Using the Shopify API to Create or Update Custom Collections (with Javascript examples)
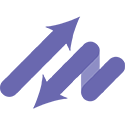
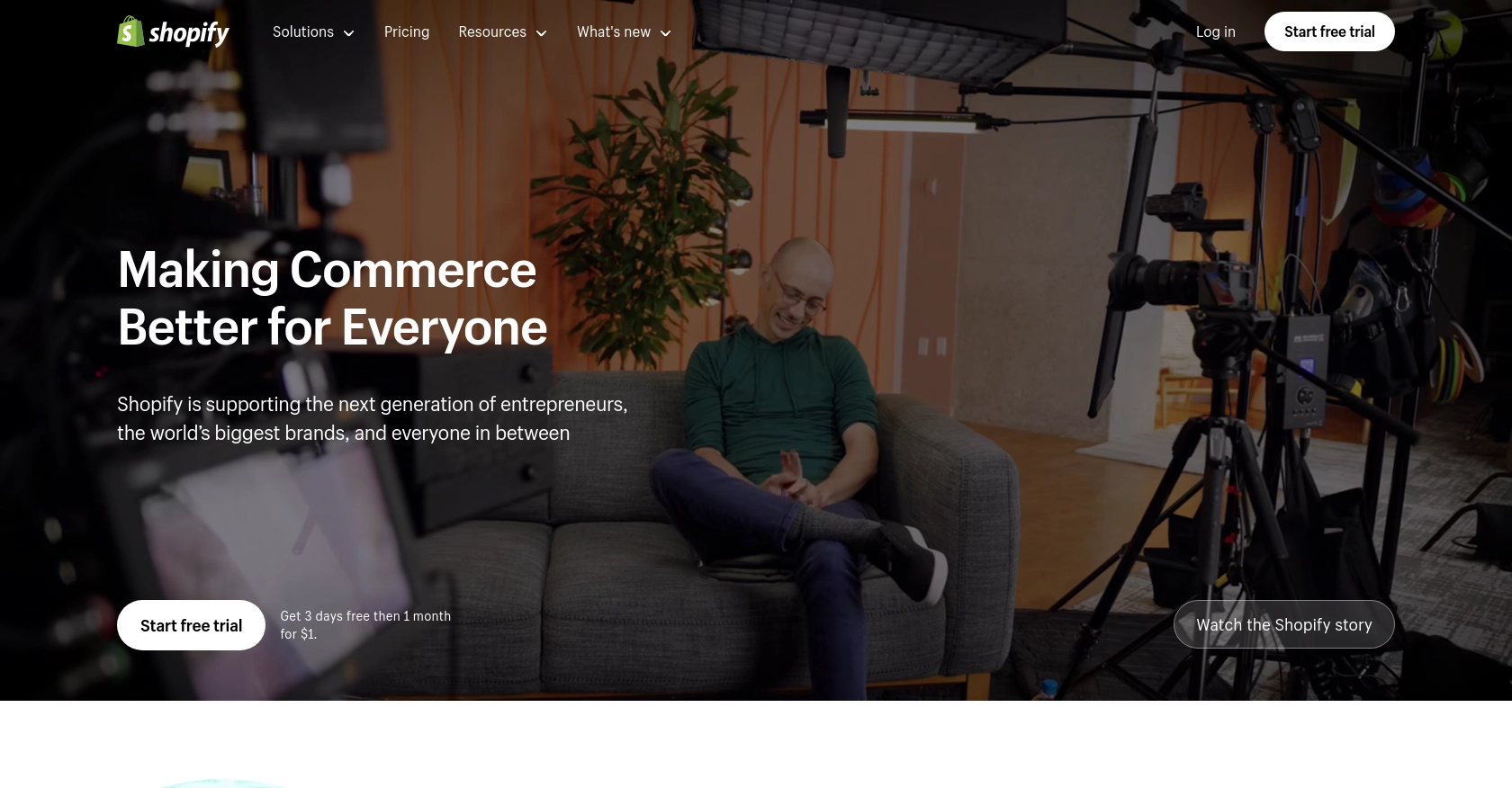
Introduction to Shopify API Integration
Shopify is a leading e-commerce platform that empowers businesses to create and manage their online stores with ease. It offers a comprehensive suite of tools for managing products, orders, and customer relationships, making it a popular choice for businesses of all sizes looking to establish a robust online presence.
Integrating with Shopify's API allows developers to enhance and automate various aspects of store management. For example, using the Shopify API, developers can create or update custom collections, enabling merchants to organize products efficiently and improve the shopping experience for their customers.
This article will guide you through the process of using JavaScript to interact with the Shopify API, specifically focusing on creating or updating custom collections. By following this tutorial, developers can streamline their workflow and leverage Shopify's powerful features to build more dynamic and responsive e-commerce solutions.
Setting Up a Shopify Test Account for API Integration
Before diving into the Shopify API, it's essential to set up a test environment. This allows developers to experiment with API calls without affecting a live store. Shopify provides a development store option that is perfect for testing and development purposes.
Creating a Shopify Development Store
To get started, you'll need to create a Shopify Partner account if you don't already have one. Follow these steps to set up a development store:
- Visit the Shopify Partners page and sign up for an account.
- Once your account is created, log in to the Shopify Partner Dashboard.
- Navigate to the "Stores" section and click on "Add store."
- Select "Development store" as the store type.
- Fill in the required details, such as store name and password, and click "Save."
Your development store is now ready, and you can use it to test API integrations without any risk to a live environment.
Setting Up OAuth Authentication for Shopify API
Shopify uses OAuth for authenticating API requests. Follow these steps to set up OAuth authentication:
- In the Shopify Partner Dashboard, go to "Apps" and click "Create app."
- Enter the app name and select the development store you created earlier.
- Under "App setup," note the API key and API secret key. These will be used for authentication.
- Set up the necessary scopes for your app. For creating or updating collections, ensure you have the "write_products" scope.
- Save your changes and use the API key and secret to generate an access token.
For more detailed information on authentication, refer to the Shopify authentication documentation.
Generating Access Tokens for API Requests
To interact with the Shopify API, you'll need to generate an access token. Here's how:
- Direct the user to the Shopify authorization URL with the necessary scopes.
- Once the user authorizes the app, Shopify will redirect to your app's redirect URI with a code parameter.
- Exchange this code for an access token by making a POST request to Shopify's token endpoint.
Include the access token in the X-Shopify-Access-Token
header for all API requests.
With your development store and OAuth authentication set up, you're ready to start making API calls to create or update custom collections in Shopify using JavaScript.
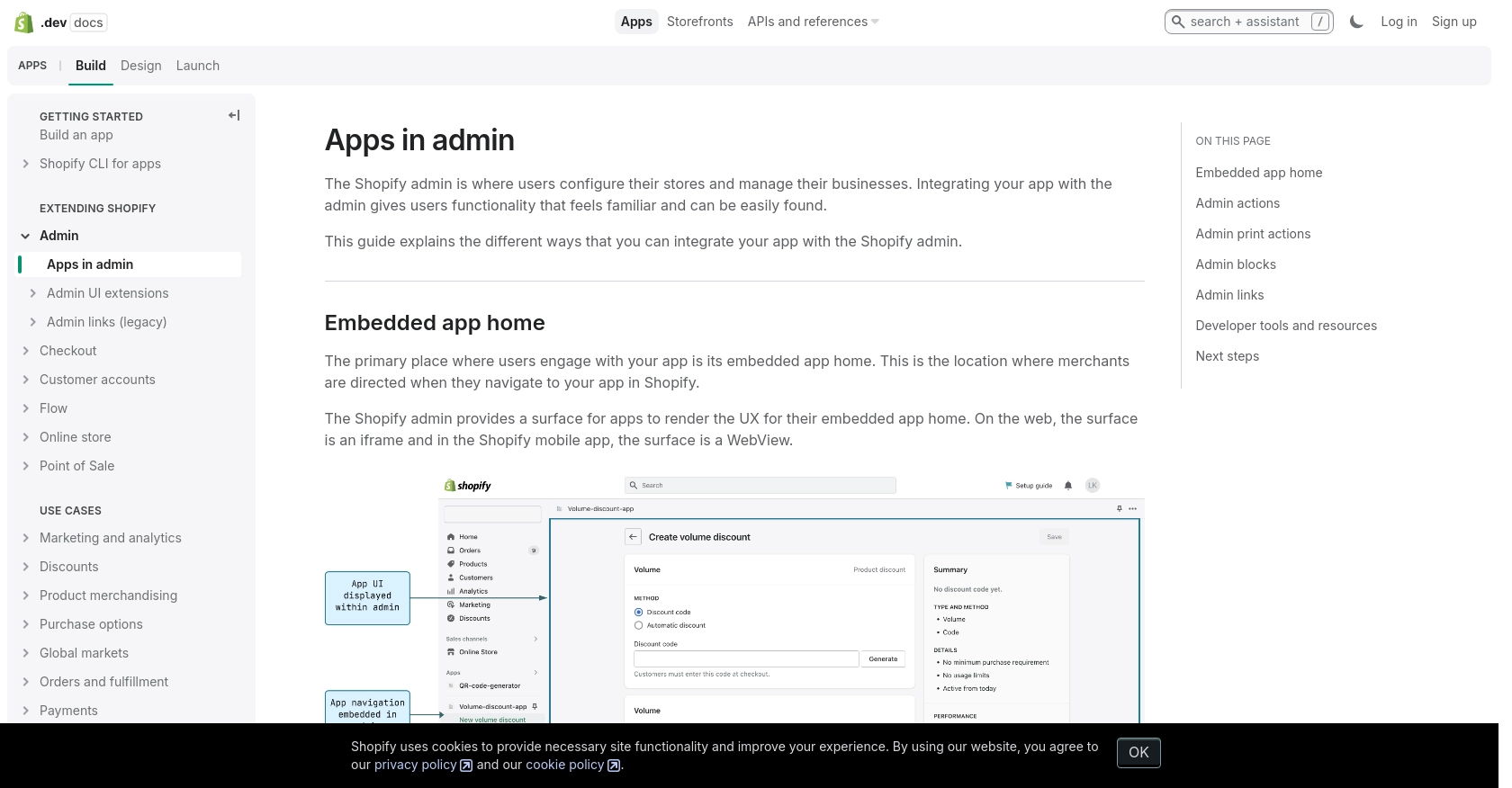
sbb-itb-96038d7
Making API Calls to Shopify for Creating or Updating Custom Collections Using JavaScript
To interact with Shopify's API for creating or updating custom collections, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process, including setting up your environment, writing the code, and handling responses and errors.
Setting Up Your JavaScript Environment for Shopify API Integration
Before making API calls, ensure you have the necessary tools and libraries installed. You'll need:
- Node.js (version 14 or higher)
- npm (Node Package Manager)
- A code editor like Visual Studio Code
Install the Axios library to simplify HTTP requests:
npm install axios
Writing JavaScript Code to Create or Update Shopify Custom Collections
With your environment set up, you can now write the JavaScript code to interact with the Shopify API. Here's a step-by-step guide:
const axios = require('axios');
// Shopify store details
const storeName = 'your-development-store';
const accessToken = 'your_access_token';
// API endpoint for creating or updating collections
const endpoint = `https://${storeName}.myshopify.com/admin/api/2024-07/custom_collections.json`;
// Function to create or update a custom collection
async function createOrUpdateCollection(collectionData) {
try {
const response = await axios.post(endpoint, collectionData, {
headers: {
'Content-Type': 'application/json',
'X-Shopify-Access-Token': accessToken
}
});
console.log('Collection created/updated successfully:', response.data);
} catch (error) {
console.error('Error creating/updating collection:', error.response.data);
}
}
// Sample collection data
const collectionData = {
custom_collection: {
title: 'New Collection',
body_html: 'Collection description',
published: true
}
};
// Call the function
createOrUpdateCollection(collectionData);
Replace your-development-store
and your_access_token
with your actual store name and access token.
Understanding the Response and Verifying Success in Shopify
After running the code, you should see a success message in the console if the collection is created or updated successfully. You can verify this by checking the Shopify admin panel under the Collections section.
Handling Errors and Shopify API Error Codes
When making API calls, it's crucial to handle potential errors. Shopify's API may return various error codes, such as:
- 401 Unauthorized: Invalid API key or access token.
- 403 Forbidden: Incorrect access scopes.
- 404 Not Found: Resource not found.
- 429 Too Many Requests: Rate limit exceeded.
Refer to the Shopify authentication documentation for more details on error handling.
By following these steps, you can efficiently create or update custom collections in Shopify using JavaScript, enhancing your e-commerce store's functionality and user experience.
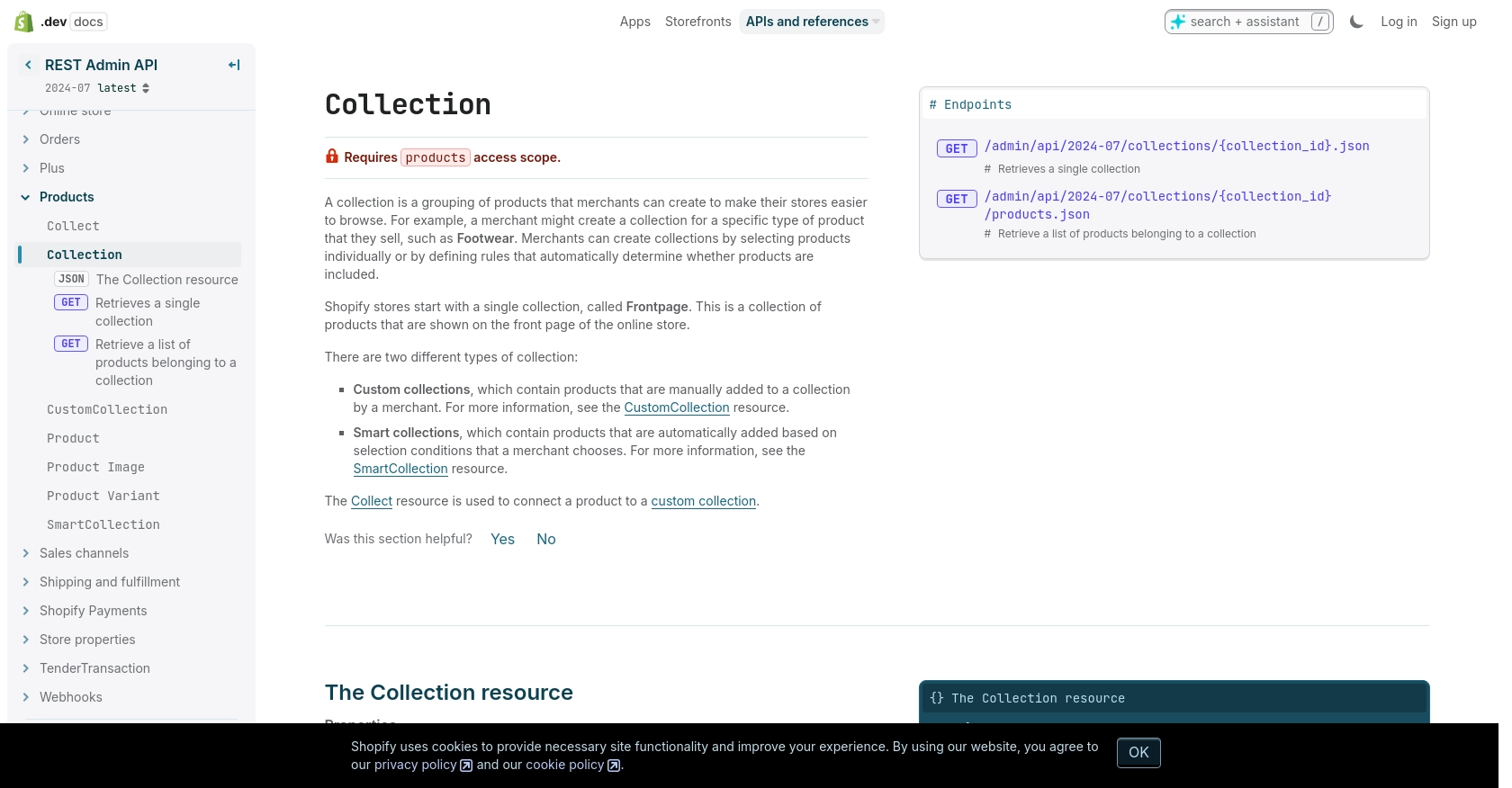
Conclusion and Best Practices for Shopify API Integration
Integrating with the Shopify API to create or update custom collections using JavaScript can significantly enhance your e-commerce store's functionality and user experience. By following the steps outlined in this article, developers can efficiently manage collections, ensuring a more organized and appealing storefront.
Best Practices for Secure and Efficient Shopify API Use
- Secure Storage of Credentials: Always store your API keys and access tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Shopify's API has a rate limit of 40 requests per app per store per minute. Implement logic to handle the
429 Too Many Requests
error by checking theRetry-After
header and pausing requests accordingly. - Data Standardization: Ensure that data fields are standardized and consistent to avoid errors and improve data integrity across your application.
Enhancing Your Integration Strategy with Endgrate
While integrating with Shopify's API provides powerful capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to multiple platforms, including Shopify. This allows developers to focus on core product development while outsourcing integration complexities.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration strategy by visiting Endgrate's website.
By adhering to these best practices and utilizing tools like Endgrate, you can optimize your Shopify API integrations, ensuring a robust and scalable e-commerce solution.
Read More
Ready to get started?