Using the Slack API to Send Messages (User DM) (with Javascript examples)
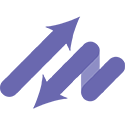
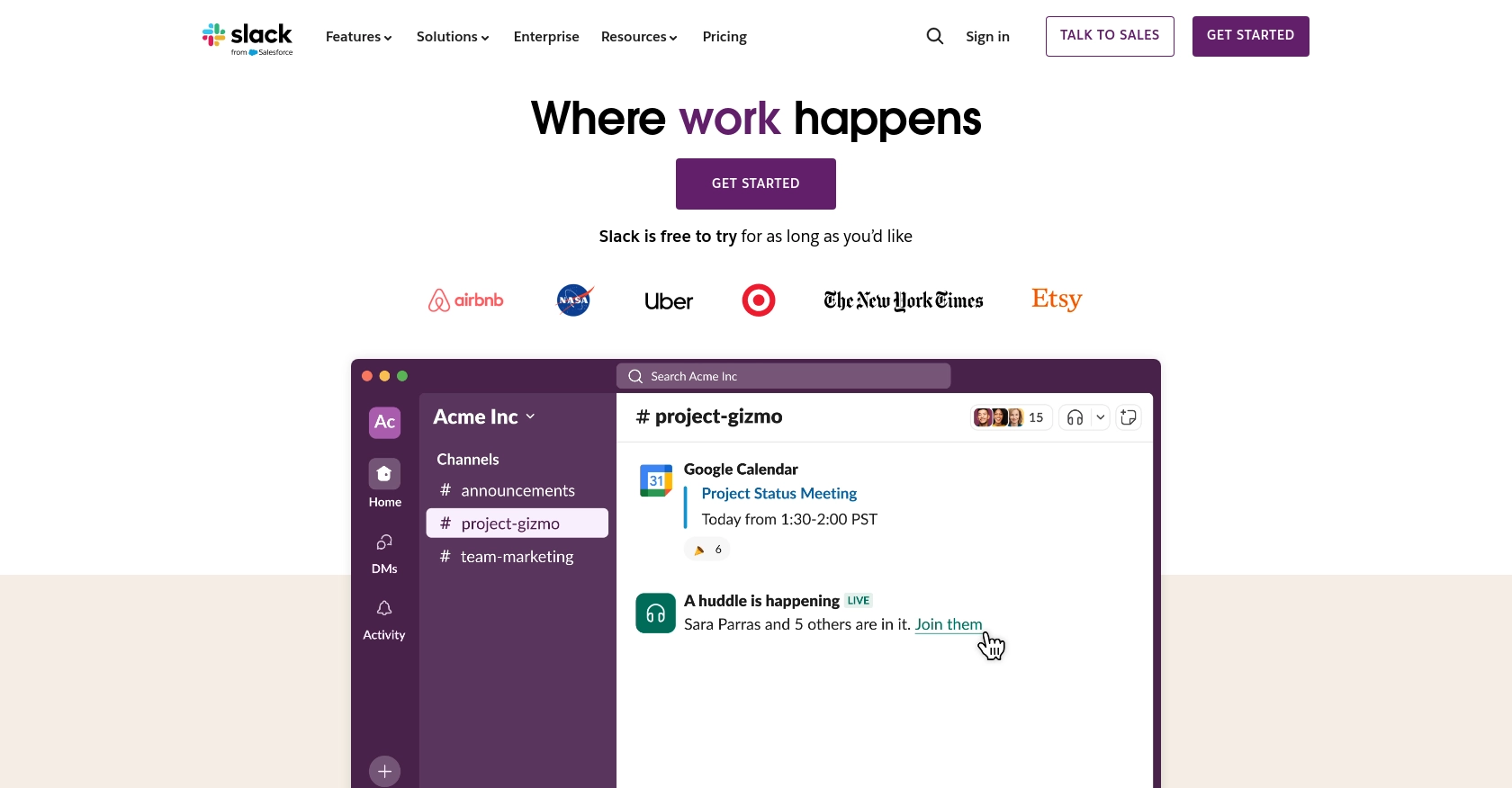
Introduction to Slack API Integration
Slack is a powerful collaboration platform that enables teams to communicate and work together efficiently. With features like channels, direct messaging, and integrations with various tools, Slack has become an essential part of many organizations' workflows.
Developers often integrate with Slack's API to enhance communication capabilities within their applications. One common use case is sending direct messages to users, which can be useful for notifications, alerts, or personalized interactions. By leveraging the Slack API, developers can automate these processes and create a more seamless user experience.
This article will guide you through using JavaScript to send user direct messages via the Slack API, providing step-by-step instructions and code examples to help you get started quickly.
Setting Up Your Slack Developer Account and App
Before you can start sending direct messages using the Slack API, you'll need to set up a Slack developer account and create an app. This process involves configuring OAuth authentication, which is essential for secure API access.
Creating a Slack Developer Account
If you don't already have a Slack account, you'll need to create one. Visit the Slack website and follow the instructions to sign up. Once you have an account, you can proceed to the Slack API portal.
Creating a Slack App for API Access
- Navigate to the Slack Apps page.
- Click on Create New App and select From scratch.
- Enter your app's name and select the workspace where you'll be developing your app. Click Create App.
Configuring OAuth and Permissions for Slack API
To interact with the Slack API, you'll need to configure OAuth permissions:
- Go to the OAuth & Permissions section in your app's settings.
- Scroll down to Scopes and add the following scopes under Bot Token Scopes:
chat:write
- Allows your app to send messages.im:write
- Allows your app to send direct messages.
- Click Save Changes.
Installing and Authorizing Your Slack App
- Return to the Basic Information section of your app's settings.
- Click Install App to Workspace and follow the authorization flow.
- Once installed, you'll receive an OAuth access token. Store this token securely as it will be used to authenticate your API requests.
With your Slack app set up and authorized, you're ready to start sending direct messages using the Slack API and JavaScript. For more detailed information, refer to the Slack Quickstart Guide and Slack Web API documentation.
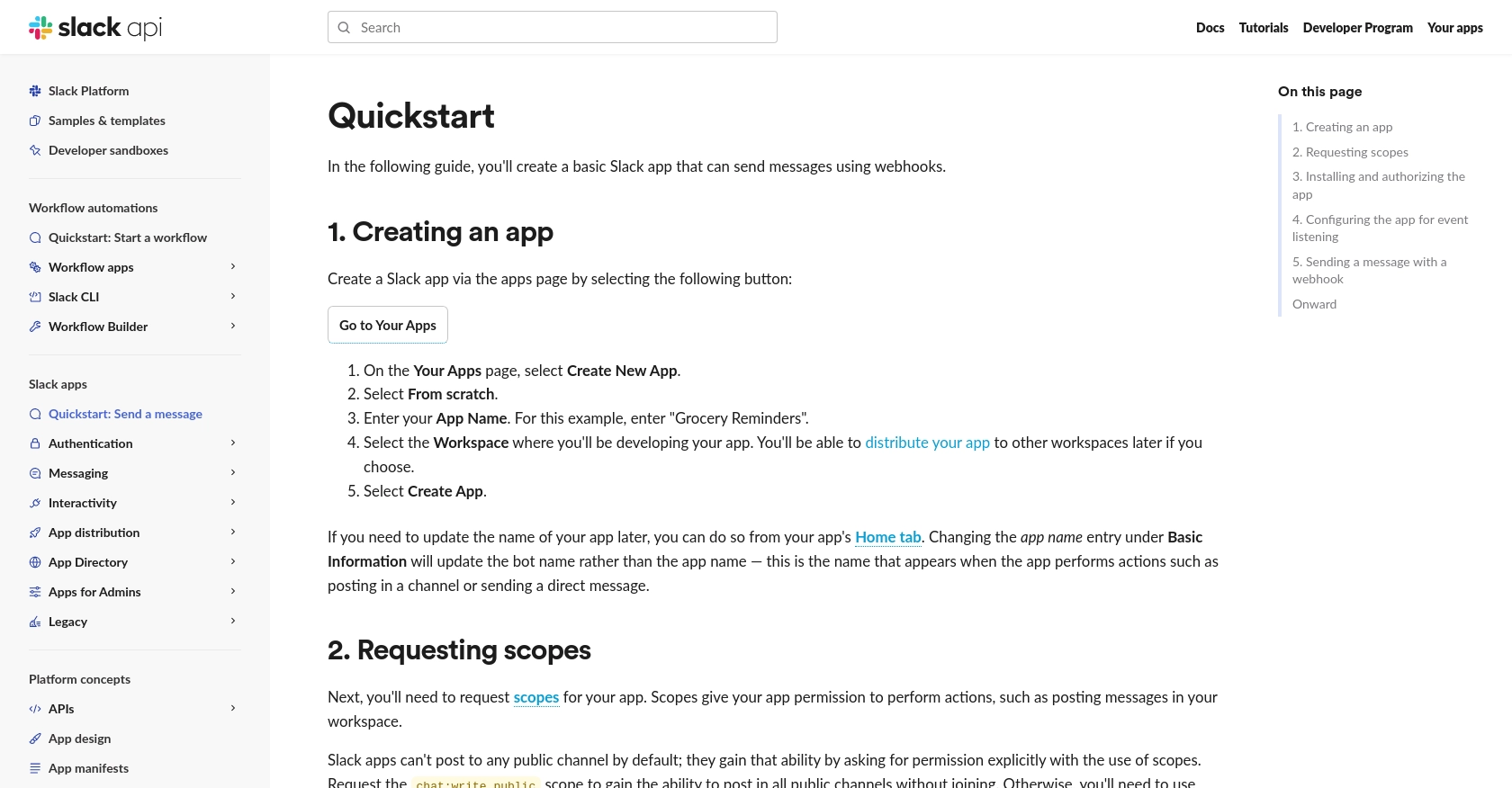
sbb-itb-96038d7
Making API Calls to Send Direct Messages with Slack API Using JavaScript
Now that your Slack app is set up and authorized, it's time to dive into making actual API calls to send direct messages. This section will guide you through the necessary steps and provide JavaScript code examples to help you interact with the Slack API effectively.
Setting Up Your JavaScript Environment for Slack API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Node.js (version 14 or higher)
- NPM (Node Package Manager)
Once you have Node.js and NPM installed, you can set up your project by creating a new directory and initializing it:
mkdir slack-api-integration
cd slack-api-integration
npm init -y
Next, install the Slack Web API client for Node.js:
npm install @slack/web-api
Sending Direct Messages Using Slack API's chat.postMessage Method
To send a direct message to a user, you'll use the chat.postMessage
method provided by the Slack Web API. Here's a step-by-step guide to implementing this in JavaScript:
- Create a new JavaScript file named
sendMessage.js
and open it in your preferred code editor. - Import the necessary modules and initialize the Slack Web API client:
const { WebClient } = require('@slack/web-api');
// Initialize the Slack Web API client with your OAuth token
const token = 'YOUR_OAUTH_ACCESS_TOKEN';
const web = new WebClient(token);
- Define a function to send a direct message:
async function sendDirectMessage(userId, message) {
try {
// Call the chat.postMessage method
const result = await web.chat.postMessage({
channel: userId,
text: message
});
console.log('Message sent: ', result.ts);
} catch (error) {
console.error('Error sending message: ', error);
}
}
- Call the function with the user ID and message you want to send:
// Replace 'USER_ID' with the actual user ID and 'Hello, world!' with your message
sendDirectMessage('USER_ID', 'Hello, world!');
Verifying Successful API Requests and Handling Errors
After executing the script, you should see the message appear in the specified user's direct message channel. The response from the Slack API will include a timestamp (ts
) if the message was sent successfully.
It's crucial to handle potential errors gracefully. The example above includes a try-catch
block to log any errors that occur during the API call. Common errors include invalid tokens, incorrect user IDs, or insufficient permissions.
Testing and Debugging Your Slack API Integration
To ensure your integration works as expected, test it in a development workspace or a dedicated test channel. Monitor the output in your terminal for any error messages and adjust your code as needed.
For more detailed information on the chat.postMessage
method and other available API methods, refer to the Slack Messaging API documentation.
.webp)
Conclusion and Best Practices for Slack API Integration
Integrating with the Slack API to send direct messages using JavaScript can significantly enhance the communication capabilities of your applications. By following the steps outlined in this guide, you can automate messaging processes and create a more seamless user experience within Slack.
Best Practices for Secure and Efficient Slack API Usage
- Securely Store OAuth Tokens: Always store your OAuth tokens securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Slack's rate limits, which are typically 1 request per second per method. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Validate API Responses: Always check the response from Slack API calls for success indicators and handle any errors appropriately to ensure robust integration.
- Use Scopes Wisely: Request only the necessary scopes for your app to minimize security risks and comply with Slack's best practices.
Enhancing Your Integration with Endgrate
While building custom integrations with Slack can be rewarding, it can also be time-consuming and complex. Consider leveraging Endgrate to streamline your integration processes. With Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations, simplifying maintenance.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can support your integration needs by visiting Endgrate's website and discover the benefits of a unified API solution.
Read More
Ready to get started?