Using the Jira API to Get Users (with Python examples)
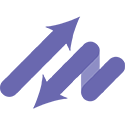
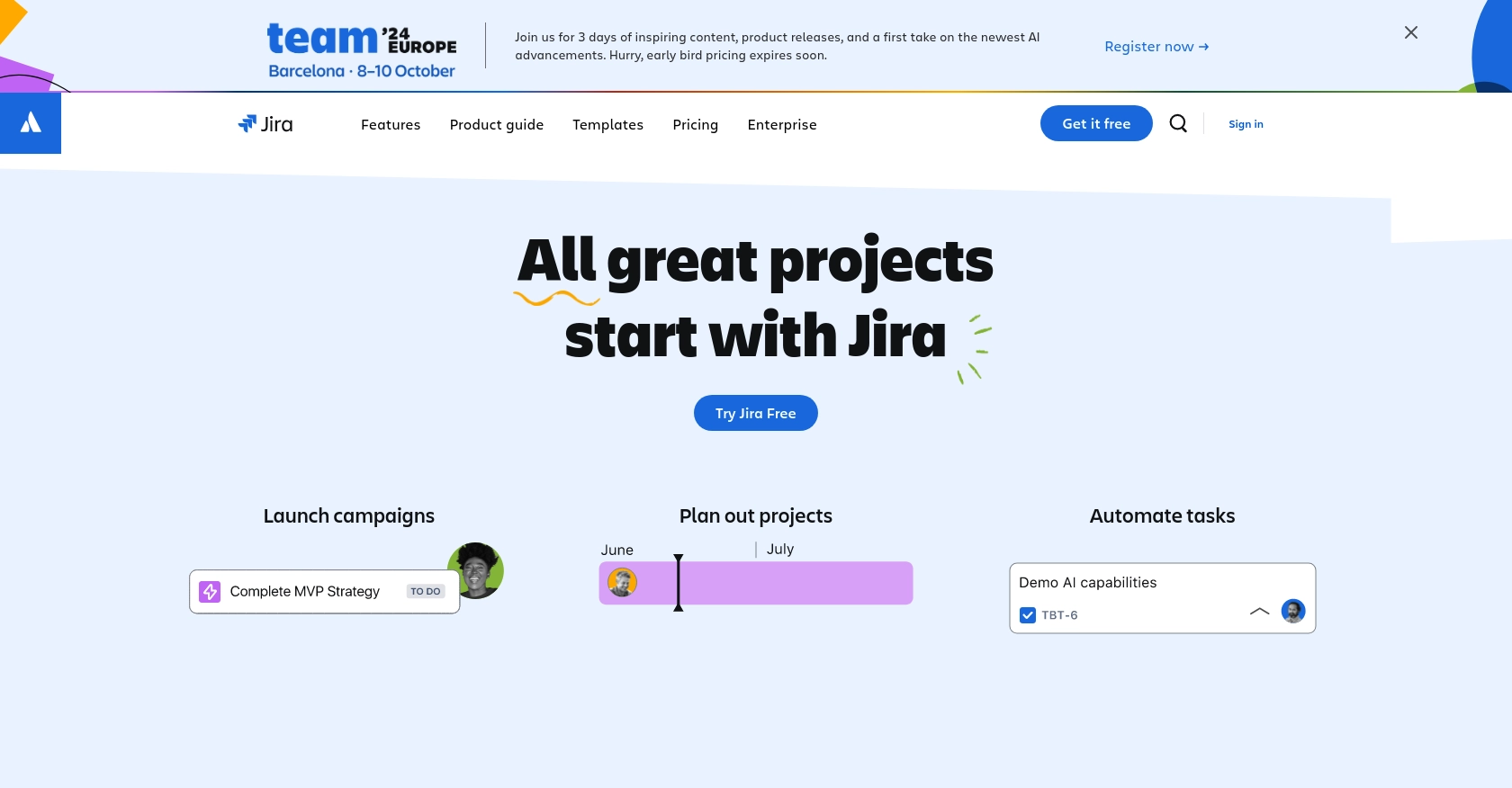
Introduction to Jira API Integration
Jira is a powerful project management tool widely used by software development teams to plan, track, and manage agile software development projects. It offers a robust set of features for issue tracking, project management, and collaboration, making it an essential tool for teams aiming to enhance productivity and streamline workflows.
Integrating with Jira's API allows developers to automate and customize their interactions with Jira, enabling seamless integration with other systems and applications. For example, developers might use the Jira API to retrieve user information, which can be useful for synchronizing user data across different platforms or for generating custom reports.
This article will guide you through the process of using Python to interact with the Jira API, specifically focusing on how to retrieve user information. By following this tutorial, you will learn how to set up your environment, authenticate with Jira using OAuth 2.0, and make API calls to fetch user data efficiently.
Setting Up Your Jira Test/Sandbox Account for API Integration
Before diving into the Jira API integration, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your production data. Follow these steps to create a Jira sandbox account and configure the necessary OAuth 2.0 authentication.
Creating a Jira Sandbox Account
If you don't already have a Jira account, you can sign up for a free trial or use an existing account to create a sandbox environment. Visit the Jira website and follow the instructions to set up your account.
- Navigate to the Jira sign-up page.
- Choose the free trial option to explore Jira's features.
- Complete the registration process by providing the necessary details.
Configuring OAuth 2.0 for Jira API Access
Jira uses OAuth 2.0 for secure API access. To authenticate your API requests, you'll need to create an OAuth 2.0 app in the Jira developer console. Follow these steps:
- Log in to your Jira account and navigate to the Atlassian Developer Console.
- Click on "Create app" and select "OAuth 2.0" as the authentication method.
- Provide a name and description for your app.
- Under "Authorization," specify the necessary scopes for your app. For retrieving user information, include scopes like
read:jira-user
. - Save your app to generate the client ID and client secret. Keep these credentials secure as they are required for API authentication.
Generating Access Tokens for Jira API Requests
With your OAuth 2.0 app configured, you can now generate access tokens to authenticate your API requests. Use the following steps to obtain an access token:
- Use a tool like Postman or a custom script to send a POST request to the token endpoint:
https://auth.atlassian.com/oauth/token
. - Include the following parameters in your request:
- grant_type:
authorization_code
- client_id: Your app's client ID
- client_secret: Your app's client secret
- code: The authorization code obtained from the authorization URL
- redirect_uri: The redirect URI specified in your app settings
- Upon successful authentication, you'll receive an access token. Use this token in the Authorization header of your API requests.
With your Jira sandbox account and OAuth 2.0 authentication set up, you're ready to start making API calls to retrieve user information. In the next section, we'll explore how to use Python to interact with the Jira API effectively.
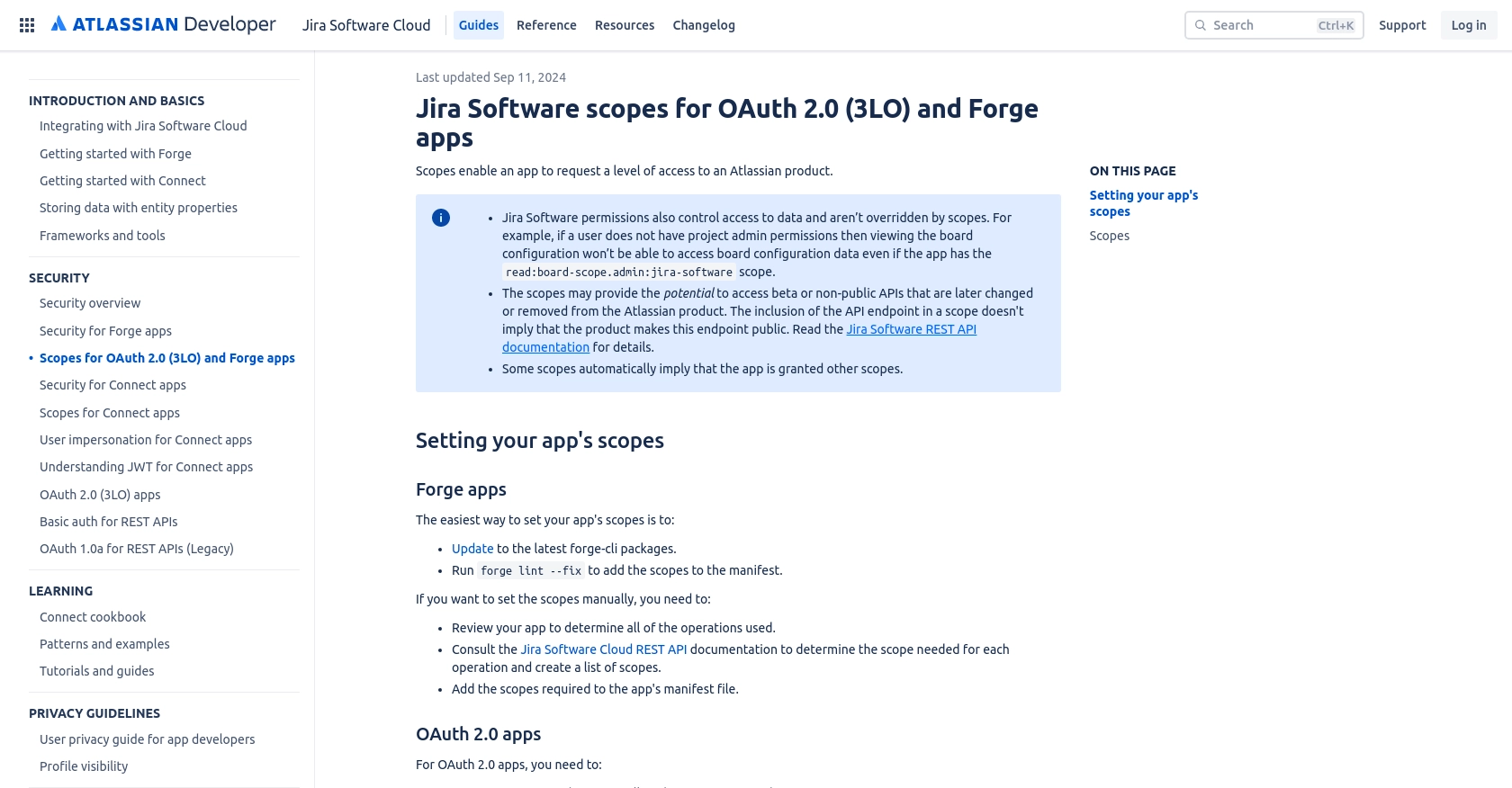
sbb-itb-96038d7
Making API Calls to Retrieve Jira Users with Python
Now that you have your Jira sandbox account and OAuth 2.0 authentication set up, it's time to make API calls to retrieve user information using Python. This section will guide you through the necessary steps, including setting up your Python environment, writing the code to interact with the Jira API, and handling potential errors.
Setting Up Your Python Environment for Jira API Integration
To interact with the Jira API using Python, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once you have these installed, open your terminal or command prompt and install the requests
library, which will be used to make HTTP requests:
pip install requests
Writing Python Code to Fetch Users from Jira API
Create a new Python file named get_jira_users.py
and add the following code to retrieve user information from Jira:
import requests
# Set the API endpoint and headers
endpoint = "https://your-domain.atlassian.net/rest/api/3/users/search"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Accept": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
users = response.json()
for user in users:
print(f"User: {user['displayName']}, Email: {user.get('emailAddress', 'N/A')}")
else:
print(f"Failed to retrieve users: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token you obtained earlier. This script sends a GET request to the Jira API to fetch user information and prints each user's display name and email address.
Verifying Successful API Requests and Handling Errors
After running the script, you should see a list of users with their display names and email addresses printed in the console. If the request fails, the script will output an error message with the status code and response text.
To verify that the request succeeded, you can cross-check the returned user data with the users in your Jira sandbox account. If there are discrepancies, ensure that your access token is valid and that you have the necessary permissions.
Handling Common Jira API Errors
When working with the Jira API, you may encounter various error codes. Here are some common ones and how to handle them:
- 401 Unauthorized: Ensure your access token is correct and has not expired.
- 403 Forbidden: Check if your app has the necessary permissions to access user data.
- 404 Not Found: Verify the API endpoint URL and ensure it is correct.
For more detailed information on error codes, refer to the Jira API documentation.
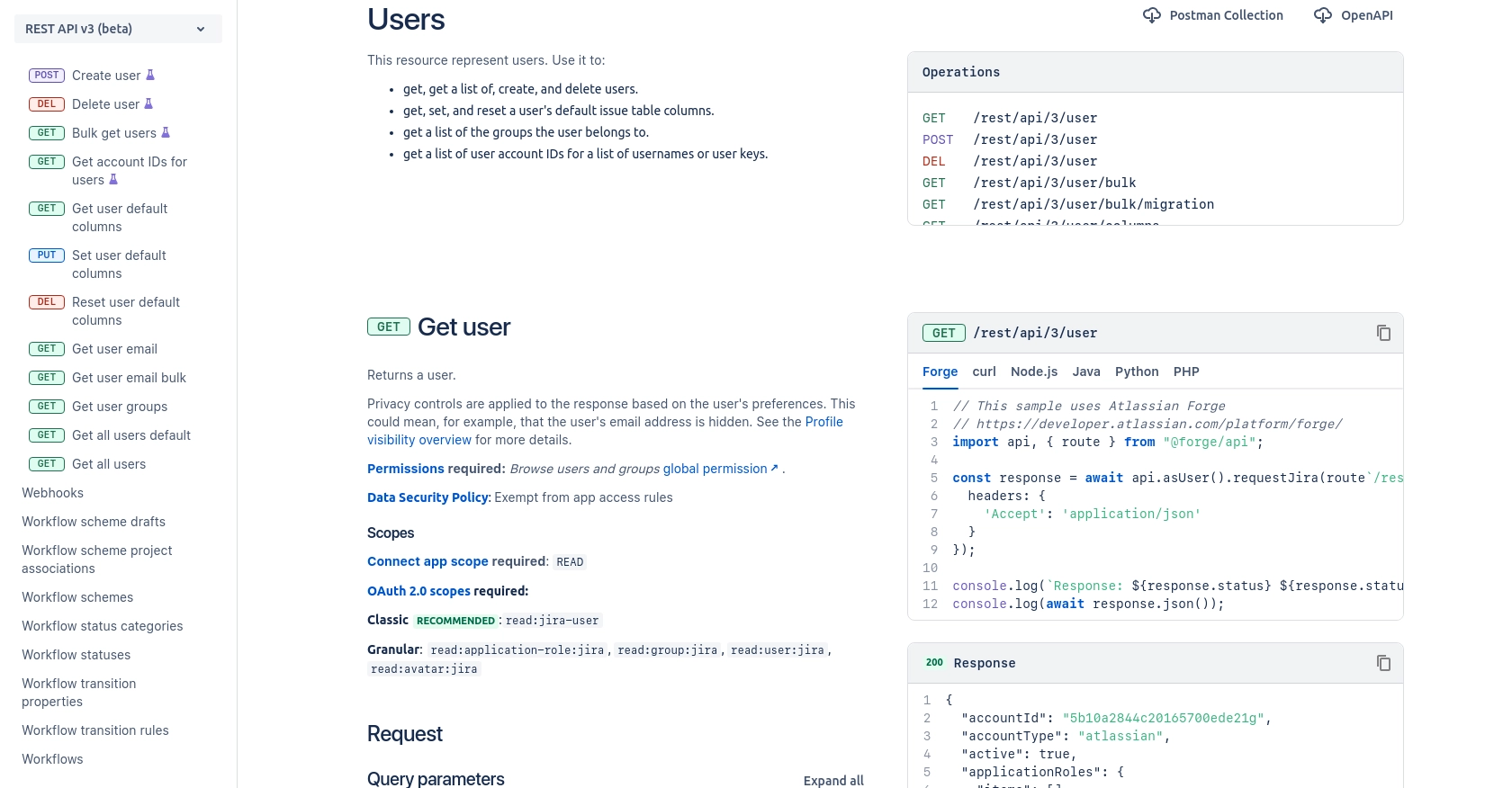
Conclusion and Best Practices for Jira API Integration
Integrating with the Jira API using Python provides a powerful way to automate and enhance your project management workflows. By following the steps outlined in this article, you can efficiently retrieve user information and integrate it with other systems, improving data synchronization and reporting capabilities.
Best Practices for Secure and Efficient Jira API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Jira API has rate limits to ensure fair usage. Implement retry logic with exponential backoff to handle
429 Too Many Requests
errors gracefully. For more details, refer to the Jira API documentation. - Data Transformation and Standardization: When integrating user data across platforms, ensure consistent data formats and handle any necessary transformations to maintain data integrity.
Streamlining Integrations with Endgrate
While integrating with Jira is beneficial, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Jira. This allows you to focus on your core product while outsourcing integration complexities.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can save you time and resources by visiting Endgrate.
Read More
Ready to get started?