How to Create Leads with the Zoho CRM API in Javascript
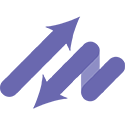
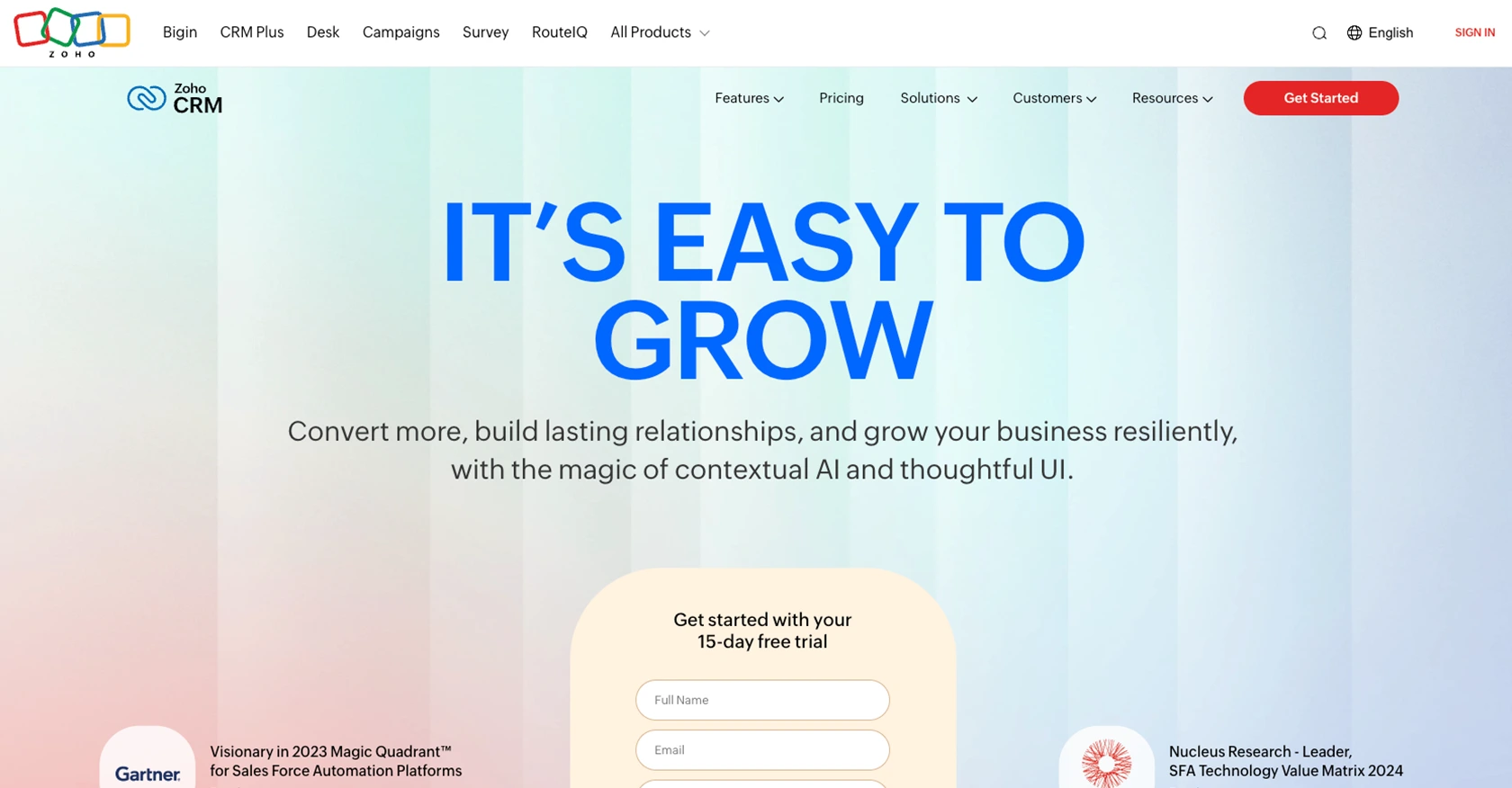
Introduction to Zoho CRM
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and customer support in one place. Known for its flexibility and scalability, Zoho CRM is a popular choice for businesses looking to enhance their customer interactions and streamline operations.
Developers often integrate with Zoho CRM to automate and optimize various business processes. By leveraging the Zoho CRM API, developers can create, update, and manage leads efficiently. For example, a developer might use the Zoho CRM API to automatically generate leads from a website form submission, ensuring that potential customers are promptly added to the sales pipeline.
Setting Up Your Zoho CRM Sandbox Account
Before you can start creating leads with the Zoho CRM API in JavaScript, you need to set up a sandbox account. This allows you to test API interactions without affecting your live data. Follow these steps to get started:
Create a Zoho CRM Developer Account
- Visit the Zoho Developer Console.
- Sign up for a developer account if you don't have one. This will give you access to the necessary tools and resources.
Register Your Application for OAuth Authentication
Zoho CRM uses OAuth 2.0 for authentication. To interact with the API, you need to register your application:
- In the Zoho Developer Console, choose the client type that suits your application (e.g., JavaScript).
- Enter the following details:
- Client Name: The name of your application.
- Homepage URL: The URL of your web page.
- Authorized Redirect URIs: A valid URL where Zoho will redirect after successful authentication.
- Click Create to generate your Client ID and Client Secret.
Generate Access and Refresh Tokens
Once your application is registered, you need to generate tokens to authenticate API requests:
- Make an authorization request to obtain an authorization code.
- Exchange the authorization code for access and refresh tokens. Refer to the Zoho CRM OAuth Overview for detailed steps.
Configure API Scopes
Ensure your application has the necessary scopes to create leads. Use the following scope format:
scope=ZohoCRM.modules.leads.CREATE
For more information on scopes, visit the Zoho CRM Scopes Documentation.
Test Your Setup
With your sandbox account and application configured, you can now test API calls to ensure everything is working correctly. Use the sandbox environment to verify lead creation without impacting live data.
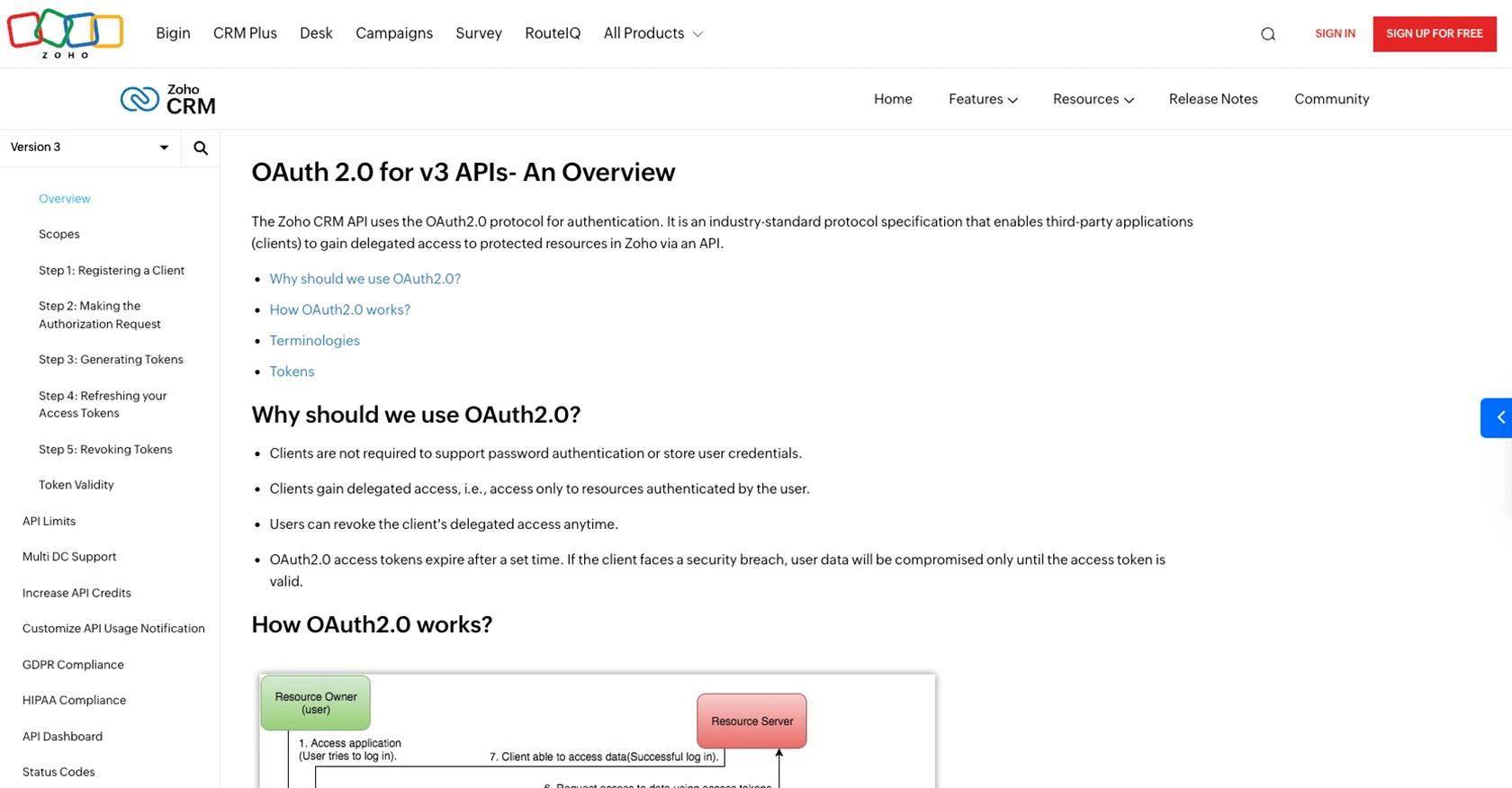
sbb-itb-96038d7
Making the Zoho CRM API Call to Create Leads Using JavaScript
To create leads in Zoho CRM using JavaScript, you'll need to interact with the Zoho CRM API. This section will guide you through the process of setting up your environment, writing the code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for Zoho CRM API
Before making API calls, ensure your development environment is ready:
- Ensure you have Node.js installed, as it provides a runtime for executing JavaScript code outside the browser.
- Use a package manager like npm to install necessary dependencies, such as the
axios
library for making HTTP requests.
npm install axios
Writing the JavaScript Code to Create Leads in Zoho CRM
Below is a sample code snippet to create a lead in Zoho CRM using the API:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://www.zohoapis.com/crm/v3/Leads';
const headers = {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Define the lead data
const leadData = {
data: [
{
Last_Name: 'Doe',
First_Name: 'John',
Company: 'Example Corp',
Email: 'john.doe@example.com'
}
]
};
// Make the POST request to create a lead
axios.post(endpoint, leadData, { headers })
.then(response => {
console.log('Lead created successfully:', response.data);
})
.catch(error => {
console.error('Error creating lead:', error.response.data);
});
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth authentication process.
Verifying Successful Lead Creation in Zoho CRM
After running the code, check your Zoho CRM sandbox account to verify that the lead has been created. You should see the new lead listed in the Leads module.
Handling Errors and Understanding Zoho CRM API Response Codes
It's crucial to handle errors gracefully and understand the API response codes:
- 200 OK: The request was successful, and the lead was created.
- 400 Bad Request: The request was malformed. Check the request body and headers.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 429 Too Many Requests: Rate limit exceeded. Implement retry logic or reduce request frequency.
For more detailed error handling, refer to the Zoho CRM Status Codes Documentation.
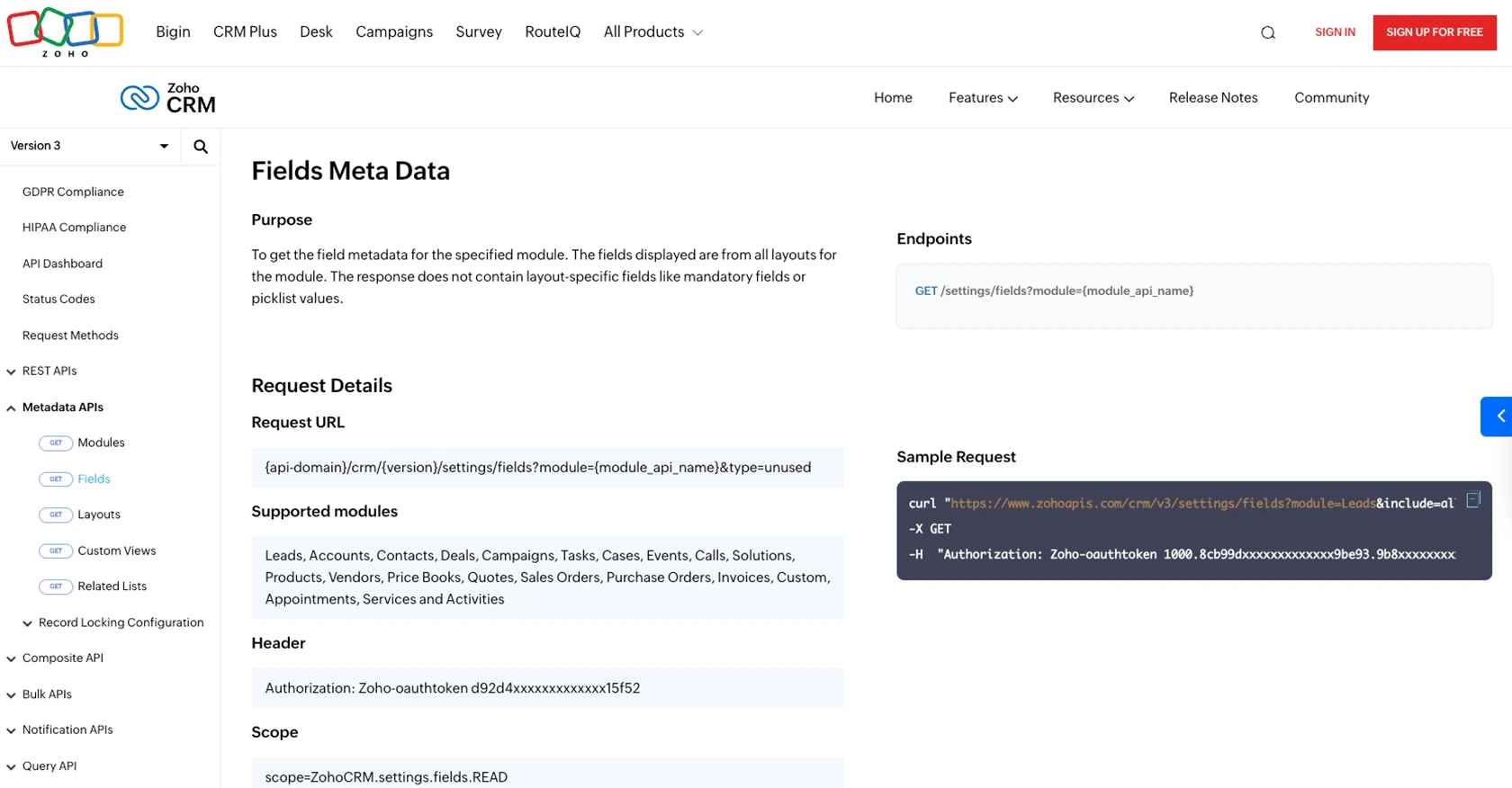
Best Practices for Using Zoho CRM API in JavaScript
When working with the Zoho CRM API, it's essential to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Zoho CRM enforces rate limits to ensure fair usage. Implement retry logic with exponential backoff to handle
429 Too Many Requests
errors gracefully. For more details, refer to the Zoho CRM API Limits Documentation. - Data Standardization: Ensure that data fields are standardized before sending them to Zoho CRM. This helps maintain consistency and accuracy across your CRM records.
- Monitor API Usage: Regularly monitor your API usage and performance. Use logging and analytics tools to track API calls and identify any potential issues.
Streamline Your Integrations with Endgrate
Integrating with multiple platforms can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoho CRM. With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/search-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/insert-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/update-records.html
Ready to get started?