Using the Pipeliner CRM API to Create Or Update Contacts in Python
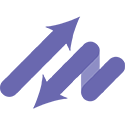
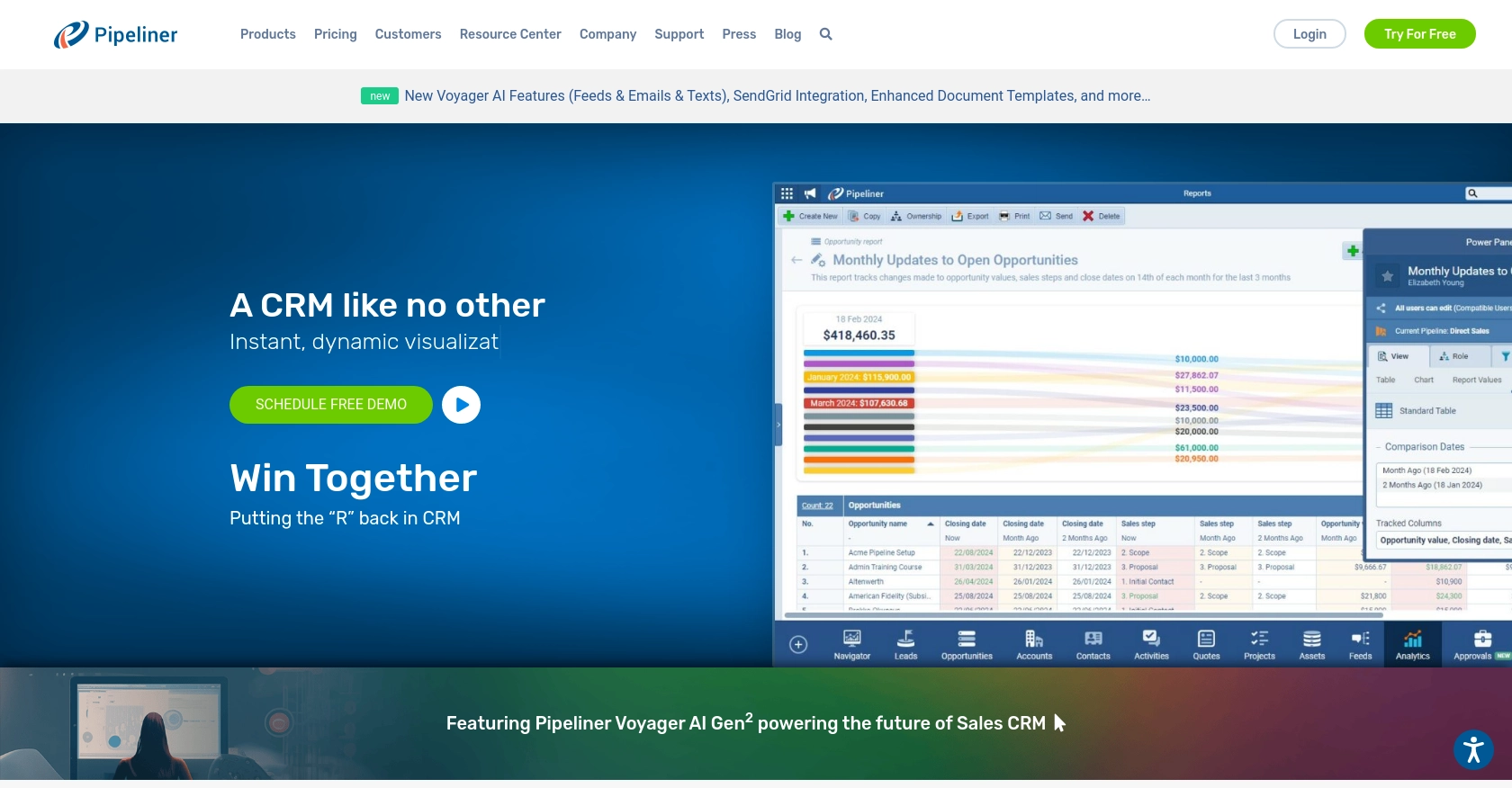
Introduction to Pipeliner CRM
Pipeliner CRM is a powerful customer relationship management tool designed to enhance sales processes and improve business efficiency. With its user-friendly interface and robust features, Pipeliner CRM helps businesses manage contacts, track opportunities, and streamline workflows.
Integrating with Pipeliner CRM's API allows developers to automate and optimize CRM tasks, such as creating or updating contact information. For example, a developer might use the Pipeliner CRM API to automatically update contact details from an external data source, ensuring that the sales team always has access to the most current information.
Setting Up Your Pipeliner CRM Test Account
Before you can start integrating with the Pipeliner CRM API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Pipeliner CRM Account
If you don't already have a Pipeliner CRM account, follow these steps to create one:
- Visit the Pipeliner CRM website and sign up for an account.
- Log in to your account and select your team space.
Generating Pipeliner CRM API Keys
To interact with the Pipeliner CRM API, you'll need to generate API keys. These keys will serve as your username and password for Basic Authentication.
- Navigate to the administration section of your Pipeliner CRM space.
- Under the "Unit, Users & Roles" tab, open "Applications."
- Create a new application and click "Show API Access" to generate your API keys.
- Store these keys securely, as they are generated only once and are essential for API access.
For more details, refer to the Pipeliner CRM Authentication Documentation.
Understanding Pipeliner CRM API Authentication
Pipeliner CRM uses Basic Authentication, which requires the API keys you generated. Each request to the API must include these keys as the username and password.
Additionally, every API request must include the Space ID and Service URL, which are specific to your Pipeliner CRM space.
Testing Your Pipeliner CRM API Setup
Once you have your API keys, you can test your setup by making a simple API call. This will ensure that your authentication is correctly configured.
import requests
# Replace with your actual API keys and space details
username = 'Your_API_Username'
password = 'Your_API_Password'
space_id = 'Your_Space_ID'
service_url = 'Your_Service_URL'
# Example API endpoint
endpoint = f"{service_url}/entities/Contacts"
# Make a GET request to test authentication
response = requests.get(endpoint, auth=(username, password))
# Check if the request was successful
if response.status_code == 200:
print("Authentication successful!")
else:
print("Authentication failed. Please check your API keys and space details.")
By following these steps, you'll be ready to start integrating with the Pipeliner CRM API using Python. For more information on API calls, refer to the Pipeliner CRM API Documentation.
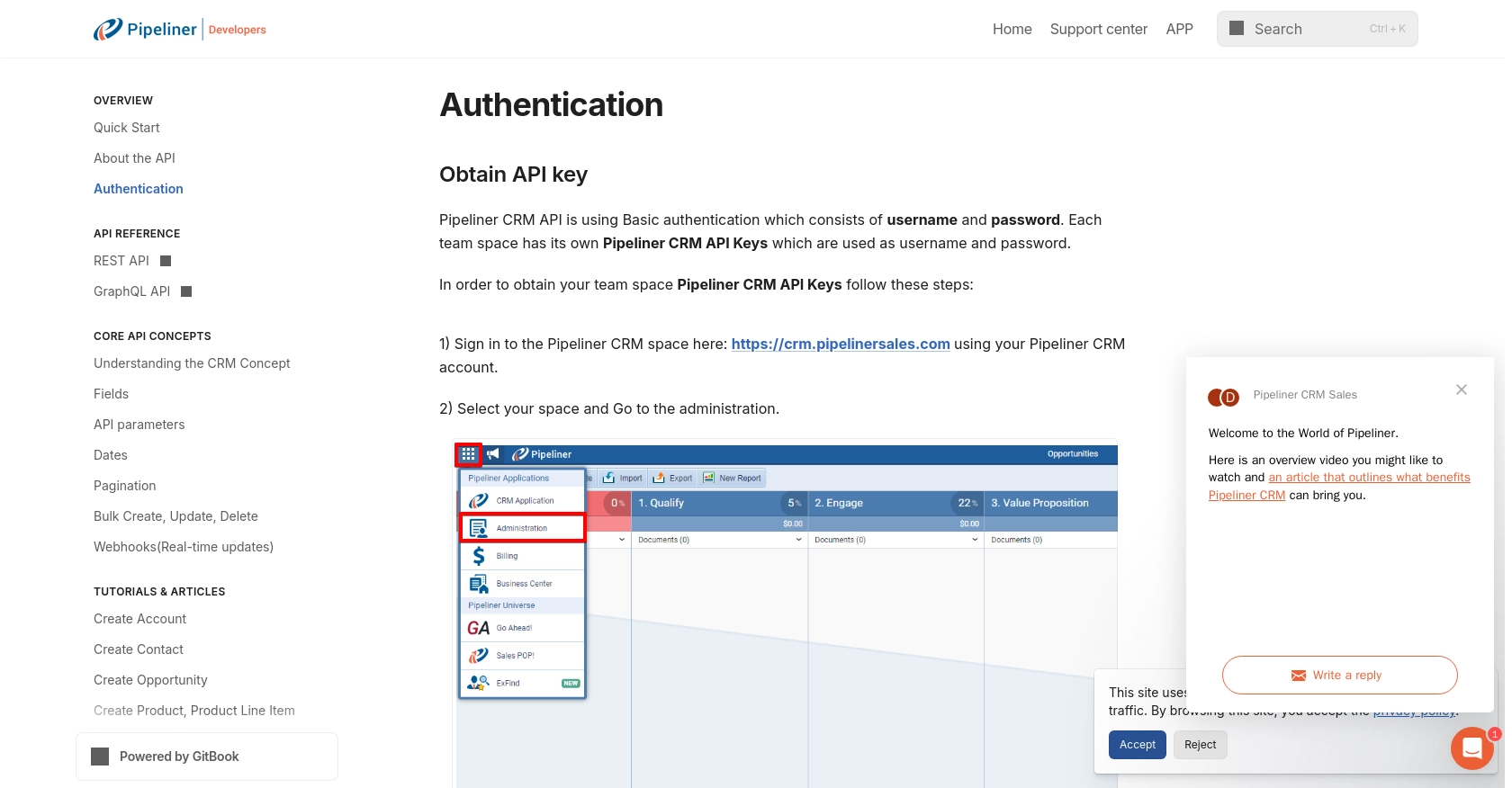
sbb-itb-96038d7
Making API Calls to Pipeliner CRM Using Python
With your Pipeliner CRM test account set up and API keys generated, you can now proceed to make API calls to create or update contacts. This section will guide you through the process using Python, ensuring you have the necessary tools and knowledge to interact with the Pipeliner CRM API effectively.
Setting Up Your Python Environment for Pipeliner CRM API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Creating or Updating Contacts in Pipeliner CRM
To create or update contacts in Pipeliner CRM, you'll need to make POST requests to the appropriate API endpoints. Below is an example of how to create a contact using Python:
import requests
import json
# Replace with your actual API keys and space details
username = 'Your_API_Username'
password = 'Your_API_Password'
space_id = 'Your_Space_ID'
service_url = 'Your_Service_URL'
# Set the API endpoint for creating a contact
create_contact_endpoint = f"{service_url}/entities/Contacts"
# Define the contact data
contact_data = {
"firstName": "John",
"lastName": "Doe",
"email": "john.doe@example.com"
}
# Make a POST request to create the contact
response = requests.post(
create_contact_endpoint,
auth=(username, password),
headers={"Content-Type": "application/json"},
data=json.dumps(contact_data)
)
# Check if the request was successful
if response.status_code == 201:
print("Contact created successfully!")
else:
print(f"Failed to create contact: {response.status_code} - {response.text}")
Replace Your_API_Username
, Your_API_Password
, Your_Space_ID
, and Your_Service_URL
with your actual credentials and space details. The example above demonstrates how to send a POST request to create a new contact in Pipeliner CRM.
Verifying API Call Success in Pipeliner CRM
After executing the API call, verify the success by checking the response status code. A status code of 201 indicates that the contact was created successfully. You can also log in to your Pipeliner CRM account to confirm that the new contact appears in your contact list.
Handling Errors and Understanding Error Codes
When making API calls, it's crucial to handle potential errors gracefully. If the request fails, the API will return an error code and message. Common error codes include 400 for bad requests and 401 for unauthorized access. Ensure your API keys and request data are correct to avoid these errors.
For more detailed information on error handling, refer to the Pipeliner CRM Authentication Documentation.
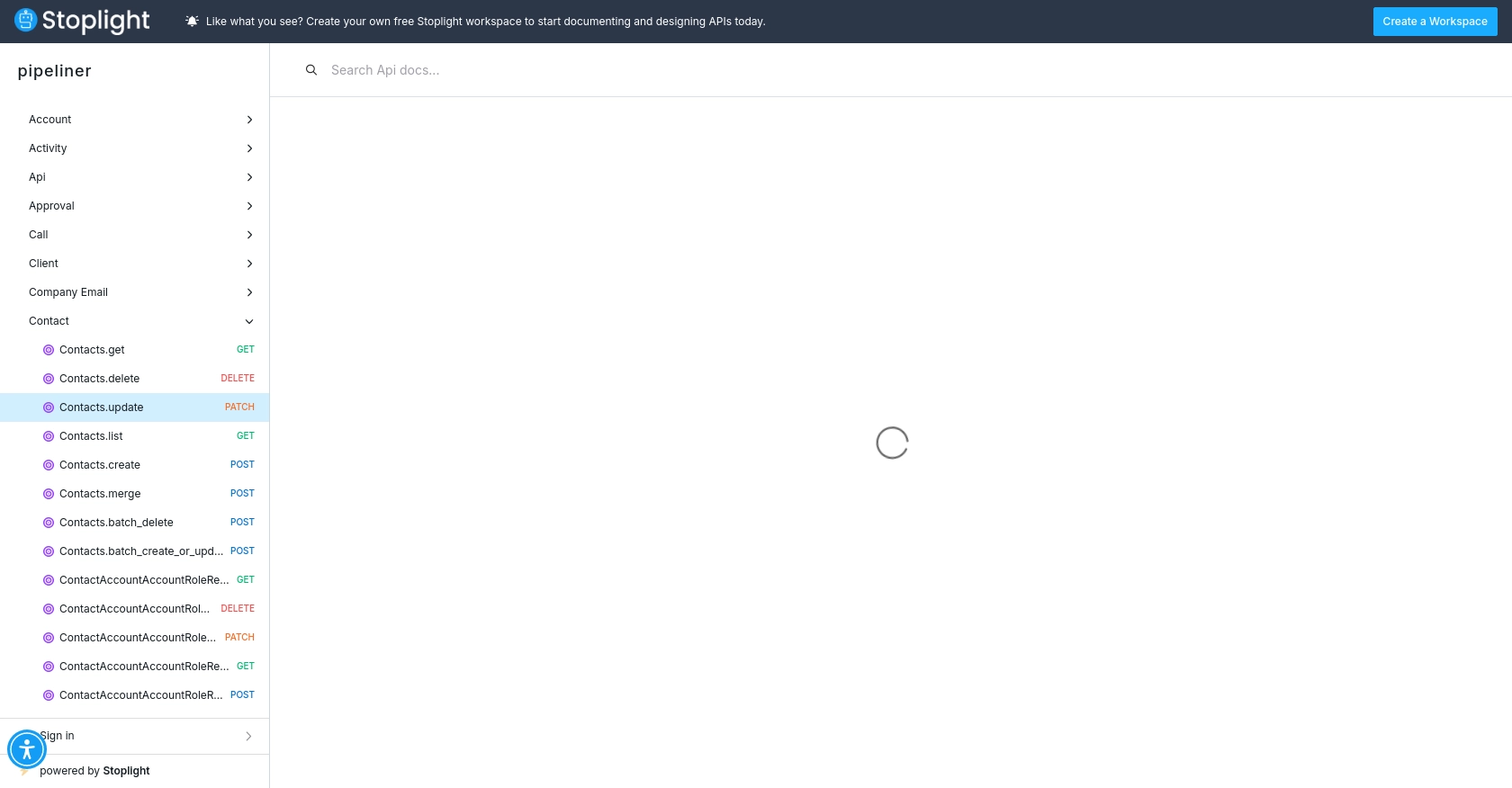
Conclusion and Best Practices for Pipeliner CRM API Integration
Integrating with the Pipeliner CRM API using Python allows developers to efficiently manage contact data, ensuring that sales teams have access to the most up-to-date information. By following the steps outlined in this guide, you can create or update contacts seamlessly within Pipeliner CRM.
Best Practices for Secure and Efficient Pipeliner CRM API Usage
- Securely Store API Credentials: Always store your API keys and credentials securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of API rate limits to avoid service disruptions. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data fields are standardized before making API calls. This helps maintain consistency and accuracy across your CRM data.
- Error Handling: Implement robust error handling to manage API call failures. Log errors and provide meaningful feedback to users or systems that rely on the integration.
Streamlining Integrations with Endgrate
While integrating with Pipeliner CRM directly is powerful, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including Pipeliner CRM. With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/pipelinercrm
- https://developers.pipelinersales.com/api-docs/overview/authentication
- https://developers.pipelinersales.com/api-docs/core-api-concepts/pagination
- https://pipeliner.stoplight.io/docs/api-docs/ah9798x4qjh7r-contacts-update
- https://pipeliner.stoplight.io/docs/api-docs/hds5thtgx28ti-contacts-create
Ready to get started?