How to Create or Update Records with the Odoo Online API in PHP
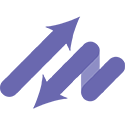
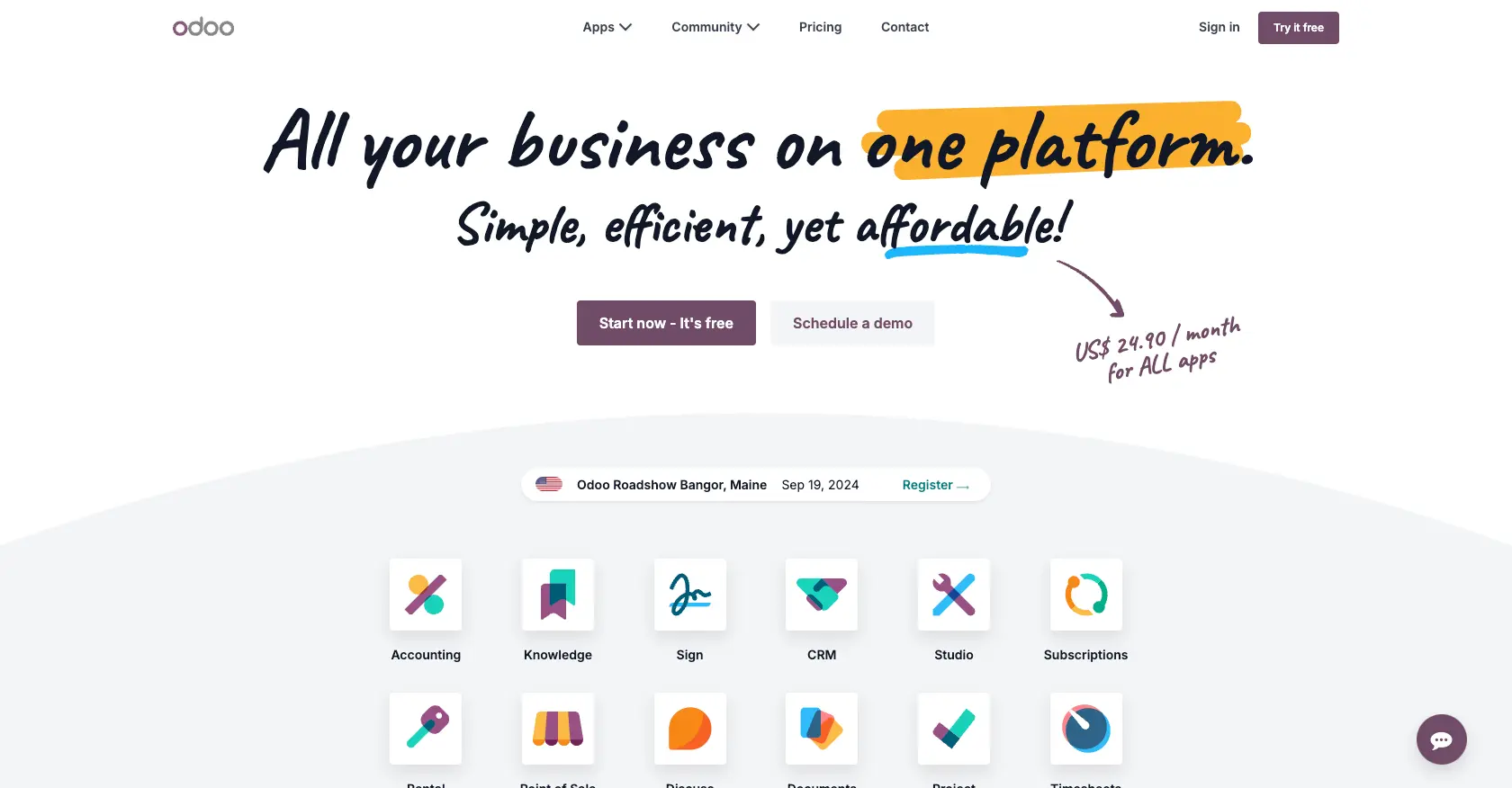
Introduction to Odoo Online API
Odoo Online is a comprehensive suite of business applications that covers a wide range of business needs, including CRM, e-commerce, accounting, inventory, and project management. Known for its flexibility and scalability, Odoo Online is a popular choice for businesses looking to streamline their operations and improve efficiency.
Integrating with the Odoo Online API allows developers to automate and manage business processes more effectively. For example, you might want to create or update customer records directly from your application, ensuring that your data remains consistent and up-to-date across platforms.
Setting Up Your Odoo Online Test/Sandbox Account
Before you can start creating or updating records with the Odoo Online API, you need to set up a test or sandbox account. This allows you to safely experiment with the API without affecting your live data.
Creating an Odoo Online Account
If you don't already have an Odoo Online account, you can sign up for a free trial on the Odoo website. Follow the instructions to create your account, and you'll gain access to a sandbox environment where you can test API interactions.
Generating API Credentials for Odoo Online
Once your account is set up, you'll need to generate API credentials to authenticate your requests. Odoo Online uses a custom authentication method, which involves obtaining a unique API key.
- Log in to your Odoo Online account.
- Navigate to the Settings section.
- Under the Users & Companies menu, select Users.
- Choose the user for whom you want to generate an API key.
- Click on Generate API Key and save the key securely. You'll use this key to authenticate your API requests.
Configuring Your Odoo Online App for API Access
To interact with the Odoo Online API, you may need to configure your app settings to ensure proper permissions:
- In the Settings section, navigate to Technical > API.
- Ensure that the necessary permissions are enabled for creating and updating records.
With your sandbox account and API credentials ready, you can now proceed to make API calls to create or update records in Odoo Online using PHP.
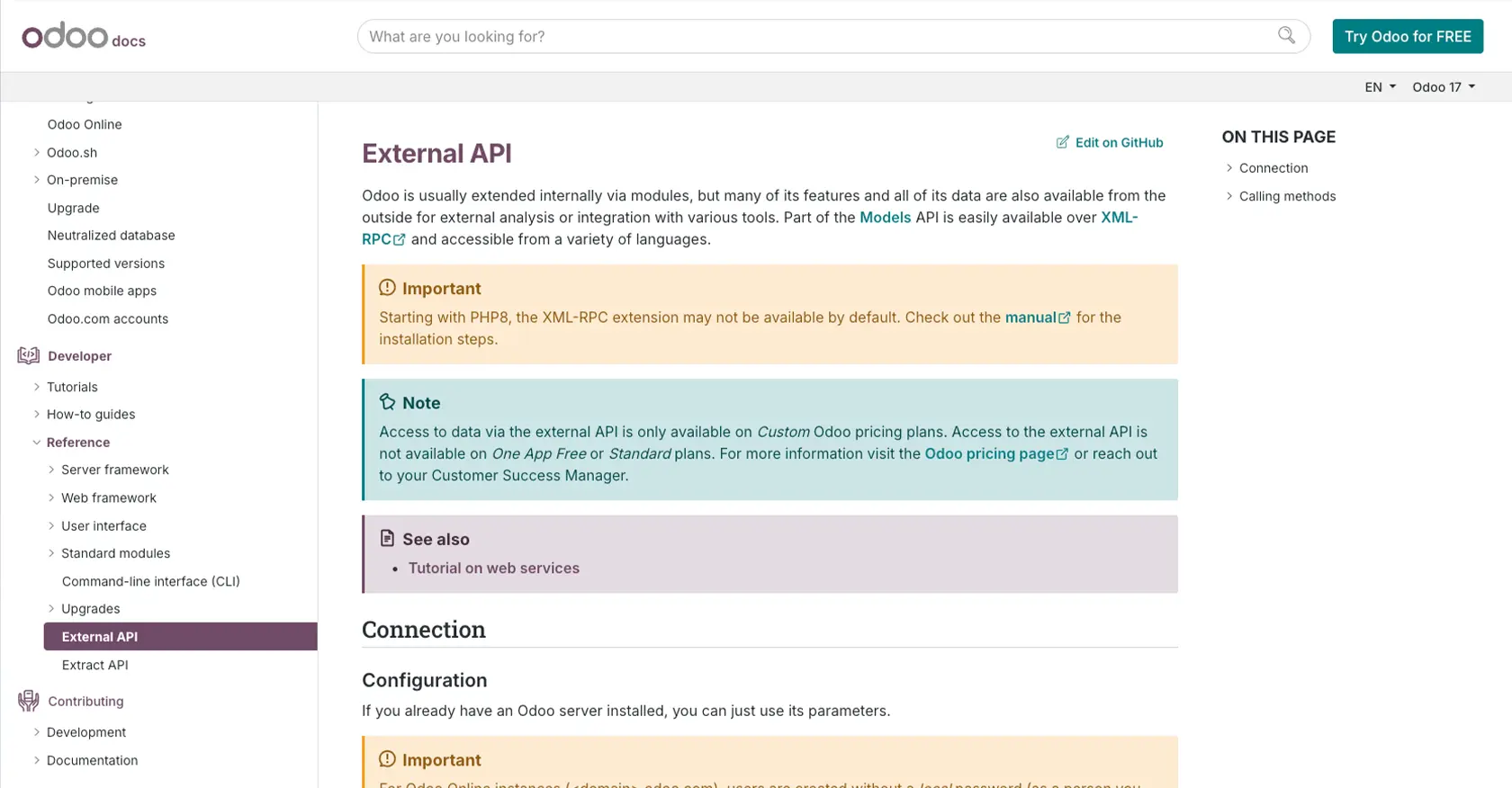
sbb-itb-96038d7
Making API Calls to Create or Update Records in Odoo Online Using PHP
To interact with the Odoo Online API and perform operations such as creating or updating records, you'll need to use PHP. This section will guide you through the process of setting up your PHP environment, making API calls, and handling responses effectively.
Setting Up Your PHP Environment for Odoo Online API
Before making API calls, ensure that your PHP environment is properly configured. You'll need the following:
- PHP 7.4 or later
- cURL extension enabled
To install the cURL extension, you can use the following command:
sudo apt-get install php-curl
Creating or Updating Records with Odoo Online API
Below is an example of how to create or update records using the Odoo Online API with PHP. This example demonstrates how to create a new customer record.
<?php
// Odoo Online API endpoint
$endpoint = "https://your-odoo-instance.odoo.com/jsonrpc";
// API key for authentication
$apiKey = "Your_API_Key";
// Data to create or update a record
$data = [
'jsonrpc' => '2.0',
'method' => 'call',
'params' => [
'service' => 'object',
'method' => 'execute_kw',
'args' => [
'database_name', // Your database name
1, // User ID
$apiKey,
'res.partner', // Model name
'create', // Method to call
[
[
'name' => 'New Customer',
'email' => 'customer@example.com'
]
]
]
],
'id' => 1
];
// Initialize cURL
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json'
]);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
// Execute cURL request
$response = curl_exec($ch);
// Close cURL session
curl_close($ch);
// Decode the response
$responseData = json_decode($response, true);
// Check for errors
if (isset($responseData['error'])) {
echo "Error: " . $responseData['error']['message'];
} else {
echo "Record created successfully with ID: " . $responseData['result'];
}
?>
Replace Your_API_Key
and database_name
with your actual API key and database name. This script initializes a cURL session, sets the necessary headers, and sends a JSON-RPC request to the Odoo Online API to create a new customer record.
Verifying API Call Success in Odoo Online
After executing the script, you can verify the success of your API call by checking the Odoo Online dashboard. Navigate to the relevant module (e.g., Contacts) to see if the new record appears.
Handling Errors and Error Codes in Odoo Online API
It's crucial to handle errors gracefully. The example above checks for errors in the response and outputs an error message if any are found. Refer to the Odoo Online API documentation for a comprehensive list of error codes and their meanings.
Conclusion and Best Practices for Using Odoo Online API with PHP
Integrating with the Odoo Online API using PHP can significantly enhance your application's ability to manage business processes efficiently. By automating tasks such as creating or updating records, you ensure data consistency and streamline operations across platforms.
Best Practices for Secure and Efficient API Integration
- Securely Store API Credentials: Always store your API keys securely, preferably in environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of Odoo Online's rate limits to avoid service disruptions. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that data fields are transformed and standardized to match Odoo Online's requirements, reducing errors and improving data integrity.
- Error Handling: Implement robust error handling to manage API errors effectively. Log errors for monitoring and debugging purposes.
Streamlining Integrations with Endgrate
While integrating with Odoo Online API can be straightforward, managing multiple integrations across different platforms can become complex and time-consuming. Endgrate offers a unified API solution that simplifies this process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Save time and resources by outsourcing integrations and ensuring a seamless connection between your application and Odoo Online.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover the benefits of a streamlined, efficient integration process.
Read More
Ready to get started?