Using the BigCommerce API to Get Product Categories in Javascript
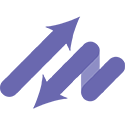
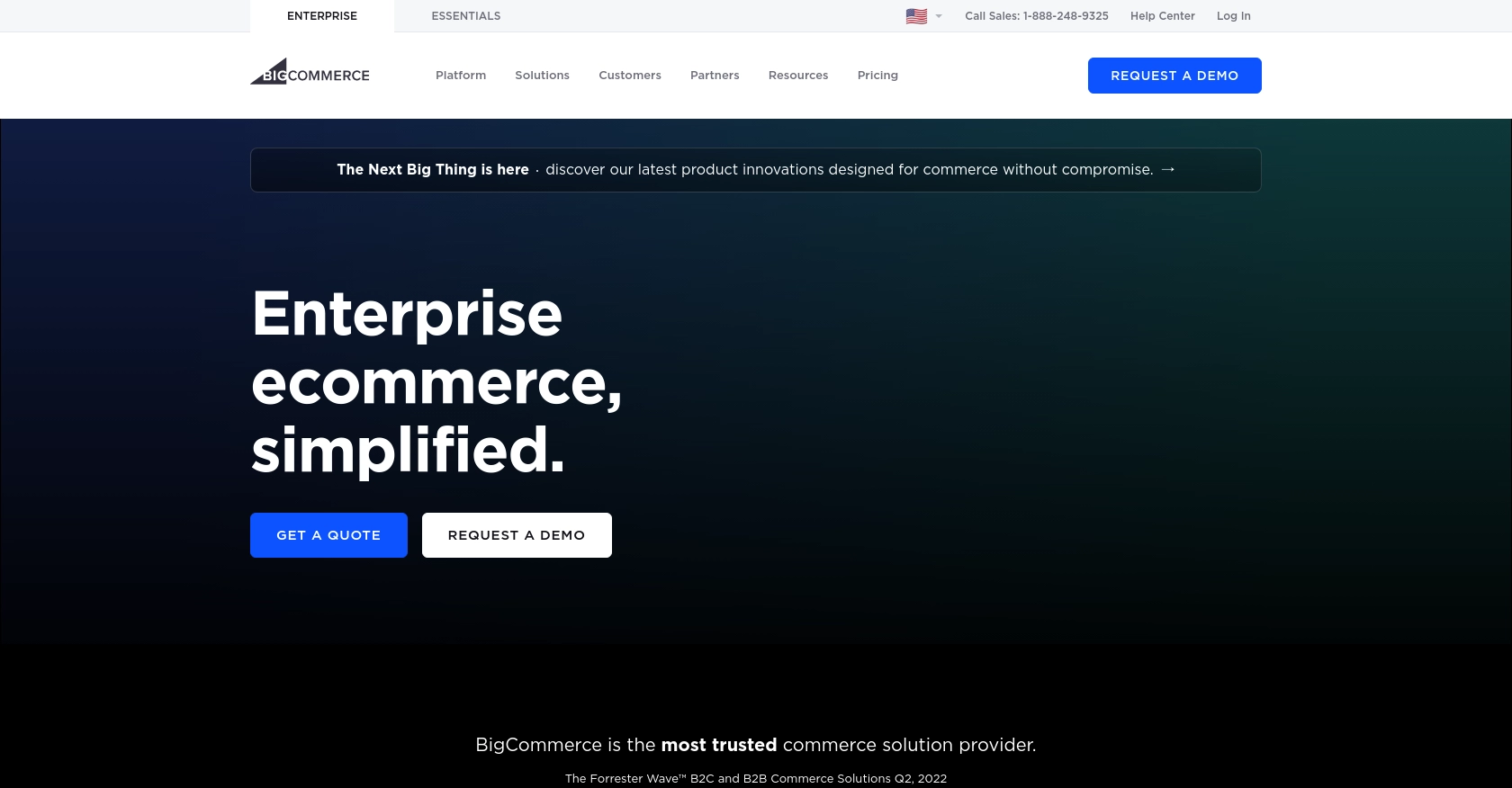
Introduction to BigCommerce API Integration
BigCommerce is a robust e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a wide array of features, including customizable templates, SEO tools, and multi-channel selling capabilities, making it a popular choice for businesses looking to expand their online presence.
For developers, integrating with the BigCommerce API can unlock powerful capabilities to manage store data programmatically. This includes accessing product information, managing orders, and retrieving product categories. For example, a developer might want to use the BigCommerce API to fetch product categories in JavaScript to dynamically display them on a custom storefront or to analyze category data for business insights.
Setting Up a BigCommerce Test or Sandbox Account
Before you can start integrating with the BigCommerce API, it's essential to set up a test or sandbox account. This allows you to experiment with API calls without affecting a live store, ensuring a safe environment for development and testing.
Creating a BigCommerce Developer Account
To begin, you'll need a BigCommerce developer account. Follow these steps to create one:
- Visit the BigCommerce Developer Portal.
- Sign up for a new account or log in if you already have one.
- Once logged in, navigate to the "My Apps" section to create a new app for testing purposes.
Generating API Credentials for BigCommerce
After setting up your developer account, the next step is to generate API credentials. These credentials will allow you to authenticate your API requests:
- In the "My Apps" section, click on "Create a New App."
- Fill in the necessary details, such as app name and description.
- Under "OAuth Scopes," select the permissions your app will need, such as "Products" and "Categories."
- Save your app to generate the client ID and client secret.
Obtaining Access Tokens for BigCommerce API
BigCommerce uses OAuth 2.0 for authentication. You'll need to obtain an access token to make API calls:
- Use the client ID and client secret to request an access token from BigCommerce.
- Send a POST request to the BigCommerce OAuth token endpoint with the required parameters.
- Upon successful authentication, you'll receive an access token that you can use in your API requests.
For more detailed information on authentication, refer to the BigCommerce Authentication Documentation.
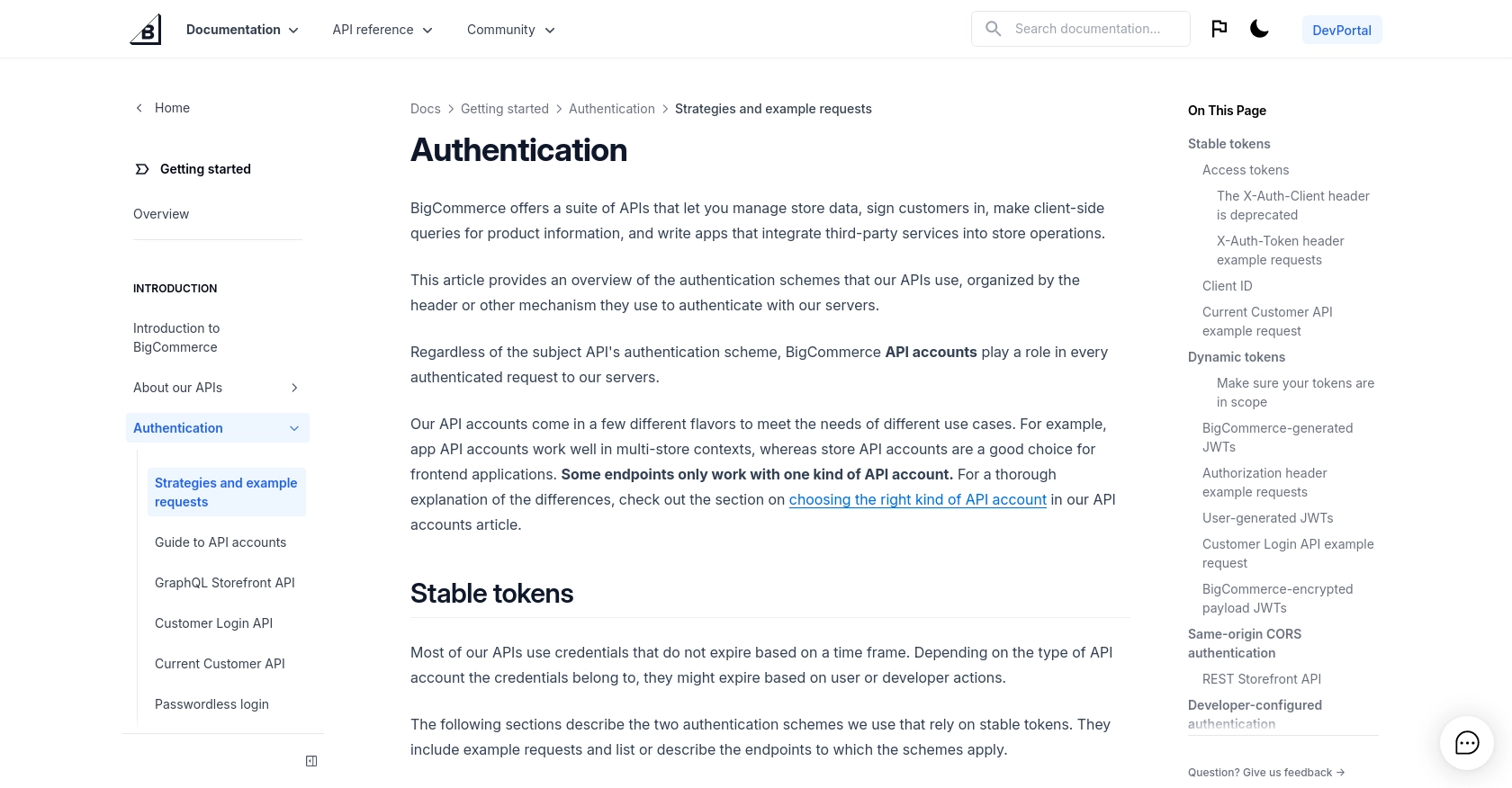
sbb-itb-96038d7
How to Make an API Call to Retrieve Product Categories Using BigCommerce API in JavaScript
Setting Up Your JavaScript Environment for BigCommerce API Integration
To interact with the BigCommerce API using JavaScript, ensure you have a modern JavaScript environment set up. This includes having Node.js installed, which provides the runtime for executing JavaScript code outside a browser.
Additionally, you'll need to install the node-fetch
package to make HTTP requests. You can install it using the following command:
npm install node-fetch
Writing JavaScript Code to Fetch Product Categories from BigCommerce
Once your environment is ready, you can write the JavaScript code to fetch product categories. The following example demonstrates how to make a GET request to the BigCommerce API to retrieve product categories:
const fetch = require('node-fetch');
// Define the API endpoint and headers
const storeHash = 'your_store_hash';
const endpoint = `https://api.bigcommerce.com/stores/${storeHash}/v3/catalog/categories`;
const headers = {
'X-Auth-Token': 'your_access_token',
'Accept': 'application/json'
};
// Function to fetch categories
async function getCategories() {
try {
const response = await fetch(endpoint, { headers });
if (!response.ok) {
throw new Error(`Error: ${response.status}`);
}
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Failed to fetch categories:', error);
}
}
// Call the function
getCategories();
Replace your_store_hash
and your_access_token
with your actual store hash and access token obtained from the BigCommerce developer portal.
Understanding the API Response and Verifying Success
Upon successful execution, the API will return a JSON object containing the list of product categories. You can verify the request's success by checking the response status code and inspecting the returned data.
Here's an example of what the response might look like:
{
"data": [
{
"id": 19,
"parent_id": 0,
"name": "Garden",
"description": "<p>This is the garden description</p>",
"is_visible": true
},
{
"id": 20,
"parent_id": 0,
"name": "Tools",
"description": "<p>This is the tools description</p>",
"is_visible": true
}
],
"meta": {}
}
Handling Errors and BigCommerce API Rate Limits
It's crucial to handle potential errors gracefully. The BigCommerce API may return various error codes, such as 400 for bad requests or 401 for unauthorized access. Always check the response status and handle errors appropriately in your code.
Additionally, be aware of the API rate limits. The default rate limit for the endpoint is 40 concurrent requests. Exceeding this limit may result in throttling, so consider implementing retry logic or request queuing if necessary.
For more details on error codes and rate limits, refer to the BigCommerce API Documentation.
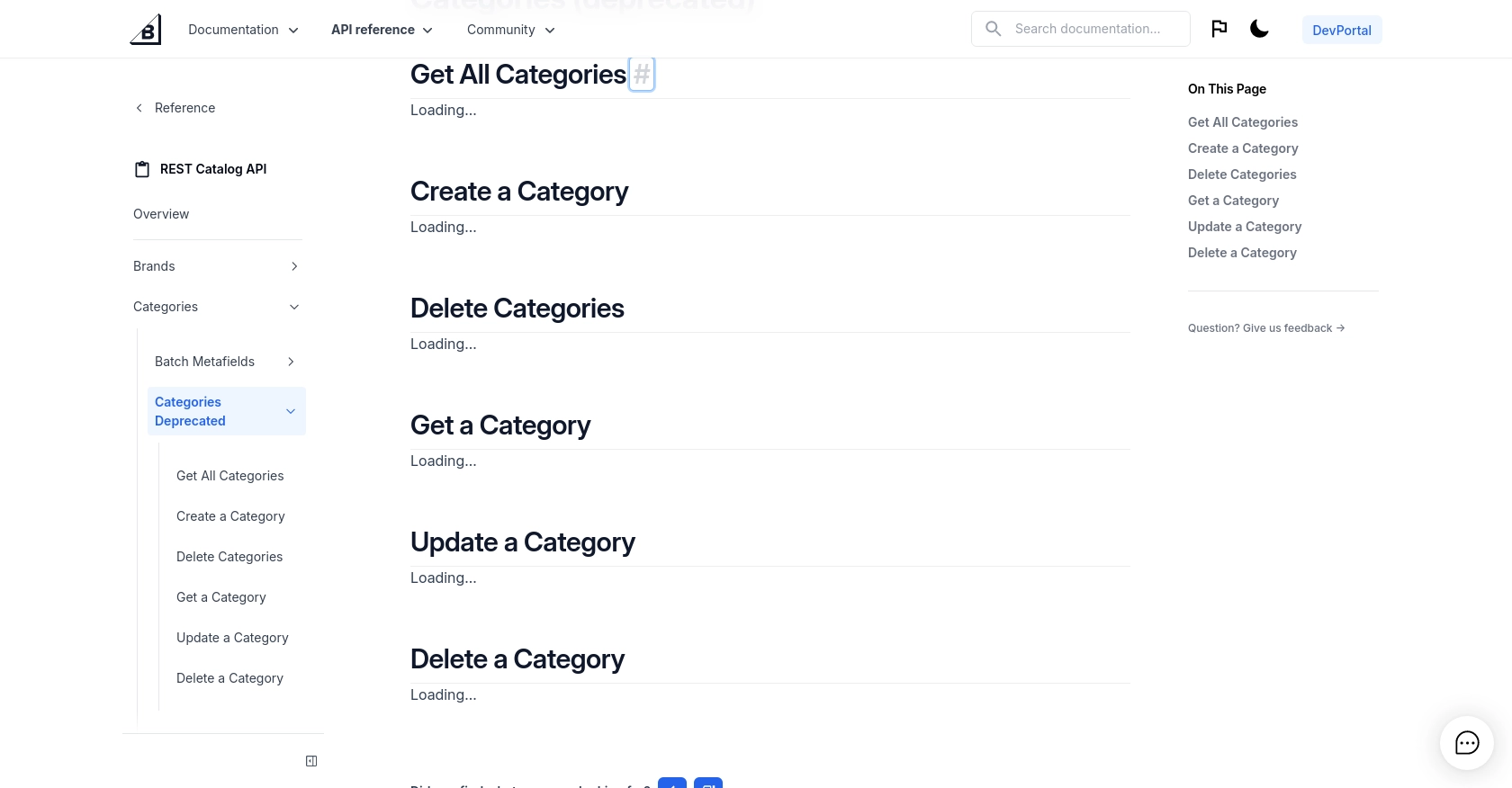
Conclusion and Best Practices for BigCommerce API Integration
Integrating with the BigCommerce API using JavaScript provides developers with the flexibility to manage and manipulate store data programmatically. By following the steps outlined in this guide, you can effectively retrieve product categories and utilize this information to enhance your e-commerce solutions.
Here are some best practices to consider when working with the BigCommerce API:
- Securely Store Credentials: Always store your API credentials, such as access tokens, securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of the API rate limits, which are set at 40 concurrent requests for the categories endpoint. Implement retry logic or request queuing to manage requests efficiently and avoid throttling.
- Standardize Data: When retrieving data from the API, consider transforming and standardizing it to fit your application's requirements. This can help maintain consistency and improve data handling.
- Error Handling: Implement robust error handling to manage potential API errors gracefully. Check response status codes and provide meaningful feedback to users or logs for debugging purposes.
By leveraging Endgrate, you can streamline your integration processes, saving time and resources. Endgrate allows you to build once for each use case, providing an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration needs by visiting Endgrate.
With these practices in mind, you can enhance your e-commerce platform's capabilities and deliver a seamless experience to your users.
Read More
Ready to get started?