How to Get Orders with the Shopify API in Python
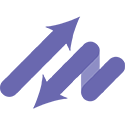
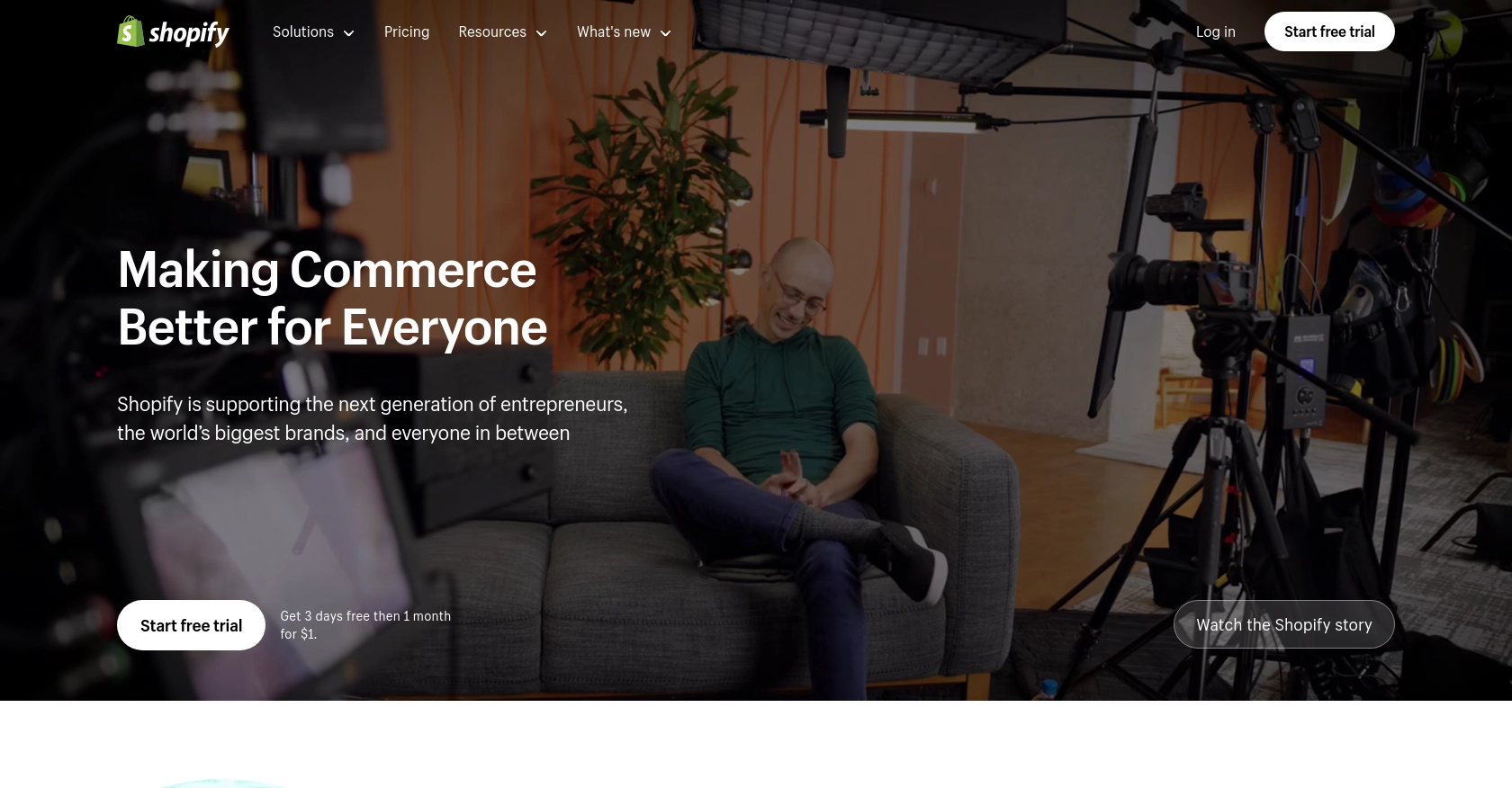
Introduction to Shopify API Integration
Shopify is a leading e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a robust set of tools for managing products, processing payments, and handling orders, making it a popular choice for businesses looking to establish an online presence.
Integrating with Shopify's API allows developers to automate and enhance various aspects of store management. For example, accessing order data through the Shopify API can enable developers to streamline order processing, track sales trends, and integrate with third-party logistics services.
This article will guide you through the process of using Python to interact with Shopify's API to retrieve order information, providing you with the tools to efficiently manage and analyze your store's sales data.
Setting Up Your Shopify Test Account for API Integration
Before you can start retrieving orders using the Shopify API, you need to set up a test account. Shopify provides developers with the ability to create a development store, which acts as a sandbox environment for testing API integrations without affecting live data.
Creating a Shopify Development Store
- Visit the Shopify Partners website and sign up for a free account if you haven't already.
- Once logged in, navigate to the "Stores" section in your Shopify Partners dashboard.
- Click on "Add store" and select "Development store" as the store type.
- Fill in the required details, such as store name and password, and click "Save" to create your development store.
Setting Up OAuth for Shopify API Access
Shopify uses OAuth for authenticating API requests. Follow these steps to set up OAuth for your app:
- In your Shopify Partners dashboard, go to "Apps" and click "Create app".
- Enter your app's name and select the development store you created earlier.
- Once the app is created, navigate to the "App setup" section to configure OAuth settings.
- Under "API credentials", note down the API key and API secret key. These will be used for authentication.
- Set the "Redirect URL" to the URL where you want Shopify to redirect users after they authorize your app.
- Under "Scopes", ensure you request the necessary permissions, such as
read_orders
, to access order data.
Generating Access Tokens
To interact with the Shopify API, you need to exchange the API key and secret key for an access token:
- Direct the user to the Shopify authorization URL, including your API key and requested scopes.
- After the user authorizes your app, Shopify will redirect them to your specified redirect URL with a code parameter.
- Use this code to request an access token from Shopify by making a POST request to the token endpoint.
import requests
# Replace with your app's credentials and the code received from Shopify
client_id = 'your_api_key'
client_secret = 'your_api_secret'
code = 'authorization_code'
# Request access token
response = requests.post(
'https://your-development-store.myshopify.com/admin/oauth/access_token',
data={
'client_id': client_id,
'client_secret': client_secret,
'code': code
}
)
access_token = response.json().get('access_token')
print("Access Token:", access_token)
With the access token, you can now make authenticated requests to the Shopify API to retrieve order data.
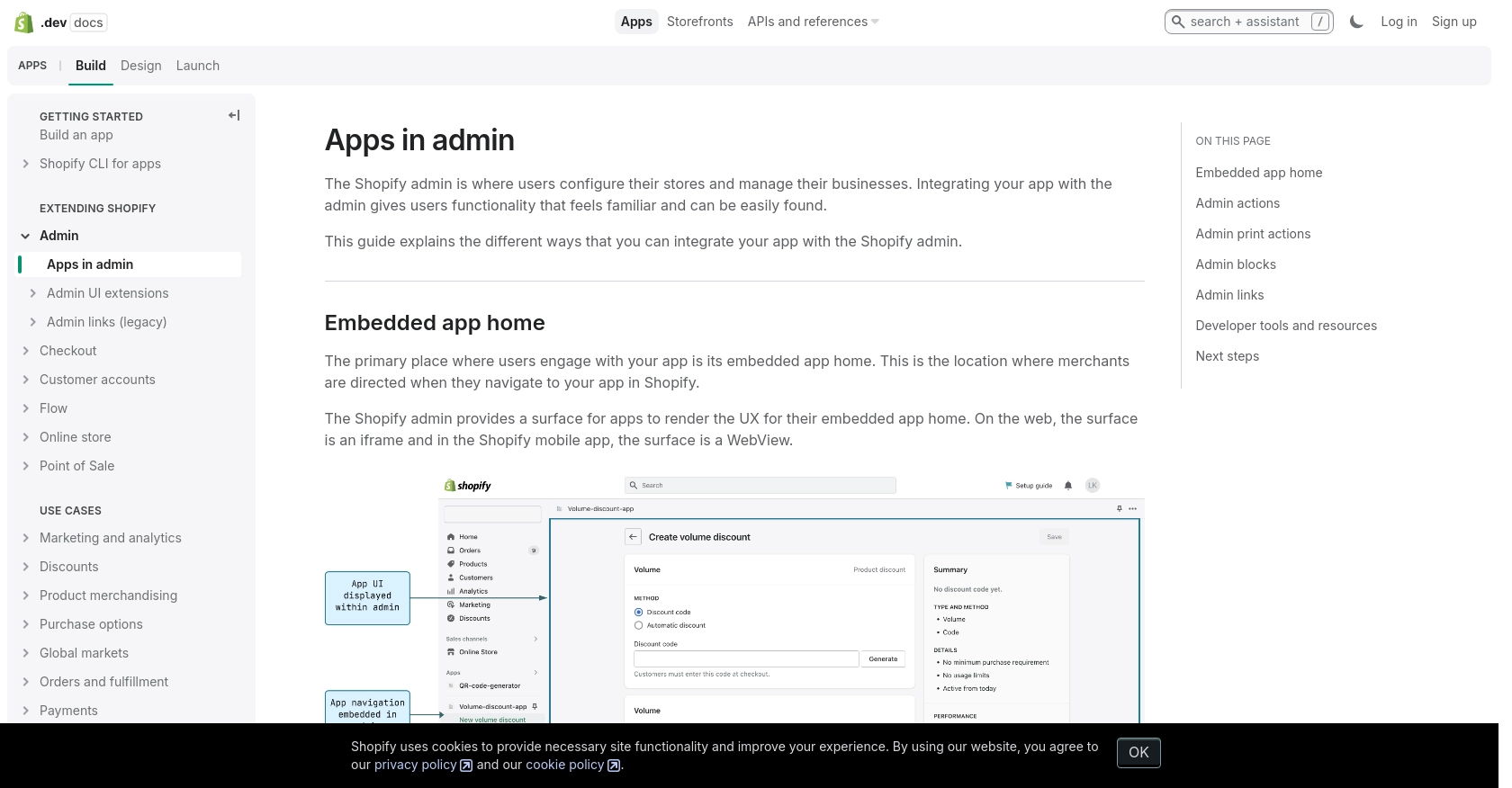
sbb-itb-96038d7
Making API Calls to Retrieve Shopify Orders Using Python
With your Shopify development store and OAuth setup complete, you can now proceed to make API calls to retrieve order data. This section will guide you through the process of using Python to interact with Shopify's API, ensuring you can efficiently access and manage order information.
Python Environment Setup for Shopify API Integration
Before making API calls, ensure your Python environment is properly configured. You'll need Python 3.x and the requests
library to handle HTTP requests. If you haven't installed the requests
library, you can do so using pip:
pip install requests
Retrieving Orders from Shopify API
To retrieve orders, you'll make a GET request to the Shopify API's orders endpoint. Here's a step-by-step guide:
- Ensure you have your access token ready, as obtained in the previous section.
- Set up the API endpoint URL for retrieving orders. The URL format is:
https://{store_name}.myshopify.com/admin/api/2024-07/orders.json
. - Include the access token in the request headers to authenticate your API call.
import requests
# Replace with your store name and access token
store_name = 'your-development-store'
access_token = 'your_access_token'
# Set the API endpoint
url = f'https://{store_name}.myshopify.com/admin/api/2024-07/orders.json'
# Set the request headers
headers = {
'Content-Type': 'application/json',
'X-Shopify-Access-Token': access_token
}
# Make the GET request to the Shopify API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
orders = response.json().get('orders', [])
for order in orders:
print(f"Order ID: {order['id']}, Total Price: {order['total_price']}")
else:
print(f"Failed to retrieve orders: {response.status_code} - {response.text}")
Verifying API Call Success and Handling Errors
After executing the code, you should see a list of orders printed in the console. If the request fails, the error message will be displayed. Shopify's API returns various status codes to indicate the result of your request:
- 200 OK: The request was successful, and the orders data is returned.
- 401 Unauthorized: Authentication failed. Check your access token.
- 429 Too Many Requests: Rate limit exceeded. Wait before retrying.
For more details on error codes, refer to the Shopify API documentation.
Handling Shopify API Rate Limits
Shopify's API has a rate limit of 40 requests per app per store per minute. If you exceed this limit, you'll receive a 429 status code. To handle rate limiting, monitor the X-Shopify-Shop-Api-Call-Limit
header in the response and implement a retry mechanism with exponential backoff if necessary.
For more information on rate limits, visit the Shopify API rate limits documentation.
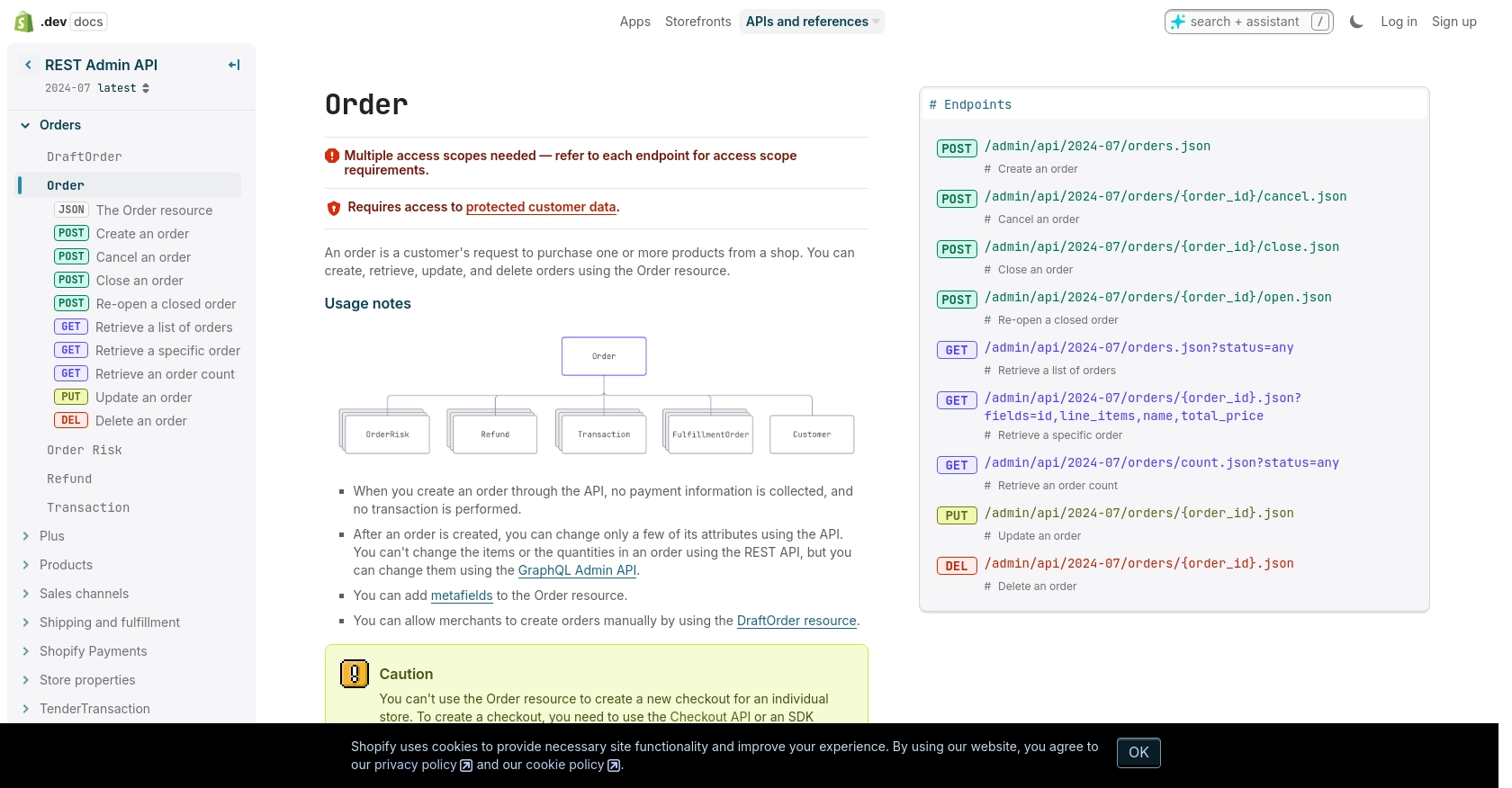
Conclusion and Best Practices for Shopify API Integration Using Python
Integrating with the Shopify API using Python provides a powerful way to automate and enhance your store management processes. By retrieving order data, you can streamline operations, gain insights into sales trends, and improve customer service.
Here are some best practices to consider when working with the Shopify API:
- Securely Store Credentials: Always store your API credentials securely. Use environment variables or secure vaults to keep your access tokens safe.
- Handle Rate Limits Gracefully: Shopify's API has a rate limit of 40 requests per app per store per minute. Implement a retry mechanism with exponential backoff to handle 429 errors effectively.
- Optimize Data Handling: When retrieving large datasets, consider using pagination to manage data efficiently and reduce load times.
- Standardize Data Formats: Ensure that the data retrieved from Shopify is transformed and standardized to fit your application's requirements.
By following these best practices, you can ensure a robust and efficient integration with Shopify's API, enhancing your store's capabilities and providing a better experience for your customers.
For developers looking to simplify and accelerate their integration processes, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Learn more at Endgrate.
Read More
Ready to get started?