Using the BigCommerce API to Get Products in Javascript
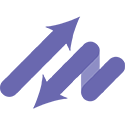
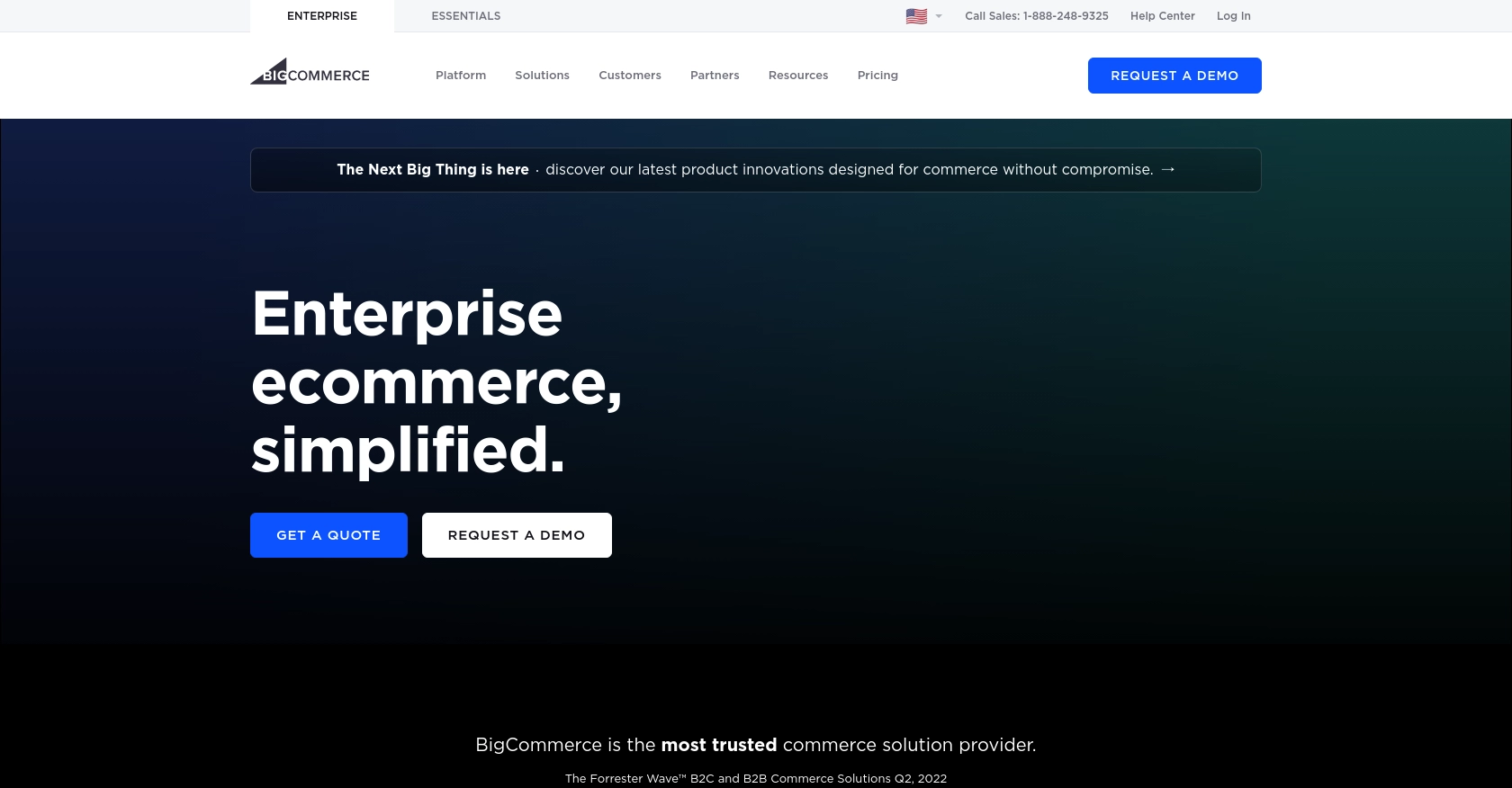
Introduction to BigCommerce API Integration
BigCommerce is a robust e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a wide range of features, including product management, order processing, and customer engagement tools, making it a popular choice for businesses looking to expand their online presence.
Integrating with the BigCommerce API allows developers to automate and streamline various store operations. For example, using the BigCommerce API, developers can retrieve product information to display on external websites or applications, ensuring that customers always see the most up-to-date product listings.
This article will guide you through the process of using JavaScript to interact with the BigCommerce API, specifically focusing on retrieving product data. By following this tutorial, you'll learn how to efficiently access and manage product information within your BigCommerce store.
Setting Up Your BigCommerce Test Account for API Integration
Before you can begin interacting with the BigCommerce API using JavaScript, you'll need to set up a test or sandbox account. This environment allows you to safely test API calls without affecting live data in your production store.
Creating a BigCommerce Sandbox Store
To start, you'll need a BigCommerce sandbox store. Follow these steps to create one:
- Visit the BigCommerce Sandbox page and sign up for a free sandbox account.
- Fill out the required information to create your account. Once completed, you'll have access to a sandbox store where you can test API interactions.
Generating API Credentials for BigCommerce
Once your sandbox store is set up, you need to generate API credentials to authenticate your requests. Here's how:
- Log in to your BigCommerce control panel.
- Navigate to Advanced Settings > API Accounts.
- Click on Create API Account and select V2/V3 API Token.
- Enter a name for your API account and configure the necessary OAuth scopes for accessing product data.
- Click Save to generate your Client ID, Client Secret, and Access Token. Make sure to store these credentials securely.
Configuring OAuth Scopes for BigCommerce API Access
To ensure your API account has the correct permissions, configure the OAuth scopes as follows:
- For retrieving product data, ensure you have the Products read scope enabled.
- Review other scopes and enable any additional permissions your application might require.
With your sandbox store and API credentials ready, you can now proceed to make API calls to retrieve product data using JavaScript.
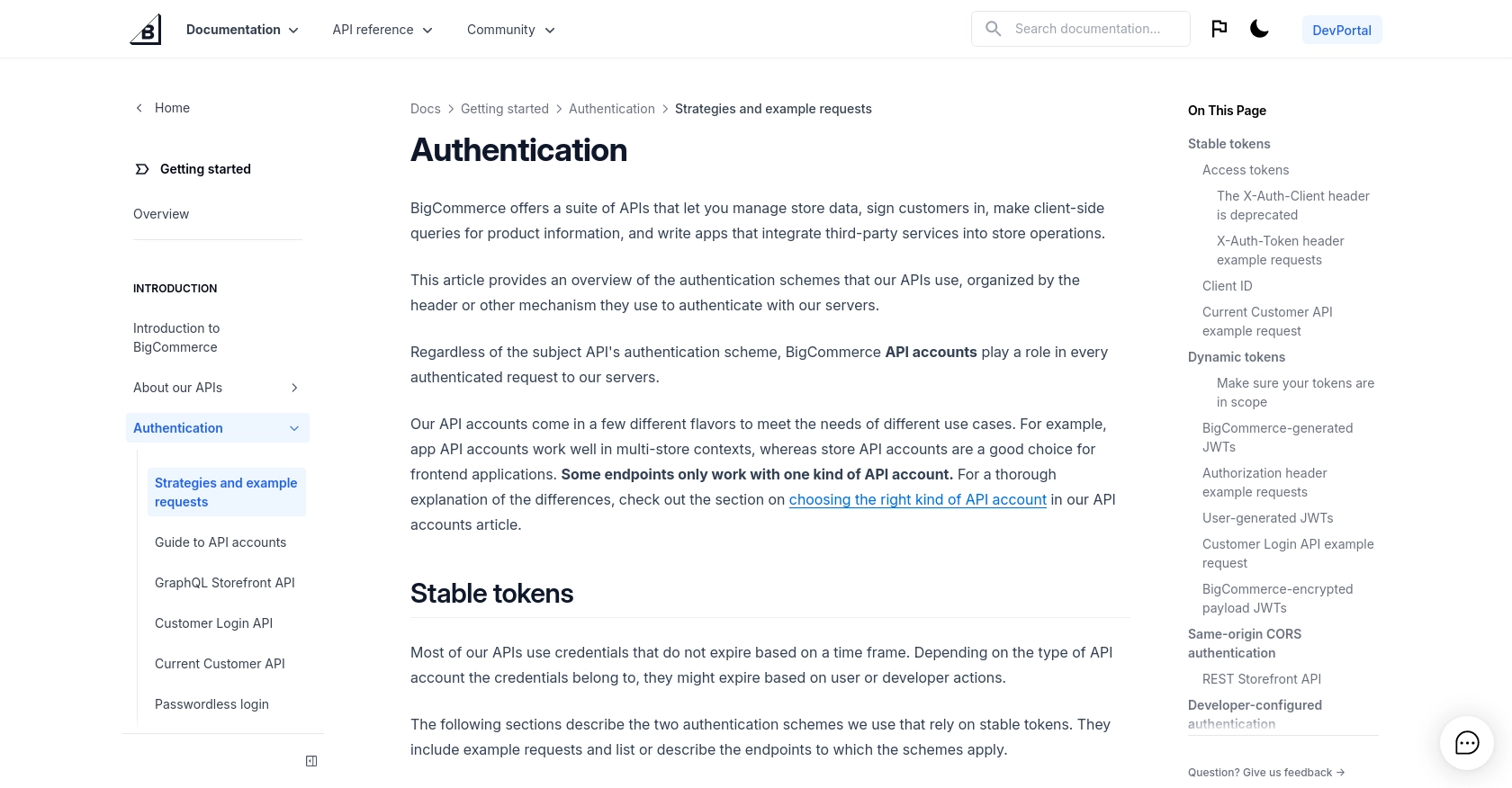
sbb-itb-96038d7
Making API Calls to Retrieve Products from BigCommerce Using JavaScript
To interact with the BigCommerce API and retrieve product data using JavaScript, you'll need to set up your development environment and write code that makes authenticated requests to the API. This section will guide you through the necessary steps, including setting up JavaScript dependencies, writing the code to make API calls, and handling the responses.
Setting Up Your JavaScript Environment for BigCommerce API Integration
Before you start coding, ensure you have the following prerequisites installed on your machine:
- Node.js (latest stable version)
- npm (Node Package Manager)
Once you have Node.js and npm installed, create a new project directory and initialize it with the following command:
npm init -y
Next, install the axios
library, which will be used to make HTTP requests to the BigCommerce API:
npm install axios
Writing JavaScript Code to Fetch Products from BigCommerce
With your environment set up, you can now write the JavaScript code to retrieve products from your BigCommerce store. Create a new file named getProducts.js
and add the following code:
const axios = require('axios');
// Replace with your store's API credentials
const storeHash = 'your_store_hash';
const accessToken = 'your_access_token';
// Set the API endpoint
const endpoint = `https://api.bigcommerce.com/stores/${storeHash}/v3/catalog/products`;
// Configure the request headers
const headers = {
'X-Auth-Token': accessToken,
'Accept': 'application/json',
'Content-Type': 'application/json'
};
// Function to fetch products
async function fetchProducts() {
try {
const response = await axios.get(endpoint, { headers });
const products = response.data.data;
console.log('Products:', products);
} catch (error) {
console.error('Error fetching products:', error.response ? error.response.data : error.message);
}
}
// Execute the function
fetchProducts();
In this code, you use the axios
library to send a GET request to the BigCommerce API endpoint for retrieving products. The request includes the necessary headers for authentication, using your store's storeHash
and accessToken
.
Running Your JavaScript Code and Verifying the Output
To execute your code and fetch products from BigCommerce, run the following command in your terminal:
node getProducts.js
If successful, you should see a list of products printed to the console. This output confirms that your API call was successful and that you have retrieved product data from your BigCommerce store.
Handling Errors and Verifying API Call Success
When making API calls, it's important to handle potential errors gracefully. The code above includes a try-catch
block to catch and log any errors that occur during the request. Common error codes you might encounter include:
- 401 Unauthorized: Check your access token and ensure it has the correct permissions.
- 404 Not Found: Verify the endpoint URL and store hash.
- 500 Internal Server Error: This may indicate a temporary issue with the BigCommerce server.
By handling errors appropriately, you can ensure a robust integration with the BigCommerce API.
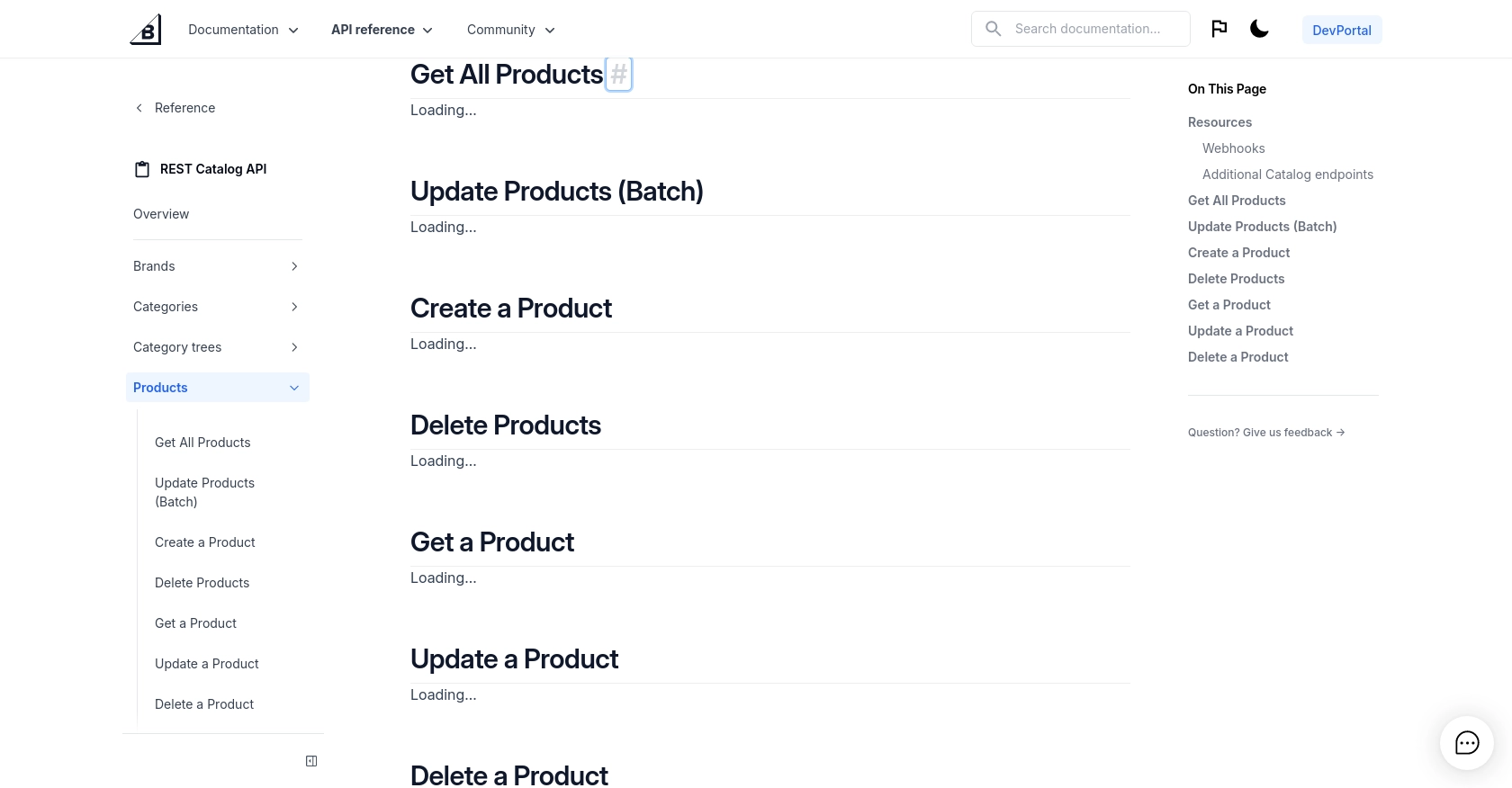
Conclusion and Best Practices for BigCommerce API Integration Using JavaScript
Integrating with the BigCommerce API using JavaScript provides a powerful way to manage and display product data efficiently. By following the steps outlined in this guide, you can seamlessly retrieve product information and enhance your e-commerce operations.
Best Practices for Secure and Efficient BigCommerce API Usage
- Secure Storage of Credentials: Always store your API credentials securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of BigCommerce's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from the API is transformed and standardized to fit your application's requirements, maintaining consistency across platforms.
Enhancing Your Integration Strategy with Endgrate
While integrating with the BigCommerce API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple platforms, including BigCommerce. This approach not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration strategy by visiting Endgrate and discover the benefits of a unified API solution.
Read More
Ready to get started?