Using the BigCommerce API to Get Orders (with PHP examples)
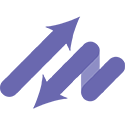
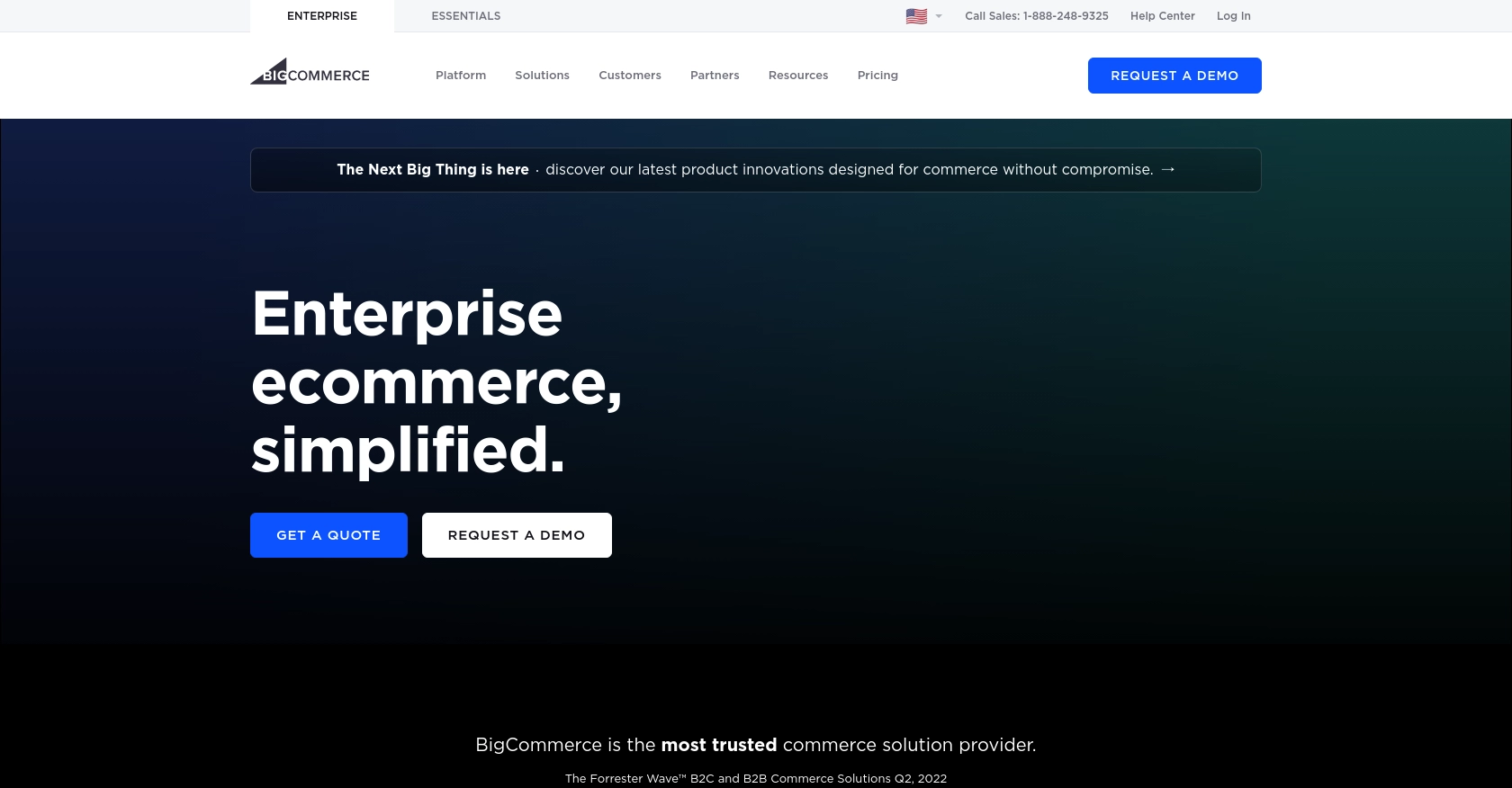
Introduction to BigCommerce API Integration
BigCommerce is a powerful e-commerce platform that provides businesses with the tools needed to create and manage online stores. With its robust set of features, it supports everything from product listings and order management to customer engagement and analytics.
Developers often seek to integrate with BigCommerce's API to streamline operations and enhance the functionality of their online stores. By connecting with the BigCommerce API, developers can automate tasks such as retrieving order data, which is crucial for inventory management, customer service, and sales analysis.
For example, a developer might use the BigCommerce API to fetch order details and update a third-party inventory system in real-time, ensuring that stock levels are always accurate and up-to-date.
Setting Up Your BigCommerce Test or Sandbox Account
Before you can start interacting with the BigCommerce API, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live store data.
Create a BigCommerce Sandbox Account
To begin, you'll need to create a sandbox account on BigCommerce. This account provides a controlled environment where you can test API interactions:
- Visit the BigCommerce Sandbox page.
- Sign up for a sandbox account by providing the necessary details.
- Once registered, you'll receive access to a sandbox store where you can test API calls.
Generate API Credentials for BigCommerce
After setting up your sandbox account, you need to generate API credentials to authenticate your requests:
- Log in to your BigCommerce sandbox store.
- Navigate to Advanced Settings > API Accounts.
- Click on Create API Account and select Create V2/V3 API Token.
- Fill in the required details, such as the name and permissions for the API account.
- Ensure you have the necessary permissions to access orders, such as Orders Read-Only.
- Click Save to generate your API credentials, including the Client ID, Client Secret, and Access Token.
- Store these credentials securely, as you'll need them to authenticate your API requests.
Understanding BigCommerce API Authentication
BigCommerce uses OAuth-based authentication for API requests. You'll need to include the X-Auth-Token
header with your access token in each request. For more details, refer to the BigCommerce Authentication Documentation.
With your sandbox account and API credentials ready, you're all set to start making API calls to retrieve order data from BigCommerce.
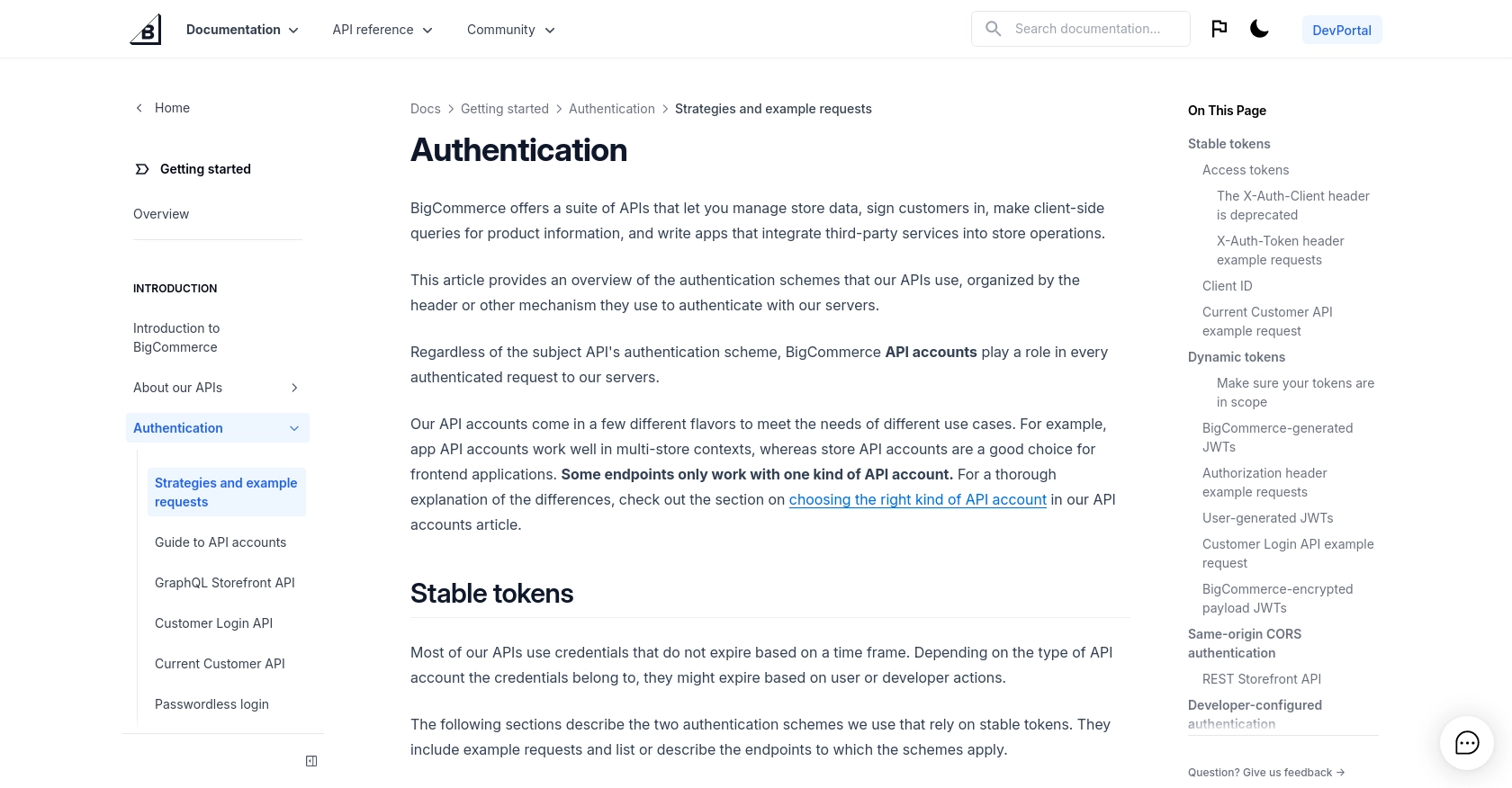
sbb-itb-96038d7
Making API Calls to Retrieve Orders from BigCommerce Using PHP
To interact with the BigCommerce API and retrieve order data, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your PHP Environment for BigCommerce API Integration
Before you begin, ensure that you have PHP installed on your system. You can download it from the official PHP website. Additionally, you'll need the cURL
extension enabled to make HTTP requests.
- Verify your PHP installation by running
php -v
in your terminal. - Ensure the
cURL
extension is enabled by checking yourphp.ini
file.
Writing PHP Code to Fetch Orders from BigCommerce
Now that your environment is ready, you can write a PHP script to retrieve orders from BigCommerce. The following example demonstrates how to make a GET request to the BigCommerce API to fetch all orders.
<?php
// Set your store hash and access token
$store_hash = 'your_store_hash';
$access_token = 'your_access_token';
// Define the API endpoint
$url = "https://api.bigcommerce.com/stores/{$store_hash}/v2/orders";
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'X-Auth-Token: ' . $access_token,
'Accept: application/json',
]);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Decode and display the response
$orders = json_decode($response, true);
foreach ($orders as $order) {
echo "Order ID: " . $order['id'] . "\n";
echo "Status: " . $order['status'] . "\n";
echo "Total: " . $order['total_inc_tax'] . "\n\n";
}
}
// Close cURL session
curl_close($ch);
?>
Replace your_store_hash
and your_access_token
with your actual store hash and access token obtained from your BigCommerce API credentials.
Verifying API Call Success and Handling Errors
After executing the script, you should see a list of orders printed in your terminal. If the request is successful, the response will contain order details such as the order ID, status, and total amount.
In case of errors, the script will output the error message. Common issues might include incorrect credentials or network problems. Ensure your access token is valid and that your network connection is stable.
For more details on error codes and handling, refer to the BigCommerce Orders API Documentation.
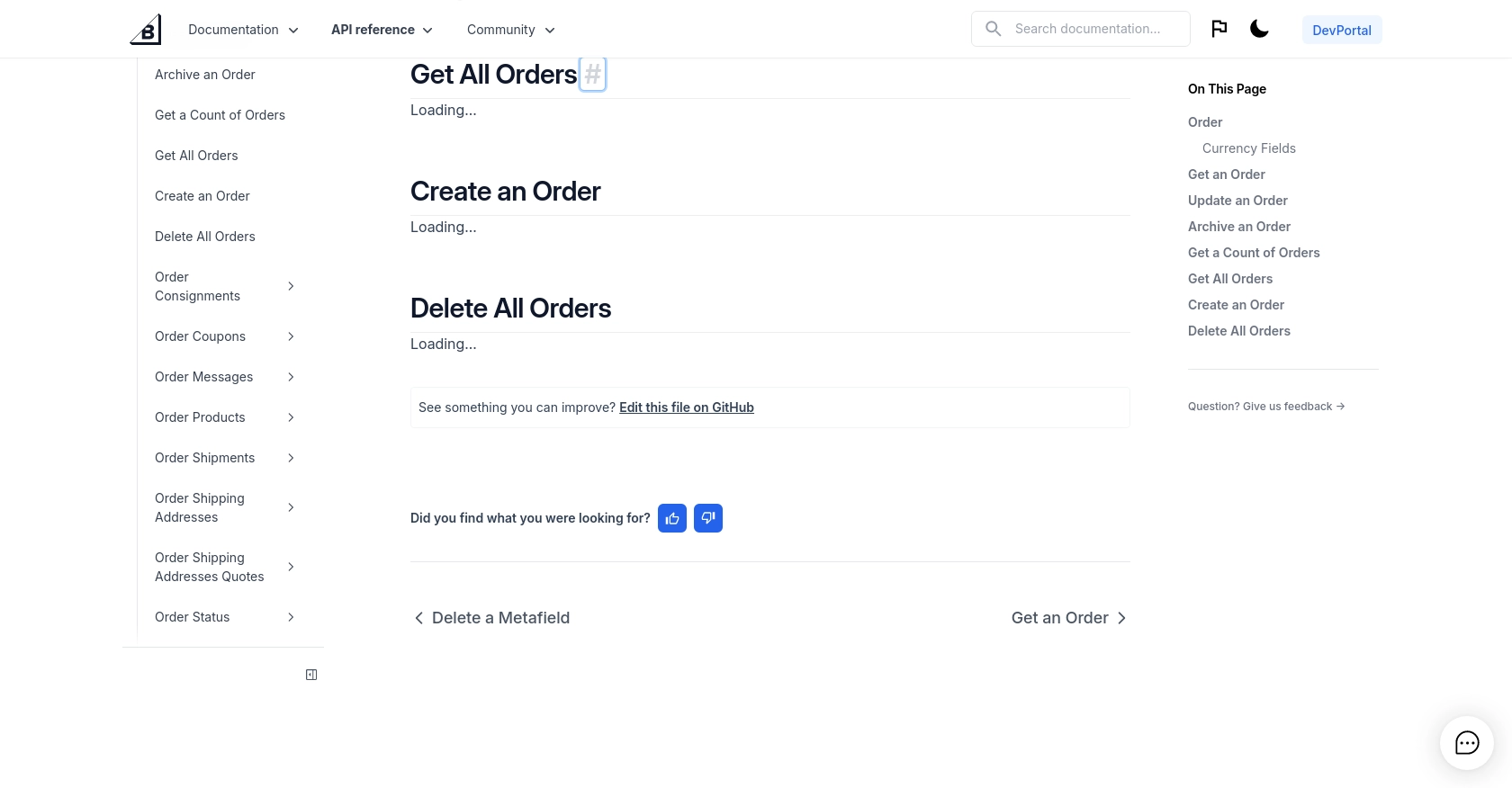
Conclusion and Best Practices for BigCommerce API Integration
Integrating with the BigCommerce API using PHP allows developers to efficiently manage order data, enhancing the overall functionality of e-commerce operations. By following the steps outlined in this guide, you can seamlessly retrieve order information and integrate it with other systems, ensuring accurate and up-to-date data management.
Best Practices for Secure and Efficient BigCommerce API Usage
- Secure Storage of Credentials: Always store your API credentials securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of BigCommerce's API rate limits to avoid throttling. Implement retry logic and exponential backoff strategies to handle rate limit responses gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from BigCommerce is transformed and standardized according to your system's requirements for consistent data handling.
- Error Handling: Implement robust error handling to manage API call failures. Log errors for further analysis and ensure that your application can recover gracefully from unexpected issues.
Streamline Your Integration Process with Endgrate
For developers looking to simplify their integration processes, consider using Endgrate. By leveraging Endgrate's unified API, you can connect to multiple platforms, including BigCommerce, through a single endpoint. This approach not only saves time and resources but also provides a seamless integration experience for your customers.
Visit Endgrate to learn more about how you can optimize your integration efforts and focus on building your core product.
Read More
Ready to get started?