How to Create Tasks with the Zoho CRM API in Python
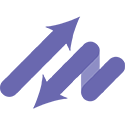
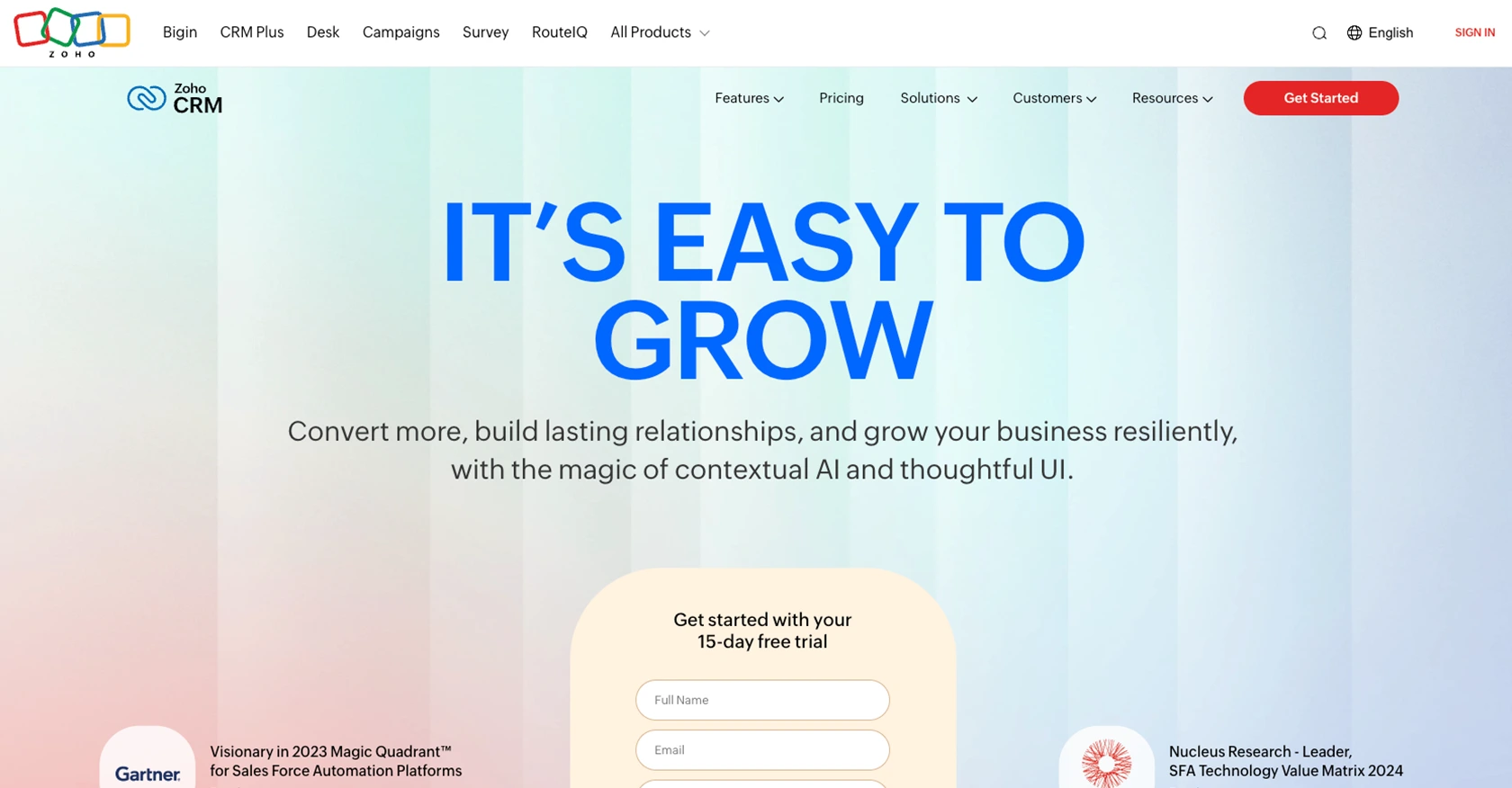
Introduction to Zoho CRM
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and customer support in a unified system. Known for its flexibility and extensive feature set, Zoho CRM is a popular choice for organizations looking to enhance their customer interactions and streamline operations.
Developers often integrate with Zoho CRM to automate and enhance business processes. For example, using the Zoho CRM API, developers can create tasks programmatically, allowing for seamless task management and improved productivity. This integration can be particularly useful for automating task creation based on specific triggers or events, ensuring that teams stay organized and efficient.
Setting Up Your Zoho CRM Test/Sandbox Account
Create a Zoho CRM Developer Account for API Integration
To begin integrating with the Zoho CRM API, you'll need to set up a developer account. This allows you to access the necessary tools and resources for testing and development.
- Visit the Zoho Developer Console.
- Sign up for a new account or log in if you already have one.
- Navigate to the "API Console" section to manage your applications.
Register Your Application for OAuth Authentication
Zoho CRM uses OAuth 2.0 for secure authentication. Follow these steps to register your application:
- In the Zoho Developer Console, select "Add Client" to create a new application.
- Choose the client type that suits your application (e.g., Web-based, Mobile).
- Fill in the required details such as Client Name, Homepage URL, and Authorized Redirect URIs.
- Click "Create" to generate your Client ID and Client Secret.
Ensure you save these credentials securely as they are essential for API authentication.
Configure OAuth Scopes for Zoho CRM API Access
Scopes define the level of access your application has to Zoho CRM resources. Configure them as follows:
- Navigate to the "Scopes" section in your application settings.
- Select the necessary scopes for task management, such as
ZohoCRM.modules.tasks.CREATE
. - Save your changes to update the application's permissions.
Generate Access and Refresh Tokens for API Calls
With your application registered, you can now generate tokens to authenticate API requests:
- Direct users to the Zoho authorization URL with your Client ID and requested scopes.
- Upon user consent, Zoho will redirect to your specified URI with an authorization code.
- Exchange this code for access and refresh tokens using the token endpoint.
Refer to the Zoho CRM OAuth Overview for detailed instructions on token generation.
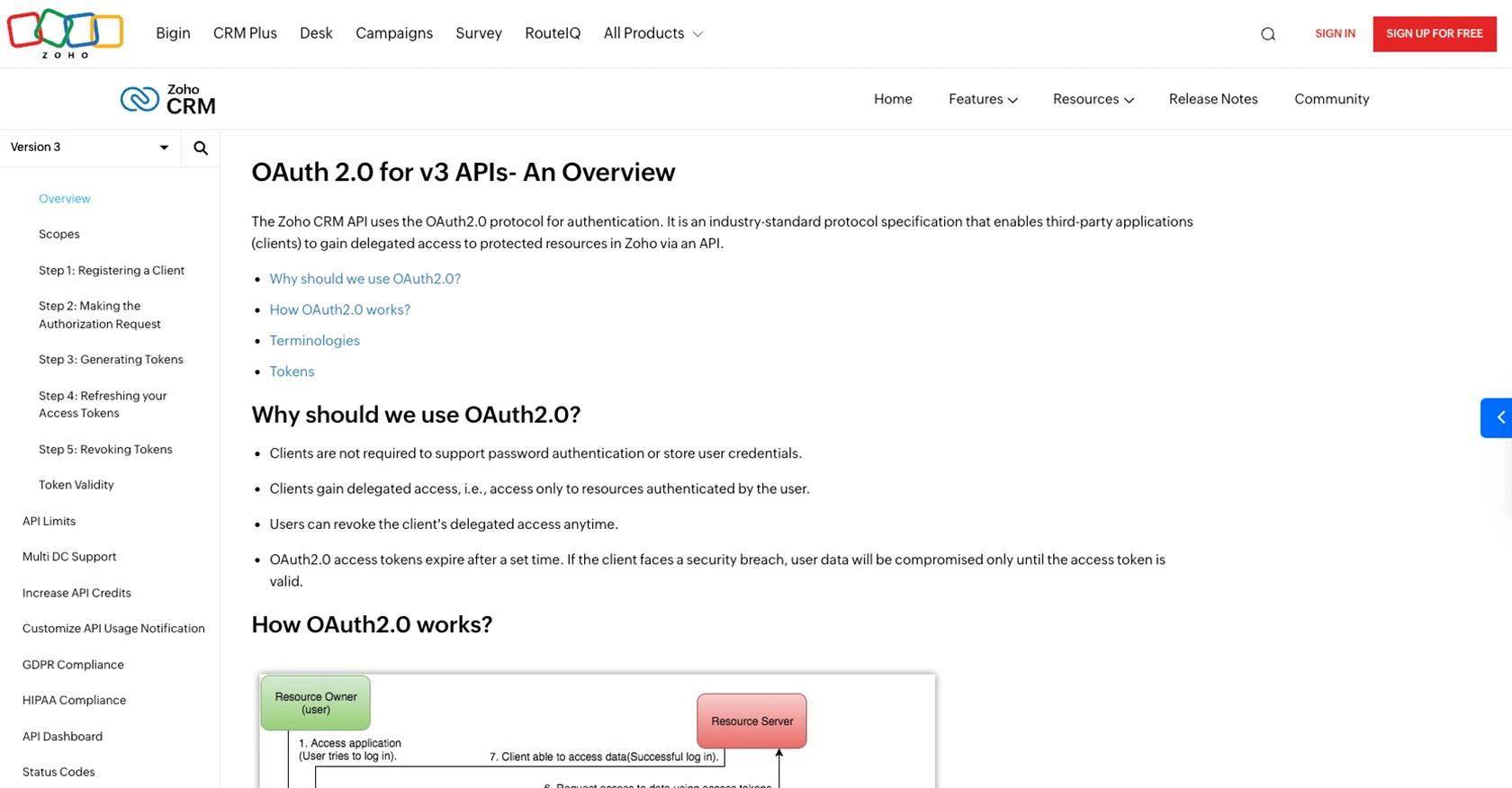
sbb-itb-96038d7
How to Make API Calls to Create Tasks in Zoho CRM Using Python
To create tasks in Zoho CRM using Python, you'll need to set up your environment and write code that interacts with the Zoho CRM API. This section will guide you through the necessary steps, including setting up Python, installing dependencies, and writing the code to make API calls.
Setting Up Your Python Environment for Zoho CRM API Integration
Before you begin, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. You will also need the requests
library to handle HTTP requests.
- Install Python from the official Python website if you haven't already.
- Open your terminal or command prompt and install the
requests
library using pip:
pip install requests
Writing Python Code to Create Tasks in Zoho CRM
With your environment set up, you can now write the Python code to create tasks in Zoho CRM. Follow these steps:
- Create a new Python file named
create_zoho_task.py
. - Add the following code to the file:
import requests
# Set the API endpoint and headers
url = "https://www.zohoapis.com/crm/v3/Tasks"
headers = {
"Authorization": "Zoho-oauthtoken Your_Access_Token",
"Content-Type": "application/json"
}
# Define the task data
task_data = {
"data": [
{
"Subject": "Follow up with client",
"Due_Date": "2024-03-15",
"Status": "Not Started"
}
]
}
# Make the POST request to create a task
response = requests.post(url, json=task_data, headers=headers)
# Check if the request was successful
if response.status_code == 201:
print("Task created successfully:", response.json())
else:
print("Failed to create task:", response.status_code, response.json())
Replace Your_Access_Token
with the access token you obtained during the OAuth setup.
Verifying Task Creation in Zoho CRM
After running the script, you can verify that the task was created successfully by checking the response or logging into your Zoho CRM account and navigating to the Tasks module.
Handling Errors and Common Issues in Zoho CRM API Calls
When making API calls, you may encounter errors. Here are some common error codes and their meanings:
- 401 Unauthorized: Check if your access token is valid and has not expired.
- 400 Bad Request: Ensure that your request payload is correctly formatted and all required fields are included.
- 429 Too Many Requests: You have exceeded the API rate limit. Refer to the Zoho CRM API Limits documentation for details.
For more detailed error handling, refer to the Zoho CRM Status Codes documentation.
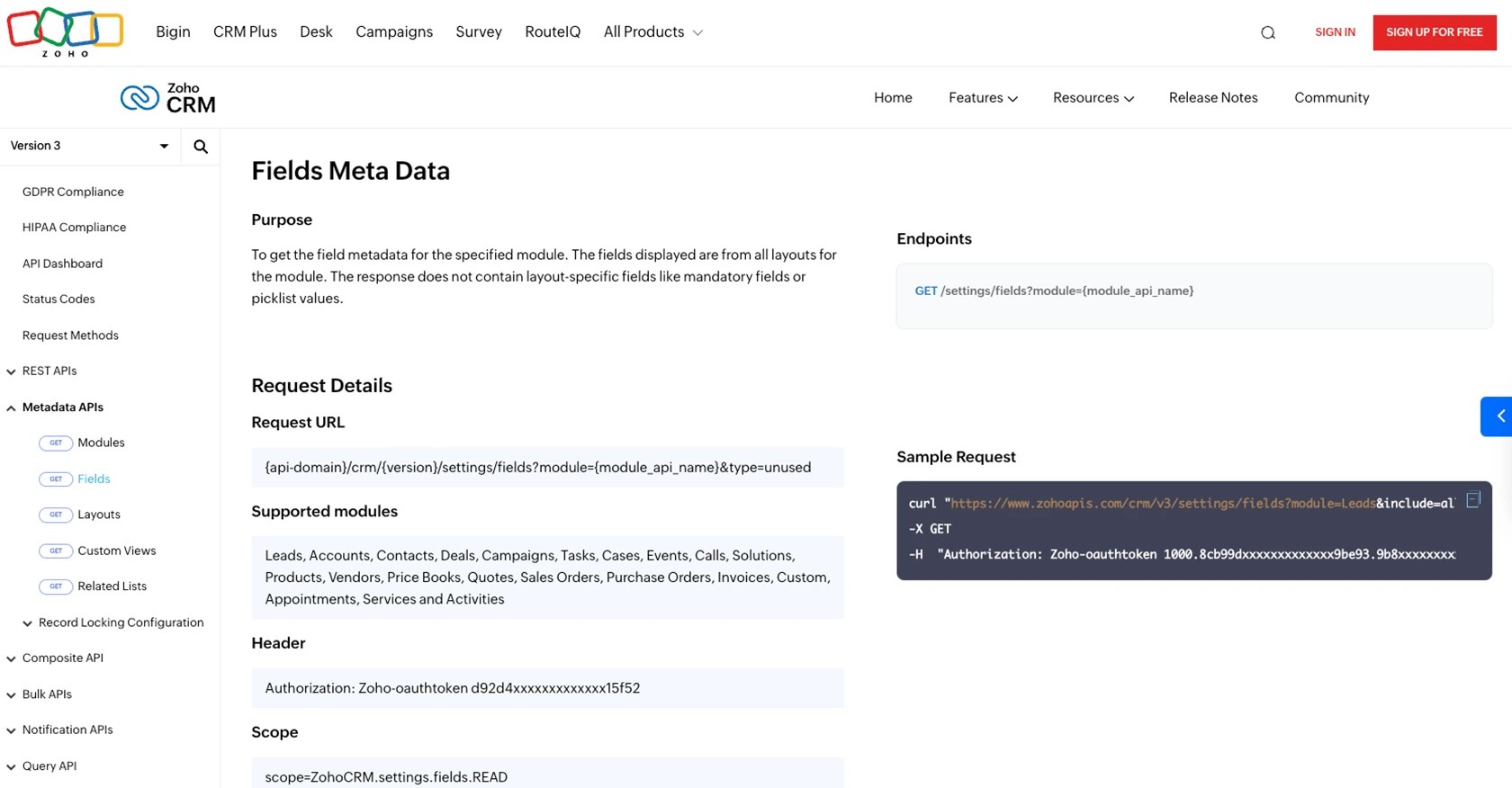
Conclusion and Best Practices for Zoho CRM API Integration
Integrating with the Zoho CRM API using Python can significantly enhance your business processes by automating task management and improving team productivity. By following the steps outlined in this guide, you can efficiently create tasks in Zoho CRM and ensure seamless integration with your existing systems.
Best Practices for Secure and Efficient Zoho CRM API Usage
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Avoid exposing them in public repositories or client-side code.
- Handle Rate Limiting: Be mindful of Zoho CRM's API rate limits. Implement retry logic and backoff strategies to handle
429 Too Many Requests
errors gracefully. Refer to the API Limits documentation for more details. - Data Standardization: Ensure that data fields are standardized and consistent across your applications to avoid discrepancies and improve data quality.
- Regularly Refresh Tokens: Implement logic to refresh access tokens using the refresh token to maintain uninterrupted API access.
Streamline Integrations with Endgrate
For developers looking to simplify and scale their integration efforts, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and leverage an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can enhance your integration strategy.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/search-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/insert-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/update-records.html
Ready to get started?