Using the Xero API to Create or Update Customers in PHP
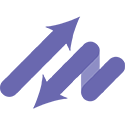
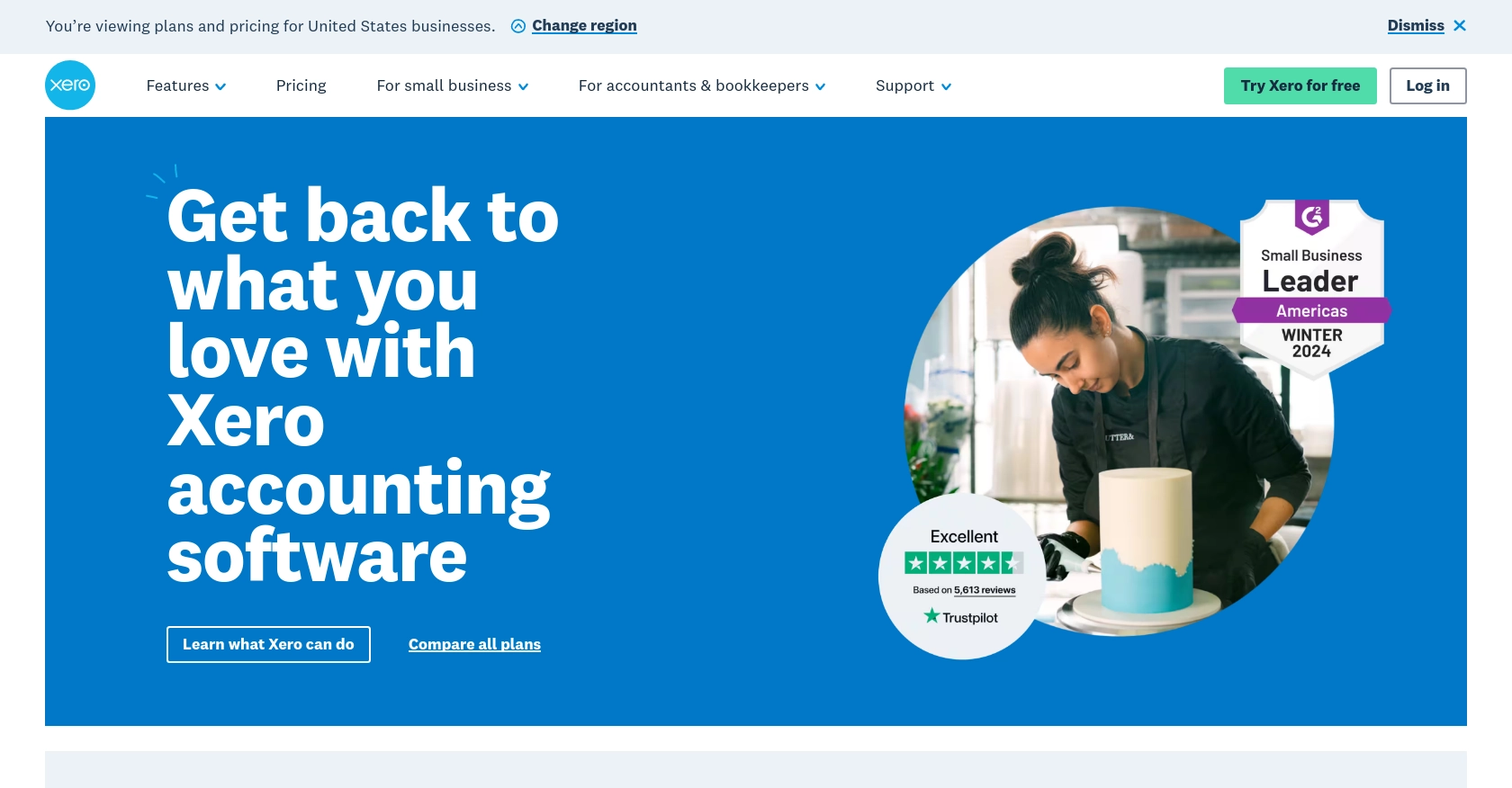
Introduction to Xero API Integration
Xero is a powerful cloud-based accounting software platform designed to meet the needs of small to medium-sized businesses. It offers a wide range of features, including invoicing, payroll, expense management, and financial reporting, making it a popular choice for businesses looking to streamline their accounting processes.
Integrating with Xero's API allows developers to automate and enhance accounting workflows by programmatically accessing and managing financial data. For example, a developer might use the Xero API to create or update customer records directly from a custom CRM system, ensuring that customer information is always up-to-date and synchronized across platforms.
Setting Up Your Xero Test/Sandbox Account for API Integration
Before diving into the integration process, it's essential to set up a Xero test or sandbox account. This environment allows developers to experiment with the Xero API without affecting live data, providing a safe space to test and refine their applications.
Creating a Xero Developer Account
To begin, you'll need a Xero developer account. Follow these steps to create one:
- Visit the Xero Developer Portal.
- Click on "Sign Up" and fill in the required details to create your account.
- Once registered, log in to access the developer dashboard.
Setting Up a Xero Sandbox Organization
With your developer account ready, the next step is to set up a sandbox organization:
- Navigate to the "My Apps" section in the developer dashboard.
- Select "Add a new app" and choose "Private App" for testing purposes.
- Fill in the app details, such as the app name and company URL.
- Under "Organization", select "Create a new organization" to set up your sandbox environment.
Configuring OAuth 2.0 Authentication
Xero uses OAuth 2.0 for secure API authentication. Follow these steps to configure it:
- In your app settings, navigate to the "OAuth 2.0" section.
- Generate your client ID and client secret, which will be used for authentication.
- Set up the redirect URI to handle the OAuth callback.
- Ensure you have the necessary scopes for accessing customer data, such as "accounting.contacts".
For more detailed information on OAuth 2.0, refer to the Xero OAuth 2.0 Documentation.
Obtaining Access Tokens
With OAuth 2.0 configured, you can now obtain access tokens to authenticate API requests:
- Use the authorization code flow to request an access token.
- Exchange the authorization code for an access token and refresh token.
- Store these tokens securely, as they will be used to authenticate your API calls.
Refer to the Xero OAuth 2.0 Auth Flow Guide for detailed steps.
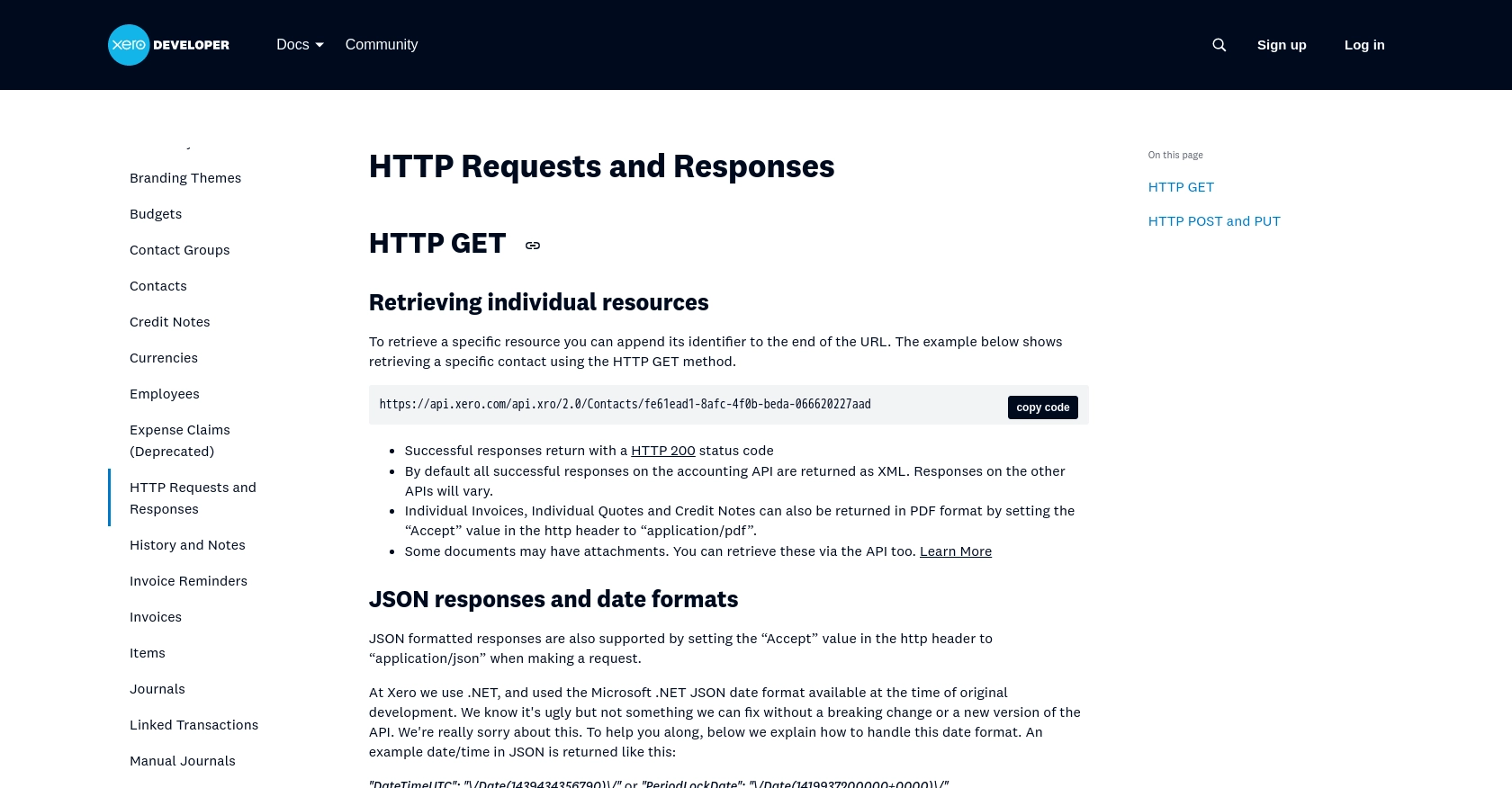
sbb-itb-96038d7
Making API Calls to Xero for Customer Management Using PHP
To interact with the Xero API for creating or updating customer records, you'll need to use PHP. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your PHP Environment for Xero API Integration
Before making API calls, ensure your PHP environment is correctly configured. You'll need:
- PHP 7.4 or later
- Composer for dependency management
- The
guzzlehttp/guzzle
package for making HTTP requests
Install the Guzzle package using Composer with the following command:
composer require guzzlehttp/guzzle
Creating or Updating Customers with Xero API in PHP
Once your environment is set up, you can proceed to write the PHP code to interact with the Xero API. Below is an example of how to create or update a customer record.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'YOUR_ACCESS_TOKEN';
$tenantId = 'YOUR_TENANT_ID';
$headers = [
'Authorization' => 'Bearer ' . $accessToken,
'Xero-tenant-id' => $tenantId,
'Content-Type' => 'application/json'
];
$body = [
'Name' => 'New Customer',
'EmailAddress' => 'customer@example.com',
'ContactStatus' => 'ACTIVE'
];
$response = $client->post('https://api.xero.com/api.xro/2.0/Contacts', [
'headers' => $headers,
'json' => $body
]);
if ($response->getStatusCode() == 200) {
echo "Customer created or updated successfully.";
} else {
echo "Failed to create or update customer.";
}
Replace YOUR_ACCESS_TOKEN
and YOUR_TENANT_ID
with your actual access token and tenant ID obtained during the OAuth 2.0 authentication process.
Verifying API Call Success and Handling Errors
After executing the API call, verify the success of the operation by checking the response status code. A status code of 200 indicates success. You can also log the response body for further inspection.
Handle potential errors by checking for other status codes and implementing error handling logic. Refer to the Xero API documentation for detailed error code descriptions.
Testing Your API Calls in the Xero Sandbox
To ensure your API calls are working as expected, test them in the Xero sandbox environment. Check the sandbox for the newly created or updated customer records to confirm the API interaction was successful.
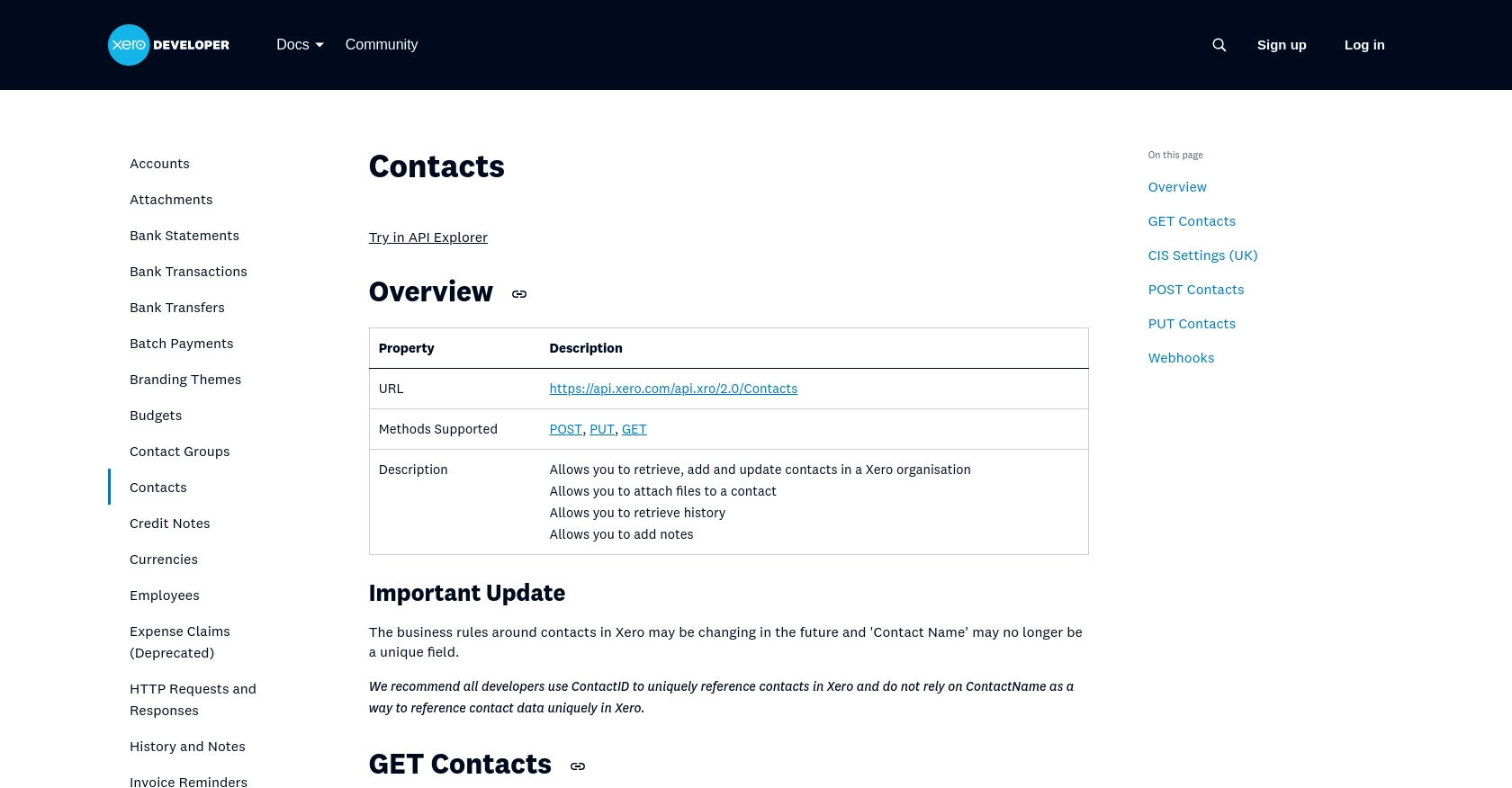
Conclusion: Best Practices for Xero API Integration in PHP
Integrating with the Xero API using PHP can significantly enhance your application's ability to manage customer data efficiently. By following the steps outlined in this guide, you can create or update customer records seamlessly, ensuring data consistency across platforms.
Storing User Credentials Securely
It's crucial to store your access tokens and client secrets securely. Consider using environment variables or secure vault services to protect sensitive information from unauthorized access.
Handling Xero API Rate Limits
Be mindful of Xero's API rate limits to avoid disruptions in service. According to the Xero OAuth 2.0 API limits, ensure your application handles rate limiting gracefully by implementing retry logic and monitoring API usage.
Transforming and Standardizing Data Fields
When integrating with Xero, ensure that data fields are transformed and standardized to match Xero's requirements. This will help prevent errors and ensure smooth data synchronization.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate allows you to build once for each use case and connect to multiple platforms with ease, saving time and resources. Visit Endgrate to learn more about how it can enhance your integration strategy.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/contacts
Ready to get started?