Using the Jira API to Get Issues (with Python examples)
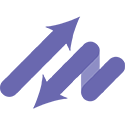
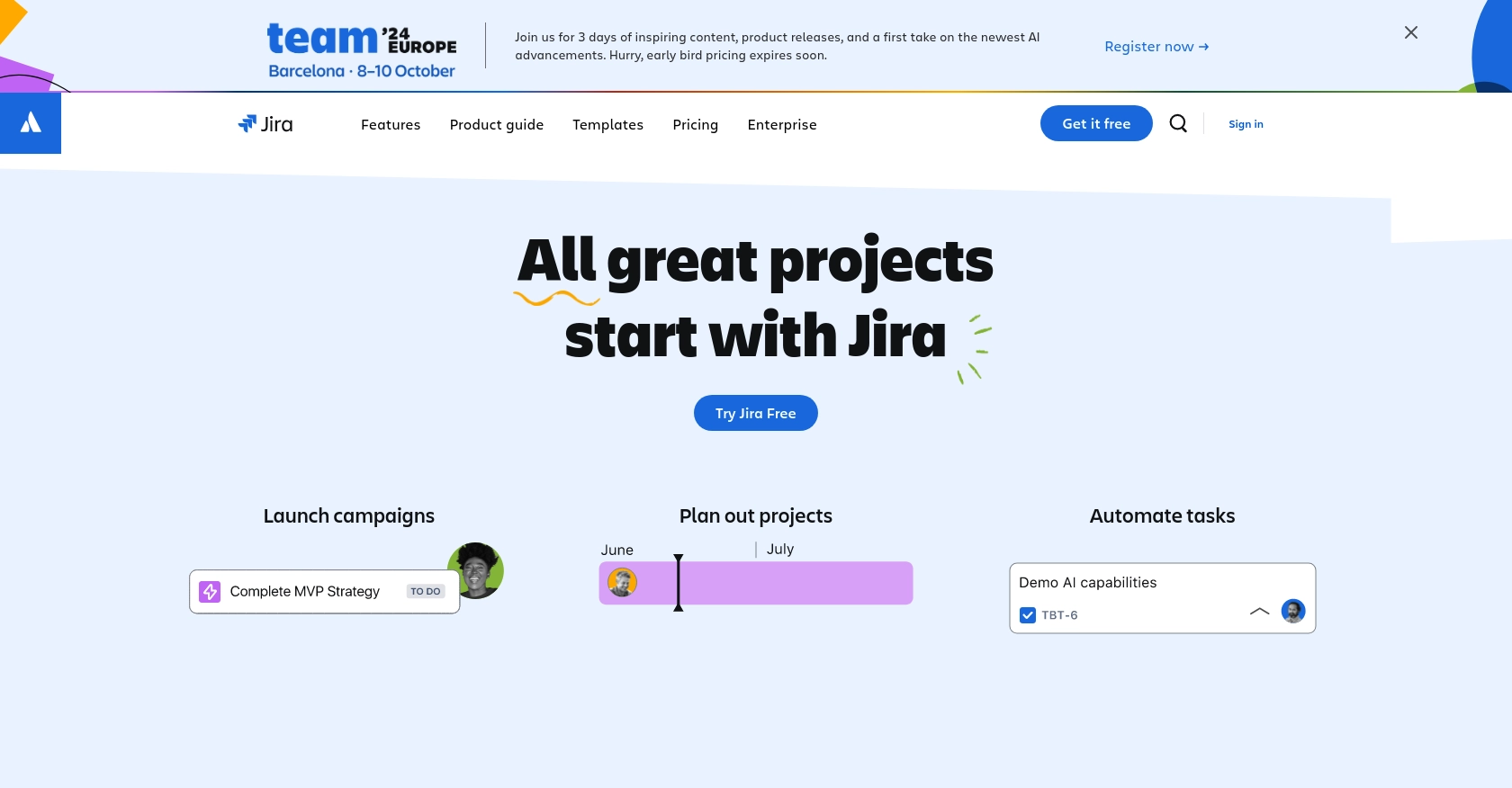
Introduction to Jira Software API
Jira Software is a powerful project management tool developed by Atlassian, widely used by agile teams to plan, track, and manage software development projects. It offers a robust set of features for issue tracking, project management, and collaboration, making it an essential tool for software developers and project managers.
Integrating with the Jira API allows developers to automate and enhance their workflows by accessing Jira's extensive functionalities programmatically. For example, a developer might want to retrieve issues from Jira to generate reports or integrate with other tools for seamless project management.
This article will guide you through using Python to interact with the Jira API, specifically focusing on retrieving issues. By the end of this tutorial, you'll be equipped to efficiently access and manage Jira issues using Python, streamlining your development processes.
Setting Up Your Jira Test/Sandbox Account for API Integration
Before diving into the Jira API, it's essential to set up a test or sandbox account. This environment allows you to experiment with API calls without affecting your live data, ensuring a safe and controlled development process.
Creating a Jira Account
If you don't already have a Jira account, you can sign up for a free trial or a demo account on the Atlassian website. This will give you access to Jira Software Cloud, where you can test API interactions.
- Visit the Jira Software sign-up page.
- Follow the instructions to create your account.
- Once your account is set up, log in to access the Jira dashboard.
Setting Up OAuth 2.0 for Jira API Authentication
Jira uses OAuth 2.0 for secure API authentication. Follow these steps to create an app and obtain the necessary credentials:
- Navigate to the Atlassian Developer Console and log in with your Jira account.
- Click on Create App and fill in the required details, such as the app name and description.
- Under the Authorization section, select OAuth 2.0 (3LO) as the authentication method.
- Define the necessary scopes for your app. For retrieving issues, you will need the
read:issue:jira-software
scope. More information on scopes can be found in the Jira Software scopes documentation. - Once the app is created, you will receive a Client ID and Client Secret. Keep these credentials secure as they are required for API authentication.
Generating an Access Token
With your app set up, you can now generate an access token to authenticate API requests:
- Use the following endpoint to request an access token:
https://api.atlassian.com/oauth/token
. - Include your Client ID and Client Secret in the request, along with the required scopes.
- Upon successful authentication, you will receive an access token, which you can use in your API calls.
For more detailed instructions, refer to the Jira Software REST API documentation.
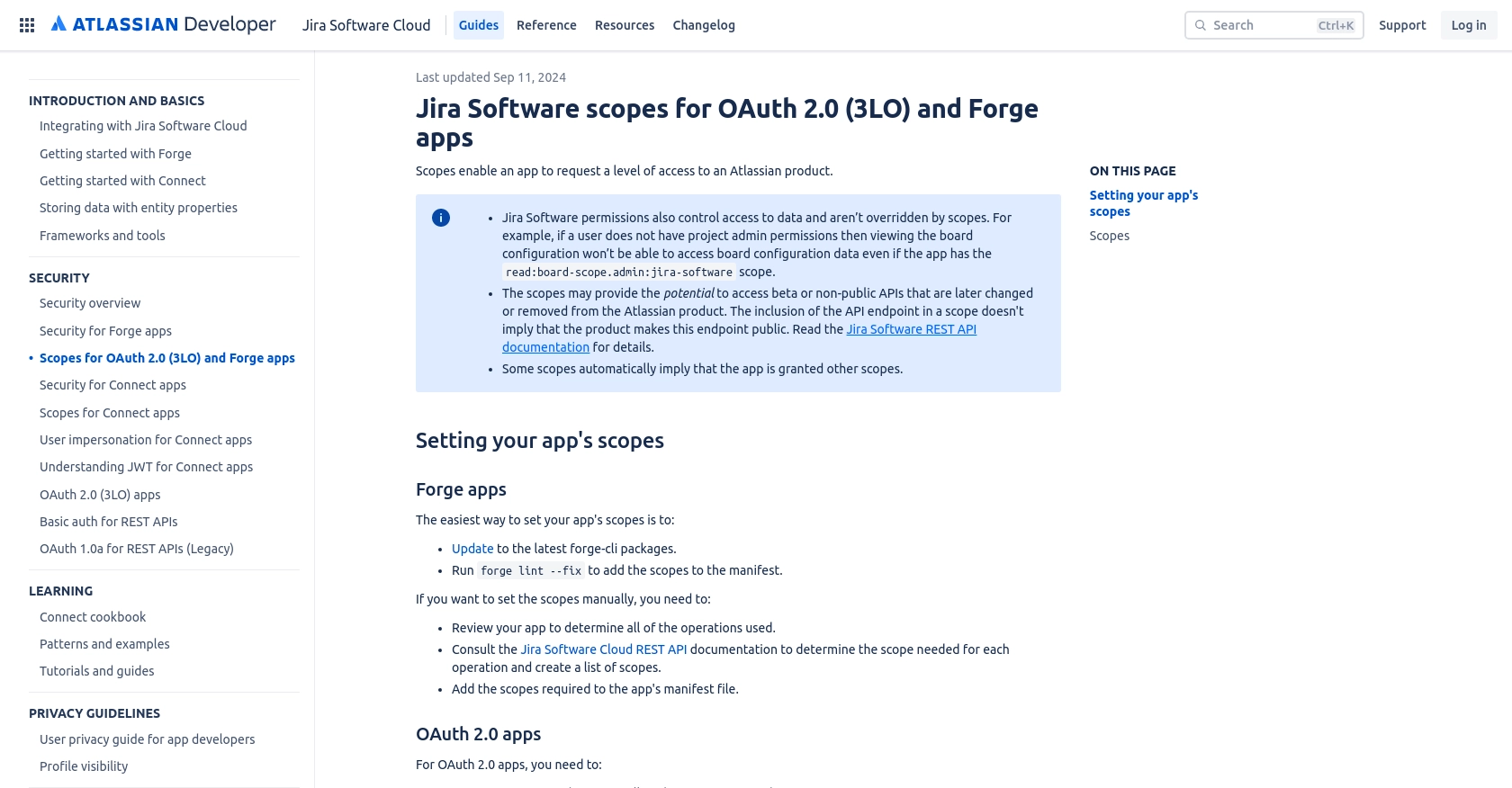
sbb-itb-96038d7
Making API Calls to Retrieve Jira Issues Using Python
Now that your Jira account is set up and authenticated, it's time to make API calls to retrieve issues using Python. This section will guide you through the necessary steps, including setting up your Python environment, writing the code, and handling responses and errors effectively.
Setting Up Your Python Environment for Jira API Integration
Before making API calls, ensure your Python environment is ready. You'll need Python 3.x and the requests
library to handle HTTP requests.
- Ensure Python 3.x is installed on your machine. You can download it from the official Python website.
- Install the
requests
library using pip:
pip install requests
Writing Python Code to Retrieve Jira Issues
With your environment set up, you can now write the Python code to interact with the Jira API and retrieve issues. Follow these steps:
import requests
# Set the API endpoint and headers
issue_id_or_key = "YOUR_ISSUE_ID_OR_KEY"
url = f"https://your-domain.atlassian.net/rest/agile/1.0/issue/{issue_id_or_key}"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Accept": "application/json"
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
issue_data = response.json()
print("Issue Retrieved Successfully:")
print(issue_data)
else:
print(f"Failed to retrieve issue: {response.status_code} - {response.text}")
Replace YOUR_ISSUE_ID_OR_KEY
with the specific issue ID or key you want to retrieve, and YOUR_ACCESS_TOKEN
with the access token obtained during authentication.
Understanding the API Response and Handling Errors
When you run the above code, it makes a GET request to the Jira API to retrieve the specified issue. If successful, the response will contain detailed information about the issue, including fields like description, project, and comments.
It's crucial to handle potential errors gracefully. Common HTTP status codes you might encounter include:
- 200 OK: The request was successful, and the issue data is returned.
- 400 Bad Request: The request was malformed. Check your URL and parameters.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 403 Forbidden: You don't have permission to access the resource.
- 404 Not Found: The specified issue ID or key does not exist.
For more details on error codes, refer to the Jira Software REST API documentation.
Verifying API Call Success in Jira Sandbox
After executing the code, verify the success of your API call by checking the retrieved data against your Jira sandbox. Ensure the issue details match the expected output, confirming that the API interaction is functioning as intended.
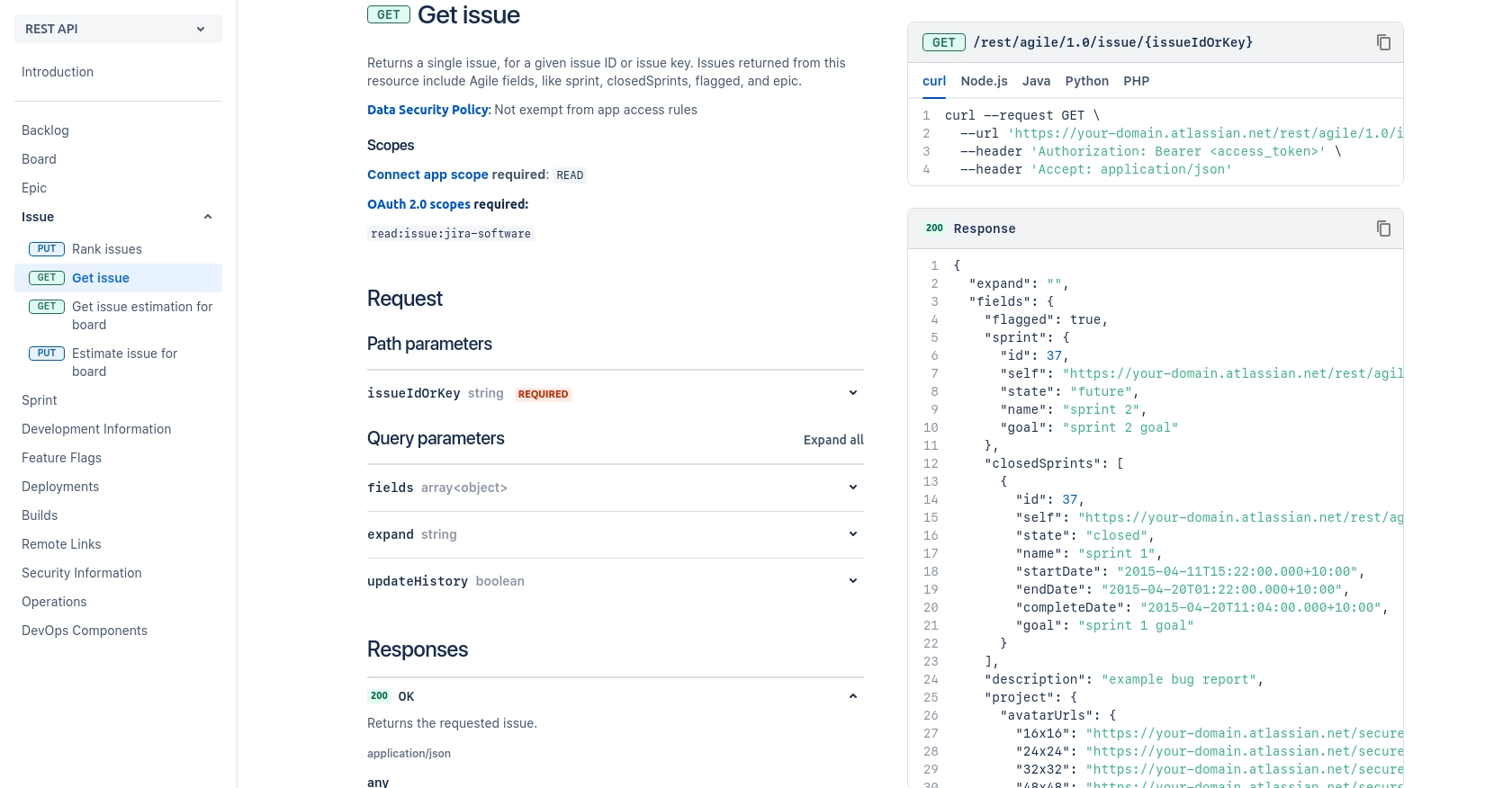
Best Practices for Secure and Efficient Jira API Integration
When integrating with the Jira API, it's essential to follow best practices to ensure security and efficiency. Here are some recommendations:
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Jira's API may impose rate limits to prevent abuse. Monitor your API usage and implement retry logic with exponential backoff to handle rate limit errors gracefully. For more details, refer to the Jira Software REST API documentation.
- Standardize Data Fields: When retrieving or updating issues, ensure that data fields are standardized and consistent with your application's requirements. This will help maintain data integrity across systems.
- Log API Interactions: Maintain logs of API requests and responses for debugging and auditing purposes. This will help you track issues and optimize your integration over time.
Enhance Your Integration Strategy with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Jira. By leveraging Endgrate, you can:
- Save Time and Resources: Focus on your core product development while Endgrate handles the integration complexities.
- Build Once, Deploy Everywhere: Create a single integration for each use case, reducing the need for multiple implementations.
- Deliver a Seamless Experience: Offer your customers an intuitive and efficient integration experience.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/jira
- https://developer.atlassian.com/cloud/jira/software/scopes-for-oauth-2-3LO-and-forge-apps/
- https://developer.atlassian.com/cloud/jira/software/rest/intro/#introduction
- https://developer.atlassian.com/cloud/jira/software/rest/api-group-issue/#api-rest-agile-1-0-issue-issueidorkey-get
Ready to get started?