How to Get Credit Notes with the Chargebee API in Python
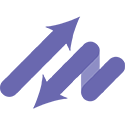
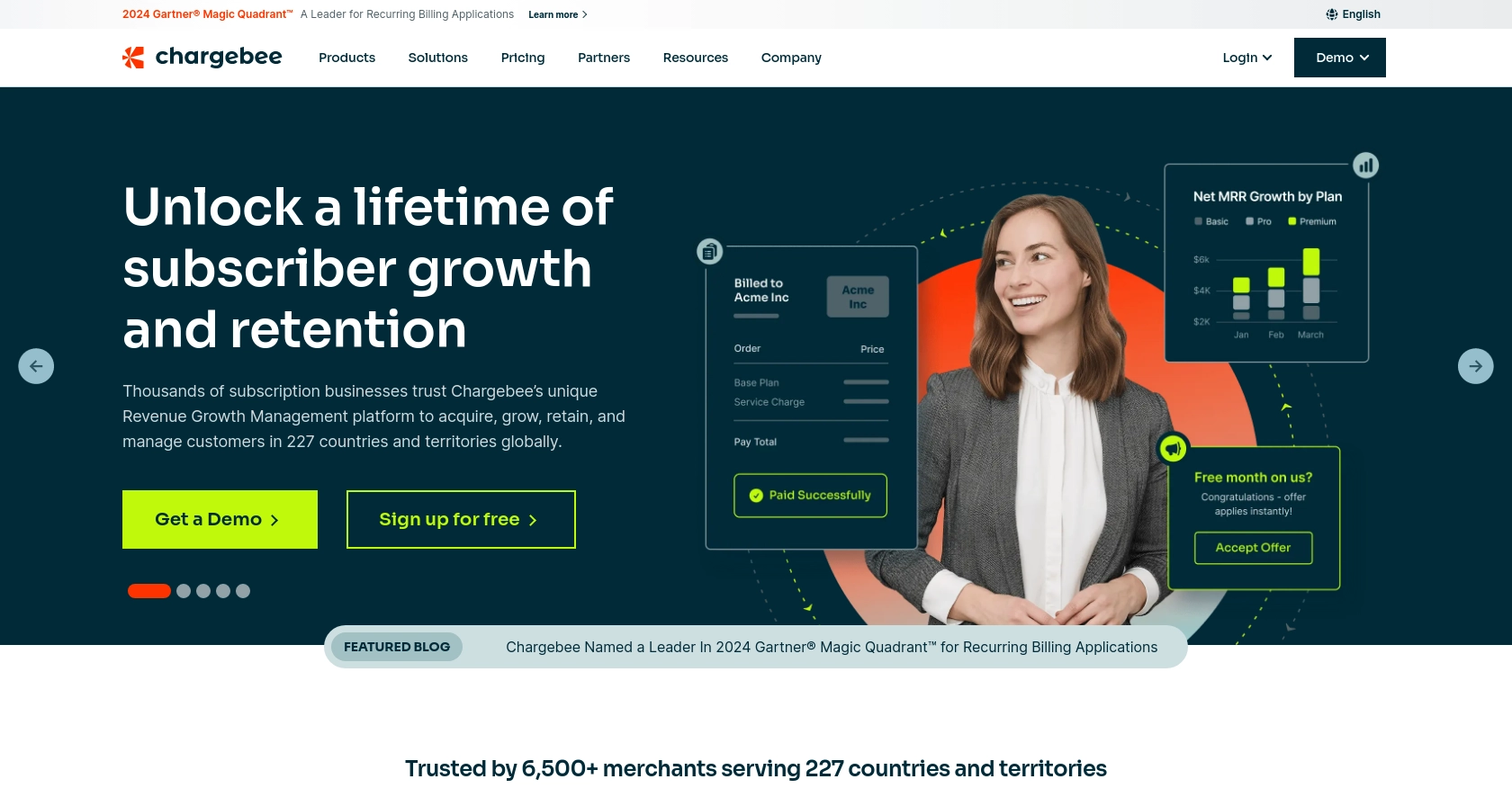
Introduction to Chargebee and Its API
Chargebee is a robust subscription management and billing platform designed to streamline the financial operations of SaaS businesses. It provides a comprehensive suite of tools to manage subscriptions, billing, invoicing, and revenue recognition, making it a preferred choice for businesses looking to automate their billing processes.
Integrating with Chargebee's API allows developers to efficiently manage financial transactions and customer billing data. For example, you might want to retrieve credit notes using the Chargebee API to automate financial reconciliations or generate detailed financial reports. This can significantly enhance the efficiency of your accounting processes and ensure accurate financial tracking.
Setting Up Your Chargebee Test/Sandbox Account
Before you can start interacting with the Chargebee API, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating a Chargebee Test Account
To begin, sign up for a Chargebee test account. Visit the Chargebee signup page and follow the instructions to create your account. Once registered, you will have access to a sandbox environment where you can test API interactions.
Generating API Keys for Chargebee
Chargebee uses HTTP Basic authentication for API calls, where your API key serves as the username and the password is left empty. Follow these steps to generate your API key:
- Log in to your Chargebee account and navigate to the 'Settings' section.
- Under 'API & Webhooks', select 'API Keys'.
- Click on 'Add API Key' to generate a new key. Make sure to note down the key, as it will be used for authentication in your API requests.
Remember, the API keys for your test site are different from those for your live site. Ensure you use the correct key for your testing environment.
Configuring OAuth for Chargebee (If Applicable)
If your integration requires OAuth, you will need to create an application in Chargebee:
- Go to 'Settings' and select 'Apps'.
- Click on 'Create App' and fill in the necessary details, such as the app name and redirect URL.
- Once created, you will receive a client ID and client secret, which are essential for OAuth-based authentication.
Use these credentials to authorize users and access Chargebee's resources securely.
With your Chargebee test account and API keys set up, you're ready to start making API calls to retrieve credit notes and more.
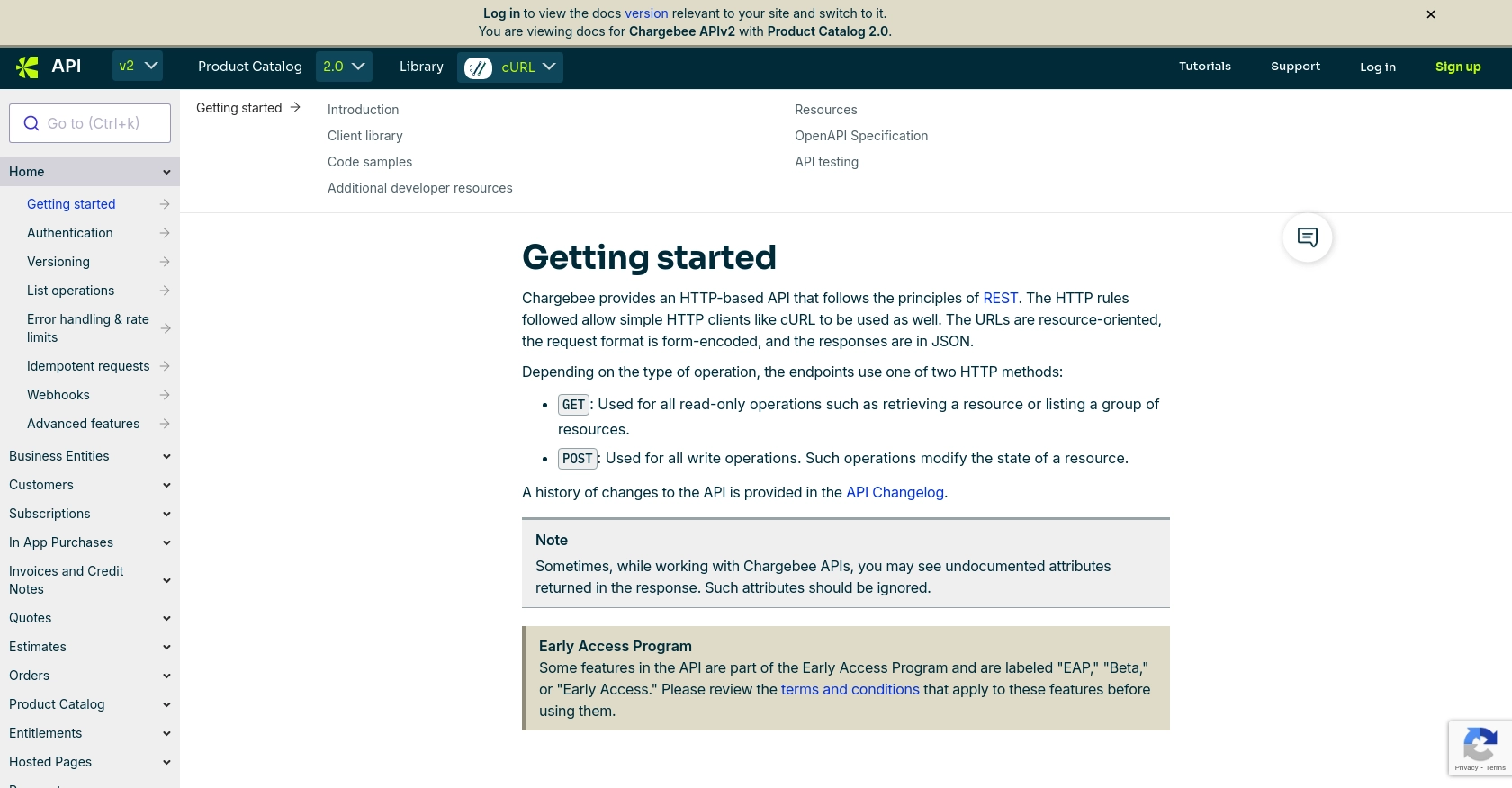
sbb-itb-96038d7
How to Make API Calls to Retrieve Credit Notes Using Chargebee API in Python
To interact with the Chargebee API and retrieve credit notes, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Chargebee API Integration
Before you start coding, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once these are installed, open your terminal or command prompt and install the requests
library, which is essential for making HTTP requests:
pip install requests
Writing Python Code to Retrieve Credit Notes from Chargebee
Create a new Python file named get_credit_notes.py
and add the following code:
import requests
# Set the API endpoint and authentication details
endpoint = "https://{your-site}.chargebee.com/api/v2/credit_notes"
api_key = "your_api_key"
# Set the request headers
headers = {
"Authorization": f"Basic {api_key}:",
"Content-Type": "application/json"
}
# Make a GET request to the Chargebee API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
credit_notes = response.json()
for credit_note in credit_notes['list']:
print(credit_note)
else:
print(f"Failed to retrieve credit notes: {response.status_code} - {response.text}")
Replace your_api_key
with the API key you generated in the Chargebee dashboard. This script makes a GET request to the Chargebee API to fetch credit notes and prints them to the console.
Verifying API Call Success and Handling Errors
After running the script, you should see a list of credit notes printed in the console. If the request fails, the script will output an error message with the status code and response text.
Chargebee uses standard HTTP status codes to indicate success or failure. Here are some common error codes you might encounter:
- 400 Bad Request: The request was invalid. Check your parameters.
- 401 Unauthorized: Authentication failed. Verify your API key.
- 404 Not Found: The requested resource was not found.
- 429 Too Many Requests: Rate limit exceeded. Refer to the Chargebee documentation for rate limit details.
For more detailed error handling, refer to the Chargebee API documentation.
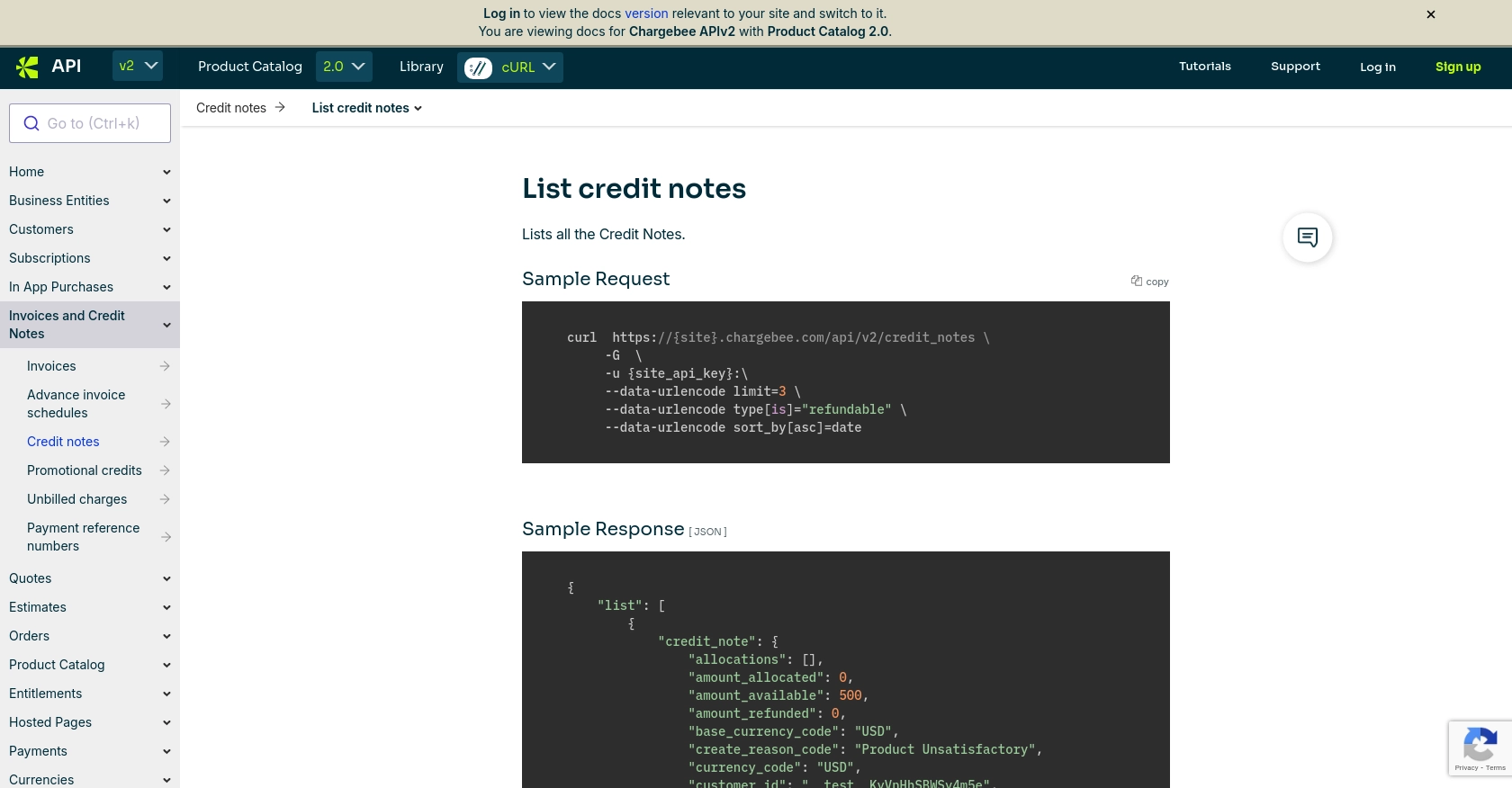
Best Practices for Using Chargebee API in Python
When integrating with the Chargebee API, it's essential to follow best practices to ensure security, efficiency, and reliability. Here are some key recommendations:
- Secure API Keys: Store your API keys securely and avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Chargebee imposes rate limits on API requests. To avoid hitting these limits, implement exponential backoff strategies and monitor your API usage. For more details, refer to the Chargebee API documentation.
- Validate API Responses: Always check the status codes and response data to handle errors gracefully. Implement logging to capture and analyze any issues that arise during API interactions.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across your application and Chargebee.
Conclusion and Call to Action for Using Endgrate for Chargebee Integrations
Integrating with Chargebee's API using Python can significantly streamline your billing and subscription management processes. By following the steps outlined in this guide, you can efficiently retrieve credit notes and automate financial tasks.
However, building and maintaining integrations can be time-consuming and complex. This is where Endgrate can help. With Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate today.
Read More
Ready to get started?