Using the Teamwork CRM API to Create or Update Contacts in Python
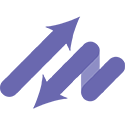
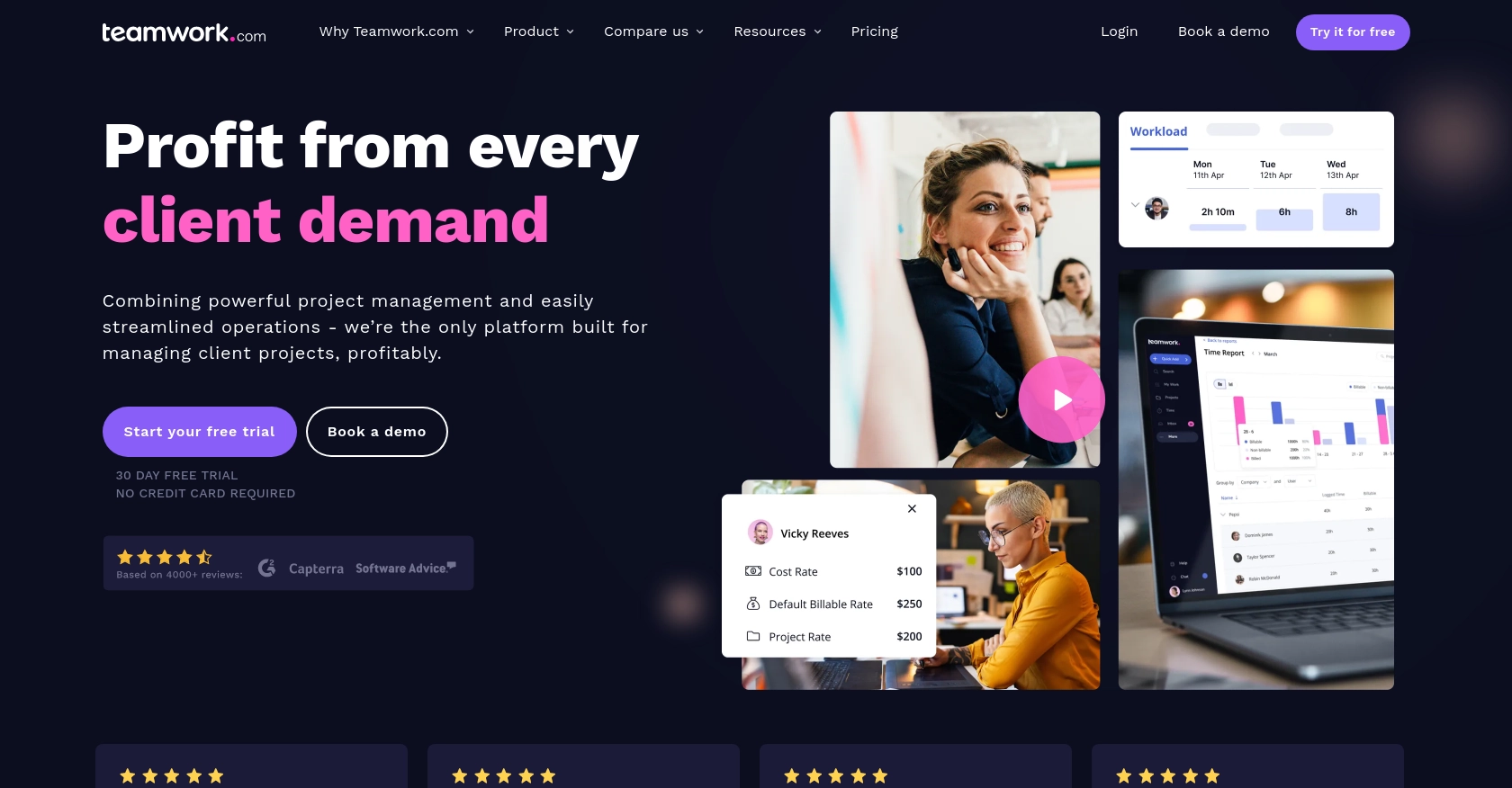
Introduction to Teamwork CRM API
Teamwork CRM is a robust customer relationship management platform designed to help businesses manage their sales processes more effectively. It offers a comprehensive suite of tools for tracking leads, managing contacts, and optimizing sales workflows, making it an essential tool for sales teams aiming to enhance productivity and close deals faster.
Integrating with the Teamwork CRM API allows developers to automate and streamline CRM tasks, such as creating or updating contact information directly from their applications. For example, a developer might use the Teamwork CRM API to automatically update contact details when a user submits a form on a website, ensuring that the sales team always has the most current information at their fingertips.
Setting Up Your Teamwork CRM Test Account
Before you can start integrating with the Teamwork CRM API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Teamwork CRM Account
If you don't already have a Teamwork CRM account, you can sign up for a free trial on the Teamwork CRM website. Follow the instructions to create your account. Once your account is set up, you'll have access to the CRM dashboard where you can manage your contacts and other CRM data.
Generating API Credentials for Teamwork CRM
To interact with the Teamwork CRM API, you'll need to generate API credentials. Teamwork CRM supports OAuth 2.0 for authentication, which requires you to register your application and obtain a client ID and client secret.
- Log in to your Teamwork CRM account and navigate to the Developer Portal.
- Register a new application by providing the necessary details such as the application name and redirect URL.
- Once registered, you'll receive a client ID and client secret. Keep these credentials secure as they are essential for authenticating your API requests.
Authenticating API Requests with OAuth 2.0
With your client ID and client secret, you can implement the OAuth 2.0 flow to obtain an access token. This token will be used to authenticate your API requests.
- Use the client ID and client secret to request an access token from the Teamwork CRM authorization server.
- Include the access token in the Authorization header of your API requests as follows:
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN"
}
Replace YOUR_ACCESS_TOKEN
with the token obtained from the OAuth 2.0 flow.
Testing Your API Setup
To ensure your setup is correct, make a simple API call to retrieve a list of contacts. If successful, this confirms that your authentication is working properly.
import requests
url = "https://{yourSiteName}.teamwork.com/crm/v2/contacts"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN"
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
print("API setup successful. Contacts retrieved.")
else:
print("Error:", response.status_code, response.text)
Replace {yourSiteName}
with your actual Teamwork CRM site name and YOUR_ACCESS_TOKEN
with your access token.
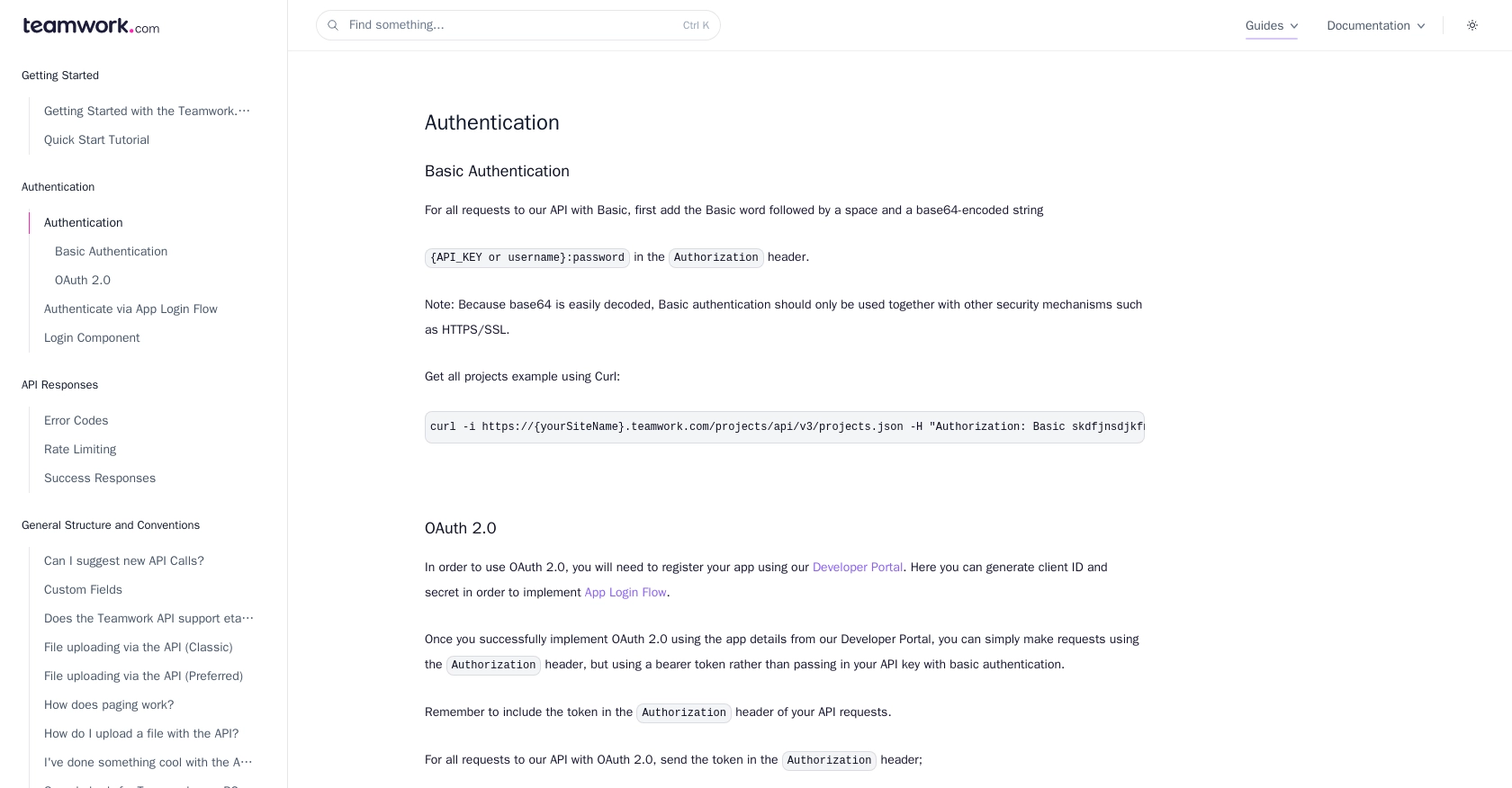
sbb-itb-96038d7
Making API Calls to Teamwork CRM for Contact Management Using Python
Setting Up Your Python Environment for Teamwork CRM API Integration
To interact with the Teamwork CRM API using Python, you need to ensure that your development environment is properly configured. This includes having the correct version of Python and necessary libraries installed.
- Ensure you have Python 3.7 or later installed on your machine.
- Install the
requests
library, which is essential for making HTTP requests. You can install it using pip:
pip install requests
Creating or Updating Contacts in Teamwork CRM Using Python
Once your environment is set up, you can proceed to create or update contacts in Teamwork CRM. The following Python code demonstrates how to perform these operations using the Teamwork CRM API.
Creating a New Contact
To create a new contact, you'll need to send a POST request to the Teamwork CRM API endpoint for contacts. Here's an example:
import requests
# Define the API endpoint for creating contacts
url = "https://{yourSiteName}.teamwork.com/crm/v2/contacts"
# Set the request headers with the access token
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Define the contact data
contact_data = {
"contact": {
"firstName": "John",
"lastName": "Doe",
"email": "john.doe@example.com"
}
}
# Make the POST request to create the contact
response = requests.post(url, headers=headers, json=contact_data)
# Check the response status
if response.status_code == 201:
print("Contact created successfully.")
else:
print("Error:", response.status_code, response.text)
Replace {yourSiteName}
with your actual Teamwork CRM site name and YOUR_ACCESS_TOKEN
with your access token.
Updating an Existing Contact
To update an existing contact, you'll need to send a PUT request to the API endpoint with the contact ID. Here's how you can do it:
# Define the API endpoint for updating a contact
contact_id = "12345" # Replace with the actual contact ID
url = f"https://{yourSiteName}.teamwork.com/crm/v2/contacts/{contact_id}"
# Define the updated contact data
updated_contact_data = {
"contact": {
"firstName": "Jane",
"lastName": "Doe",
"email": "jane.doe@example.com"
}
}
# Make the PUT request to update the contact
response = requests.put(url, headers=headers, json=updated_contact_data)
# Check the response status
if response.status_code == 200:
print("Contact updated successfully.")
else:
print("Error:", response.status_code, response.text)
Ensure you replace contact_id
with the actual ID of the contact you wish to update.
Handling API Responses and Errors
When making API calls, it's crucial to handle responses and potential errors effectively. Teamwork CRM API provides various status codes to indicate the result of your requests:
- 201 Created: Indicates that a new contact was successfully created.
- 200 OK: Indicates that an existing contact was successfully updated.
- 400 Bad Request: The request was invalid. Check your data and try again.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 422 Unprocessable Entity: The server understands the request but can't process it. Check your JSON structure.
For more detailed error handling, refer to the Teamwork CRM error codes documentation.
Verifying API Call Success in Teamwork CRM
After making API calls, you can verify their success by checking the Teamwork CRM dashboard. Newly created or updated contacts should appear in the contacts list, reflecting the changes made through your API requests.
Best Practices for Using Teamwork CRM API in Python
When integrating with the Teamwork CRM API, it's essential to follow best practices to ensure a smooth and secure implementation. Here are some recommendations:
- Securely Store Credentials: Always store your API credentials, such as client ID, client secret, and access tokens, securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Teamwork CRM enforces rate limits based on your subscription plan. For example, the Grow Plan allows 150 requests per minute. Monitor the
X-Rate-Limit-Remaining
header in API responses to avoid exceeding limits. Implement retry logic with exponential backoff to handle429 Too Many Requests
errors gracefully. For more details, refer to the Teamwork CRM rate limiting documentation. - Validate API Responses: Always check the status codes and response data to ensure your API calls are successful. Implement error handling for common issues such as
400 Bad Request
and401 Unauthorized
. - Data Transformation and Standardization: When dealing with contact data, ensure that fields are standardized and validated before sending them to the API. This helps maintain data integrity and consistency.
Streamlining Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex, especially when dealing with multiple platforms. Endgrate simplifies this process by providing a unified API endpoint that connects to various services, including Teamwork CRM.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product by outsourcing integration tasks to Endgrate, reducing development time and costs.
- Build Once, Deploy Everywhere: Create a single integration for each use case and deploy it across multiple platforms without additional development effort.
- Enhance User Experience: Offer your customers a seamless and intuitive integration experience with Endgrate's robust API solutions.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?