Using the Quickbooks API to Get Purchase Orders (with PHP examples)
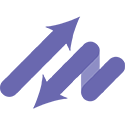
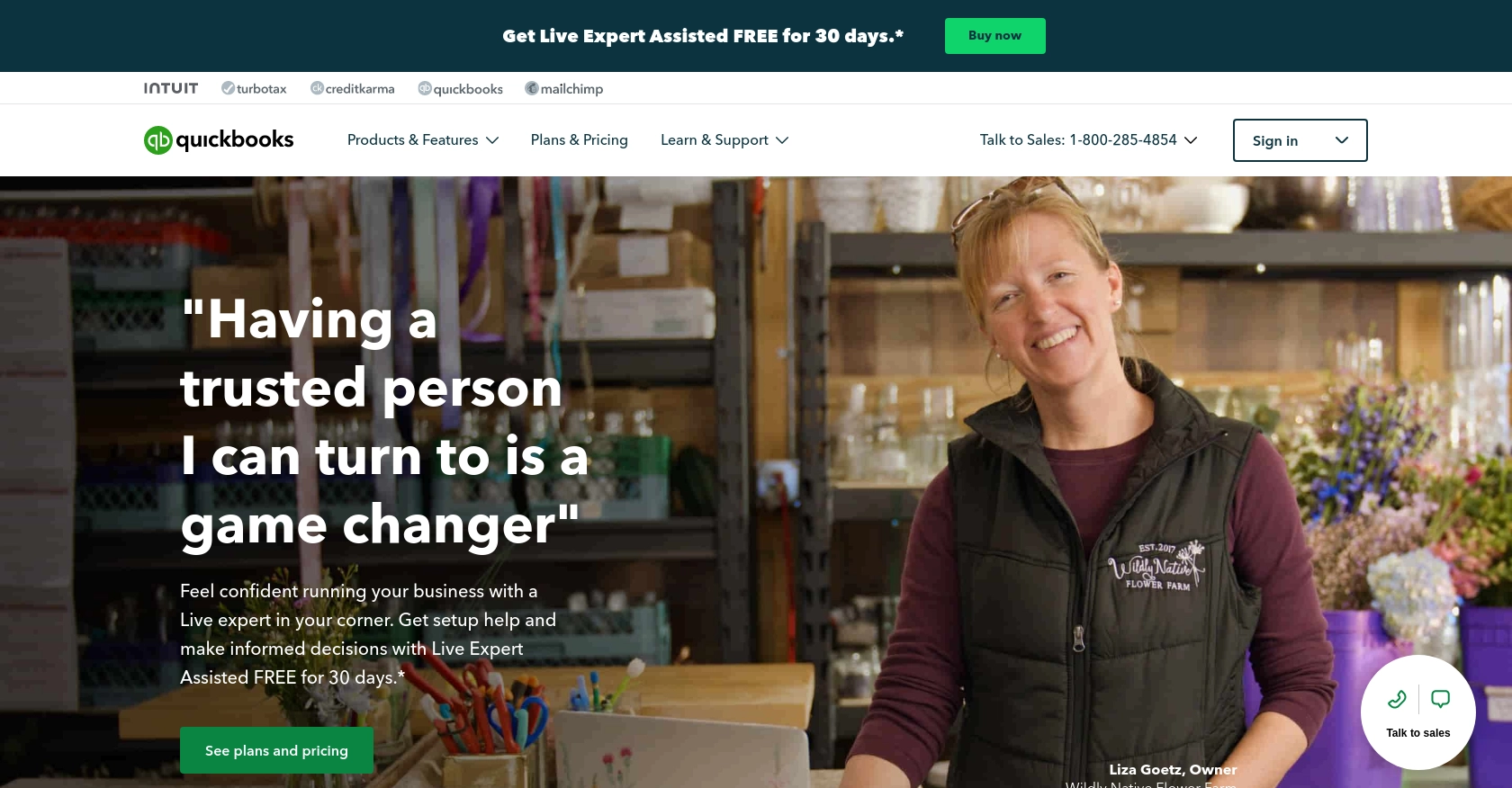
Introduction to QuickBooks API
QuickBooks is a widely-used accounting software that offers comprehensive financial management solutions for businesses of all sizes. It provides tools for invoicing, expense tracking, payroll, and more, making it an essential platform for managing business finances efficiently.
Integrating with the QuickBooks API allows developers to automate and streamline financial processes, such as retrieving purchase orders. For example, a developer might use the QuickBooks API to automatically fetch purchase order data and integrate it into a custom reporting tool, enhancing financial visibility and decision-making.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start interacting with the QuickBooks API, you'll need to set up a sandbox account. This environment allows you to test your integration without affecting live data, ensuring a smooth development process.
Creating a QuickBooks Developer Account
To begin, you'll need a QuickBooks Developer account. Follow these steps to create one:
- Visit the Intuit Developer Portal.
- Click on "Sign Up" and fill in the required information to create your account.
- Once registered, log in to your developer account.
Setting Up a QuickBooks Sandbox Company
After creating your developer account, you need to set up a sandbox company:
- Navigate to the "Dashboard" in your developer account.
- Select "Sandbox" from the menu and click on "Add Sandbox Company."
- Follow the prompts to create a new sandbox company, which will be used for testing your API integration.
Creating a QuickBooks App for OAuth Authentication
To interact with the QuickBooks API, you'll need to create an app to obtain the necessary OAuth credentials:
- Go to the "My Apps" section in your developer dashboard.
- Click on "Create an App" and choose "QuickBooks Online and Payments."
- Fill in the app details, such as name and description.
- Once the app is created, navigate to the "Keys & OAuth" section to find your Client ID and Client Secret.
These credentials are essential for authenticating API requests. Ensure you store them securely.
Configuring OAuth Scopes for Access
To access purchase orders, you'll need to configure the appropriate OAuth scopes:
- In your app settings, go to the "Scopes" section.
- Select the necessary scopes that allow access to purchase orders and other relevant data.
- Save your changes to update the app's permissions.
With your sandbox account and app set up, you're ready to start making API calls to QuickBooks. For more detailed instructions, refer to the Intuit Developer Documentation.
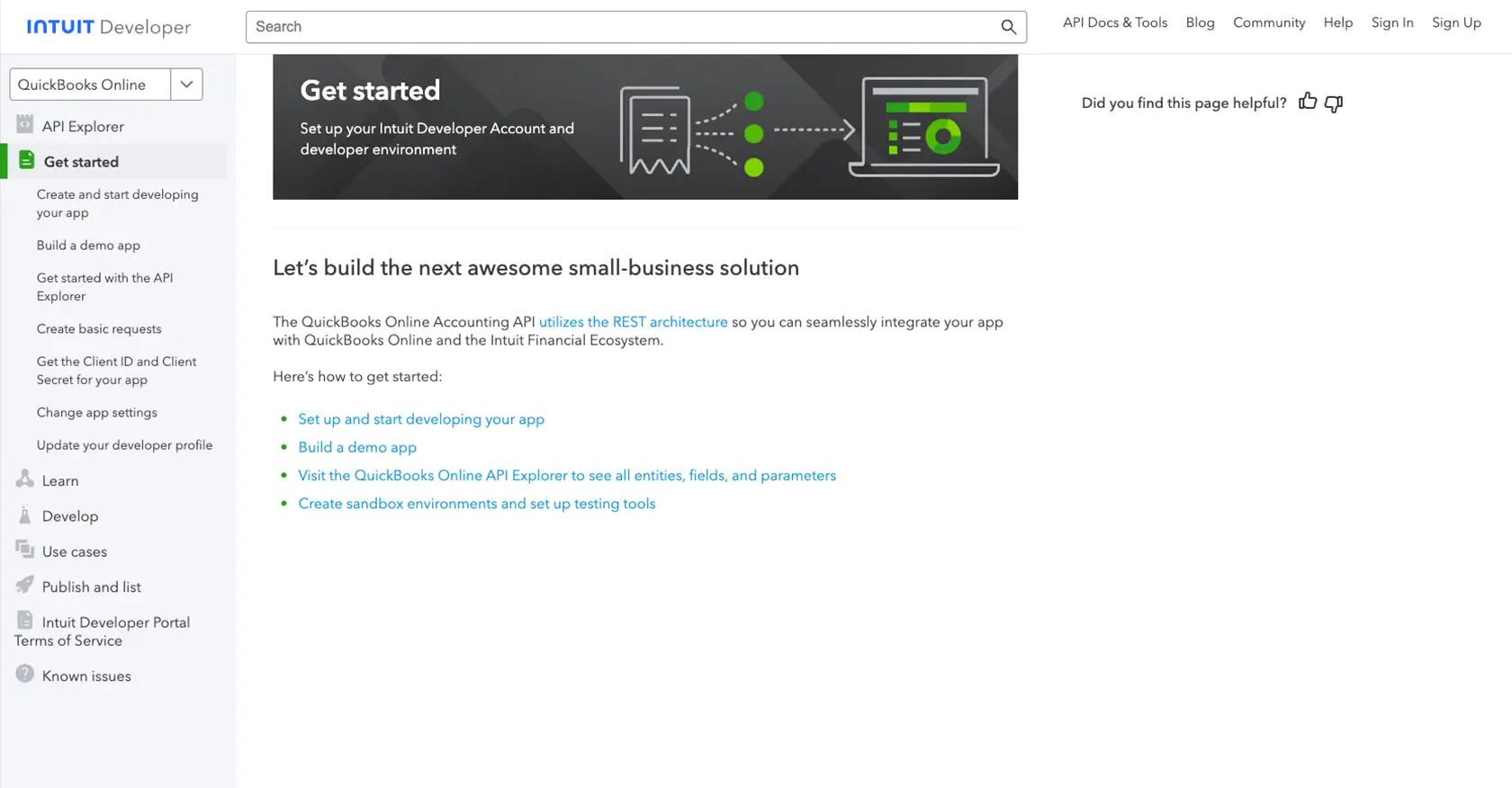
sbb-itb-96038d7
Making API Calls to QuickBooks to Retrieve Purchase Orders Using PHP
To interact with the QuickBooks API and retrieve purchase orders, you'll need to set up your PHP environment and write the necessary code to make API calls. This section will guide you through the process, including setting up your PHP environment, writing the code, and handling potential errors.
Setting Up Your PHP Environment for QuickBooks API Integration
Before making API calls, ensure your development environment is ready:
- Install PHP version 7.4 or higher.
- Ensure you have Composer installed for managing dependencies.
- Install the
guzzlehttp/guzzle
package for making HTTP requests by running the following command:
composer require guzzlehttp/guzzle
Writing PHP Code to Fetch Purchase Orders from QuickBooks
With your environment set up, you can now write the PHP code to interact with the QuickBooks API:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your OAuth access token
$realmId = 'Your_Realm_ID'; // Replace with your QuickBooks company ID
$url = "https://quickbooks.api.intuit.com/v3/company/$realmId/query";
$query = "SELECT * FROM PurchaseOrder";
$response = $client->request('POST', $url, [
'headers' => [
'Authorization' => "Bearer $accessToken",
'Accept' => 'application/json',
'Content-Type' => 'application/text'
],
'body' => $query
]);
$data = json_decode($response->getBody(), true);
foreach ($data['QueryResponse']['PurchaseOrder'] as $purchaseOrder) {
echo "Purchase Order ID: " . $purchaseOrder['Id'] . "\n";
echo "Vendor: " . $purchaseOrder['VendorRef']['name'] . "\n";
echo "Total Amount: " . $purchaseOrder['TotalAmt'] . "\n\n";
}
?>
Replace Your_Access_Token
and Your_Realm_ID
with your actual OAuth access token and QuickBooks company ID, respectively. This script uses Guzzle to send a POST request to the QuickBooks API, querying for all purchase orders.
Verifying API Call Success and Handling Errors
After running the script, you should see the purchase order details printed in your terminal. To verify the success of your API call, check the response status code:
- A status code of
200
indicates a successful request. - If you encounter errors, refer to the QuickBooks API documentation for error codes and troubleshooting tips. Common errors include invalid tokens or insufficient permissions.
For more details on handling errors, consult the Intuit Developer Documentation.
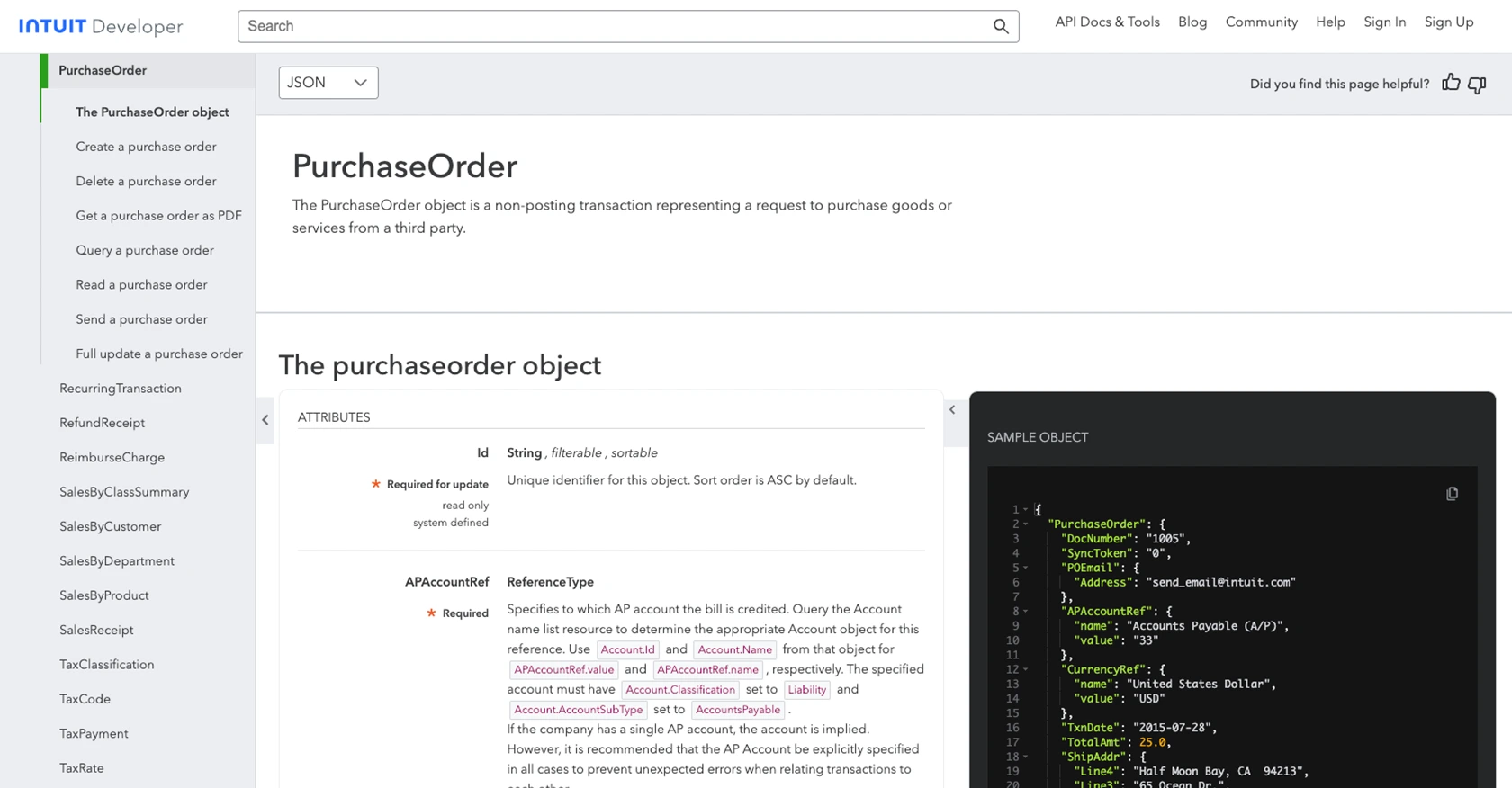
Conclusion and Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API to retrieve purchase orders using PHP can significantly enhance your financial management processes by automating data retrieval and improving reporting capabilities. However, to ensure a successful integration, it's crucial to follow best practices and handle potential challenges effectively.
Best Practices for Storing QuickBooks API Credentials Securely
When dealing with sensitive information such as OAuth credentials, it's essential to store them securely. Consider the following practices:
- Use environment variables to store your Client ID, Client Secret, and Access Token, keeping them out of your source code.
- Implement encryption for any stored credentials to prevent unauthorized access.
- Regularly rotate your credentials and update your application accordingly.
Handling QuickBooks API Rate Limiting
QuickBooks API imposes rate limits to ensure fair usage. To manage these limits effectively:
- Monitor your API usage and implement logic to handle rate limit responses gracefully.
- Consider implementing exponential backoff strategies to retry requests after hitting rate limits.
- Refer to the Intuit Developer Documentation for specific rate limit details.
Transforming and Standardizing Data from QuickBooks API
When integrating QuickBooks data into your systems, ensure that data is transformed and standardized to match your application's requirements:
- Map QuickBooks data fields to your internal data structures for consistency.
- Implement validation checks to ensure data integrity and accuracy.
- Consider using middleware or data transformation libraries to streamline this process.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. With Endgrate, you can:
- Save time and resources by outsourcing integration management, allowing you to focus on your core product.
- Build once for each use case, reducing the need for multiple integration efforts.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can streamline your integration processes by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/purchaseorder
Ready to get started?