Using the Zendesk Sell API to Get Users in PHP
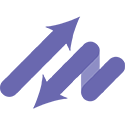
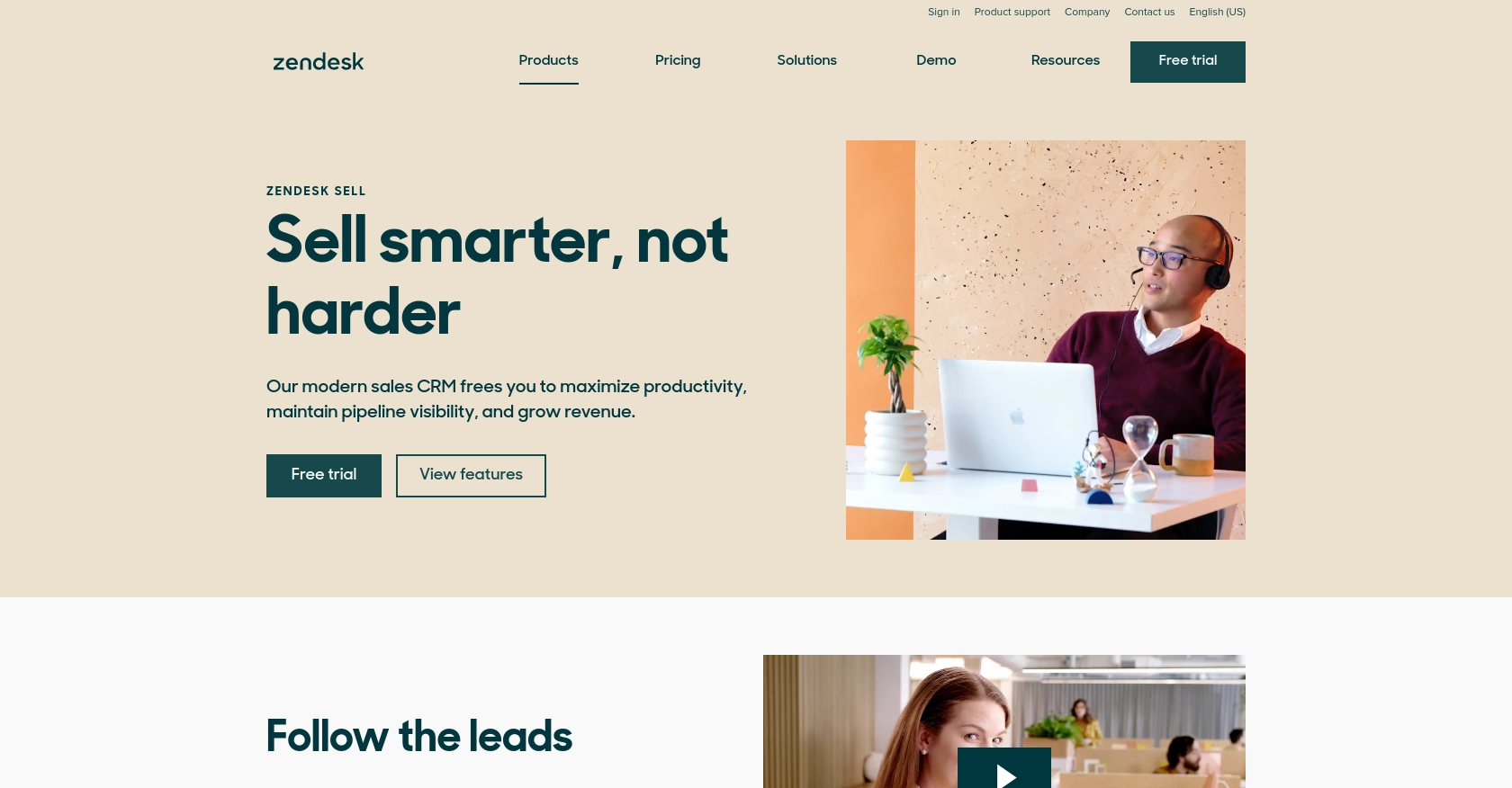
Introduction to Zendesk Sell API
Zendesk Sell is a powerful sales CRM platform designed to enhance productivity and streamline sales processes for businesses. It offers a comprehensive suite of tools that help sales teams manage leads, contacts, and deals efficiently. With its robust API, developers can integrate Zendesk Sell into their applications, enabling seamless data synchronization and automation of sales workflows.
Connecting with the Zendesk Sell API allows developers to access and manage user data, which can be crucial for building custom sales solutions. For example, a developer might use the API to retrieve user information and integrate it with a third-party analytics platform, providing deeper insights into sales team performance.
Setting Up a Zendesk Sell Test Account for API Integration
Before you can start using the Zendesk Sell API to retrieve user data, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting live data. Follow these steps to create and configure your Zendesk Sell account for API access.
Creating a Zendesk Sell Account
- Visit the Zendesk Sell website and sign up for a free trial or demo account.
- Follow the on-screen instructions to complete the registration process. Once registered, you will have access to the Zendesk Sell dashboard.
Configuring OAuth Authentication for Zendesk Sell API
The Zendesk Sell API uses OAuth 2.0 for authentication. This ensures secure access to your data. Follow these steps to set up OAuth authentication:
- Log in to your Zendesk Sell account and navigate to the Settings section.
- Under Integrations, select API & OAuth.
- Click on Create a new OAuth client to register your application.
- Fill in the required details, such as the client name and redirect URL. Ensure the redirect URL matches the one used in your application.
- Once created, you will receive a Client ID and Client Secret. Store these securely as they are needed for API requests.
Generating Access Tokens for Zendesk Sell API
With your OAuth client set up, you can now generate access tokens to authenticate API requests:
- Use the Authorization Code Flow to obtain an authorization code. Direct users to the following URL, replacing
$CLIENT_ID
and$CLIENT_REDIRECT_URI
with your values: - After authorization, exchange the authorization code for an access token by making a POST request to the token endpoint:
- Store the received access token securely. It will be used in the Authorization header for API requests.
https://api.getbase.com/oauth2/authorize?client_id=$CLIENT_ID&response_type=code&redirect_uri=$CLIENT_REDIRECT_URI
curl -X POST https://api.getbase.com/oauth2/token \
-u "$CLIENT_ID:$CLIENT_SECRET" \
-d "grant_type=authorization_code" \
-d "code=$AUTHORIZATION_CODE" \
-d "redirect_uri=$CLIENT_REDIRECT_URI"
With your Zendesk Sell account and OAuth authentication configured, you're now ready to start making API calls to retrieve user data.
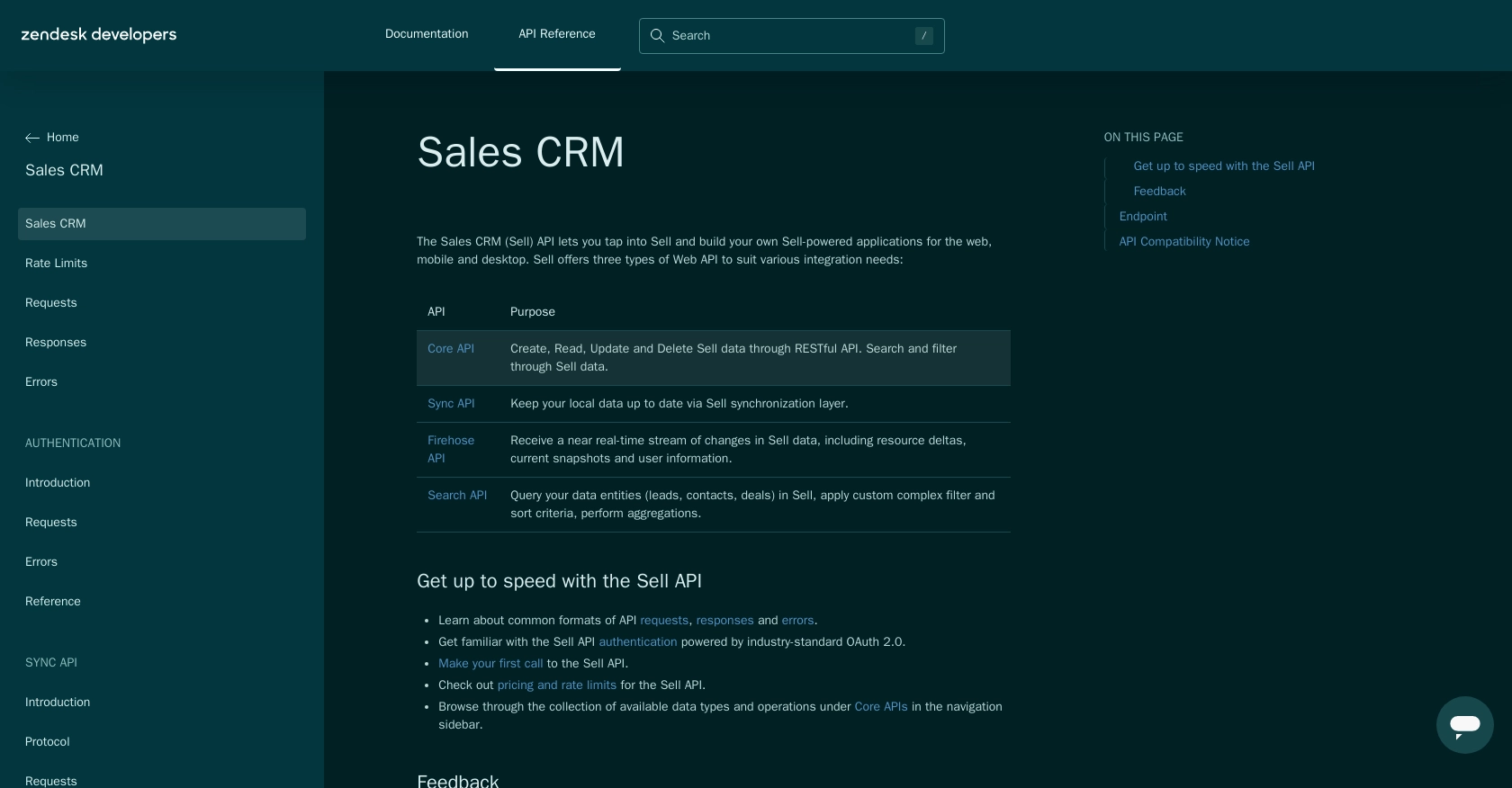
sbb-itb-96038d7
Making API Calls to Retrieve Users from Zendesk Sell Using PHP
To interact with the Zendesk Sell API and retrieve user data, you'll need to make HTTP requests using PHP. This section will guide you through the process of setting up your PHP environment, making the API call, and handling the response.
Setting Up Your PHP Environment for Zendesk Sell API
Before making API calls, ensure your PHP environment is properly configured:
- Ensure you have PHP 7.4 or later installed on your machine.
- Install the
cURL
extension for PHP, which is necessary for making HTTP requests.
Installing Required PHP Dependencies
To make HTTP requests, you can use the cURL
library. Ensure it's enabled in your php.ini
file:
extension=curl
Example Code to Retrieve Users from Zendesk Sell
Below is a sample PHP script to retrieve users from Zendesk Sell:
<?php
// Set the API endpoint and access token
$endpoint = "https://api.getbase.com/v2/users";
$accessToken = "Your_Access_Token";
// Initialize cURL session
$ch = curl_init();
// Set cURL options
curl_setopt($ch, CURLOPT_URL, $endpoint);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Bearer $accessToken",
"Accept: application/json"
]);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Decode the JSON response
$data = json_decode($response, true);
// Display user information
foreach ($data['items'] as $user) {
echo "Name: " . $user['data']['name'] . "\n";
echo "Email: " . $user['data']['email'] . "\n";
echo "Role: " . $user['data']['role'] . "\n\n";
}
}
// Close cURL session
curl_close($ch);
?>
Understanding the API Response
Upon successful execution, the API will return a JSON response containing user data. The script above decodes this JSON and prints user details such as name, email, and role.
Handling Errors and Verifying API Requests
It's crucial to handle potential errors when making API calls. Common HTTP status codes to watch for include:
- 200 OK: The request was successful.
- 401 Unauthorized: The access token is missing or invalid.
- 429 Too Many Requests: Rate limit exceeded. You can make up to 36,000 requests per hour (10 requests/token/second) as per Zendesk's rate limits documentation.
For more detailed error handling, refer to the Zendesk Sell API error documentation.
Verifying Successful Data Retrieval in Zendesk Sell
After executing the script, verify that the retrieved user data matches the information in your Zendesk Sell account. This ensures the API call was successful and the data is accurate.
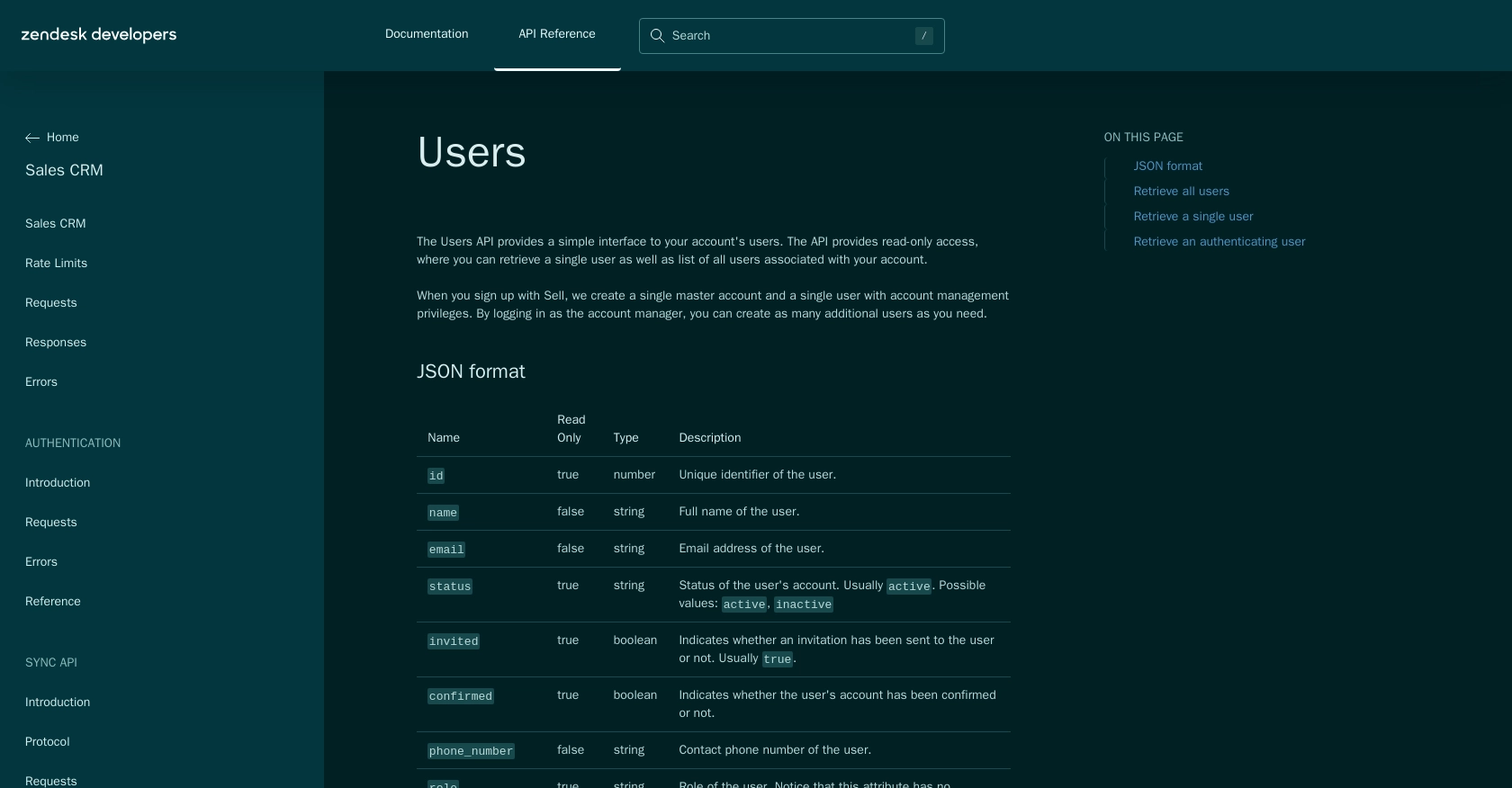
Best Practices for Using Zendesk Sell API in PHP
When integrating with the Zendesk Sell API, it's essential to follow best practices to ensure security, efficiency, and maintainability of your application. Here are some recommendations:
Securely Storing Zendesk Sell API Credentials
Always store your API credentials, such as the Client ID, Client Secret, and Access Token, securely. Consider using environment variables or a secure vault service to keep these sensitive details out of your codebase.
Handling Zendesk Sell API Rate Limits
Be mindful of the rate limits imposed by Zendesk Sell, which allow up to 36,000 requests per hour (10 requests/token/second). Implement logic to handle the 429 Too Many Requests
response code by retrying the request after a delay. For more information, refer to the Zendesk Sell API rate limits documentation.
Standardizing and Transforming Data from Zendesk Sell API
When retrieving data from the Zendesk Sell API, consider transforming and standardizing the data fields to match your application's data model. This will facilitate seamless integration and data consistency across your systems.
Streamlining Zendesk Sell Integrations with Endgrate
Building and maintaining integrations with multiple platforms can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zendesk Sell. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy and intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zendesksell
- https://developer.zendesk.com/api-reference/sales-crm/introduction/
- https://developer.zendesk.com/api-reference/sales-crm/rate-limits/
- https://developer.zendesk.com/api-reference/sales-crm/requests/
- https://developer.zendesk.com/api-reference/sales-crm/errors/
- https://developer.zendesk.com/api-reference/sales-crm/authentication/introduction/
- https://developer.zendesk.com/api-reference/sales-crm/authentication/requests/#client-authentication
- https://developer.zendesk.com/api-reference/sales-crm/resources/users/
Ready to get started?