Using the Constant Contact API to Get Contacts in PHP
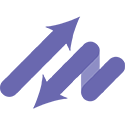
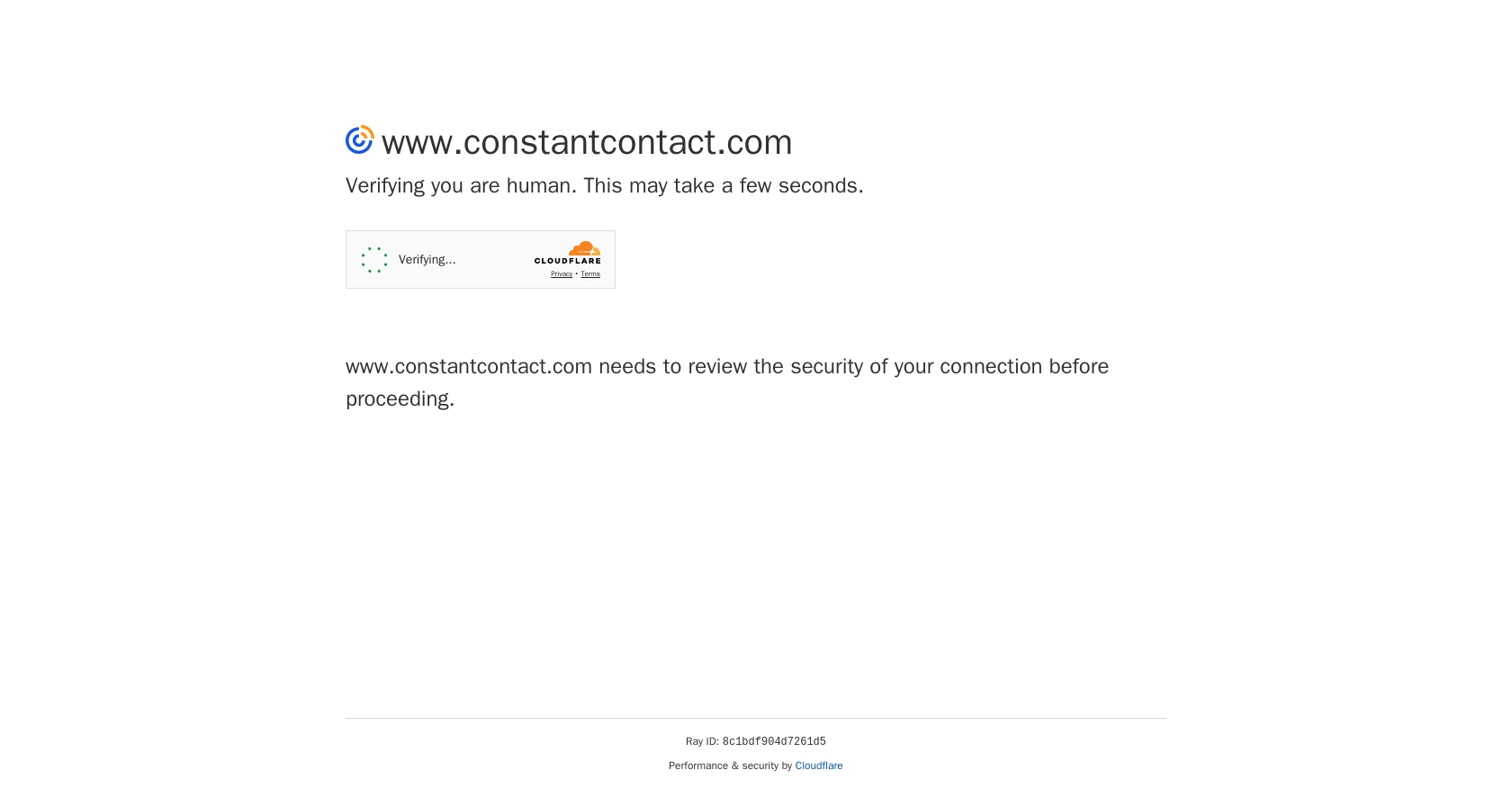
Introduction to Constant Contact API
Constant Contact is a powerful email marketing platform that enables businesses to create and manage effective email campaigns. With features like customizable templates, contact management, and detailed analytics, Constant Contact helps businesses engage with their audience and drive results.
Integrating with the Constant Contact API allows developers to automate and streamline email marketing processes. For example, you can use the API to retrieve contact information, enabling personalized email campaigns based on user behavior and preferences.
In this article, we will explore how to use PHP to interact with the Constant Contact API, specifically focusing on retrieving contact data. This integration can help developers enhance their marketing strategies by leveraging Constant Contact's robust features programmatically.
Setting Up Your Constant Contact Developer Account
Before you can start integrating with the Constant Contact API, you'll need to set up a developer account. This will allow you to create an application and obtain the necessary credentials for API access.
Follow these steps to set up your Constant Contact developer account:
- Visit the Constant Contact Developer Portal and sign up for a developer account.
- Once registered, log in to the portal and navigate to the "My Applications" section.
- Click on "Create a New Application" to start the process of setting up your app.
- Fill in the required details, such as the application name and description.
- Specify the redirect URI, which is the URL where users will be redirected after they authorize your application.
Creating a Constant Contact App for OAuth2 Authentication
Constant Contact uses OAuth2 for authentication, which requires you to create an app to obtain the client ID and client secret. Follow these steps to create your app:
- In the "My Applications" section, click on your newly created application.
- Under the "Authentication" tab, note down the client ID and client secret. These are crucial for authenticating API requests.
- Ensure you have set the appropriate scopes for your application. For accessing contacts, you will need the
contact_data
scope.
Generating Access Tokens for API Requests
To interact with the Constant Contact API, you'll need to generate an access token using the OAuth2 flow. Here's how:
- Direct users to the Constant Contact authorization endpoint with the required query parameters, including your client ID and desired scopes.
- Once users authorize your application, they will be redirected to your specified redirect URI with an authorization code.
- Exchange this authorization code for an access token by making a POST request to the Constant Contact token endpoint.
- Use the access token in the Authorization header of your API requests to authenticate.
For more details on OAuth2 authentication, refer to the Constant Contact OAuth2 Overview.
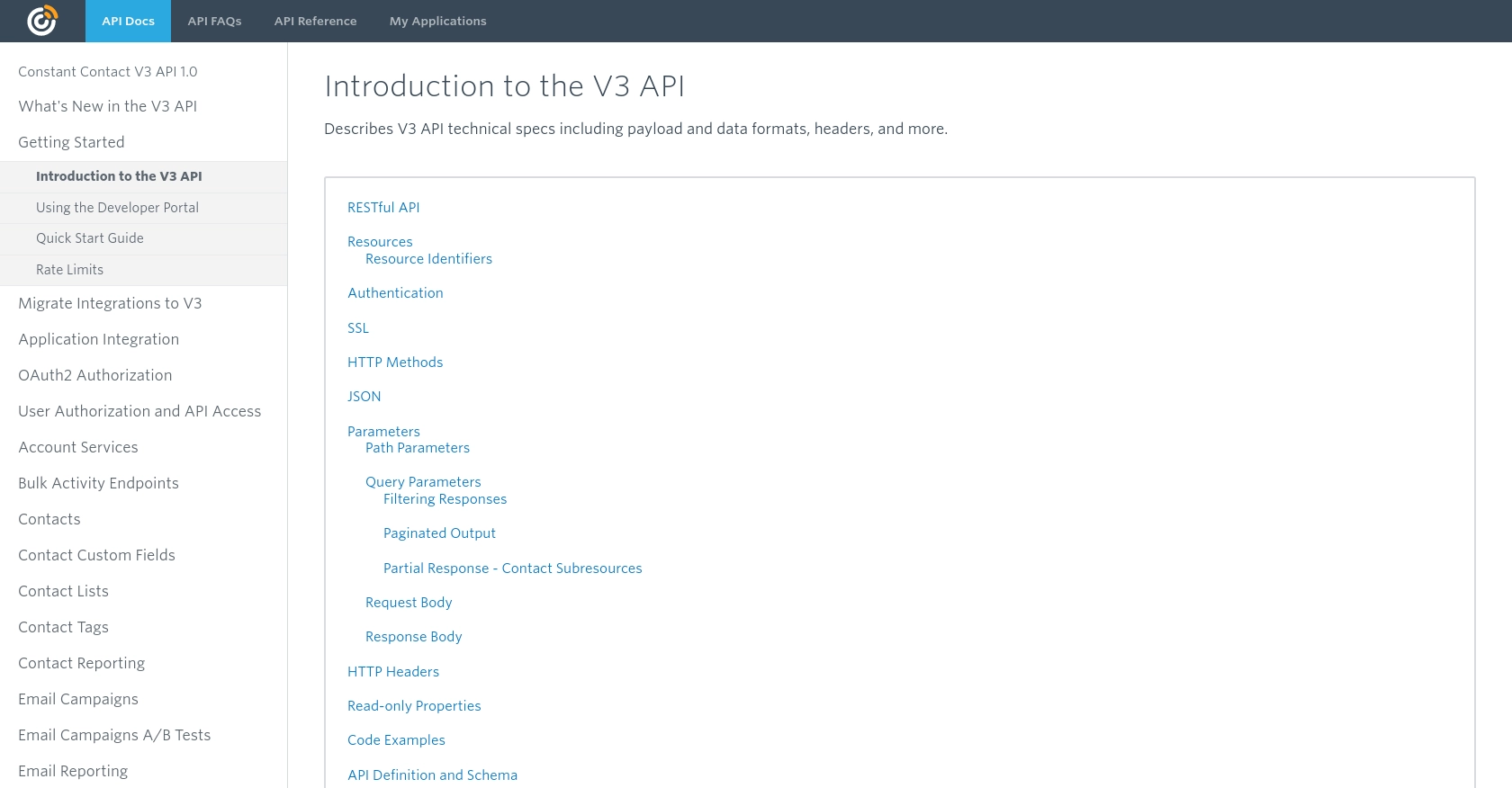
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Constant Contact Using PHP
To interact with the Constant Contact API and retrieve contact data using PHP, you'll need to set up your environment and write the necessary code to make API requests. This section will guide you through the process, ensuring you have everything you need to successfully connect to Constant Contact and fetch contact information.
Setting Up Your PHP Environment for Constant Contact API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need:
- PHP 7.4 or higher
- cURL extension enabled
To verify your PHP version and installed extensions, run the following command in your terminal:
php -v
Ensure cURL is enabled by checking your php.ini
file or running:
php -m | grep curl
Installing Required PHP Dependencies for Constant Contact API
To handle HTTP requests, you can use the Guzzle HTTP client. Install it via Composer:
composer require guzzlehttp/guzzle
Writing PHP Code to Fetch Contacts from Constant Contact API
With your environment set up, you can now write the PHP code to make an API call to Constant Contact and retrieve contacts. Create a new PHP file, get_contacts.php
, and add the following code:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token';
try {
$response = $client->request('GET', 'https://api.cc.email/v3/contacts', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/json',
],
]);
$contacts = json_decode($response->getBody(), true);
foreach ($contacts['contacts'] as $contact) {
echo 'Name: ' . $contact['first_name'] . ' ' . $contact['last_name'] . "\n";
echo 'Email: ' . $contact['email_address'] . "\n\n";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_Access_Token
with the access token you obtained during the OAuth2 authentication process.
Running the PHP Script and Verifying API Call Success
Execute the script from your terminal:
php get_contacts.php
If successful, you should see a list of contacts printed in your terminal. Verify the data by checking your Constant Contact account to ensure it matches the retrieved information.
Handling Errors and Understanding Constant Contact API Response Codes
When making API calls, it's crucial to handle potential errors. The Constant Contact API uses standard HTTP response codes to indicate success or failure:
- 200 OK: The request was successful.
- 401 Unauthorized: Invalid or expired access token. Generate a new token.
- 403 Forbidden: Insufficient permissions. Check your scopes.
- 429 Too Many Requests: Rate limit exceeded. Wait before retrying.
For more details on error handling, refer to the Constant Contact API Response Codes.
Conclusion and Best Practices for Using Constant Contact API with PHP
Integrating with the Constant Contact API using PHP can significantly enhance your email marketing strategies by automating contact management and personalizing campaigns. By following the steps outlined in this article, you can efficiently retrieve contact data and leverage Constant Contact's robust features programmatically.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Constant Contact imposes a rate limit of 10,000 requests per day and 4 requests per second. Implement logic to handle
429 Too Many Requests
errors by waiting before retrying. For more details, refer to the Constant Contact OAuth2 Overview. - Refresh Tokens Appropriately: Use refresh tokens to extend the lifespan of access tokens without requiring user reauthorization. Only request a new access token when the current one is expired or about to expire.
- Data Transformation and Standardization: Ensure that the data retrieved from Constant Contact is transformed and standardized according to your application's requirements for seamless integration.
Streamline Your Integrations with Endgrate
While integrating with Constant Contact API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Constant Contact. By using Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration experience by visiting Endgrate's website and discover how you can streamline your integration efforts efficiently.
Read More
- https://endgrate.com/provider/constantcontact
- https://developer.constantcontact.com/api_guide/v3_technical_overview.html
- https://developer.constantcontact.com/api_guide/auth_overview.html
- https://developer.constantcontact.com/api_guide/scopes.html
- https://developer.constantcontact.com/api_guide/glossary_responses.html
Ready to get started?