Using the Sap Business One API to Create or Update Items in PHP
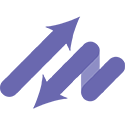
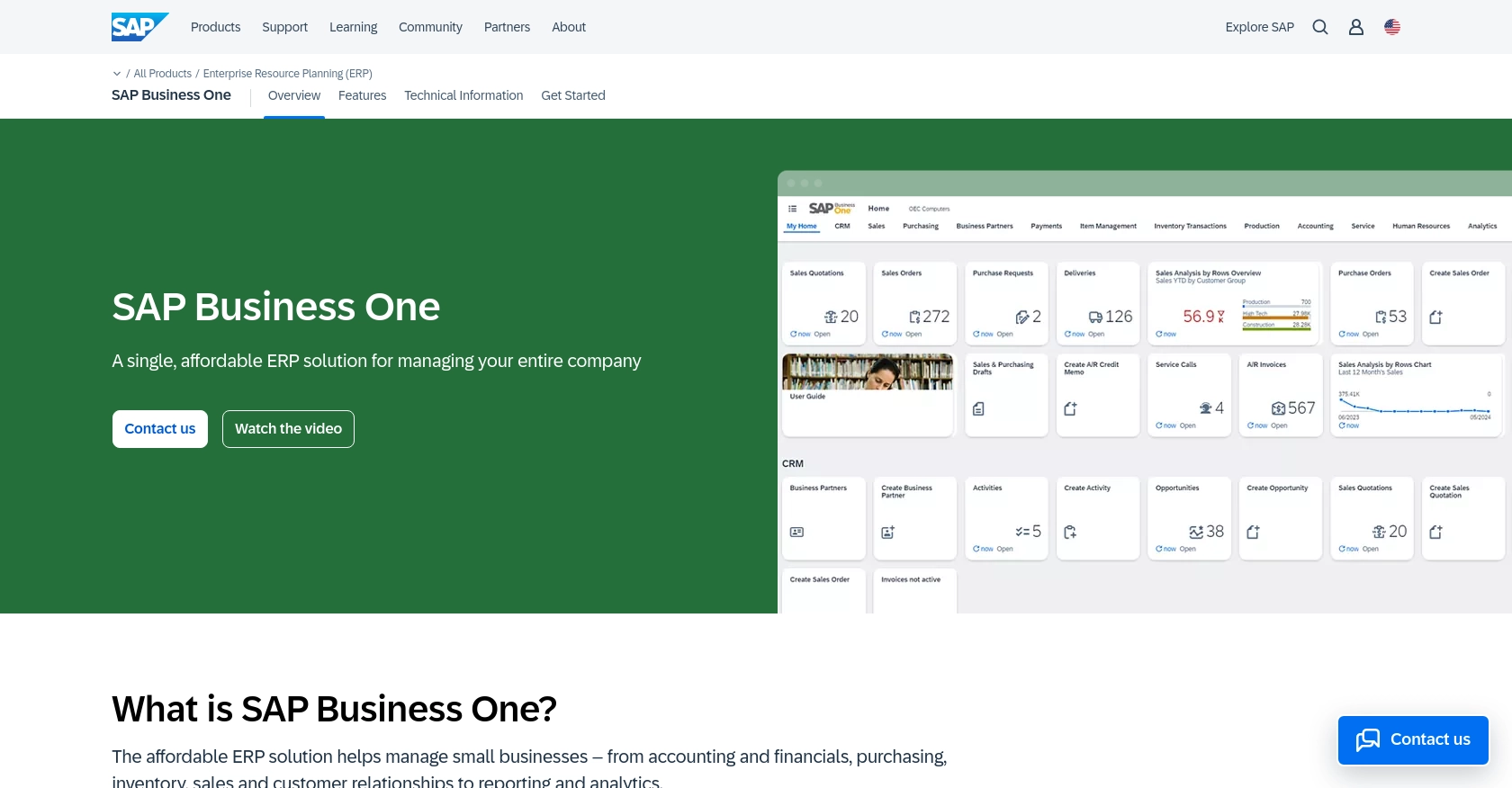
Introduction to SAP Business One API
SAP Business One is a comprehensive enterprise resource planning (ERP) solution designed for small to medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, inventory, and operations. By integrating with the SAP Business One API, developers can enhance business processes by automating tasks and connecting various business functions seamlessly.
Developers might want to interact with the SAP Business One API to manage inventory items efficiently. For example, a developer could use the API to create or update product items in the system, ensuring that the inventory data is always up-to-date and accurate. This can be particularly useful for businesses that need to synchronize their inventory data with other systems or platforms.
Setting Up a Test or Sandbox Account for SAP Business One API
Before you can start integrating with the SAP Business One API, it's essential to set up a test or sandbox environment. This allows you to safely experiment with API calls without affecting your live data. SAP Business One provides a comprehensive sandbox environment for developers to test their integrations.
Creating a SAP Business One Sandbox Account
To begin, you'll need to create a sandbox account. Follow these steps to set up your environment:
- Visit the SAP Business One Service Layer documentation for detailed instructions on accessing the sandbox environment.
- Sign up for a developer account if you haven't already. This will grant you access to the necessary tools and resources.
- Once your account is set up, log in to the SAP Business One portal and navigate to the sandbox section to activate your test environment.
Configuring OAuth Authentication for SAP Business One API
SAP Business One uses a custom authentication method. Follow these steps to configure OAuth authentication:
- In your sandbox account, navigate to the API management section.
- Create a new application and note down the client ID and client secret provided.
- Set the necessary permissions for your application to interact with inventory items.
- Use the client ID and client secret to generate an access token, which will be used to authenticate your API requests.
Generating API Keys for SAP Business One
If your integration requires API key-based access, follow these steps to generate and obtain your API key:
- Go to the API keys section in your sandbox account.
- Click on "Generate New API Key" and provide a name for your key.
- Copy the generated API key and store it securely, as it will be required for making API calls.
With your sandbox account set up and authentication configured, you're now ready to start making API calls to create or update items in SAP Business One using PHP.
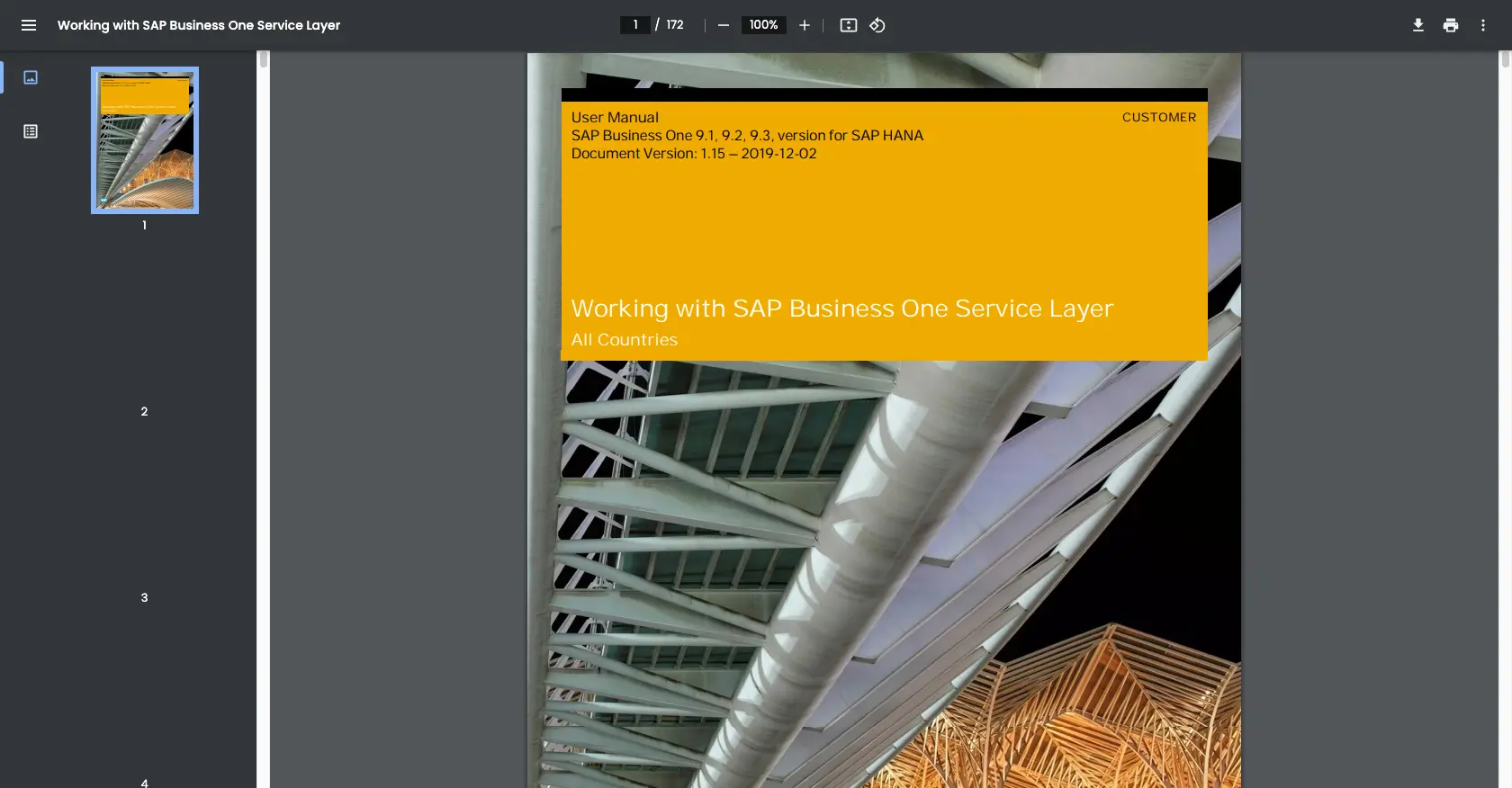
sbb-itb-96038d7
Making API Calls to Create or Update Items in SAP Business One Using PHP
To interact with the SAP Business One API using PHP, you need to ensure you have the correct environment set up. This includes having the right PHP version and necessary libraries installed. In this section, we'll guide you through making API calls to create or update items in SAP Business One.
Setting Up Your PHP Environment for SAP Business One API
Before you begin, make sure your development environment is ready:
- Ensure you have PHP version 7.4 or higher installed on your machine.
- Install the
cURL
extension for PHP, which is essential for making HTTP requests.
You can install the cURL extension using the following command:
sudo apt-get install php-curl
Creating or Updating Items in SAP Business One
Now that your environment is set up, you can proceed to create or update items using the SAP Business One API. Below is a sample PHP script to demonstrate how to perform these actions.
<?php
// Set the API endpoint
$endpoint = "https://your-sap-business-one-instance.com/b1s/v1/Items";
// Set the headers, including the access token for authentication
$headers = [
"Content-Type: application/json",
"Authorization: Bearer Your_Access_Token"
];
// Define the item data to be created or updated
$itemData = [
"ItemCode" => "A0001",
"ItemName" => "Sample Item",
"ItemType" => "itInventory",
"InventoryItem" => true
];
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($itemData));
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo "cURL error: " . curl_error($ch);
} else {
// Parse the response
$responseData = json_decode($response, true);
if (isset($responseData['error'])) {
echo "Error: " . $responseData['error']['message']['value'];
} else {
echo "Item created/updated successfully.";
}
}
// Close cURL session
curl_close($ch);
?>
Replace Your_Access_Token
with the token you generated during the authentication setup. This script initializes a cURL session, sets the necessary headers, and sends a POST request to the SAP Business One API to create or update an item.
Verifying API Call Success in SAP Business One
After executing the script, you can verify the success of your API call by checking the SAP Business One sandbox environment. Navigate to the inventory section to see if the item has been created or updated as expected.
Handling Errors and Common Error Codes
While making API calls, you might encounter errors. It's crucial to handle these gracefully. Common error codes include:
- 400 Bad Request: The request was invalid. Check your data format and required fields.
- 401 Unauthorized: Authentication failed. Ensure your access token is correct.
- 500 Internal Server Error: An error occurred on the server. Try again later or contact support.
Always log errors and review the response data to troubleshoot issues effectively.
Best Practices for Using SAP Business One API in PHP
When working with the SAP Business One API, it's essential to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Securely Store Credentials: Always store your API keys and access tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the SAP Business One API. Implement retry logic and exponential backoff strategies to handle rate limit responses gracefully.
- Data Transformation and Standardization: Ensure that the data you send and receive is properly formatted and standardized. This helps maintain data integrity across different systems.
- Error Handling: Implement robust error handling to manage API call failures. Log errors for troubleshooting and provide meaningful feedback to users.
Streamlining Integration with Endgrate
Integrating with multiple APIs can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including SAP Business One. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the complexities of API integrations.
- Build Once, Deploy Anywhere: Develop a single integration that works across multiple platforms, reducing development effort.
- Enhance User Experience: Provide your customers with a seamless and intuitive integration experience.
Visit Endgrate to learn more about how you can streamline your integration processes and enhance your product offerings.
Read More
Ready to get started?