Using the Microsoft Dynamics 365 API to Get Users (with Javascript examples)
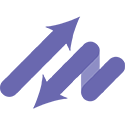
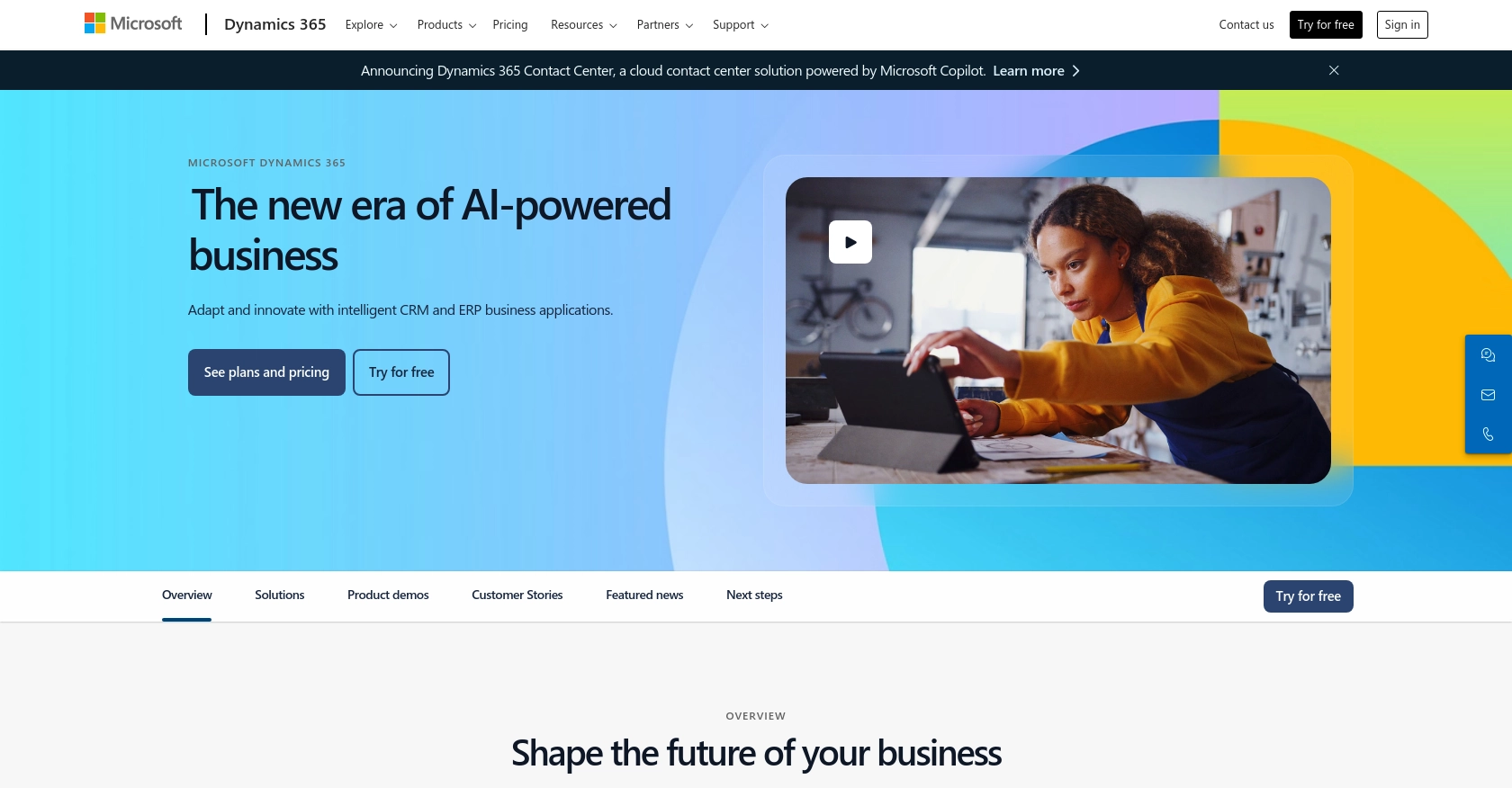
Introduction to Microsoft Dynamics 365 API
Microsoft Dynamics 365 is a comprehensive suite of business applications that combines CRM and ERP capabilities to streamline business processes and improve customer engagement. It offers a range of tools for sales, customer service, finance, operations, and more, making it a versatile choice for businesses looking to enhance their operational efficiency.
Developers often integrate with the Microsoft Dynamics 365 API to access and manage user data, enabling seamless interaction with the platform's robust features. For example, a developer might use the API to retrieve user information and synchronize it with other systems, ensuring consistent data across platforms.
This article will guide you through using JavaScript to interact with the Microsoft Dynamics 365 API, specifically focusing on retrieving user data. By following this tutorial, you'll learn how to efficiently access and manage user information within the Dynamics 365 environment.
Setting Up a Microsoft Dynamics 365 Test or Sandbox Account
Before you can begin integrating with the Microsoft Dynamics 365 API, it's essential to set up a test or sandbox account. This environment allows developers to safely experiment with API calls without affecting live data. Microsoft Dynamics 365 offers a free trial and sandbox environments specifically for development and testing purposes.
Creating a Microsoft Dynamics 365 Free Trial Account
- Visit the Microsoft Dynamics 365 website and navigate to the free trial section.
- Sign up for a free trial by providing the necessary details, such as your name, email, and company information.
- Follow the instructions to complete the registration process. Once registered, you'll receive access to a trial version of Dynamics 365.
Setting Up a Microsoft Dynamics 365 Sandbox Environment
If you already have a Dynamics 365 account, you can set up a sandbox environment for testing:
- Log in to your Microsoft Dynamics 365 account.
- Navigate to the Admin Center and select Environments.
- Click on New to create a new environment and choose Sandbox as the type.
- Configure the environment settings as needed and click Create.
Registering an Application for OAuth Authentication
To interact with the Microsoft Dynamics 365 API, you'll need to register an application in Microsoft Entra ID for OAuth authentication. Follow these steps:
- Go to the Azure Portal and sign in with your Microsoft account.
- Navigate to Azure Active Directory and select App registrations.
- Click on New registration and fill in the application details, such as name and redirect URI.
- Once registered, note down the Application (client) ID and Directory (tenant) ID.
- Under Certificates & secrets, create a new client secret and save it securely.
For more detailed instructions on OAuth authentication, refer to the official documentation: Use OAuth authentication with Microsoft Dataverse.
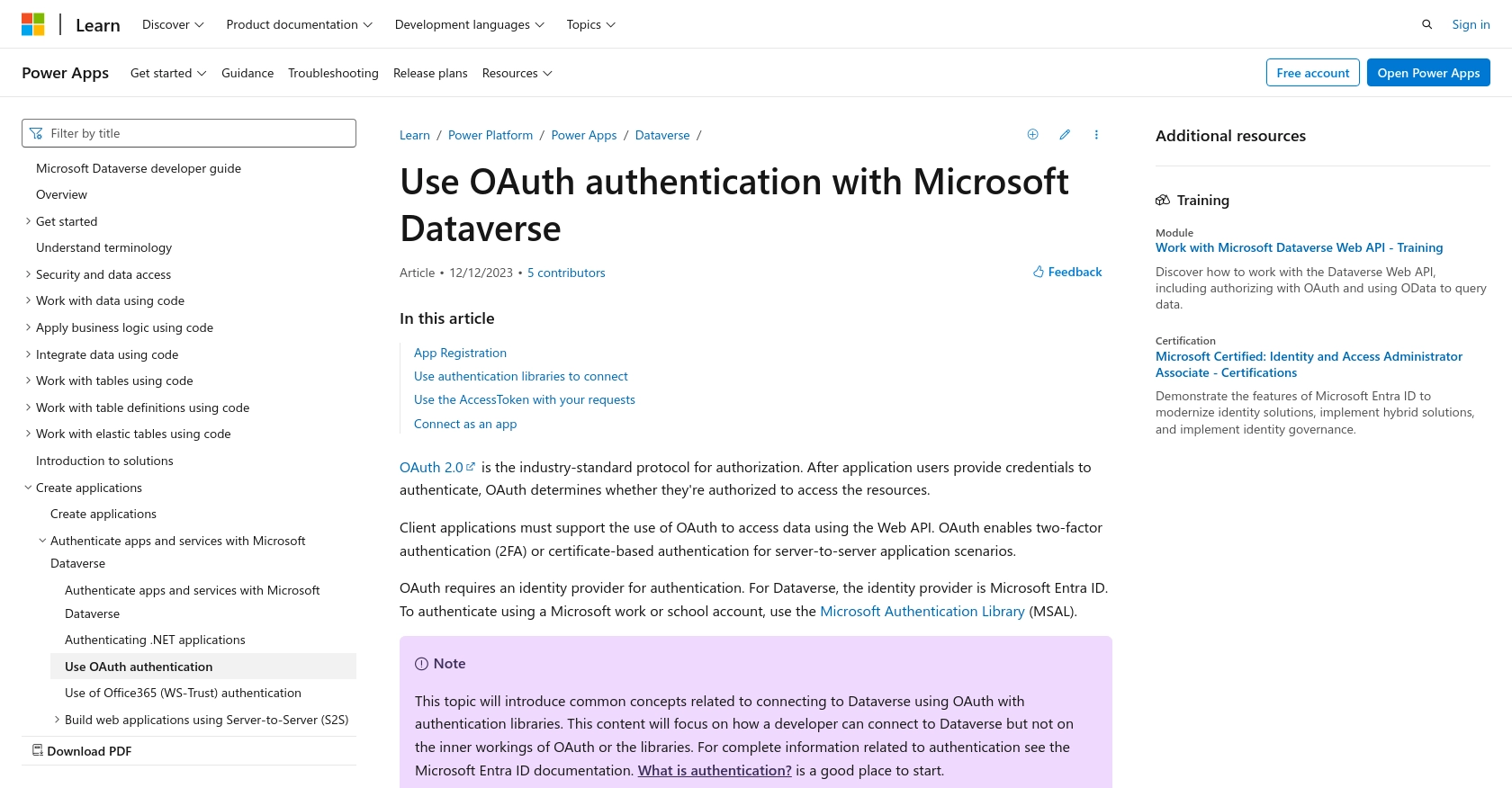
sbb-itb-96038d7
Making API Calls to Retrieve Users from Microsoft Dynamics 365 Using JavaScript
To interact with the Microsoft Dynamics 365 API and retrieve user data, you'll need to make HTTP requests using JavaScript. This section will guide you through the process, including setting up your environment, writing the code, and handling responses.
Setting Up Your JavaScript Environment for Microsoft Dynamics 365 API
Before making API calls, ensure you have the necessary tools and libraries installed. You'll need:
- A modern web browser with developer tools.
- A text editor or IDE for writing JavaScript code.
- The
axios
library for making HTTP requests. You can include it in your project by adding the following script tag to your HTML file:
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
Writing JavaScript Code to Retrieve Users from Microsoft Dynamics 365
With your environment set up, you can now write the JavaScript code to make API calls to Microsoft Dynamics 365. Follow these steps:
- Define the API endpoint and headers. You'll need to include the access token obtained during OAuth authentication.
- Use the
axios.get
method to make a GET request to the API endpoint. - Handle the response to extract and display user data.
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://yourorg.crm.dynamics.com/api/data/v9.2/systemusers';
const headers = {
'Authorization': 'Bearer Your_Access_Token',
'OData-MaxVersion': '4.0',
'OData-Version': '4.0',
'Accept': 'application/json'
};
// Make a GET request to the API
axios.get(endpoint, { headers })
.then(response => {
// Parse and display user data
const users = response.data.value;
users.forEach(user => {
console.log(`User ID: ${user.systemuserid}, Full Name: ${user.fullname}`);
});
})
.catch(error => {
console.error('Error fetching users:', error);
});
Replace Your_Access_Token
with the token obtained during the OAuth authentication process. This code uses axios
to send a GET request to the Microsoft Dynamics 365 API, retrieves user data, and logs the user ID and full name to the console.
Verifying Successful API Requests in Microsoft Dynamics 365
After running your JavaScript code, verify the success of your API requests by checking the response data. You should see a list of users with their IDs and full names. Additionally, you can log into your Microsoft Dynamics 365 sandbox environment to confirm the data matches.
Handling Errors and Understanding Error Codes
When making API calls, it's crucial to handle potential errors. The Microsoft Dynamics 365 API may return various error codes, such as:
- 401 Unauthorized: Indicates issues with authentication. Ensure your access token is valid.
- 403 Forbidden: Suggests insufficient permissions. Verify your app's permissions in Azure.
- 404 Not Found: The requested resource doesn't exist. Check the endpoint URL.
For more detailed error handling, refer to the official documentation: SystemUser EntityType Reference.
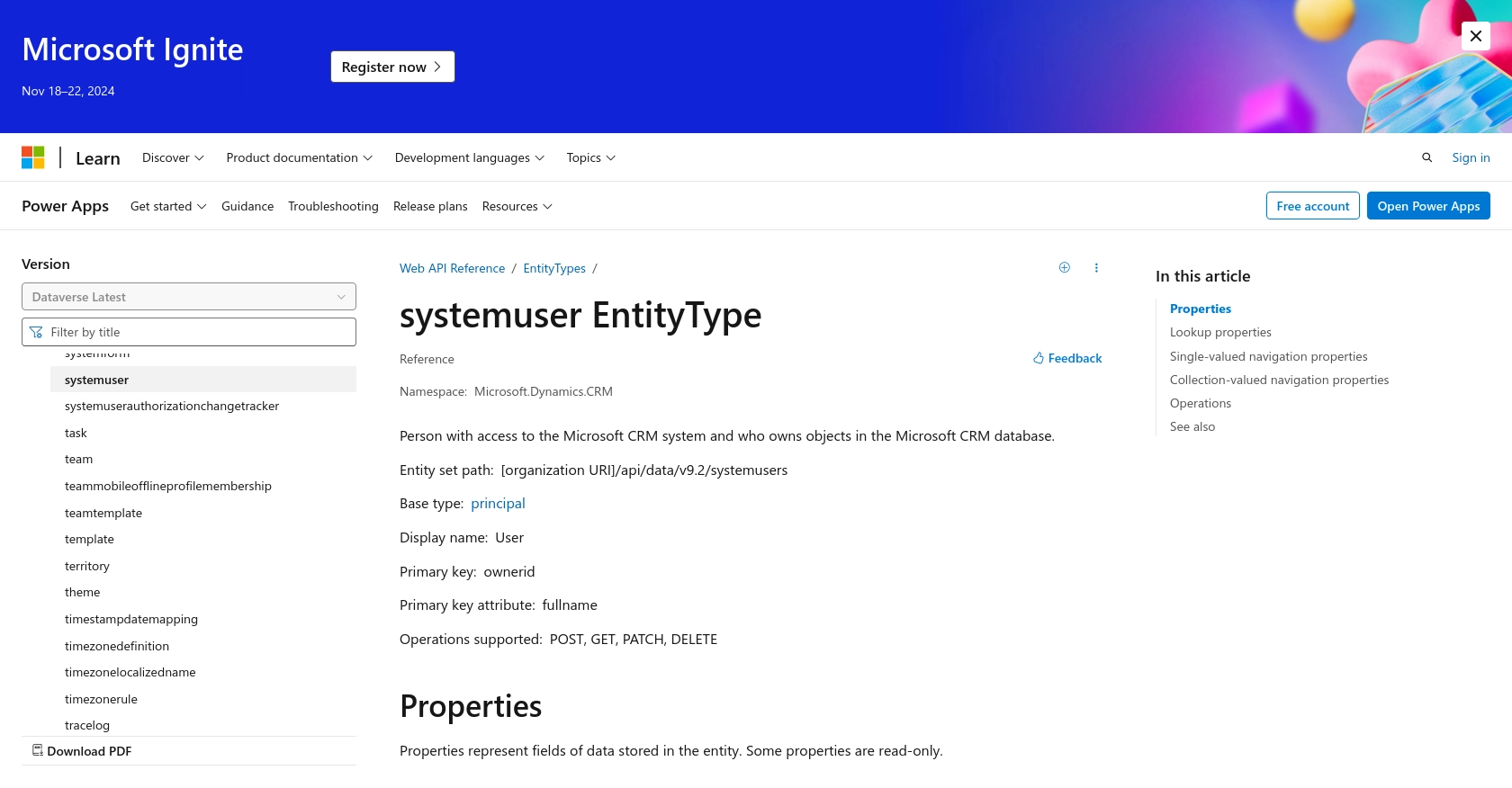
Conclusion and Best Practices for Using Microsoft Dynamics 365 API with JavaScript
Integrating with the Microsoft Dynamics 365 API using JavaScript provides developers with powerful capabilities to manage and synchronize user data across platforms. By following the steps outlined in this guide, you can efficiently retrieve user information and ensure seamless interaction with Dynamics 365's robust features.
Best Practices for Storing User Credentials and Handling Authentication
- Always store user credentials securely, using encryption and secure storage solutions to protect sensitive information.
- Regularly update and rotate access tokens to minimize security risks and ensure compliance with best practices.
- Utilize environment variables to manage sensitive data like client IDs and secrets, keeping them out of your source code.
Managing API Rate Limits and Error Handling
When working with the Microsoft Dynamics 365 API, it's essential to be aware of rate limits to avoid throttling. Implement logic to handle rate limiting gracefully, such as retry mechanisms with exponential backoff.
Additionally, ensure robust error handling by checking for specific error codes and implementing appropriate fallback strategies. This will enhance the reliability and user experience of your application.
Data Transformation and Standardization
When integrating data from Microsoft Dynamics 365 with other systems, consider transforming and standardizing data fields to maintain consistency. This practice helps in reducing discrepancies and ensures smooth data flow across platforms.
Leverage Endgrate for Streamlined Integrations
If you're looking to simplify the integration process and focus on your core product, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to build once for each use case instead of multiple times for different platforms. Take advantage of an easy, intuitive integration experience for your customers by exploring Endgrate's offerings at Endgrate.
Read More
- https://endgrate.com/provider/microsoftdynamics365
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/webapi/reference/systemuser?view=dataverse-latest
Ready to get started?