How to Get Policies with the Applied Epic API in Javascript
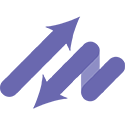
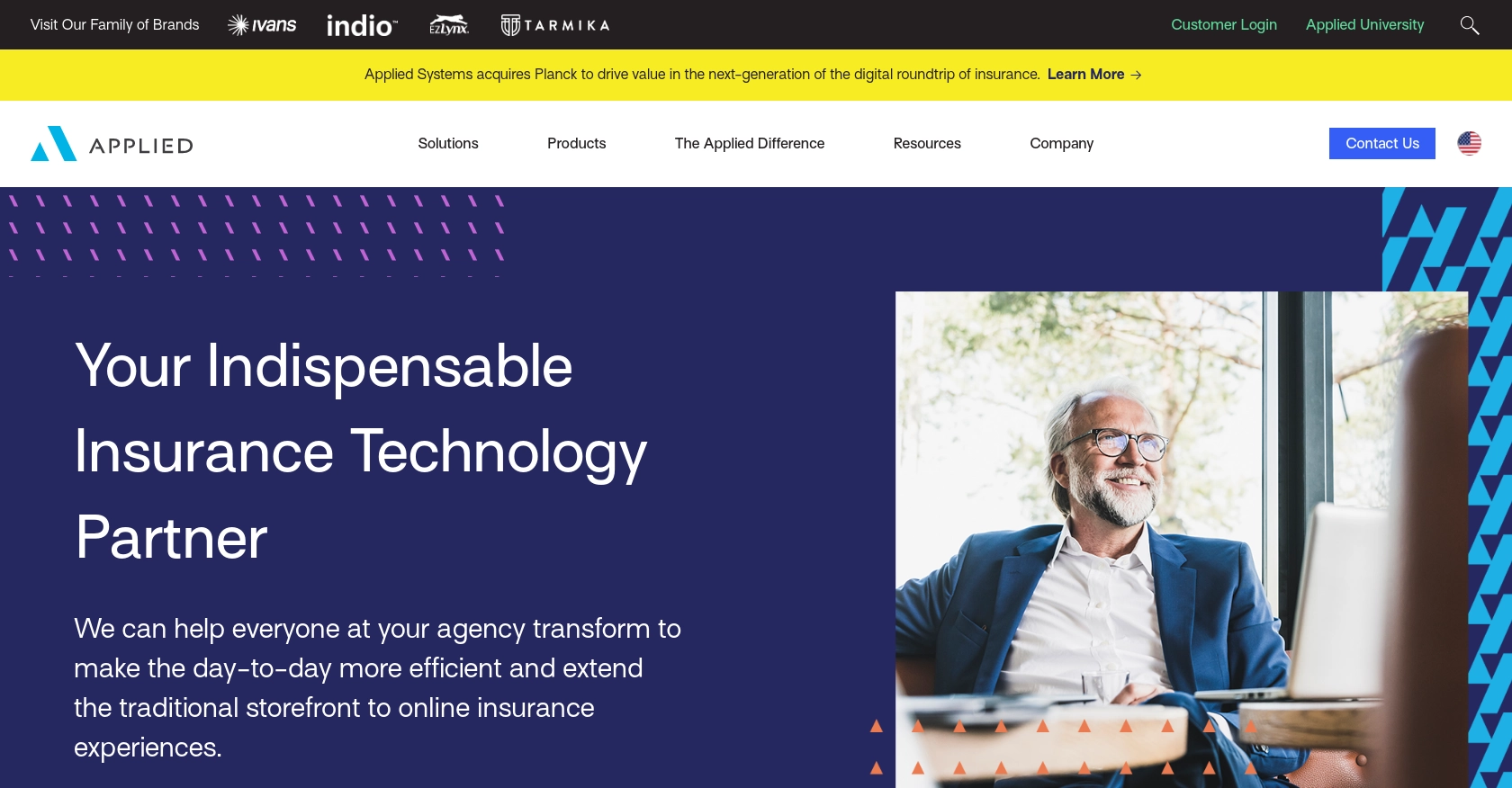
Introduction to Applied Epic API
Applied Epic is a comprehensive insurance management platform designed to streamline operations for insurance agencies and brokerages. It offers a unified solution for managing policies, claims, and customer interactions, making it an essential tool for insurance professionals.
Integrating with the Applied Epic API allows developers to access and manage policy data programmatically. This can be particularly useful for automating tasks such as retrieving policy details for reporting or integrating with other systems. For example, a developer might use the Applied Epic API to fetch policy information for a specific client and display it in a custom dashboard, enhancing the agency's operational efficiency.
Setting Up Your Applied Epic Test Account
Before you can start interacting with the Applied Epic API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating an Applied Epic Developer Account
To begin, you'll need to sign up for an Applied Epic developer account. Visit the Applied Developer Portal and follow the registration instructions. Once registered, you'll have access to the necessary tools and resources to create and manage your applications.
Generating API Credentials for Applied Epic
Applied Epic uses a custom authentication method for API access. To generate the required credentials, follow these steps:
- Log in to your Applied Epic developer account.
- Navigate to the "Applications" section and create a new application.
- Once your application is created, you'll receive a client ID and client secret. Keep these credentials secure as they are essential for authenticating your API requests.
Configuring OAuth for Applied Epic API Access
Applied Epic API requires OAuth for secure access. Here's how to configure it:
- In your application settings, set up the OAuth redirect URI to match your development environment.
- Use the client ID and client secret to request an access token. This token will be used to authenticate your API calls.
For more detailed information, refer to the Applied Epic API documentation.
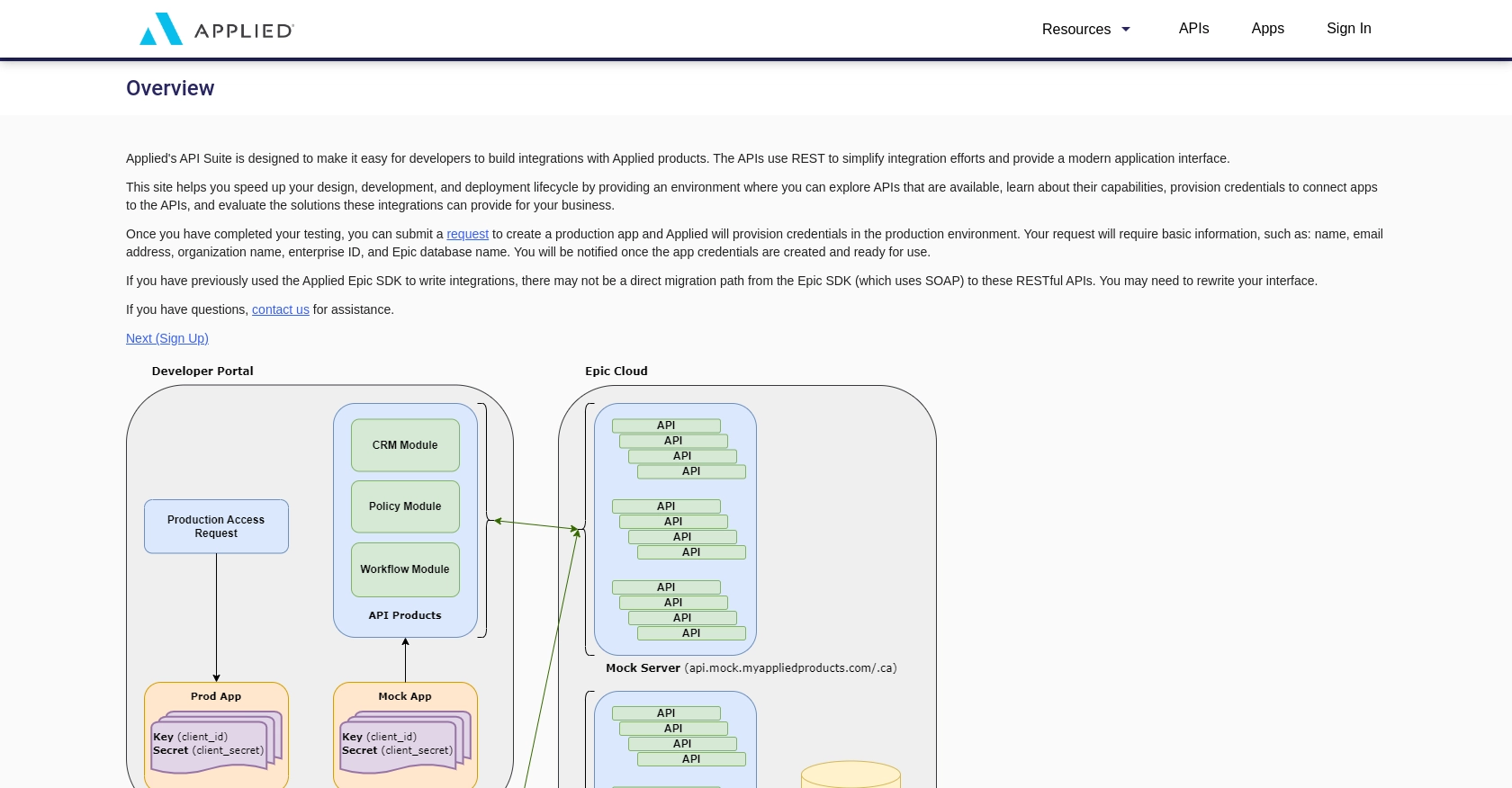
sbb-itb-96038d7
Making API Calls to Retrieve Policies with Applied Epic in JavaScript
To interact with the Applied Epic API and retrieve policy data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your JavaScript Environment for Applied Epic API
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides the runtime environment for executing JavaScript code outside a browser.
You'll also need to install the axios
library, which simplifies making HTTP requests. Run the following command in your terminal to install it:
npm install axios
Writing JavaScript Code to Fetch Policies from Applied Epic API
Once your environment is ready, you can write the JavaScript code to fetch policies. Create a file named getPolicies.js
and add the following code:
const axios = require('axios');
// Replace with your actual client ID and access token
const clientId = 'your_client_id';
const accessToken = 'your_access_token';
// Define the API endpoint
const endpoint = `https://api.myappliedproducts.com/policy/v1/clients/${clientId}/policies`;
// Set up the request headers
const headers = {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
};
// Function to fetch policies
async function fetchPolicies() {
try {
const response = await axios.get(endpoint, { headers });
console.log('Policies:', response.data._embedded.policies);
} catch (error) {
console.error('Error fetching policies:', error.response ? error.response.data : error.message);
}
}
// Call the function
fetchPolicies();
Replace your_client_id
and your_access_token
with your actual client ID and access token obtained from the Applied Epic developer portal.
Understanding the API Response and Handling Errors
When the API call is successful, you'll receive a JSON response containing the policy data. The example code logs the policies to the console. You can further process this data as needed for your application.
In case of errors, the code handles them by logging the error message. Common HTTP status codes to watch for include:
- 400 Bad Request: The request was invalid. Check your parameters.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 403 Forbidden: You don't have permission to access the resource.
- 404 Not Found: The specified resource could not be found.
For more detailed error handling, refer to the Applied Epic API documentation.
Verifying API Call Success in the Applied Epic Sandbox
After running the code, you can verify the success of your API call by checking the policy data in your Applied Epic sandbox account. Ensure the data returned matches the expected policies for the specified client ID.
Best Practices for Using Applied Epic API in JavaScript
When working with the Applied Epic API, it's crucial to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Securely Store Credentials: Always store your client ID and access token securely. Avoid hardcoding them in your source code. Consider using environment variables or a secure vault.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from the API is transformed and standardized according to your application's requirements. This will help maintain consistency across different systems.
Enhance Your Integration Strategy with Endgrate
While integrating with the Applied Epic API can streamline your operations, managing multiple integrations can become complex and time-consuming. This is where Endgrate can make a significant difference.
Endgrate offers a unified API endpoint that simplifies integration with various platforms, including Applied Epic. By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an easy and intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?