Using the NetHunt API to Get Records in PHP
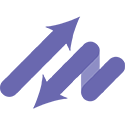
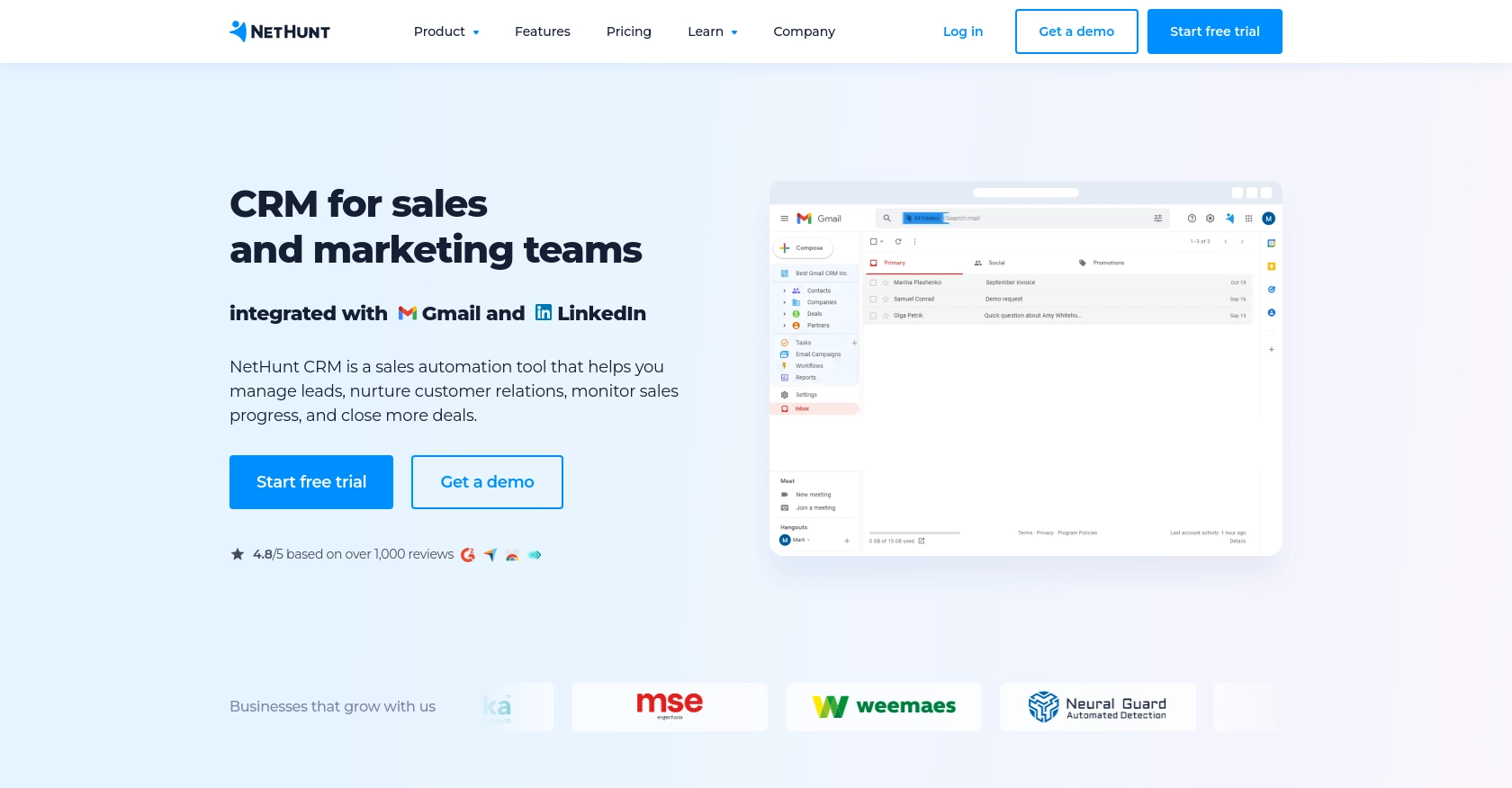
Introduction to NetHunt CRM
NetHunt CRM is a powerful customer relationship management tool that seamlessly integrates with Gmail, providing businesses with an efficient way to manage customer interactions and data. Its robust features include lead management, email campaigns, and sales automation, making it a popular choice for businesses looking to streamline their CRM processes.
Developers might want to integrate with NetHunt's API to automate and enhance their CRM workflows. For example, using the NetHunt API, a developer can retrieve records to generate detailed sales reports or synchronize customer data with other business applications, ensuring a unified data management system.
Setting Up Your NetHunt CRM Account for API Access
Before you can start using the NetHunt API to retrieve records, you need to set up your NetHunt CRM account and obtain the necessary API key for authentication. This key is essential for verifying your requests and ensuring secure access to your data.
Creating a NetHunt CRM Account
If you don't already have a NetHunt CRM account, you can sign up for a free trial on the NetHunt website. Follow the instructions to create your account and log in to access the dashboard.
Generating Your NetHunt API Key
To interact with the NetHunt API, you need to generate an API key. Follow these steps to obtain your API key:
- Log in to your NetHunt CRM account.
- Navigate to the Settings section in the top navigation bar.
- Select Integrations from the menu.
- Locate the option to generate an API key and follow the prompts to create one.
- Copy the generated API key and store it securely, as you will need it for authentication in your API requests.
For more detailed instructions, you can refer to the official documentation on where to get the NetHunt API key.
Understanding NetHunt API Authentication
NetHunt uses Basic authentication for API requests. This involves combining your email and API key, separated by a colon, and encoding the resulting string in base64. Here’s how you can set up your authentication header:
// Example of setting up Basic authentication in PHP
$email = 'your-email@example.com';
$apiKey = 'your-api-key';
$credentials = base64_encode("$email:$apiKey");
$headers = [
'Authorization: Basic ' . $credentials,
'Content-Type: application/json'
];
Ensure you replace your-email@example.com
and your-api-key
with your actual NetHunt email and API key.
With your API key ready and authentication set up, you can now proceed to make API calls to retrieve records from NetHunt CRM.
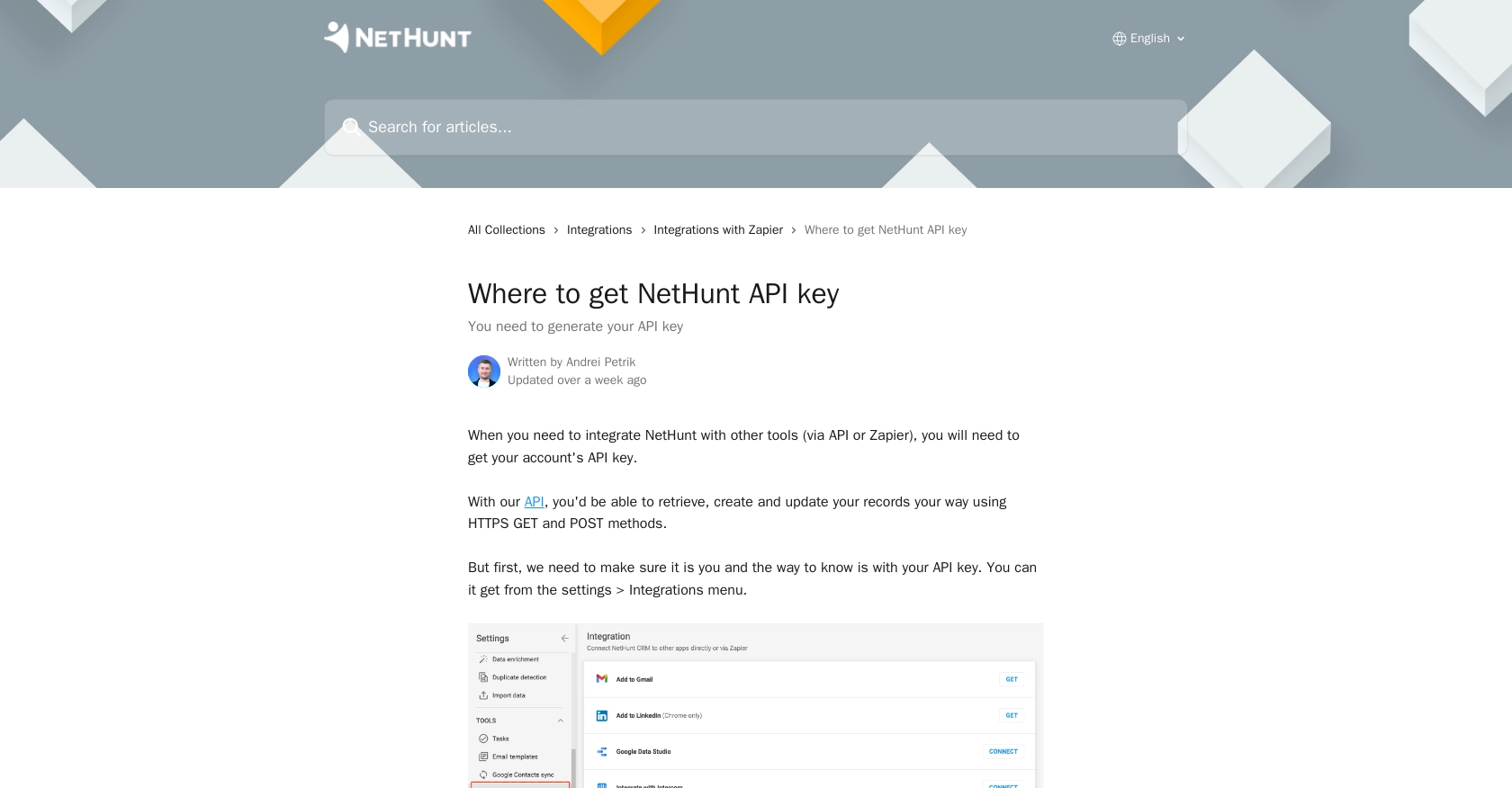
sbb-itb-96038d7
Making API Calls to Retrieve NetHunt Records Using PHP
To interact with the NetHunt API and retrieve records, you'll need to set up your PHP environment and make HTTP requests using the appropriate methods. This section will guide you through the process of making API calls to NetHunt CRM to fetch records efficiently.
Setting Up Your PHP Environment for NetHunt API Integration
Before making API calls, ensure you have PHP installed on your machine. You will also need the cURL
extension enabled to handle HTTP requests. You can verify this by running the following command in your terminal:
php -m | grep curl
If cURL
is not listed, you may need to enable it in your php.ini
file or install it via your package manager.
Installing Required PHP Dependencies
To make HTTP requests, you can use the built-in cURL
library in PHP. Ensure your PHP environment is configured to use this library.
Example Code to Retrieve Records from NetHunt CRM
Below is a sample PHP script to retrieve records from NetHunt CRM using the API:
<?php
// Set your NetHunt API credentials
$email = 'your-email@example.com';
$apiKey = 'your-api-key';
$credentials = base64_encode("$email:$apiKey");
// Set the API endpoint
$url = 'https://nethunt.com/api/v1/zapier/searches/find-record/{folderId}?query=Name%3ADoe&limit=10';
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Basic ' . $credentials,
'Content-Type: application/json'
]);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Parse and display the response
$data = json_decode($response, true);
foreach ($data as $record) {
echo 'Record ID: ' . $record['recordId'] . '<br>';
echo 'Name: ' . $record['fields']['Name'] . '<br>';
}
}
// Close cURL session
curl_close($ch);
?>
Replace your-email@example.com
and your-api-key
with your actual NetHunt email and API key. Also, replace {folderId}
with the appropriate folder ID you wish to query.
Verifying Successful API Requests in NetHunt CRM
After executing the script, you should see the records displayed in your terminal or browser. To verify the request's success, log in to your NetHunt CRM account and check the relevant folder for the retrieved records.
Handling Errors and Common Error Codes
When making API calls, it's crucial to handle potential errors gracefully. Common HTTP error codes include:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server.
Ensure you check the response status and handle errors appropriately in your application.
Conclusion and Best Practices for Using NetHunt API with PHP
Integrating with the NetHunt API using PHP can significantly enhance your CRM workflows by automating data retrieval and synchronization processes. By following the steps outlined in this guide, you can efficiently access and manage your NetHunt CRM records.
Best Practices for Secure and Efficient NetHunt API Integration
- Secure Storage of Credentials: Always store your API key securely. Consider using environment variables or a secure vault to keep your credentials safe.
- Handle Rate Limiting: Be mindful of any rate limits imposed by NetHunt. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that data retrieved from NetHunt is transformed and standardized to fit your application's requirements, maintaining consistency across systems.
- Error Handling: Implement robust error handling to manage API call failures and ensure your application can recover gracefully.
Streamlining Integrations with Endgrate
If managing multiple integrations is becoming complex, consider using Endgrate to simplify the process. Endgrate allows you to build once for each use case and leverage a unified API endpoint to connect with various platforms, including NetHunt. This approach saves time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover a more intuitive integration experience for your customers.
Read More
Ready to get started?