need to generate an API key to authenticate your requests to the SendGrid API. Follow these steps to create an API key:
- Log in to your SendGrid account.
- Navigate to the "Settings" section in the left-hand menu.
- Select "API Keys" from the dropdown menu.
- Click on "Create API Key" to generate a new key.
- Provide a name for your API key and select the appropriate permissions. For contact management, ensure you have the necessary read permissions.
- Click "Create & View" to generate the key. Make sure to copy and store the key securely, as you won't be able to view it again.
Configure API Key Permissions
Ensure that your API key has the correct permissions to access the contacts endpoint. You can set these permissions during the API key creation process. For more details on permissions, refer to the SendGrid API Authentication Documentation.
Verify API Key Functionality
To verify that your API key is working correctly, you can make a simple API call to check its functionality. Use the following Python code snippet to test your API key:
import requests
url = "https://api.sendgrid.com/v3/marketing/contacts"
headers = {
"Authorization": "Bearer YOUR_API_KEY",
"Content-Type": "application/json"
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
print("API Key is working correctly.")
else:
print("Failed to authenticate. Check your API Key and permissions.")
Replace YOUR_API_KEY
with the API key you generated. If the response status code is 200, your API key is set up correctly.
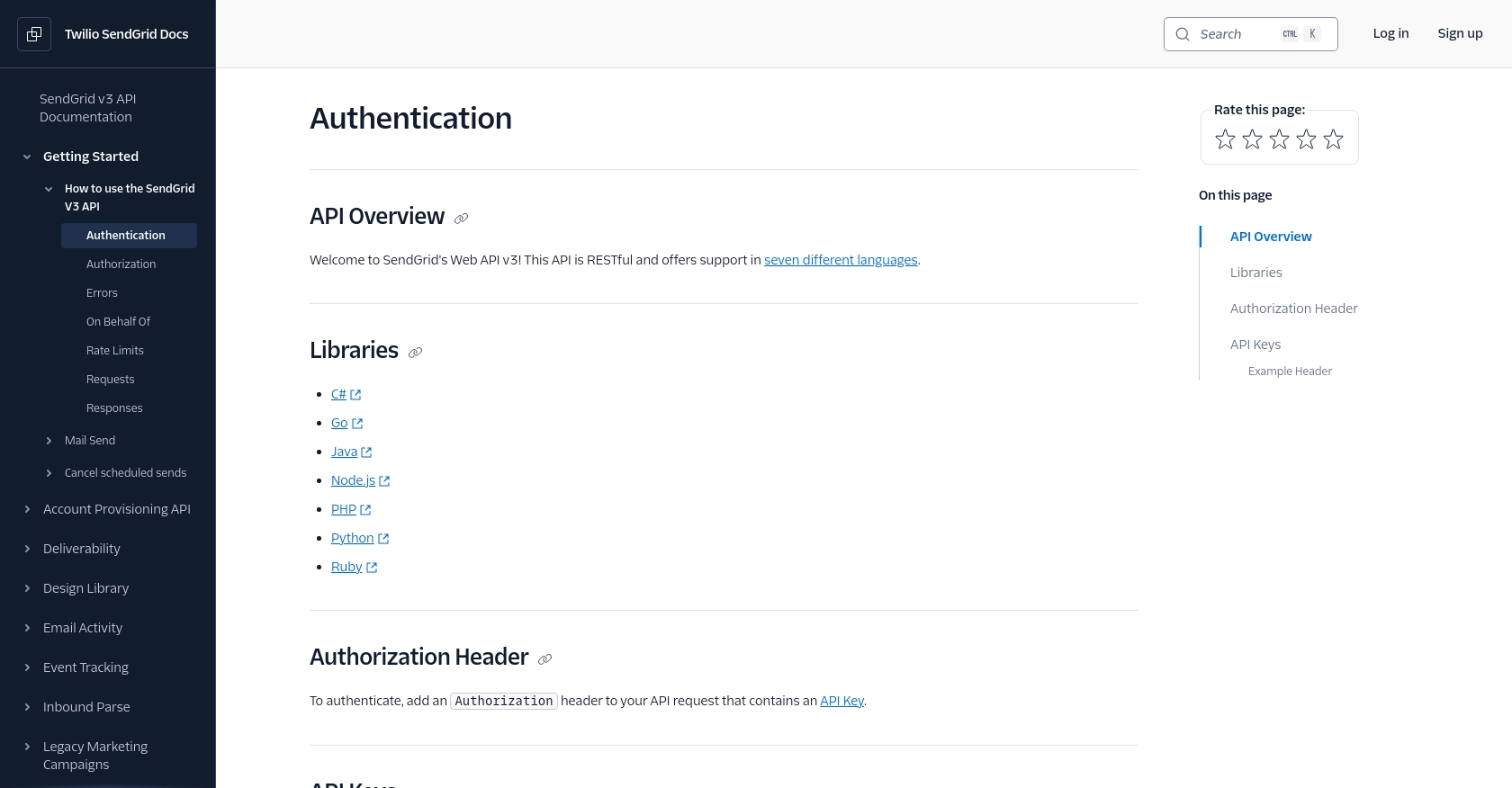
sbb-itb-96038d7
Making API Calls to Retrieve Contacts Using SendGrid API in Python
To interact with the SendGrid API and retrieve contacts by their email addresses, you'll need to use Python. This section will guide you through setting up your Python environment, installing necessary dependencies, and executing the API call to fetch contact data.
Setting Up Your Python Environment for SendGrid API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Writing Python Code to Fetch Contacts from SendGrid
Create a new Python file named get_sendgrid_contacts.py
and add the following code to retrieve contacts by email addresses:
import requests
# Define the API endpoint and headers
url = "https://api.sendgrid.com/v3/marketing/contacts/search/emails"
headers = {
"Authorization": "Bearer YOUR_API_KEY",
"Content-Type": "application/json"
}
# Define the request body with email addresses to search
data = {
"emails": [
"jane_doe@example.com",
"john_doe@example.com"
]
}
# Make a POST request to the API
response = requests.post(url, headers=headers, json=data)
# Check if the request was successful
if response.status_code == 200:
contacts = response.json()
print("Contacts retrieved successfully:")
print(contacts)
else:
print(f"Failed to retrieve contacts. Status code: {response.status_code}")
print(response.json())
Replace YOUR_API_KEY
with your actual SendGrid API key. The emails
array should contain the email addresses you wish to search for.
Understanding the API Response and Handling Errors
When the API call is successful, the response will include the contact details for the provided email addresses. If any email address is not found, the response will include an error message for that address. Here’s how you can handle different response scenarios:
- Success (200): The contacts are retrieved successfully. The response will include the contact details.
- Not Found (404): No contacts are found for the provided email addresses.
- Invalid Request (400): The request contains invalid email addresses.
For more details on error handling, refer to the SendGrid API Authentication Documentation.
Verifying the API Call in Your SendGrid Account
After running the script, you can verify the retrieved contacts by checking your SendGrid account. Ensure the contacts match the data returned by the API call. This verification step helps confirm that your integration is working correctly.
Handling Rate Limits with SendGrid API
SendGrid imposes rate limits on API requests. If you exceed these limits, you'll receive a status code 429. To handle rate limits effectively, consider implementing retry logic with exponential backoff. For more information, see the SendGrid Rate Limits Documentation.
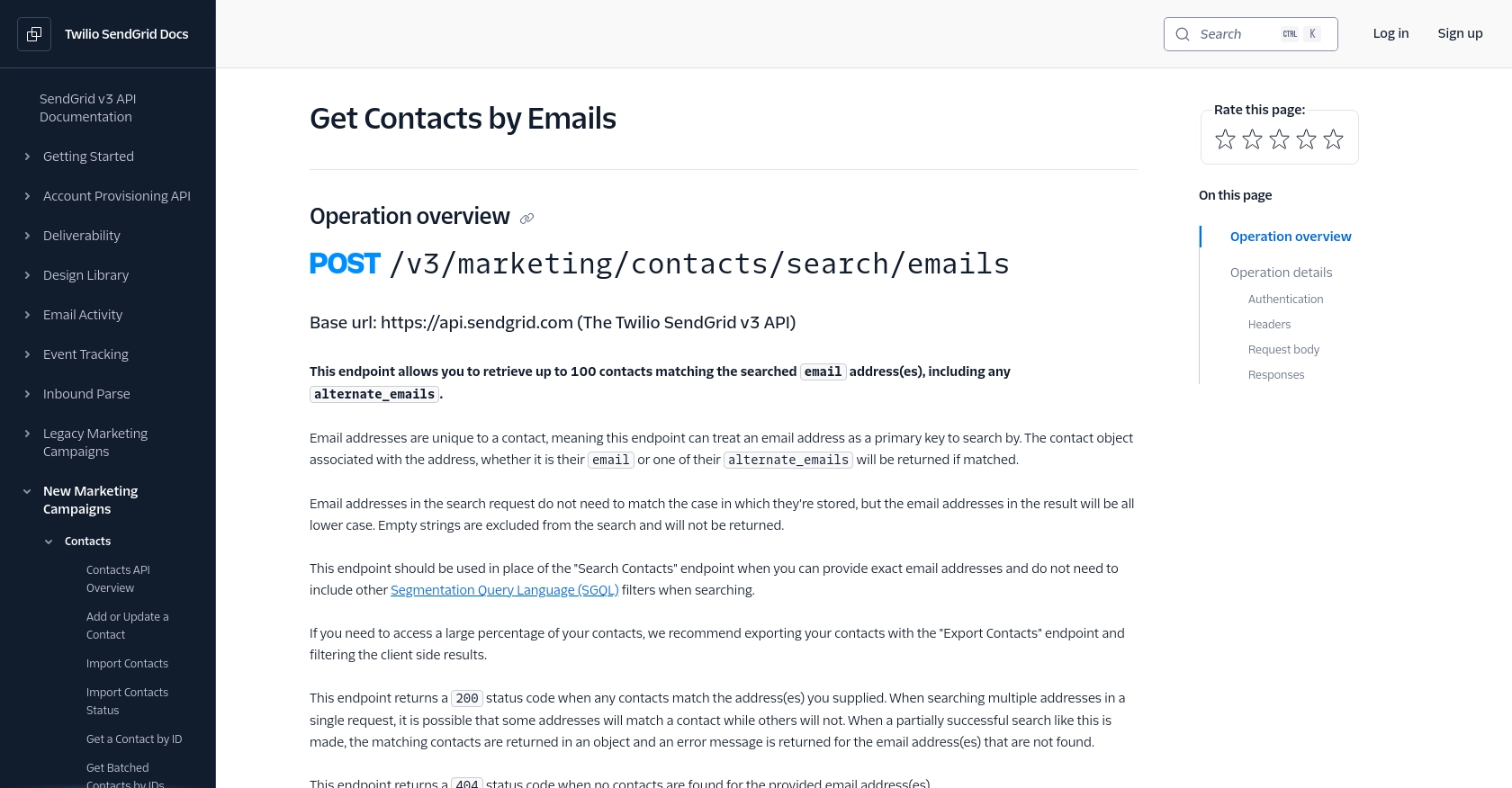
Conclusion and Best Practices for Using SendGrid API in Python
Integrating with the SendGrid API using Python allows developers to efficiently manage and retrieve contact information, enhancing email marketing efforts. By following the steps outlined in this guide, you can streamline your contact management processes and improve communication with your audience.
Best Practices for Secure and Efficient API Usage
- Secure API Key Storage: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limits: Implement retry logic with exponential backoff to manage rate limits effectively. This ensures your application remains responsive even when limits are reached. For more details, refer to the SendGrid Rate Limits Documentation.
- Data Standardization: Ensure that contact data is standardized and validated before making API calls to avoid errors and maintain data integrity.
- Error Handling: Implement comprehensive error handling to manage different response scenarios, such as invalid requests or not found errors, to improve user experience.
Enhance Your Integration Strategy with Endgrate
While integrating with SendGrid is a powerful way to manage your email marketing contacts, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including SendGrid.
By using Endgrate, you can save time and resources, allowing you to focus on your core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/sendgrid
- https://www.twilio.com/docs/sendgrid/api-reference/how-to-use-the-sendgrid-v3-api/authentication
- https://www.twilio.com/docs/sendgrid/api-reference/how-to-use-the-sendgrid-v3-api/authorization
- https://www.twilio.com/docs/sendgrid/api-reference/how-to-use-the-sendgrid-v3-api/rate-limits
- https://www.twilio.com/docs/sendgrid/api-reference/contacts/get-contacts-by-emails
Ready to get started?