How to Get Notes with the Intercom API in PHP
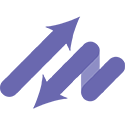
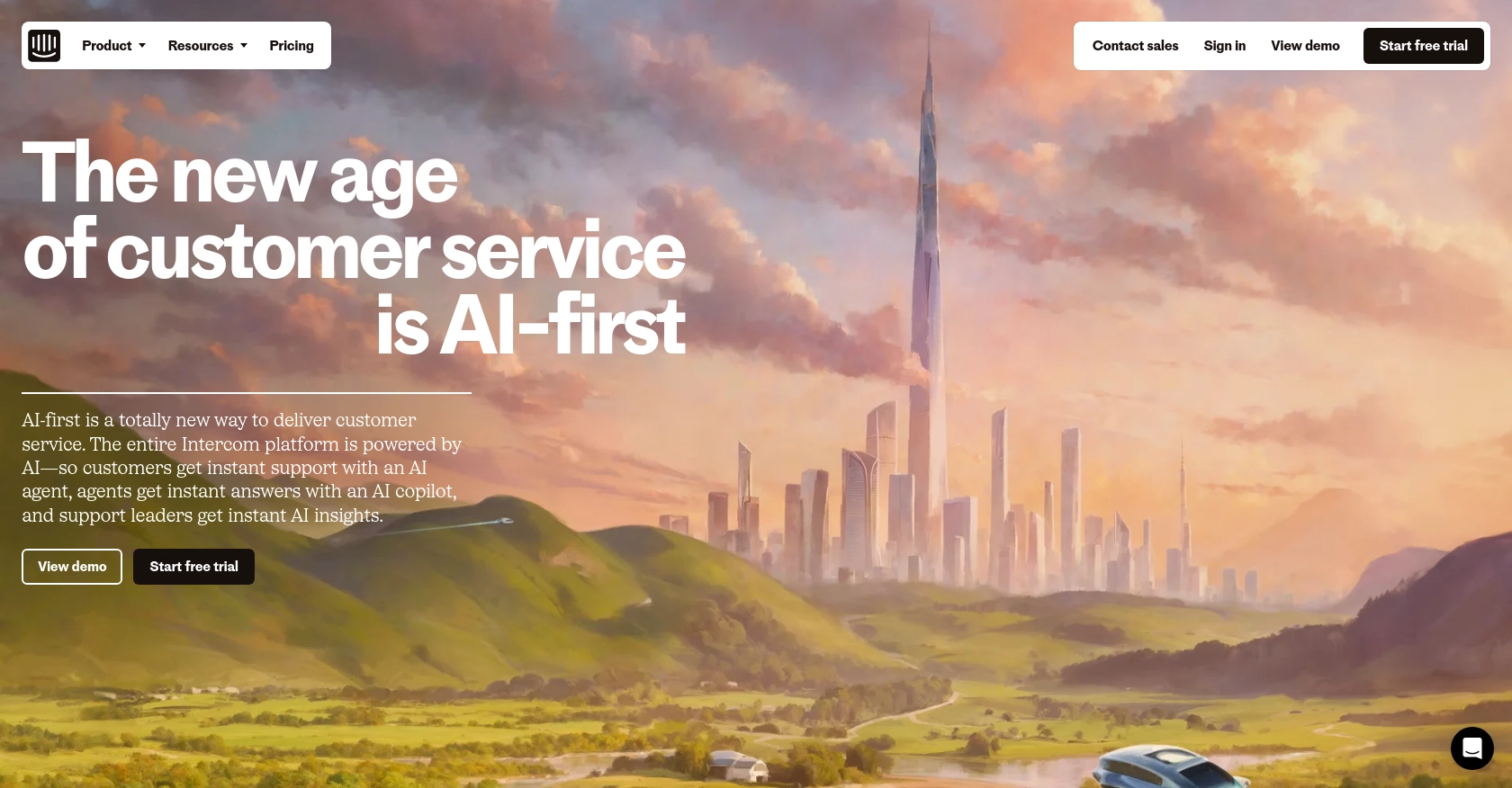
Introduction to Intercom API Integration
Intercom is a powerful customer communication platform that enables businesses to engage with their customers through messaging, email, and chat. It offers a suite of tools designed to enhance customer support, marketing, and engagement efforts, making it a popular choice for businesses looking to improve their customer interactions.
Developers may want to integrate with the Intercom API to access and manage customer data, such as notes, which can be used to annotate and comment on customer interactions. For example, a developer might use the Intercom API to retrieve notes associated with a contact, allowing for better tracking of customer interactions and providing personalized support.
This article will guide you through the process of using PHP to interact with the Intercom API, specifically focusing on retrieving notes. By following this tutorial, you will learn how to efficiently access and manage notes within the Intercom platform using PHP.
Setting Up Your Intercom Test Account for API Integration
Before you can start interacting with the Intercom API using PHP, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Intercom provides a development workspace specifically for this purpose.
Creating a Development Workspace in Intercom
To begin, you'll need to create a development workspace in Intercom. This workspace will give you access to the Developer Hub, where you can configure your app and set up OAuth authentication.
- Visit the Intercom Developer Hub and sign up for a free account if you haven't already.
- Once logged in, navigate to the Developer Hub and create a new app. This app will be used to interact with the Intercom API.
- Ensure that your app is associated with your main Intercom workspace from the dropdown menu.
Configuring OAuth for Intercom API Access
Since the Intercom API uses OAuth for authentication, you'll need to configure OAuth settings for your app. This involves setting up redirect URLs and obtaining client credentials.
- In the Developer Hub, go to the Authentication page and enable the "Use OAuth" option.
- Provide the necessary redirect URLs. These URLs must use HTTPS and will be used to receive the authorization code after user consent.
- Specify the permissions your app requires. Only select the scopes necessary for your use case to ensure a smooth review process.
- Save your client ID and client secret, which will be used to authenticate API requests.
For more detailed instructions, refer to the Intercom OAuth setup guide.
Generating an Access Token for API Requests
With OAuth configured, you can now generate an access token to authenticate your API requests. Follow these steps:
- Redirect users to the Intercom authorization URL, including your client ID and a state parameter for security.
- After user approval, Intercom will redirect to your specified URL with an authorization code.
- Exchange this code for an access token by making a POST request to the Intercom token endpoint.
Once you have the access token, you can use it to authenticate your API calls and start retrieving notes from Intercom.
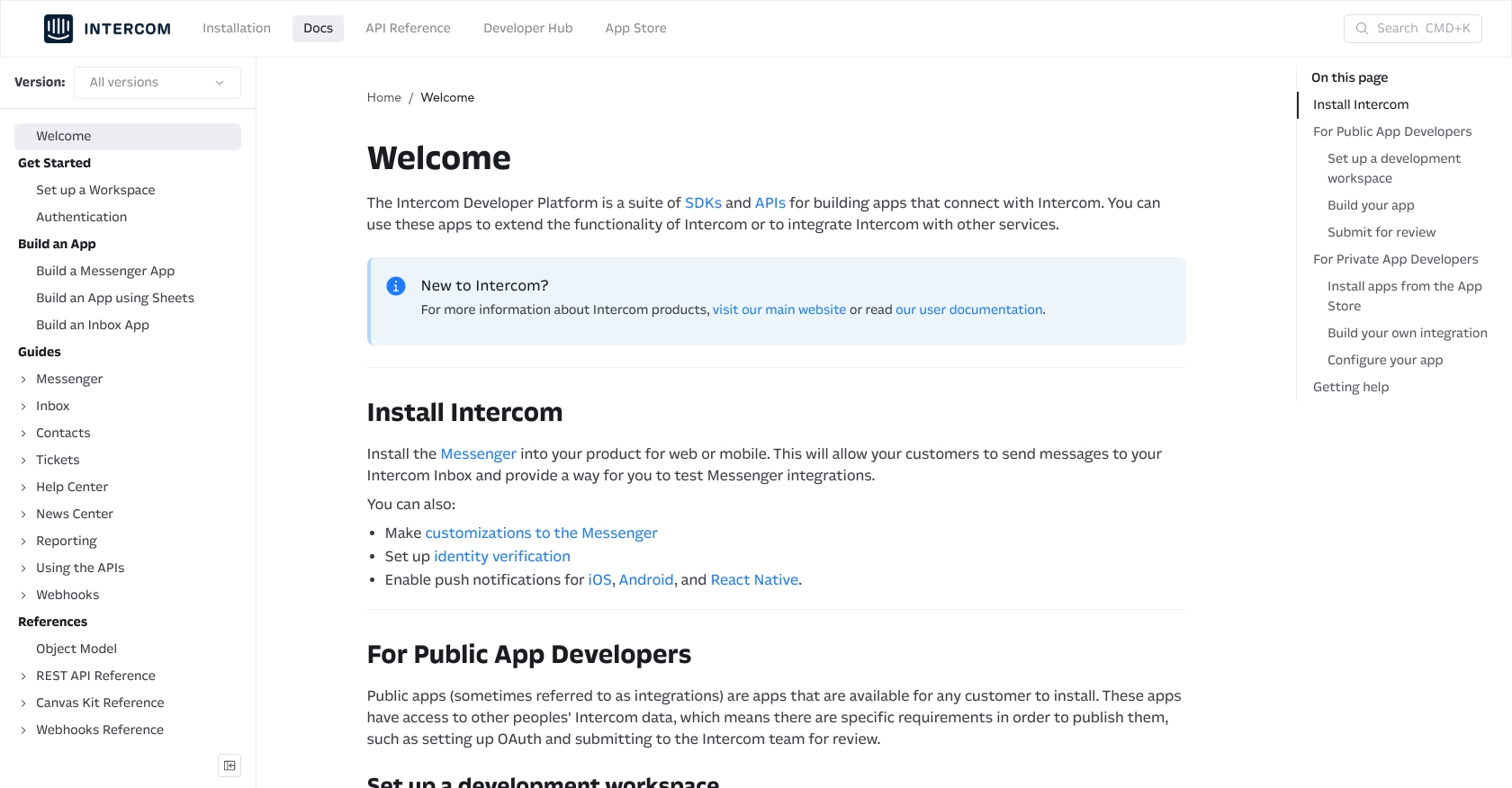
sbb-itb-96038d7
Making API Calls to Retrieve Notes with Intercom API in PHP
To interact with the Intercom API and retrieve notes using PHP, you need to ensure your development environment is properly set up. This section will guide you through the necessary steps, including setting up PHP, installing required dependencies, and executing the API call to fetch notes.
Setting Up PHP Environment for Intercom API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or later
- Composer, the PHP package manager
Once you have these installed, use Composer to install the Guzzle HTTP client, which will help you make HTTP requests:
composer require guzzlehttp/guzzle
Executing the API Call to Retrieve Notes from Intercom
With your environment ready, you can now write the PHP code to make an API call to Intercom and retrieve notes associated with a contact. Follow the steps below:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'YOUR_ACCESS_TOKEN';
$contactId = 'CONTACT_ID';
$response = $client->request('GET', "https://api.intercom.io/contacts/{$contactId}/notes", [
'headers' => [
'Authorization' => "Bearer {$accessToken}",
'Intercom-Version' => '2.11',
'Accept' => 'application/json',
],
]);
$notes = json_decode($response->getBody(), true);
foreach ($notes['data'] as $note) {
echo "Note ID: " . $note['id'] . "\n";
echo "Created At: " . date('Y-m-d H:i:s', $note['created_at']) . "\n";
echo "Body: " . strip_tags($note['body']) . "\n\n";
}
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth setup, and CONTACT_ID
with the ID of the contact whose notes you wish to retrieve.
Verifying Successful API Requests and Handling Errors
After running the script, you should see the notes printed in your terminal. To verify the request's success, check the response status code:
if ($response->getStatusCode() === 200) {
echo "Notes retrieved successfully.";
} else {
echo "Failed to retrieve notes. Status code: " . $response->getStatusCode();
}
Handle errors by checking the response status code and implementing appropriate error messages. Refer to the Intercom API documentation for more details on error codes and handling.
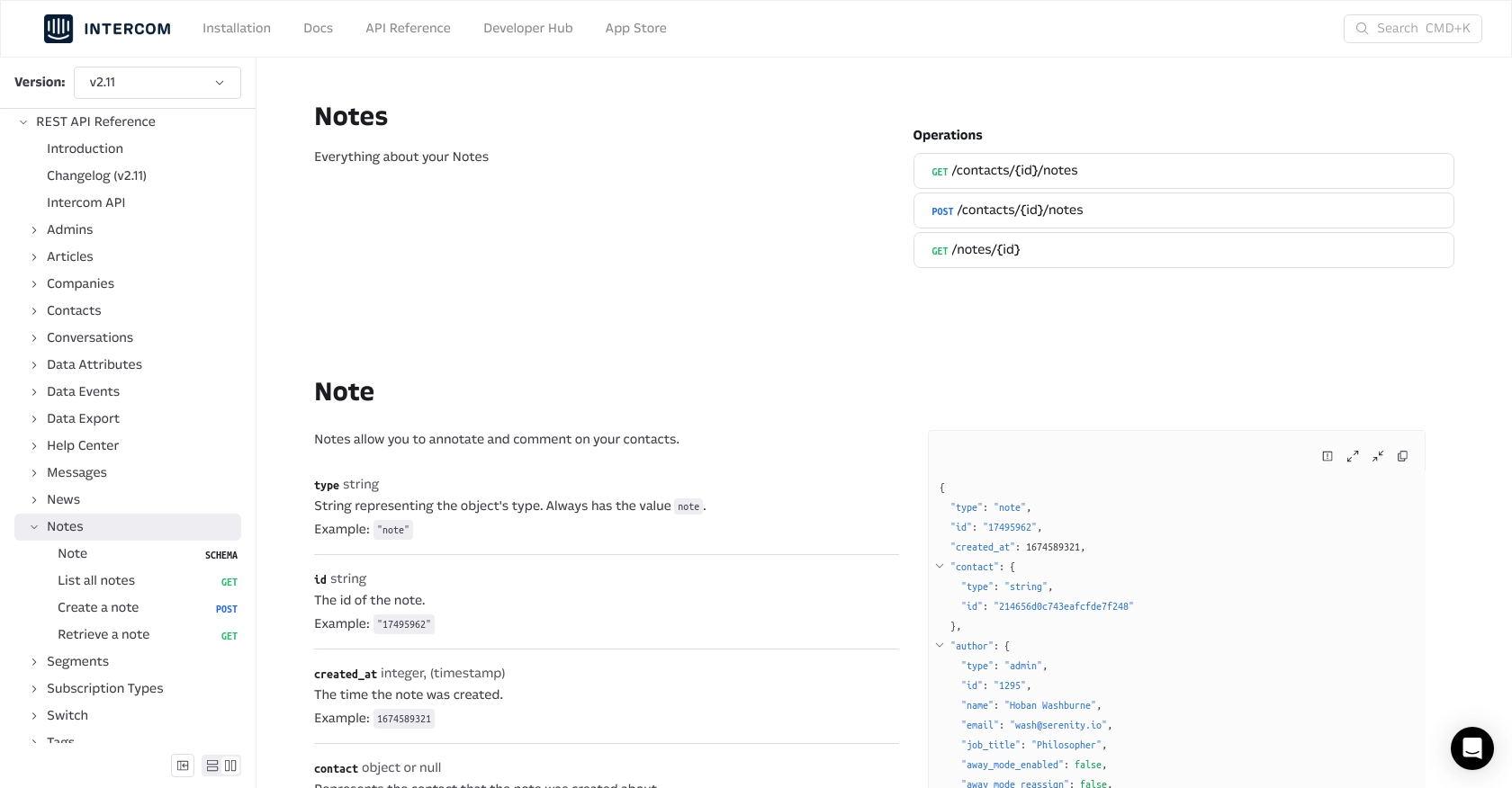
Conclusion and Best Practices for Intercom API Integration in PHP
Integrating with the Intercom API using PHP allows developers to efficiently manage customer interactions by retrieving and handling notes. This integration can enhance customer support and engagement by providing valuable insights into customer interactions.
Best Practices for Secure and Efficient Intercom API Integration
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be aware of Intercom's rate limits to avoid exceeding them. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data retrieved from Intercom is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage API errors and exceptions. Log errors for monitoring and debugging purposes.
Streamlining Integration with Endgrate
While integrating with the Intercom API can be straightforward, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including Intercom. By using Endgrate, developers can save time and resources, focusing on core product development while ensuring a seamless integration experience for their customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover the benefits of a streamlined integration process.
Read More
Ready to get started?