Using the Sugar Market API to Create Or Update Accounts (with PHP examples)
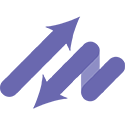
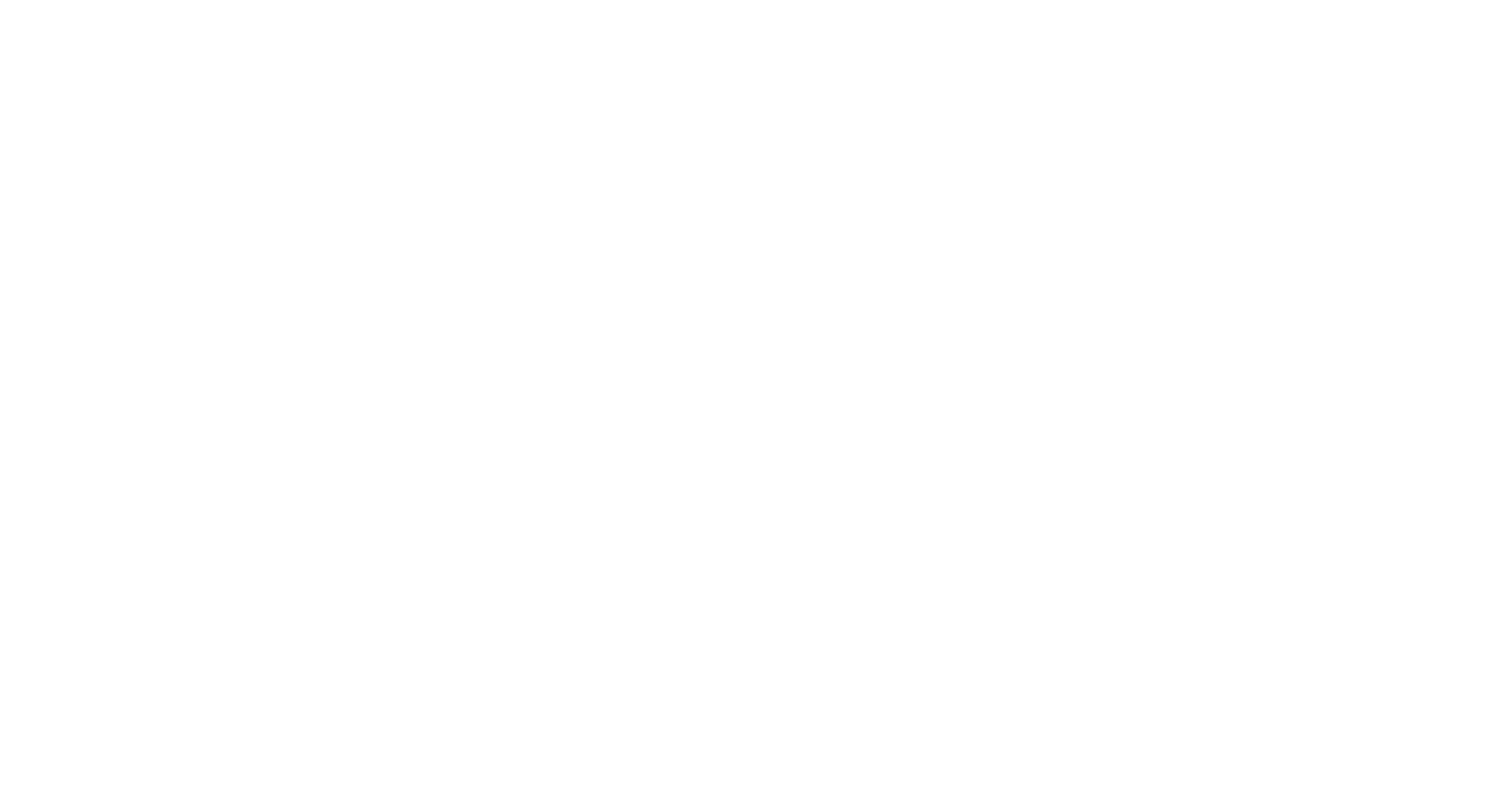
Introduction to Sugar Market API
Sugar Market, a part of the SugarCRM suite, is a powerful marketing automation platform designed to help businesses streamline their marketing efforts. It offers a range of features including email marketing, lead nurturing, and analytics, making it a valuable tool for businesses aiming to enhance their marketing strategies.
Developers may want to integrate with the Sugar Market API to automate and manage marketing tasks, such as creating or updating account information. For example, a developer could use the API to synchronize account data between Sugar Market and other CRM systems, ensuring that marketing efforts are aligned with the most current customer information.
Setting Up Your Sugar Market Test or Sandbox Account
Before you can start integrating with the Sugar Market API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating a Sugar Market Account
If you don't already have a Sugar Market account, you can sign up for a free trial or request access to a sandbox environment through the Sugar Market website. This will provide you with the necessary credentials to access the API.
Generating API Credentials for Sugar Market
Once your account is set up, you'll need to generate API credentials to authenticate your requests. Sugar Market uses a custom authentication method, so follow these steps to obtain your credentials:
- Log in to your Sugar Market account.
- Navigate to the API settings section, which is typically found under the developer or integrations menu.
- Create a new API application. You will be prompted to provide a name and description for your application.
- Once the application is created, you will receive a client ID and client secret. Make sure to store these securely as they will be used to authenticate your API requests.
Configuring OAuth for Sugar Market API Access
Although the Sugar Market API uses custom authentication, you may need to configure OAuth settings if your integration requires it. Follow these steps to set up OAuth:
- In the API settings, locate the OAuth configuration section.
- Enter the necessary redirect URIs and scopes required for your application.
- Save the configuration to apply the changes.
With your test account and API credentials ready, you can now proceed to make API calls to create or update accounts using PHP. For more detailed information on authentication, refer to the Sugar Market API documentation.
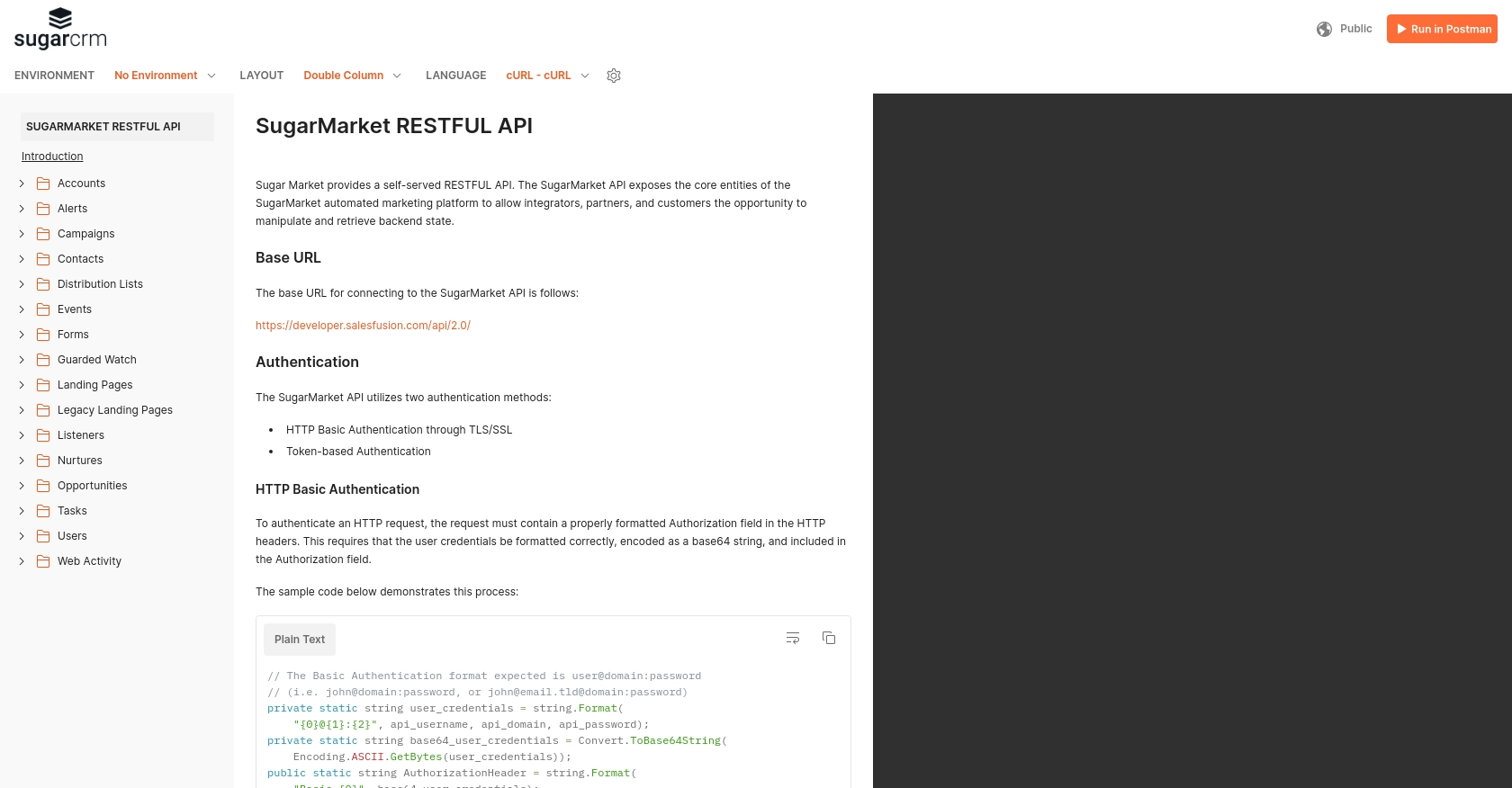
sbb-itb-96038d7
Making API Calls to Create or Update Accounts in Sugar Market Using PHP
To interact with the Sugar Market API for creating or updating accounts, you'll need to use PHP to send HTTP requests. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code, and handling responses.
Setting Up Your PHP Environment for Sugar Market API Integration
Before making API calls, ensure that your PHP environment is properly configured. You'll need PHP 7.4 or later and the cURL extension enabled. You can verify your PHP version and extensions by running the following commands:
php -v
php -m | grep curl
If cURL is not enabled, you can enable it by modifying your php.ini
file and restarting your web server.
Writing PHP Code to Create or Update Accounts in Sugar Market
With your environment ready, you can now write the PHP code to interact with the Sugar Market API. Below is an example of how to create or update an account:
<?php
// Define the API endpoint and credentials
$endpoint = "https://api.sugarmarket.com/v1/accounts";
$clientId = "your_client_id";
$clientSecret = "your_client_secret";
// Prepare the account data
$accountData = [
"name" => "Example Account",
"email" => "example@company.com",
"phone" => "123-456-7890"
];
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Content-Type: application/json",
"Authorization: Bearer " . base64_encode("$clientId:$clientSecret")
]);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($accountData));
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo "cURL error: " . curl_error($ch);
} else {
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
if ($httpCode == 200 || $httpCode == 201) {
echo "Account created or updated successfully.";
} else {
echo "Failed to create or update account. HTTP Code: $httpCode";
}
}
// Close cURL session
curl_close($ch);
?>
Replace your_client_id
and your_client_secret
with your actual API credentials. This script initializes a cURL session, sets the necessary headers, and sends a POST request with the account data.
Verifying API Call Success in Sugar Market
After executing the PHP script, you can verify the success of your API call by checking the Sugar Market dashboard. The account should appear with the details you provided. If the call was unsuccessful, review the HTTP response code and error messages for troubleshooting.
Handling Errors and Error Codes in Sugar Market API
Proper error handling is crucial for robust API integration. The Sugar Market API may return various HTTP status codes to indicate success or failure:
- 200 OK: The request was successful.
- 201 Created: The account was successfully created.
- 400 Bad Request: The request was malformed or invalid.
- 401 Unauthorized: Authentication failed. Check your credentials.
- 500 Internal Server Error: An error occurred on the server.
Refer to the Sugar Market API documentation for more detailed information on error codes and handling strategies.
Best Practices for Sugar Market API Integration
When integrating with the Sugar Market API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some key considerations:
- Secure Storage of Credentials: Always store your client ID and client secret securely. Consider using environment variables or a secure vault to keep these credentials safe.
- Rate Limiting: Be mindful of any rate limits imposed by the Sugar Market API. Implement logic to handle rate limiting gracefully, such as retry mechanisms with exponential backoff.
- Data Transformation and Standardization: Ensure that data fields are consistently transformed and standardized to match the requirements of both Sugar Market and any other systems you are integrating with.
Enhance Your Integration Strategy with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Sugar Market. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations.
- Provide an intuitive integration experience for your customers, enhancing their satisfaction and engagement.
Explore how Endgrate can simplify your integration efforts by visiting Endgrate's website and discover the benefits of a unified integration approach.
Read More
Ready to get started?