How to Create or Update Records with the MongoDB API in Python
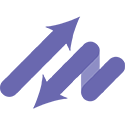
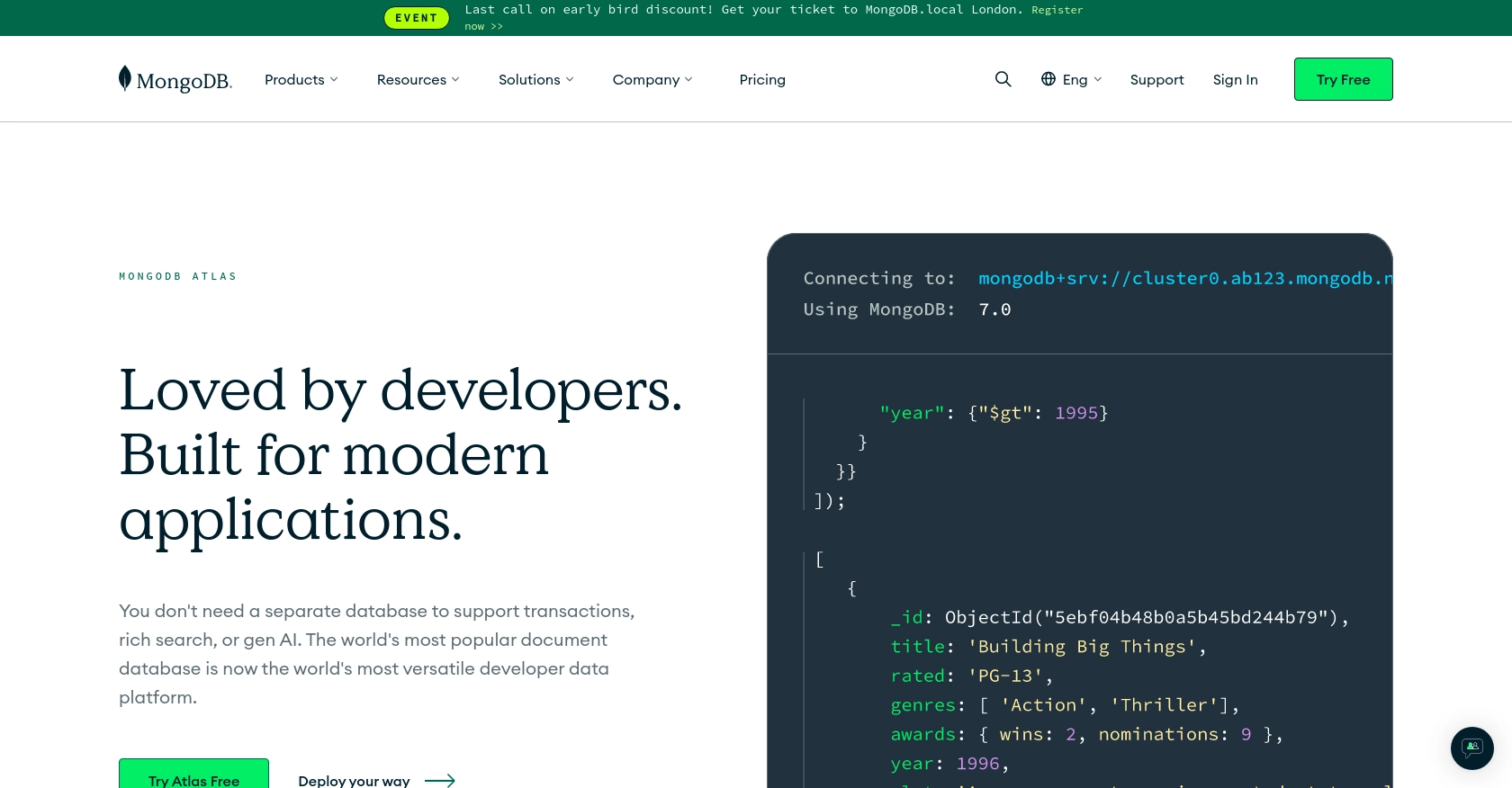
Introduction to MongoDB
MongoDB is a powerful, flexible NoSQL database that allows developers to store and manage data in a document-oriented format. Known for its scalability and performance, MongoDB is widely used in modern applications to handle large volumes of data efficiently.
Integrating with MongoDB's API enables developers to perform CRUD operations seamlessly, making it an ideal choice for applications that require dynamic data handling. For example, a developer might use the MongoDB API to create or update records in a database, facilitating real-time data updates in a web application.
Setting Up Your MongoDB Test or Sandbox Account
Before you can start interacting with the MongoDB API, you'll need to set up a test or sandbox account. MongoDB offers a free tier through MongoDB Atlas, which is a fully managed cloud database service. This allows you to experiment with MongoDB's features without any cost, making it an ideal choice for development and testing purposes.
Creating a MongoDB Atlas Account
To get started, follow these steps to create your MongoDB Atlas account:
- Visit the MongoDB Atlas website and click on the "Sign Up" button.
- Fill in the required information to create your account. You can sign up using your email or through a third-party service like Google or GitHub.
- Once your account is created, log in to the MongoDB Atlas dashboard.
Setting Up a Free Tier Cluster
After logging in, you'll need to set up a cluster to start using MongoDB:
- In the MongoDB Atlas dashboard, click on the "Build a Cluster" button.
- Select the "Free Tier" option to create a free cluster.
- Choose your preferred cloud provider and region. The free tier offers limited options, but you can select the one closest to your location for optimal performance.
- Click "Create Cluster" to initiate the setup. This process may take a few minutes.
Generating API Keys for MongoDB Access
To interact with the MongoDB API, you'll need to generate API keys:
- Navigate to the "Access Manager" section in the MongoDB Atlas dashboard.
- Click on "API Keys" and then "Create API Key."
- Provide a name for your API key and select the appropriate permissions for your use case. For creating or updating records, ensure you have read and write permissions.
- Once created, copy the API key and store it securely. You'll need it to authenticate your API requests.
With your MongoDB Atlas account and API keys set up, you're ready to start integrating with the MongoDB API using Python. In the next section, we'll cover how to make API calls to create or update records in your MongoDB database.
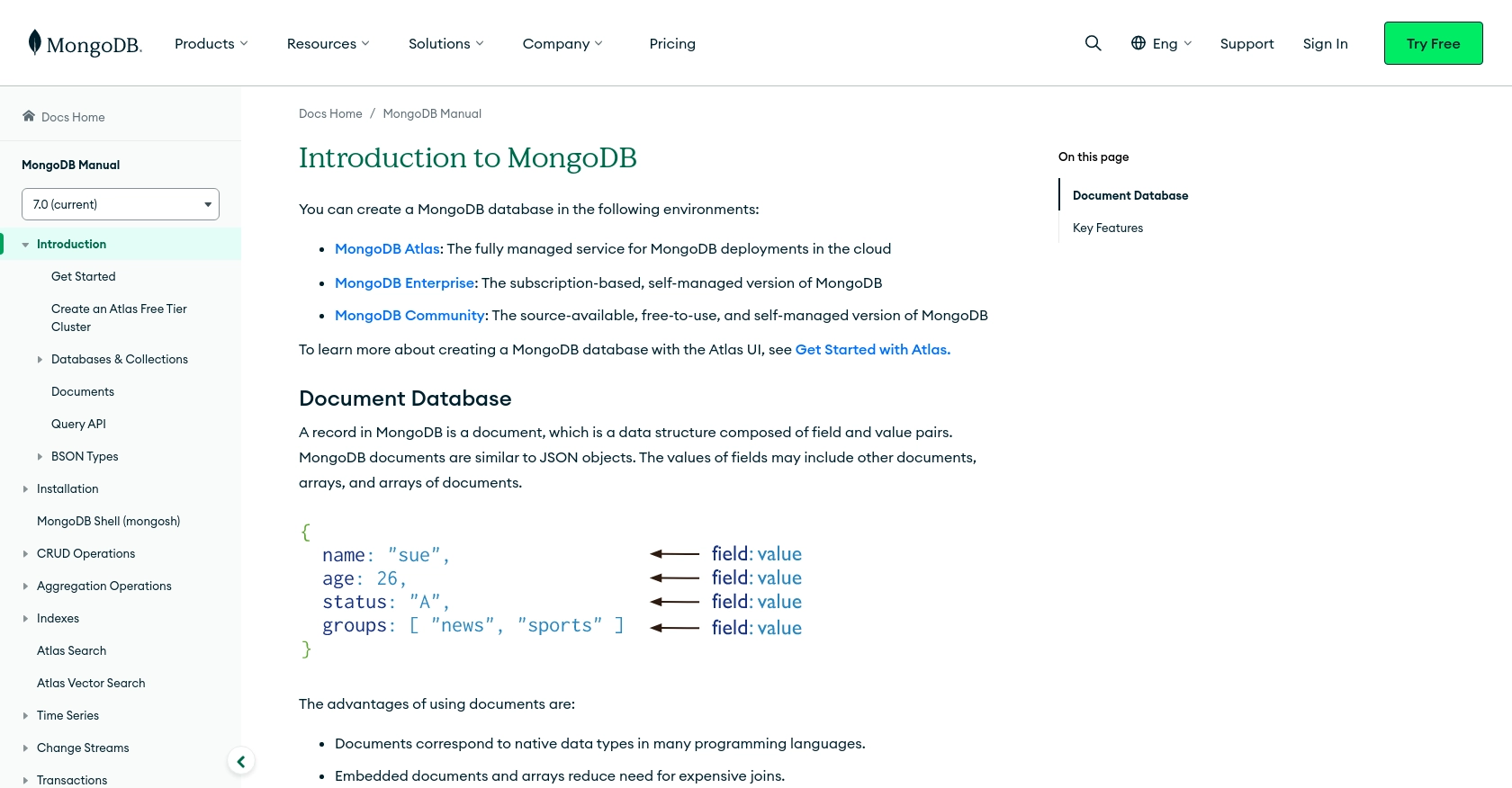
sbb-itb-96038d7
Making API Calls to Create or Update Records in MongoDB Using Python
To interact with MongoDB's API and perform operations such as creating or updating records, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for MongoDB API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the pymongo
library, which provides tools to interact with MongoDB.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Use pip to install the
pymongo
library by running the following command in your terminal:
pip install pymongo
Writing Python Code to Create or Update Records in MongoDB
With your environment set up, you can now write the Python code to interact with MongoDB. The following example demonstrates how to create or update a record in a MongoDB collection.
from pymongo import MongoClient
# Replace 'your_connection_string' with your MongoDB Atlas connection string
client = MongoClient('your_connection_string')
# Access the database and collection
db = client['your_database_name']
collection = db['your_collection_name']
# Define the record to be inserted or updated
record = {
"name": "John Doe",
"email": "johndoe@example.com",
"age": 30
}
# Use the update_one method to update the record if it exists, or insert it if it doesn't
result = collection.update_one(
{"email": "johndoe@example.com"}, # Query to find the record
{"$set": record}, # Update operation
upsert=True # Insert the record if it doesn't exist
)
# Check if the operation was successful
if result.upserted_id:
print("Record inserted with ID:", result.upserted_id)
else:
print("Record updated successfully")
In the code above, replace your_connection_string
, your_database_name
, and your_collection_name
with your actual MongoDB Atlas connection string, database name, and collection name, respectively. The update_one
method is used to update an existing record or insert a new one if it doesn't exist, thanks to the upsert=True
option.
Verifying the Success of Your MongoDB API Request
After running the code, you can verify the success of your operation by checking the MongoDB Atlas dashboard. Navigate to your database and collection to see if the record has been created or updated as expected.
Handling Errors and Understanding MongoDB API Error Codes
When interacting with MongoDB's API, it's essential to handle potential errors gracefully. Common issues include network errors, authentication failures, and incorrect queries. Use try-except blocks in Python to catch exceptions and handle them appropriately.
try:
# Your MongoDB operation code here
except Exception as e:
print("An error occurred:", e)
By implementing error handling, you can ensure that your application remains robust and provides informative feedback when something goes wrong.
Conclusion and Best Practices for MongoDB API Integration in Python
Integrating with the MongoDB API using Python provides a robust solution for managing and manipulating data in a flexible, document-oriented database. By following the steps outlined in this guide, developers can efficiently create or update records in MongoDB, ensuring real-time data handling in their applications.
Best Practices for Secure and Efficient MongoDB API Usage
- Securely Store Credentials: Always store your MongoDB connection strings and API keys securely. Consider using environment variables or a secrets management tool to keep sensitive information safe.
- Handle Rate Limiting: Be mindful of MongoDB's rate limits to avoid service disruptions. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Optimize Data Models: Design your MongoDB data models to take advantage of embedded documents and arrays, reducing the need for expensive joins and improving query performance.
- Implement Error Handling: Use try-except blocks to catch and handle exceptions, providing informative feedback and maintaining application stability.
Streamline Your Integration Process with Endgrate
While integrating with MongoDB's API directly can be powerful, it can also be time-consuming and complex, especially when managing multiple integrations. Endgrate offers a unified API solution that simplifies the integration process, allowing developers to focus on their core product development.
By using Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Save time and resources by outsourcing integrations and ensuring a seamless connection with MongoDB and other platforms.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?