Using the Sap Business One API to Create or Update Orders in Python
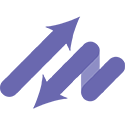
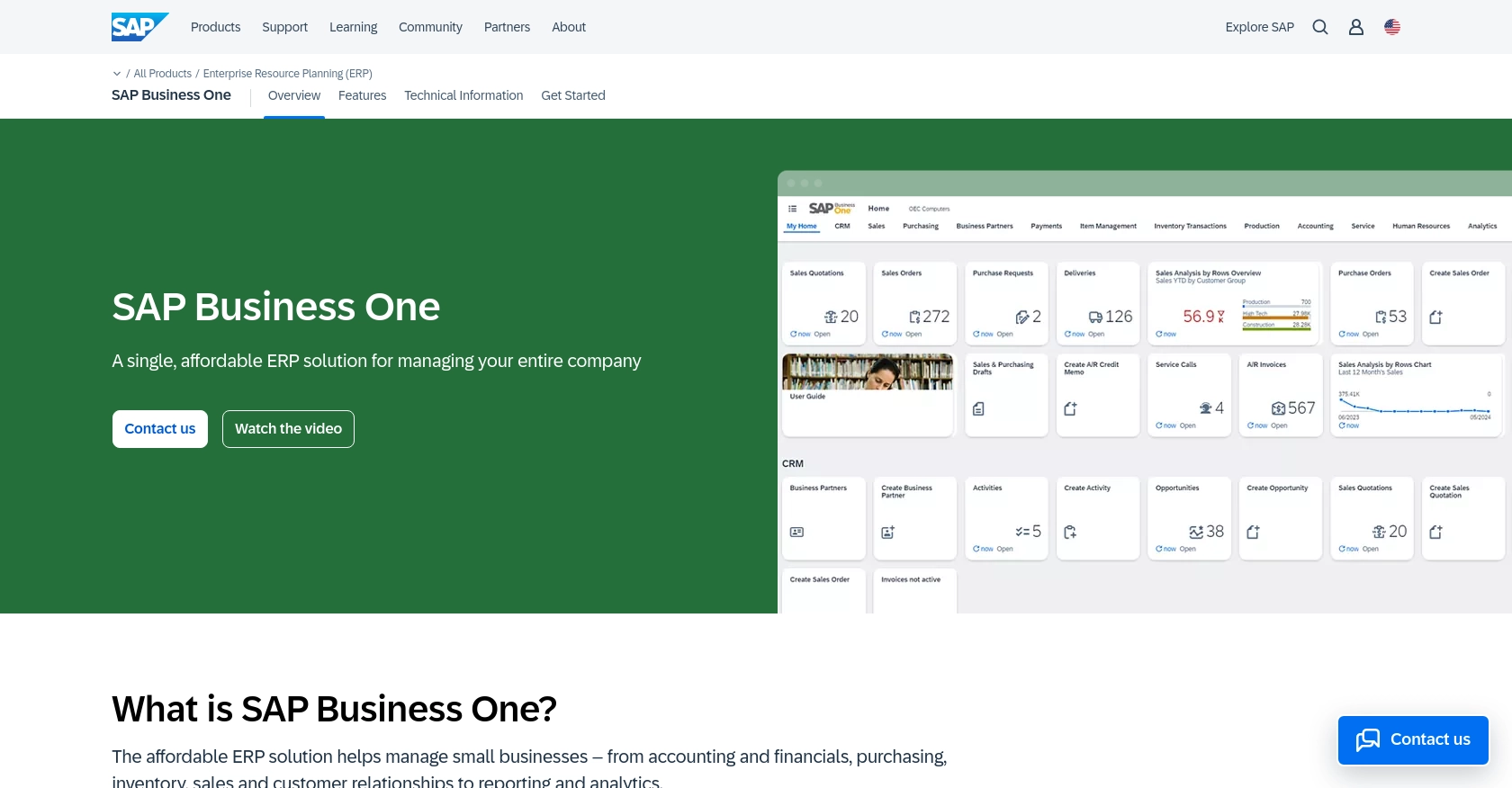
Introduction to SAP Business One API
SAP Business One is a comprehensive enterprise resource planning (ERP) solution designed for small to medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and inventory control. By providing a unified platform, SAP Business One helps businesses streamline operations and improve efficiency.
Integrating with the SAP Business One API allows developers to automate and enhance business processes, such as creating or updating orders. For example, a developer might use the API to automatically update order statuses in real-time as they are processed, ensuring that sales and inventory data remain accurate and up-to-date.
Setting Up Your SAP Business One Test/Sandbox Account
Before you can start integrating with the SAP Business One API, you need to set up a test or sandbox account. This environment allows you to safely experiment with the API without affecting your live data.
Creating a SAP Business One Sandbox Account
To begin, you need access to a SAP Business One sandbox environment. If your organization already uses SAP Business One, you can request access to a sandbox instance from your system administrator. If not, you may need to contact SAP directly or work with a SAP partner to set up a trial or demo account.
Configuring OAuth Authentication for SAP Business One API
SAP Business One uses a custom authentication method. Follow these steps to configure your authentication:
- Log in to your SAP Business One sandbox account.
- Navigate to the administration panel and locate the API management section.
- Create a new application to obtain your client ID and client secret. These credentials will be used to authenticate your API requests.
- Ensure that your application has the necessary permissions to create and update orders.
Generating API Keys for SAP Business One
If your SAP Business One instance uses API key-based authentication, follow these steps:
- Access the API key management section in your SAP Business One account.
- Generate a new API key and make sure to store it securely. You will need this key to authenticate your API calls.
For more detailed information on authentication, refer to the official SAP documentation: SAP Business One Service Layer Documentation and Service Layer API Reference.
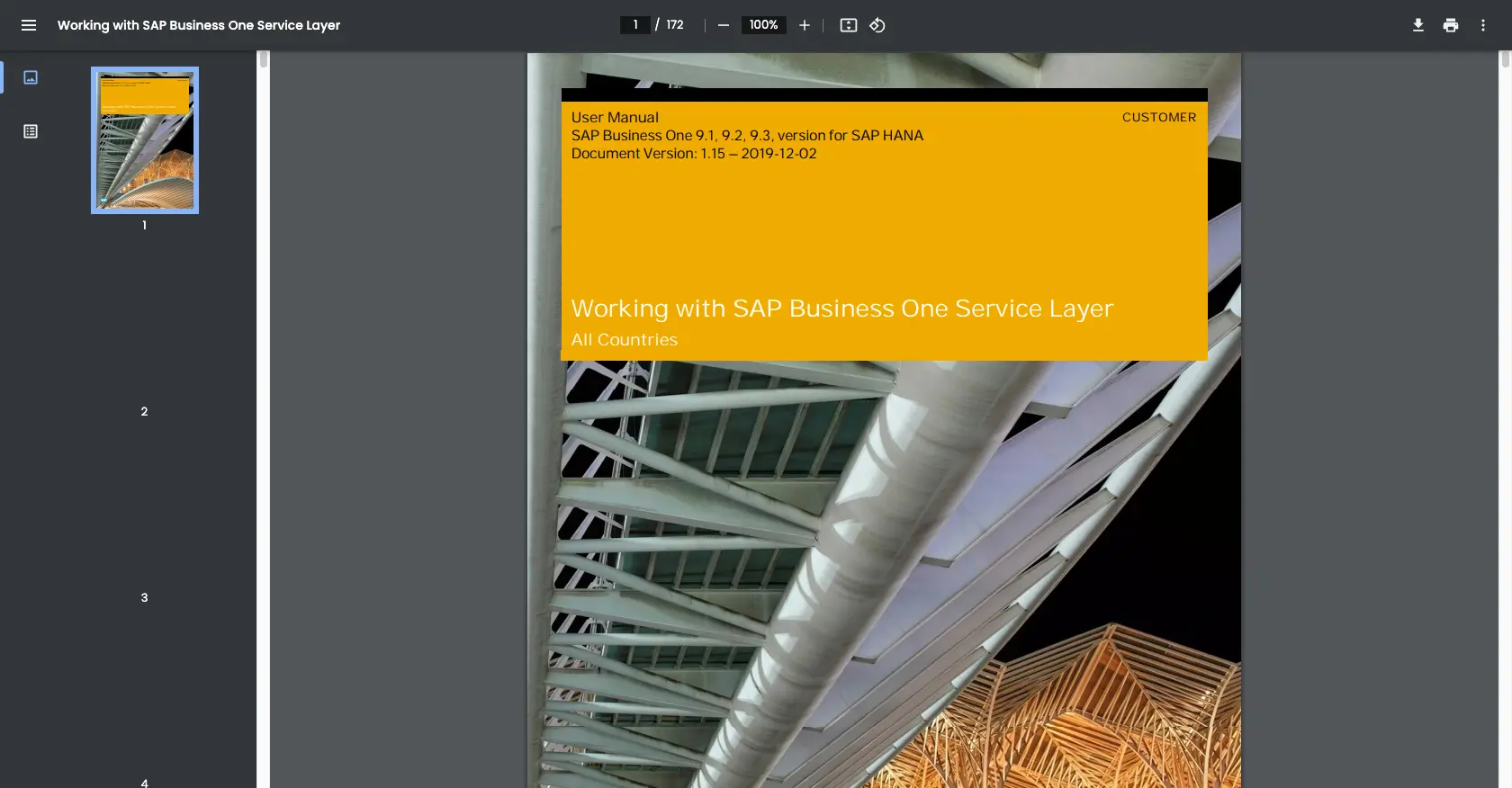
sbb-itb-96038d7
Making API Calls to Create or Update Orders in SAP Business One Using Python
To interact with the SAP Business One API for creating or updating orders, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the necessary steps to set up your environment, make API calls, and handle responses effectively.
Setting Up Your Python Environment for SAP Business One API Integration
Before you begin, ensure you have Python installed on your machine. We recommend using Python 3.11.1 or later for compatibility. Additionally, you'll need the requests
library to handle HTTP requests.
pip install requests
Creating or Updating Orders with SAP Business One API
Once your environment is ready, you can proceed to write the code to create or update orders. Below is a sample Python script demonstrating how to make an API call to SAP Business One.
import requests
import json
# Define the API endpoint and headers
url = "https://your-sap-business-one-instance.com/b1s/v1/Orders"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the order data
order_data = {
"CardCode": "C20000",
"DocDueDate": "2023-10-15",
"DocumentLines": [
{
"ItemCode": "A00001",
"Quantity": 2,
"Price": 100
}
]
}
# Make a POST request to create a new order
response = requests.post(url, headers=headers, data=json.dumps(order_data))
# Check if the request was successful
if response.status_code == 201:
print("Order created successfully:", response.json())
else:
print("Failed to create order:", response.status_code, response.text)
Replace Your_Access_Token
with the token obtained during authentication. This script sends a POST request to the SAP Business One API to create a new order. If successful, it will print the order details; otherwise, it will display an error message.
Verifying API Call Success in SAP Business One Sandbox
After executing the script, verify the success of your API call by checking the SAP Business One sandbox environment. Navigate to the orders section to confirm that the new order appears with the specified details.
Handling Errors and Common Error Codes in SAP Business One API
When working with the SAP Business One API, it's crucial to handle potential errors gracefully. Common error codes include:
- 400 Bad Request: The request was malformed. Check your JSON structure and required fields.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 404 Not Found: The specified resource could not be found. Ensure the endpoint URL is correct.
- 500 Internal Server Error: An error occurred on the server. Try again later or contact support.
Implement error handling in your code to manage these scenarios and provide meaningful feedback to users.
Conclusion and Best Practices for SAP Business One API Integration
Integrating with the SAP Business One API to create or update orders can significantly enhance your business processes by automating tasks and ensuring data accuracy. However, it's essential to follow best practices to ensure a smooth and secure integration.
Best Practices for Storing User Credentials Securely
Always store user credentials securely. Use environment variables or secure vaults to manage sensitive information like API keys and access tokens. Avoid hardcoding these credentials in your source code.
Handling SAP Business One API Rate Limiting
Be mindful of the API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully. Although specific rate limit information is not provided, always refer to the official SAP documentation for updates.
Data Transformation and Standardization
Ensure that data sent to and received from the SAP Business One API is correctly transformed and standardized. This includes converting date formats, currency values, and other data types to match the API's requirements.
Call to Action: Simplify Your Integrations with Endgrate
While integrating with SAP Business One can be highly beneficial, managing multiple integrations can be complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint for various platforms, including SAP Business One. Save time and resources by outsourcing your integrations to Endgrate, allowing you to focus on your core product development.
Explore how Endgrate can transform your integration experience by visiting Endgrate today.
Read More
Ready to get started?