Using the Pipedrive API to Get People in PHP
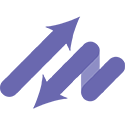
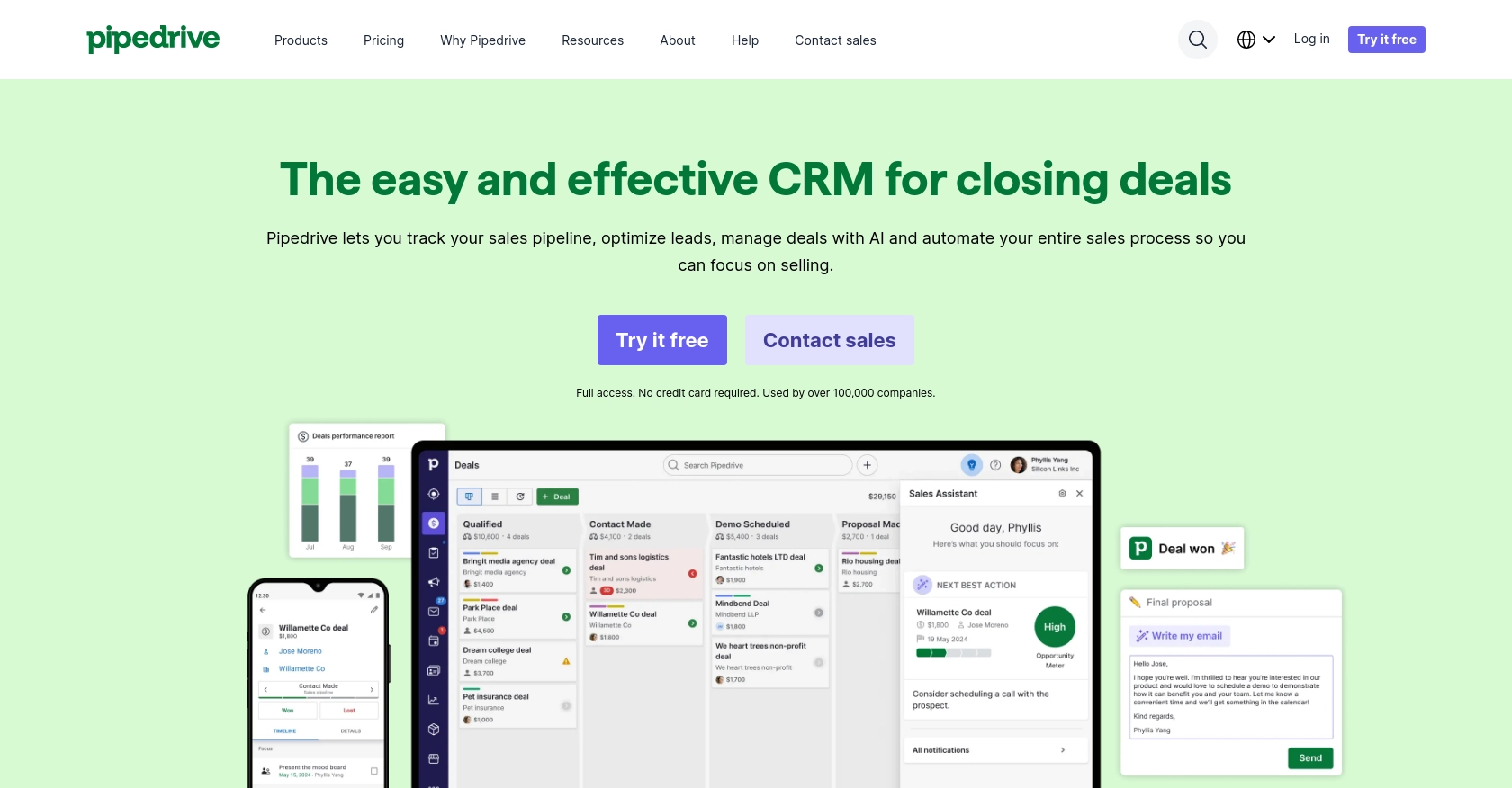
Introduction to Pipedrive CRM
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes more effectively. With its intuitive interface and robust features, Pipedrive enables sales teams to track leads, manage customer relationships, and close deals efficiently.
For developers, integrating with Pipedrive's API offers the opportunity to automate and enhance sales workflows. By connecting to Pipedrive, you can access and manage contact data, such as retrieving a list of people associated with your sales pipeline. This can be particularly useful for syncing contact information with other business tools or creating custom sales reports.
In this article, we'll explore how to use PHP to interact with the Pipedrive API, specifically focusing on retrieving people data. This integration can streamline your sales operations and provide valuable insights into your customer interactions.
Setting Up Your Pipedrive Developer Sandbox Account
Before you can start integrating with the Pipedrive API using PHP, you'll need to set up a developer sandbox account. This account provides a safe environment to test and develop your applications without affecting live data.
Creating a Pipedrive Developer Sandbox Account
To create a sandbox account, follow these steps:
- Visit the Pipedrive Developer Sandbox Account page.
- Fill out the form to request access to a sandbox account. This account is similar to a regular Pipedrive company account and allows you to perform testing in a risk-free environment.
- Once your sandbox account is set up, you can import sample data to familiarize yourself with the Pipedrive interface. Navigate to “...” (More) > Import data > From a spreadsheet in the Pipedrive web app to import template spreadsheets.
Creating a Pipedrive App for OAuth Authentication
Since the Pipedrive API uses OAuth 2.0 for authentication, you'll need to create an app to obtain the necessary credentials:
- Log in to your Pipedrive sandbox account and navigate to the Developer Hub.
- Register your app by providing the required details. This will generate a client ID and client secret, which are essential for the OAuth flow.
- Ensure your app has the necessary scopes and permissions to access the data you need. For retrieving people data, make sure to include the appropriate scopes related to contacts.
With your sandbox account and app set up, you're ready to start making API calls to Pipedrive using PHP. In the next section, we'll guide you through the process of retrieving people data from Pipedrive.
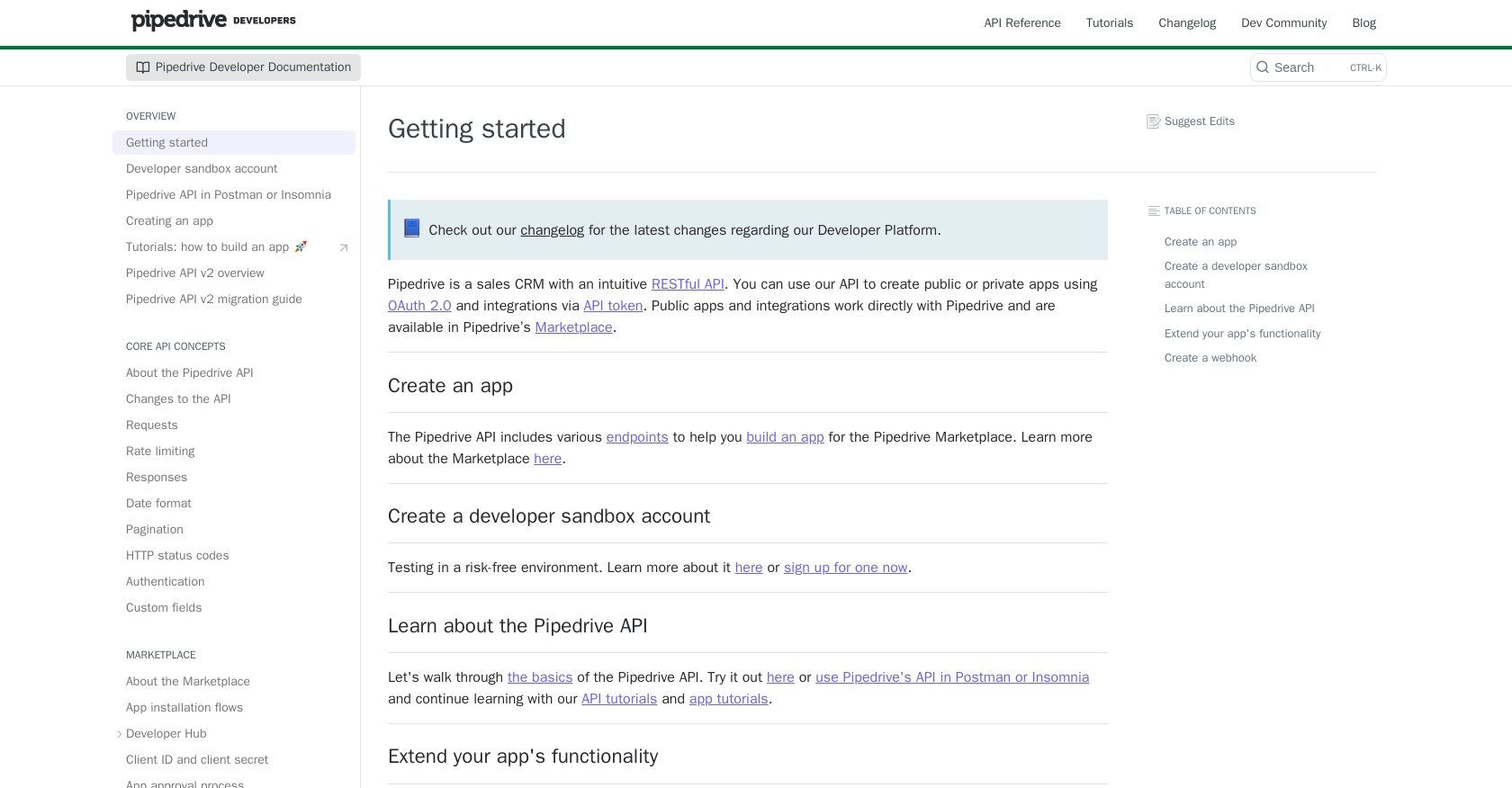
sbb-itb-96038d7
Making API Calls to Retrieve People Data from Pipedrive Using PHP
To interact with the Pipedrive API and retrieve people data using PHP, you'll need to set up your environment and write the necessary code to make API requests. This section will guide you through the process, including setting up PHP, installing dependencies, and writing the code to interact with the Pipedrive API.
Setting Up Your PHP Environment for Pipedrive API Integration
Before making API calls, ensure your PHP environment is correctly configured. You'll need:
- PHP 7.4 or later
- Composer for dependency management
Install the required dependencies using Composer. Run the following command in your terminal:
composer require guzzlehttp/guzzle
This command installs Guzzle, a PHP HTTP client that simplifies making API requests.
Writing PHP Code to Retrieve People Data from Pipedrive
With your environment set up, you can now write the PHP code to retrieve people data from Pipedrive. Create a new PHP file, for example, get_pipedrive_people.php
, and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize the Guzzle HTTP client
$client = new Client();
// Set the API endpoint and headers
$endpoint = 'https://api.pipedrive.com/v1/persons';
$accessToken = 'Your_Access_Token'; // Replace with your access token
$headers = [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json'
];
// Make a GET request to the API
$response = $client->request('GET', $endpoint, [
'headers' => $headers
]);
// Parse the JSON data from the response
$data = json_decode($response->getBody(), true);
// Check if the request was successful
if ($data['success']) {
foreach ($data['data'] as $person) {
echo 'Name: ' . $person['name'] . '<br>';
}
} else {
echo 'Failed to retrieve people data.';
}
Replace Your_Access_Token
with the access token obtained from your Pipedrive app setup. This code initializes a Guzzle client, sets the necessary headers, and makes a GET request to the Pipedrive API to retrieve people data. If successful, it will print the names of the people retrieved.
Verifying the API Request and Handling Errors
After running the script, verify the output by checking the list of people displayed. If the request fails, ensure your access token is correct and that your app has the necessary permissions.
Handle potential errors by checking the response status code and error messages. The Pipedrive API uses standard HTTP status codes to indicate success or failure:
- 200 OK: Request was successful.
- 401 Unauthorized: Invalid access token.
- 429 Too Many Requests: Rate limit exceeded.
For more detailed error handling, refer to the Pipedrive HTTP Status Codes documentation.
Checking API Rate Limits
Be mindful of Pipedrive's rate limits to avoid exceeding them. The rate limit for OAuth apps using access tokens is 80 requests per 2 seconds for the Essential plan. For more details, see the Pipedrive Rate Limiting documentation.
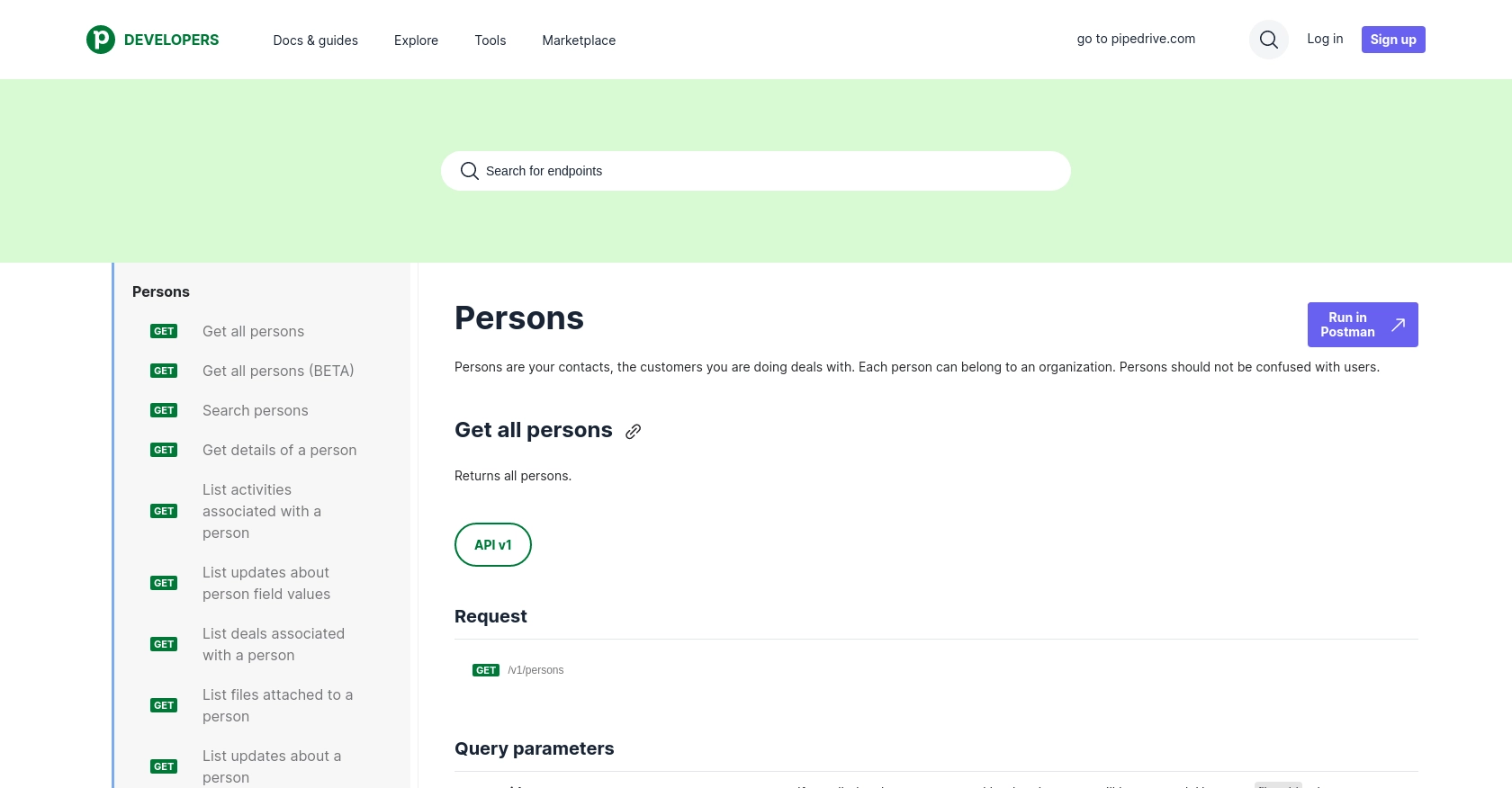
Conclusion and Best Practices for Using Pipedrive API with PHP
Integrating with the Pipedrive API using PHP can significantly enhance your sales operations by automating data retrieval and management. By following the steps outlined in this article, you can efficiently access and manipulate people data within your Pipedrive account, providing valuable insights and streamlining your sales processes.
Best Practices for Secure and Efficient Pipedrive API Integration
- Securely Store Credentials: Always store your access tokens and client secrets securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limits Gracefully: Be aware of Pipedrive's rate limits and implement logic to handle HTTP 429 errors. Consider using exponential backoff strategies to retry requests.
- Optimize Data Handling: Use pagination to efficiently handle large datasets and reduce the load on your application and Pipedrive's servers.
- Regularly Update Tokens: Ensure your OAuth tokens are refreshed regularly to maintain uninterrupted access to the API.
Enhancing Your Integration Experience with Endgrate
While integrating with Pipedrive directly can be powerful, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management across various platforms, including Pipedrive.
By using Endgrate, you can:
- Save time and resources by outsourcing integration maintenance.
- Build once for each use case and leverage the same integration across multiple platforms.
- Provide an intuitive integration experience for your customers, enhancing their satisfaction and engagement.
Explore how Endgrate can streamline your integration processes by visiting Endgrate and discover the benefits of a unified API approach.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Persons
- https://developers.pipedrive.com/docs/api/v1/PersonFields
Ready to get started?